Functions as First-Class Objects in Python
What does “Functions as First Class Objects” mean? You’ll see in this lesson that it means that functions can be passed around into lists and used as arguments for other functions. In the next lesson you’ll learn how to define functions inside functions.
00:00 When I say that functions are first-class objects, I mean that they can be used as arguments also, just like any other object—a string, an integer, a float, a list, and so forth. Let me have you dive into that.
00:12
Let’s make a couple quick functions and see what can be done. These are two printing functions that are slightly different. The first one, say_hello()
, is going to use an f-string and return it saying f"Hello "
with curly braces around the argument. To call the function, say_hello()
, and then put in a name
.
00:34 The second function is a little more flowery.
00:45
I’m going to have you call it in the same way. Let’s try that with "Gregor"
. So these objects say_hello
and be_awesome
—they’re both functions. Could they be put into a list? Let’s make a list.
00:57
my_list
, square brackets, put say_hello
in, comma, and then be_awesome
. Sure! Can you call them out of it? For the first index, yup—there’s say_hello
.
01:11
What if you wanted to call one of them? Can you put your arguments in just after where you’re pulling it out of the list? Sure! Let’s tell 'Thomas'
how awesome we are.
01:24 Great. If a function can be put into a list and called out of a list the same way that any other variable can, can a function be put into a function as an argument?
01:36
Can one function literally call another function? Let’s try it out. For the next function—I’ll have you call it greet_bob()
—and greet_bob()
takes a function, some form of a greeter function, as its argument.
01:48
So instead of a name it’s saying, “Well, what function do you want?” and then it returns that greeter function with this name, "Bob"
. So it’s going to plug "Bob"
into whatever greeter function as an argument.
02:04
So, how would you use it? greet_bob
itself is a function, so to use greet_bob()
, you enter in the function you want. You have two to choose from. say_hello
—you don’t need to put an argument here, it’s going to get it here.
02:22
So you just passed a function, say_hello
, into another function, and called it. You just accomplished some pretty cool stuff.
02:34
So this one, I’ll have you say, be_awesome
, and Bob will get the awesome greeting.
02:42
So again, what are you referencing? You created this function named say_hello()
, and be_awesome()
is your other function. They’re saved at those memory locations.
02:55 When you reference them, here, it’s calling them into whichever one has been picked. Let’s go to the next level of inception and talk about functions inside of functions.
bhaskarj4 on Dec. 25, 2020
What does return f
do?
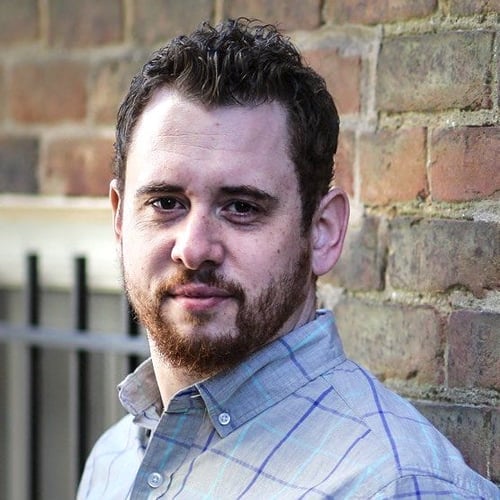
Ricky White RP Team on Dec. 27, 2020
Hi @bhaskarj4. The f at the beginning of the quotes is how you denote an f-string in Python. This is one way in which you can format a string. To learn more about f-strings you can use this course: realpython.com/courses/formatting-python-strings/
shuhuali1010 on March 7, 2021
What is a first-class object in Python? Is there a tutorial about it? I have watched OOP in Python and it wasn’t covered.
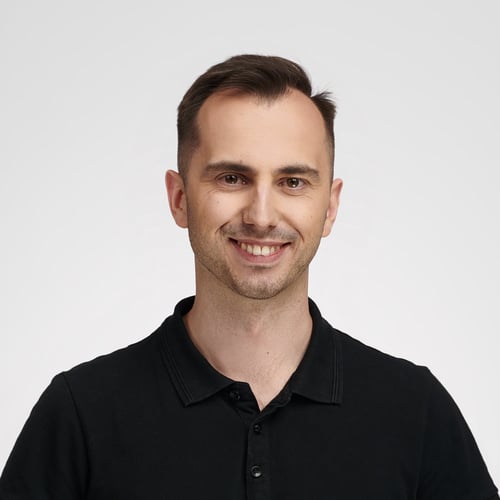
Bartosz Zaczyński RP Team on March 8, 2021
@shuhuali1010 The phrase first-class object or first-class citizen is informal jargon, which isn’t specific to Python. Essentially, it’s a fancy way of saying that functions behave the same as regular values like strings or numbers.
