Python Basics Book Resources
Bonus resources, exercises, and errata for Python Basics: A Practical Introduction to Python 3. This is where you can find all of the bonus material for the book and leave your feedback. Thanks for getting a copy!
Key Bonus Resources
These are the key online resources that come with the book you should not miss out on:
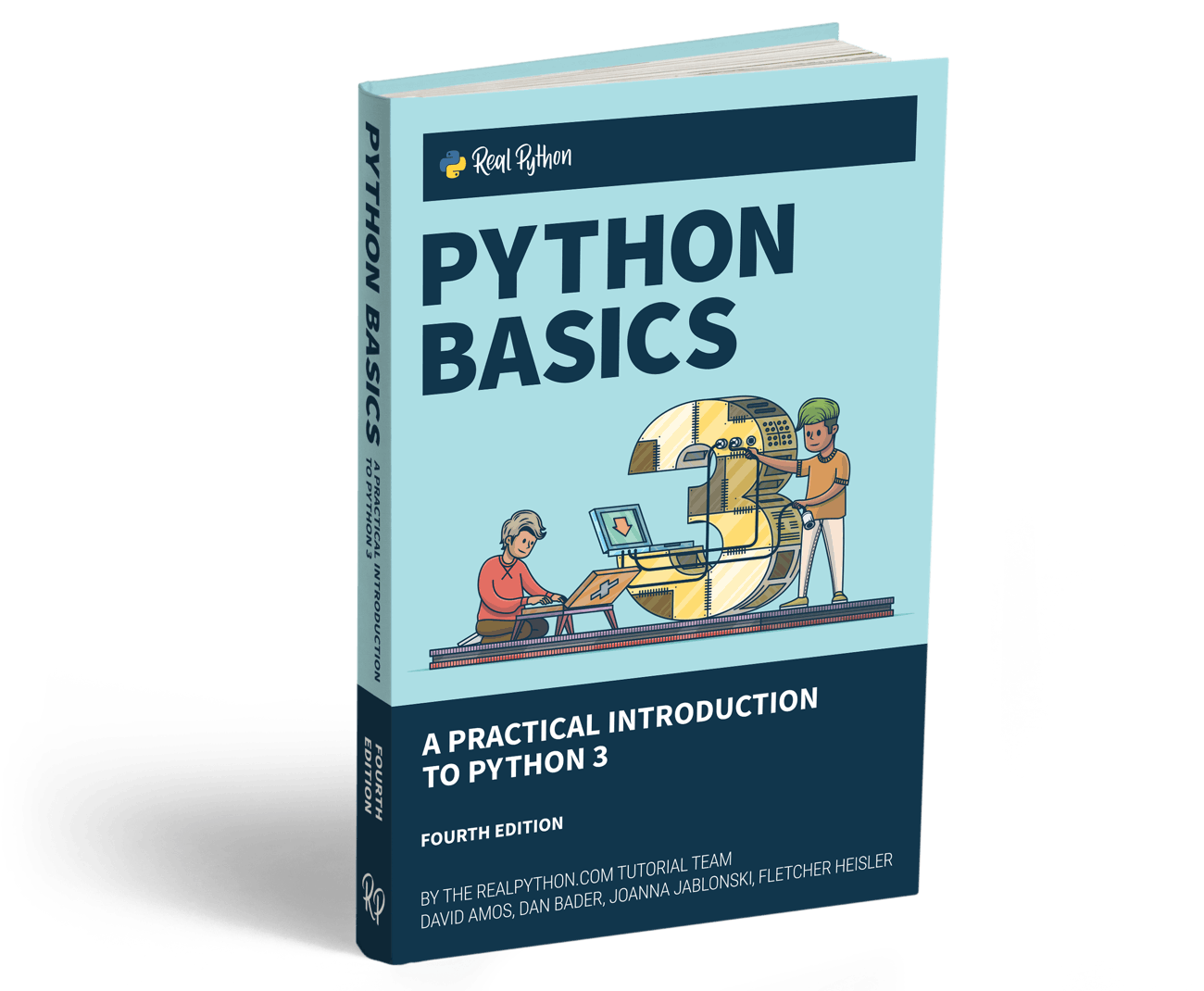
- Guided Tour / Learning Path
- On-Demand Courses for Python Basics
- Solutions for Exercises & Challenges
- Questions / Support / Getting Help
- Interactive Quizzes
- Recommended Resources
- Python Fundamentals
- Chapter 1: Introduction
- Chapter 2: Getting Started
- Chapter 3: Your First Python Program
- Chapter 4: Strings and Methods
- Chapter 5: Numbers in Python
- Chapter 6: Functions and Loops
- Chapter 7: Finding and Fixing Code Bugs
- Chapter 8: Conditional Logic and Control Flow
- Chapter 9: Tuples, Lists, and Dictionaries
- Chapter 10: Object-Oriented Programming (OOP)
- Chapter 11: Modules and Packages
- Chapter 12: File Input and Output
- Chapter 13: Installing Packages With Pip
- Chapter 14: Creating and Modifying PDF Files
- Chapter 15: Working With Databases
- Chapter 16: Interacting With the Web
- Chapter 17: Scientific Computing and Graphing
- Chapter 18: Graphical User Interfaces
- Chapter 19: Final Thoughts and Next Steps
- Errata
- Feedback
Last Updated: May 31, 2024
Note: If you don’t own a copy of our book Python Basics: A Practical Introduction to Python 3. You can learn more about the book and purchase your copy by clicking here.
Guided Tour / Learning Path
This learning path is meant to accompany our Python Basics book. It will guide you through the chapters, quizzes, and exercises in the book:
Learning Path
Python Basics Book (Supporting Materials & Bonus Resources)
19 Resources β Skills: Python 3 Fundamentals, Real-World Projects
On-Demand Courses for Python Basics
We’ve also adapted the chapters in the book into a series of on-demand video courses, available for Real Python members. With the course series you’ll learn by doing, with the guidance of experienced instructors showing exactly what to do through screen recordings:
Learning Path
Python Basics: Introduction to Python
17 Resources β Skills: Python 3 Fundamentals, Real-World Projects
Solutions for Exercises & Challenges
The book comes with a full set of coding exercises and project-based assignments. You can find the exercises and code challenges at the end of many sections in the book.