For example, you can assign a function to a variable and use that variable to call it:
>>> def add(x, y):
... return x + y
...
>>> operation = add
>>> operation(2, 3)
5
You can also pass a function to another function as a parameter:
>>> veggies = ["eggplant", "corn", "tomato"]
>>> sorted(veggies, key=len)
['corn', 'tomato', 'eggplant']
Finally, you can return a function from a so-called factory function:
>>> def make_adder(amount):
... def add(x):
... return x + amount
... return add
...
>>> add3 = make_adder(3)
>>> add3(2)
5
It’s a common way of defining decorators in Python.
Maram-dev on April 27, 2021
Amazing! Now I can understand functions!!! Thank you Chris! :)
sndselecta on Jan. 4, 2024
As @Paul M, mentioned. What’s the rule of thumb when not to include the () in a function. When a function is assigned to a variable, or used as an argument in another function or used in an iterable? Or more simply only use the () when called?
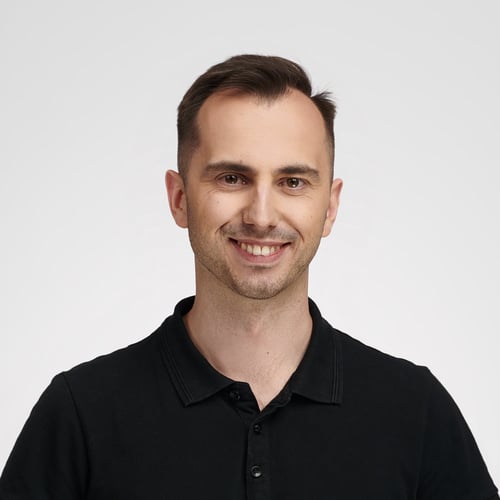
Bartosz Zaczyński RP Team on Jan. 4, 2024
@sndselecta Your last sentence sums it up, i.e., you’d only append the parens when calling the function. Otherwise, you can use the function name as a reference to call later from a different place in your code.
Ed Schneider on Jan. 4, 2024
This issue, when to and when not to use parens with function names, was discussed extensively in the Dec 20th office hours. See discussion in Slack under the office hours channel.
sndselecta on Jan. 4, 2024
I’m a bit confused. Where is the association between the greeter_func()
as well as its argument and the other two functions? I would have expected an undefined error, since greeter_func()
has never been defined globally or locally.
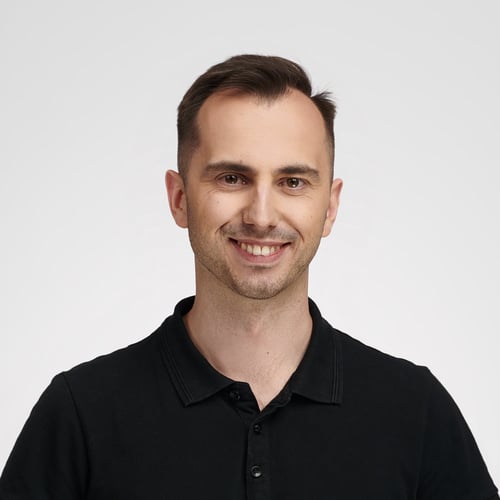
Bartosz Zaczyński RP Team on Jan. 4, 2024
@sndselecta There’s no formal association becuase it’s all happening at runtime. greeter_func
is an arbitrarily chosen name for the greet_bob()
function’s only argument. It allows you to access a callable object that was passed into the greet_bob()
function when someone called that function. In this case, greet_bob()
proxies the call to whatever function gets passed as an argument and immediately returns with the value to the caller.
Perhaps this code snippet will help you see the whole picture better:
>>> def greet_bob(greeter_func):
... return greeter_func("Bob")
...
>>> def say_hello(name):
... return f"Hello, {name}"
...
>>> def say_bye(name):
... return f"Bye, {name}"
...
>>> greet_bob(say_hello)
'Hello, Bob'
>>> greet_bob(say_bye)
'Bye, Bob'
You start by defining a higher-level function, greet_bob()
, which accepts another function as an argument. Then, you proceed to define two other functions that return a relevant message. Finally, you call your higher-level function with the two functions to print different messages to Bob.
sndselecta on Jan. 4, 2024
Oh I see it now, association → the greeter_func
doesn’t have to be defined as it is defined as an argument.
Become a Member to join the conversation.
Paul M on Aug. 11, 2020
One thing that might be helpful to point out to readers here is that if your application passes or uses an function as an object, make sure not to actually call the function (i.e. include the
()
). Something like:This tripped me up in a QT application and was not fun to debug…