You can access and download solutions and sample implementations from the realpython/python-basics-exercises
GitHub repository.
Alternatively, you can download a .zip archive with the exercise solutions by clicking the button below.
Browse exercise solutions Download as .zip file
Questions / Support / Getting Help
Got Python questions, want to share your exercise solutions, or just chat with a group of likeminded learners? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session with an expert from the RP Team. We’ll see you there ππ
Interactive Quizzes
Most chapters in this book come with a free online quiz to check your learning progress.
How to Access the Quizzes Associated with the Book
You can access the quizzes using the links provided at the end of the chapter or in the learning path linked above. The quizzes are hosted on the Real Python website and can be viewed on your phone or computer.
How Real Python Quizzes Work
Each quiz takes you through a series of questions related to a particular chapter in the book. Some of them are multiple choice, some will ask you to type in an answer, and for some you’ll be asked to write actual Python code. As you make your way through each quiz, it keeps score on which questions you answered correctly.
At the end of the quiz you receive a grade based on your result. If you don’t score a 100% on your first try—don’t fret! These quizzes are meant to challenge you and it’s expected that you go through them several times, improving your score with each run.
We intended these quizzes as a fun way for you to check your learning progress as you work your way through the book. They will help you identify weak spots in your Python knowledge. Use this to figure out which chapters and exercises in the book you should re-read. Once you can complete the full set of quizzes for the book with a 100% score you should feel proud of yourself. You’re a real Pythonista now!
Use your mouse or a touch-screen device to complete each quiz. If you prefer using keyboard controls, the following shortcuts are also available:
- β / β to move the cursor selection up or down
- Space to select the item at the cursor
- Enter to submit your answer or move on to the next question
Recommended Resources
Links and additional learning resources we recommend to further deepen your Python skills. We will update and expand this over time.
Note: If you find a helpful link or resource that should be featured here, please send it to us via the feedback form so we can share it with your fellow Pythonistas. Thanks!
Python Fundamentals
Learning Path
Revisit Python Fundamentals
12 Resources β Skills: Python, Variables, Data Types, REPL, Operators, Expressions, Exceptions, Comments, Keywords, IDLE, String Conversion
Chapter 1: Introduction
Chapter 2: Getting Started
- Python 3 Installation & Setup Guide
- Python IDEs and Code Editors (Guide)
- IDLE Documentation
- Online Python REPLs:
Chapter 3: Your First Python Program
- 11 Beginner Tips for Learning Python Programming
- Interacting with Python
- Variables in Python
- Writing Comments in Python (Guide)
- Python Application Layouts: A Reference
Chapter 4: Strings and Methods
- Strings and Character Data in Python
- Python String Formatting Best Practices
- Python 3’s f-Strings: An Improved String Formatting Syntax (Guide)
- Splitting, Concatenating, and Joining Strings in Python
Chapter 5: Numbers in Python
- Basic Data Types in Python
- How to Round Numbers in Python
- “How much math do I need to know to program?” Not That Much, Actually.
- PEP 8
Chapter 6: Functions and Loops
- Python’s
range()
Function (Guide) - Python “while” Loops (Indefinite Iteration)
- Functions as First-Class Objects in Python
Chapter 7: Finding and Fixing Code Bugs
- Python Debugging With Pdb
- For some great examples of refactoring, check out the following YouTube videos: Transforming Code into Beautiful, Idiomatic Python by Raymond Hettinger, and Refactoring Python by Brett Slatkin.
Chapter 8: Conditional Logic and Control Flow
Chapter 9: Tuples, Lists, and Dictionaries
Chapter 10: Object-Oriented Programming (OOP)
- Official Python documentation
- OOP Articles on Real Python
- Intro to Object-Oriented Programming (OOP) in Python (Course)
- OOP Criticisms
Chapter 11: Modules and Packages
- Python Modules and Packages Course
- Absolute and Relative Imports
- Managing Python Dependencies Course
Chapter 12: File Input and Output
- Reading and Writing Files in Python (Guide)
- Working With Files in Python
- Reading and Writing CSV Files in Python
- Python 3’s
pathlib
Module: Taming the File System - Escape Characters
Chapter 13: Installing Packages With Pip
- Managing Python Dependencies Course
- Python Virtual Environments: A Primer
- Pipenv: A Guide to the New Python Packaging Tool
Chapter 14: Creating and Modifying PDF Files
Chapter 15: Working With Databases
Chapter 16: Interacting With the Web
- Mozilla Developer Network: HTML
- Practical Introduction to Web Scraping in Python
- API Integration in Python
Chapter 17: Scientific Computing and Graphing
Chapter 18: Graphical User Interfaces
Chapter 19: Final Thoughts and Next Steps
- Real Python Newsletter
- PyCoderβs Weekly
- PythonistaCafe
- StackOverflow
- The Hitchhiker’s Guide to Python
- Real Python Tutorials
- Real Python “Office Hours” Live Q&A Sessions
- Real Python Community Chat
Errata
Here we’ll list any errata, bugs, and corrections for the book.
- Page 327, Chapter 12.2 “Working With File Paths in Python”, under “Using the / Operator” the description text refers to a
Documents
subdirectory, but the code example shows aDesktop
subdirectory. The name of the subdirectory should be the same in both cases. Reported by Peter Matulis. - Page 342, Chapter 12.3 “Common File System Operations”, the code example shows the output of
list(new_dir.glob("?older_?"))
and showsfolder_a
andfolder_c
getting selected, but the description text below the code example mentions “folder_a/
andfolder_b/
” instead of “folder_a
andfolder_c/
” as the selected directories. Reported by Peter Matulis. - Page 447, Chapter 15.1 “An Introduction to SQLite”, code snippet defines
create_table
but then misspells it ascrate_table
further down. Reported by Edward Plant.
Any issues found we generally fix right away in the ebook version available on Real Python. You can always find the latest version of the ebook files under “My Purchases” in your realpython.com account.
Feedback
We welcome ideas, suggestions, feedback, and the occasional rant about the book. Did you find a topic confusing? Or did you find an error in the text or code? Did we omit a topic you would love to know more about? Drop us a line!
Your feedback really helps make this book an even better learning resource for Pythonistas around the globe.
Whatever the reason, good or bad, please send in your feedback at the link below: