At this point, you should have a working Python 3 interpreter at hand. If you need help getting Python set up correctly, please refer to the previous section in this tutorial series.
Here’s what you’ll learn in this tutorial: Now that you have a working Python setup, you’ll see how to actually execute Python code and run Python programs. By the end of this article, you’ll know how to:
- Use Python interactively by typing code directly into the interpreter
- Execute code contained in a script file from the command line
- Work within a Python Integrated Development Environment (IDE)
It’s time to write some Python code!
Hello, World!
There is a long-standing custom in the field of computer programming that the first code written in a newly installed language is a short program that simply displays the string Hello, World!
to the console.
Note: This is a time-honored tradition dating back to the 1970s. See Hello, World! for a brief history. You seriously risk upsetting the qi of the universe if you don’t abide by this custom.
The simplest Python 3 code to display Hello, World!
is:
print("Hello, World!")
You will explore several different ways to execute this code below.
Using the Python Interpreter Interactively
The most straightforward way to start talking to Python is in an interactive Read-Eval-Print Loop (REPL) environment. That simply means starting up the interpreter and typing commands to it directly. The interpreter:
- Reads the command you enter
- Evaluates and executes the command
- Prints the output (if any) to the console
- Loops back and repeats the process
The session continues in this manner until you instruct the interpreter to terminate. Most of the example code in this tutorial series is presented as REPL interaction.
Starting the Interpreter
In a GUI desktop environment, it is likely that the installation process placed an icon on the desktop or an item in the desktop menu system that starts Python.
For example, in Windows, there will likely be a program group in the Start menu labeled Python 3.x, and under it a menu item labeled Python 3.x (32-bit), or something similar depending on the particular installation you chose.
Clicking on that item will start the Python interpreter:
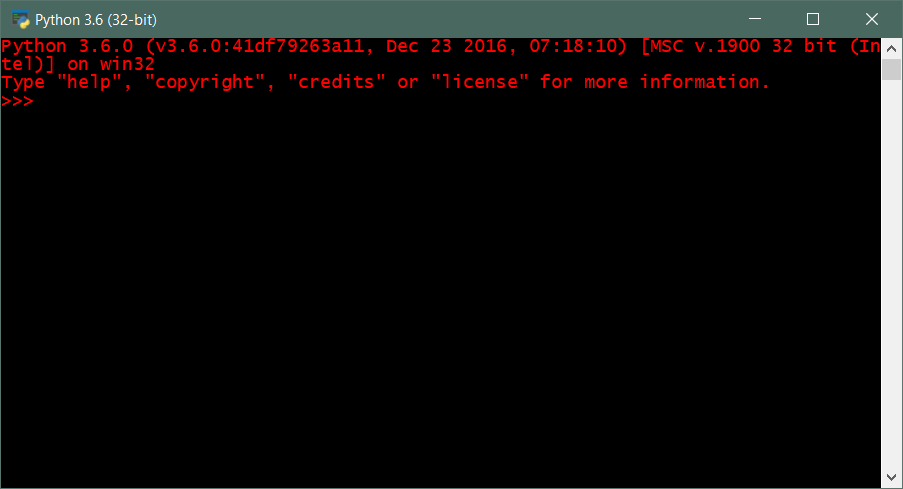
Alternatively, you can open a terminal window and run the interpreter from the command line. How you go about opening a terminal window varies depending on which operating system you’re using:
- In Windows, it is called Command Prompt.
- In macOS or Linux, it should be called Terminal.
Using your operating system’s search function to search for “command” in Windows or “terminal” in macOS or Linux should find it.
Once a terminal window is open, if paths have been set up properly by the Python install process, you should be able to just type python
. Then, you should see a response from the Python interpreter.
This example is from the Windows Command Prompt window:
C:\Users\john>python
Python 3.6.0 (v3.6.0:41df79263a11, Dec 23 2016, 07:18:10) [MSC v.1900 32 bit (Intel)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>>
Technical Note: If you are on a Linux system and installed Python 3, it may be that both Python 2 and Python 3 are installed. In that case, it is possible that typing python
at the prompt will start Python 2. Starting Python 3 may require typing something else, like python3
.
If you installed a more recent version of Python 3 than the one that was included in the distribution, you may even need to specify the version you installed specifically—for example python3.6
.
If you are not seeing the >>>
prompt, then you are not talking to the Python interpreter. This could be because Python is either not installed or not in your terminal window session’s path. It’s also possible that you just haven’t found the correct command to execute it. You can refer to our installing Python tutorial for help.
Executing Python Code
If you are seeing the prompt, you’re off and running! The next step is to execute the statement that displays Hello, World!
to the console:
- Ensure that the
>>>
prompt is displayed, and the cursor is positioned after it. - Type the command
print("Hello, World!")
exactly as shown. - Press the Enter key.
The interpreter’s response should appear on the next line. You can tell it is console output because the >>>
prompt is absent:
>>> print("Hello, World!")
Hello, World!
If your session looks like the above, then you have executed your first Python code! Take a moment to celebrate.
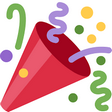
Did something go wrong? Perhaps you made one of these mistakes:
-
You forgot to enclose the string to be printed in quotation marks:
PythonCopied!>>> print(Hello, World!) File "<stdin>", line 1 print(Hello, World!) ^ SyntaxError: invalid syntax
-
You remembered the opening quotation mark but forgot the closing one:
PythonCopied!>>> print("Hello, World!) File "<stdin>", line 1 print("Hello, World!) ^ SyntaxError: EOL while scanning string literal
-
You used different opening and closing quotation marks:
PythonCopied!>>> print("Hello, World!') File "<stdin>", line 1 print("Hello, World!') ^ SyntaxError: EOL while scanning string literal
-
You forgot the parentheses:
PythonCopied!>>> print "Hello, World!" File "<stdin>", line 1 print "Hello, World!" ^ SyntaxError: Missing parentheses in call to 'print'
-
You entered extra whitespace characters before the command:
PythonCopied!>>> print("Hello, World!") File "<stdin>", line 1 print("Hello, World!") ^ IndentationError: unexpected indent
(You will see in an upcoming section why this matters.)
If you got some sort of error message, go back and verify that you typed the command exactly as shown above.
Exiting the Interpreter
When you are finished interacting with the interpreter, you can exit a REPL session in several ways:
-
Type
exit()
and press Enter:PythonCopied!>>> exit() C:\Users\john>
-
In Windows, type Ctrl+Z and press Enter:
PythonCopied!>>> ^Z C:\Users\john>
-
In Linux or macOS, type Ctrl+D. The interpreter terminates immediately; pressing Enter is not needed.
- If all else fails, you can simply close the interpreter window. This isn’t the best way, but it will get the job done.
Running a Python Script from the Command Line
Entering commands to the Python interpreter interactively is great for quick testing and exploring features or functionality.
Eventually though, as you create more complex applications, you will develop longer bodies of code that you will want to edit and run repeatedly. You clearly don’t want to re-type the code into the interpreter every time! This is where you will want to create a script file.
A Python script is a reusable set of code. It is essentially a Python program—a sequence of Python instructions—contained in a file. You can run the program by specifying the name of the script file to the interpreter.
Python scripts are just plain text, so you can edit them with any text editor. If you have a favorite programmer’s editor that operates on text files, it should be fine to use. If you don’t, the following are typically installed natively with their respective operating systems:
- Windows: Notepad
- Unix/Linux: vi or vim
- macOS: TextEdit
Using whatever editor you’ve chosen, create a script file called hello.py
containing the following:
print("Hello, World!")
Now save the file, keeping track of the directory or folder you chose to save into.
Start a command prompt or terminal window. If the current working directory is the same as the location in which you saved the file, you can simply specify the filename as a command-line argument to the Python interpreter: python hello.py
For example, in Windows it would look like this:
C:\Users\john\Documents\test>dir
Volume in drive C is JFS
Volume Serial Number is 1431-F891
Directory of C:\Users\john\Documents\test
05/20/2018 01:31 PM <DIR> .
05/20/2018 01:31 PM <DIR> ..
05/20/2018 01:31 PM 24 hello.py
1 File(s) 24 bytes
2 Dir(s) 92,557,885,440 bytes free
C:\Users\john\Documents\test>python hello.py
Hello, World!
If the script is not in the current working directory, you can still run it. You’ll just have to specify the path name to it:
C:\>cd
C:\
C:\>python c:\Users\john\Documents\test\hello.py
Hello, World!
In Linux or macOS, your session may look more like this:
jfs@jfs-xps:~$ pwd
/home/jfs
jfs@jfs-xps:~$ ls
hello.py
jfs@jfs-xps:~$ python hello.py
Hello, World!
A script file is not required to have a .py
extension. The Python interpreter will run the file no matter what it’s called, so long as you properly specify the file name on the command line:
jfs@jfs-xps:~$ ls
hello.foo
jfs@jfs-xps:~$ cat hello.foo
print("Hello, World!")
jfs@jfs-xps:~$ python hello.foo
Hello, World!
But giving Python files a .py
extension is a useful convention because it makes them more easily identifiable. In desktop-oriented folder/icon environments like Windows and macOS, this will also typically allow for setting up an appropriate file association, so that you can run the script just by clicking its icon.
Interacting with Python through an IDE
An Integrated Development Environment (IDE) is an application that more or less combines all the functionality you have seen so far. IDEs usually provide REPL capability as well as an editor with which you can create and modify code to then submit to the interpreter for execution.
You may also find cool features such as:
- Syntax highlighting: IDEs often colorize different syntax elements in the code to make it easier to read.
- Context-sensitive help: Advanced IDEs can display related information from the Python documentation or even suggested fixes for common types of code errors.
- Code-completion: Some IDEs can complete partially typed pieces of code (like function names) for you—a great time-saver and convenience feature.
- Debugging: A debugger allows you to run code step-by-step and inspect program data as you go. This is invaluable when you are trying to determine why a program is behaving improperly, as will inevitably happen.
IDLE
Most Python installations contain a rudimentary IDE called IDLE. The name ostensibly stands for Integrated Development and Learning Environment, but one member of the Monty Python troupe is named Eric Idle, which hardly seems like a coincidence.
The procedure for running IDLE varies from one operating system to another.
Starting IDLE in Windows
Go to the Start menu and select All Programs or All Apps. There should be a program icon labeled IDLE (Python 3.x 32-bit) or something similar. This will vary slightly between Win 7, 8, and 10. The IDLE icon may be in a program group folder named Python 3.x. You can also find the IDLE program icon by using the Windows search facility from the start menu and typing in IDLE
.
Click on the icon to start IDLE.
Starting IDLE in macOS
Open Spotlight Search. Typing Cmd+Space is one of several ways to do this. In the search box, type terminal
and press Enter.
In the terminal window, type idle3
and press Enter.
Starting IDLE in Linux
IDLE is available with the Python 3 distribution but may not have been installed by default. To find out whether it is, open a terminal window. This varies depending on the Linux distribution, but you should be able to find it by using the desktop search function and searching for terminal
. In the terminal window, type idle3
and press Enter.
If you get an error saying command not found
or something to that effect, then IDLE is apparently not installed, so you’ll need to install it.
The method for installing apps also varies from one Linux distribution to the next. For example, with Ubuntu Linux, the command to install IDLE is sudo apt-get install idle3
. Many Linux distributions have GUI-based application managers that you can use to install apps as well.
Follow whatever procedure is appropriate for your distribution to install IDLE. Then, type idle3
in a terminal window and press Enter to run it. Your installation procedure may have also set up a program icon somewhere on the desktop to start IDLE as well.
Whew!
Using IDLE
Once IDLE is installed and you have started it successfully, you should see a window titled Python 3.x.x Shell, where 3.x.x corresponds to your version of Python:
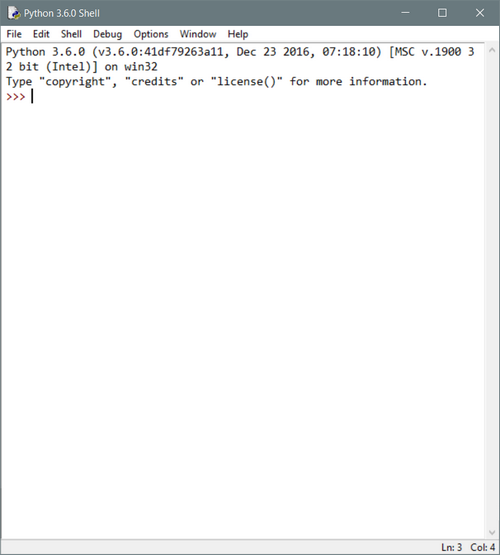
The >>>
prompt should look familiar. You can type REPL commands interactively, just like when you started the interpreter from a console window. Mindful of the qi of the universe, display Hello, World!
again:
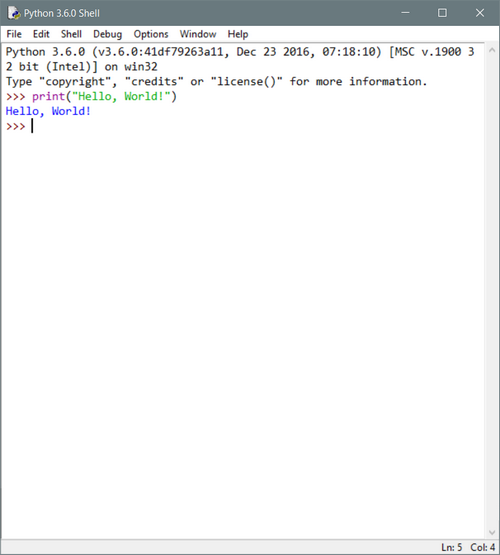
The interpreter behaves more or less the same as when you ran it directly from the console. The IDLE interface adds the perk of displaying different syntactic elements in distinct colors to make things more readable.
It also provides context-sensitive help. For example, if you type print(
without typing any of the arguments to the print function or the closing parenthesis, then flyover text should appear specifying usage information for the print()
function.
One other feature IDLE provides is statement recall:
- If you have typed in several statements, you can recall them with Alt+P and Alt+N in Windows or Linux.
- Alt+P cycles backward through previously executed statements; Alt+N cycles forward.
- Once a statement has been recalled, you can use editing keys on the keyboard to edit it and then execute it again. The corresponding commands in macOS are Cmd+P and Cmd+N.
You can also create script files and run them in IDLE. From the Shell window menu, select File → New File. That should open an additional editing window. Type in the code to be executed:
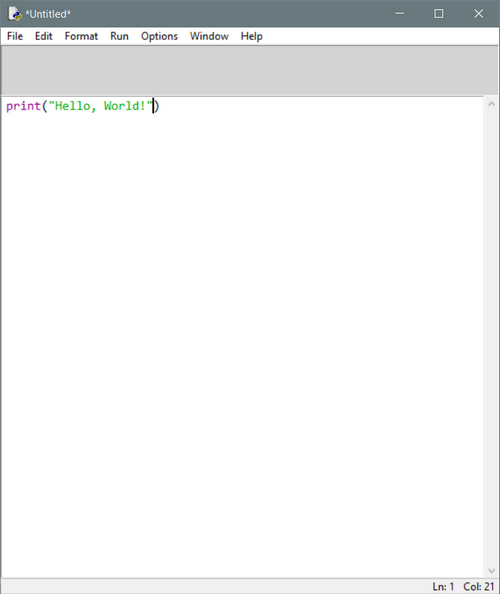
From the menu in that window, select File → Save or File → Save As… and save the file to disk. Then select Run → Run Module. The output should appear back in the interpreter Shell window:
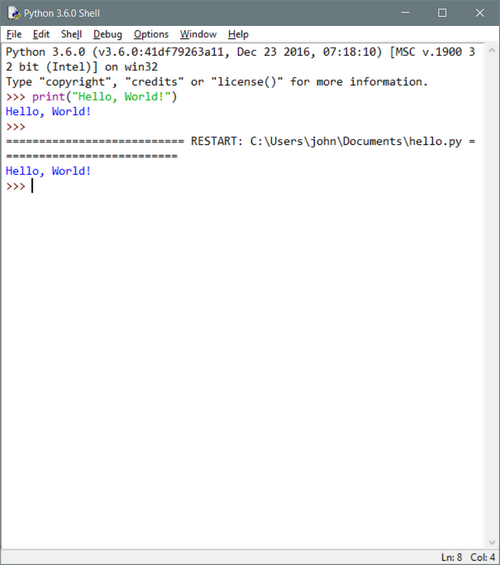
OK, that’s probably enough Hello, World!
. The qi of the universe should be safe.
Once both windows are open, you can switch back and forth, editing the code in one window, running it and displaying its output in the other. In that way, IDLE provides a rudimentary Python development platform.
Although it is somewhat basic, it supports quite a bit of additional functionality, including code completion, code formatting, and a debugger. See the IDLE documentation for more details.
Thonny
Thonny is free Python IDE developed and maintained by the Institute of Computer Science at the University of Tartu, Estonia. It is targeted at Python beginners specifically, so the interface is simple and uncluttered as well as easy to understand and get comfortable with quickly.
Like IDLE, Thonny supports REPL interaction as well as script file editing and execution:
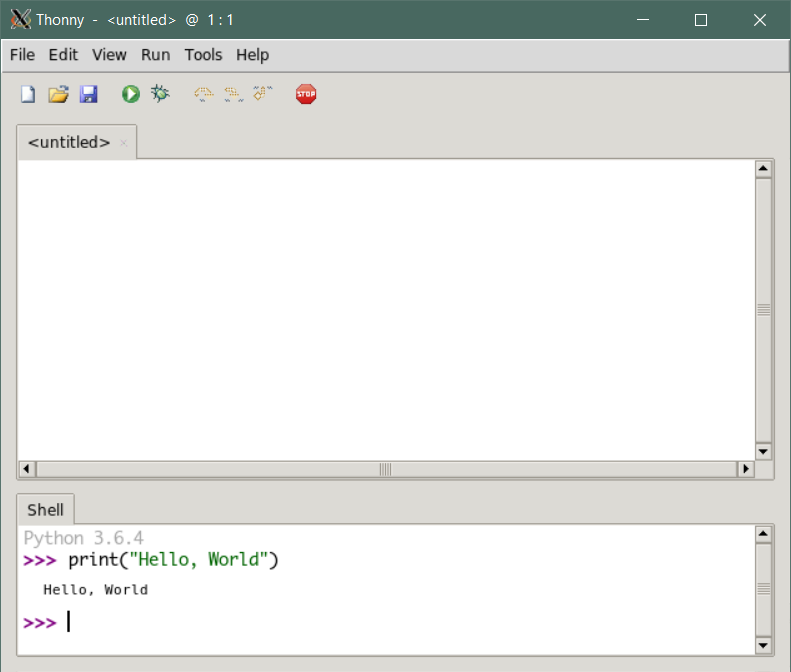
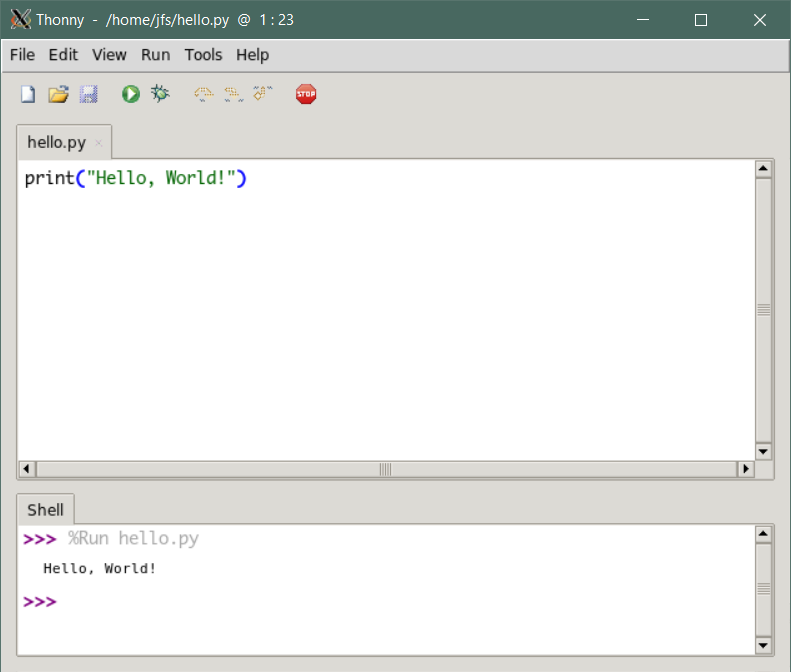
Thonny performs syntax highlighting and code completion in addition to providing a step-by-step debugger. One feature that is particularly helpful to those learning Python is that the debugger displays values in expressions as they are evaluated while you are stepping through the code:
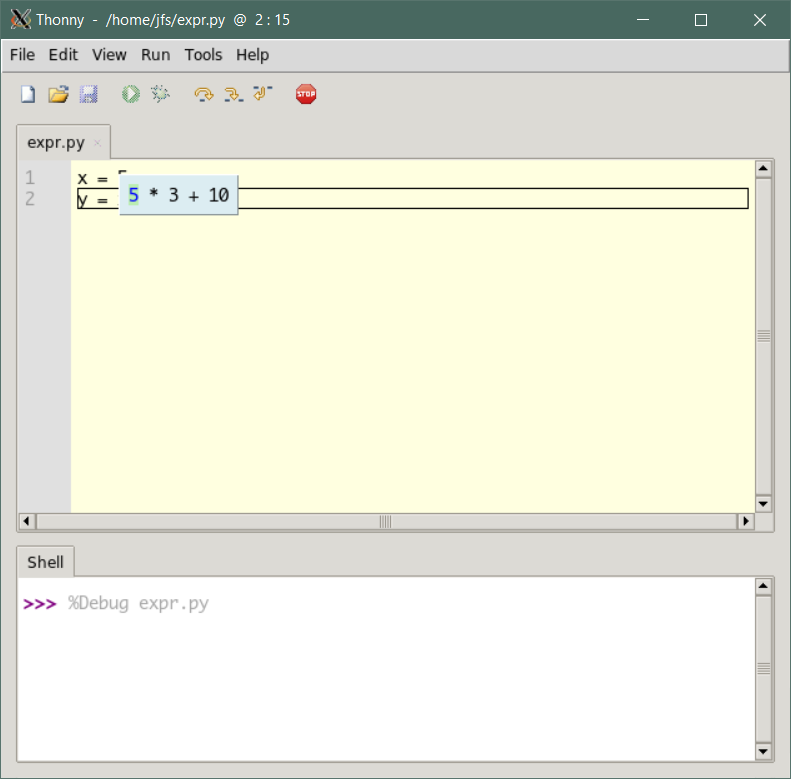
Thonny is especially easy to get started with because it comes with Python 3.6 built in. So you only need to perform one install, and you’re ready to go!
Versions are available for Windows, macOS, and Linux. The Thonny website has download and installation instructions.
IDLE and Thonny are certainly not the only games going. There are many other IDEs available for Python code editing and development. See our Python IDEs and Code Editors Guide for additional suggestions.
Online Python REPL Sites
As you saw in the previous section, there are websites available that can provide you with interactive access to a Python interpreter online without you having to install anything locally.
This approach may be unsatisfactory for some of the more complicated or lengthy examples in this tutorial. But for simple REPL sessions, it should work well.
The Python Software Foundation provides an Interactive Shell on their website. On the main page, click on the button that looks like one of these:

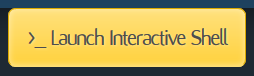
Or go directly to https://www.python.org/shell.
You should get a page with a window that looks something like this:
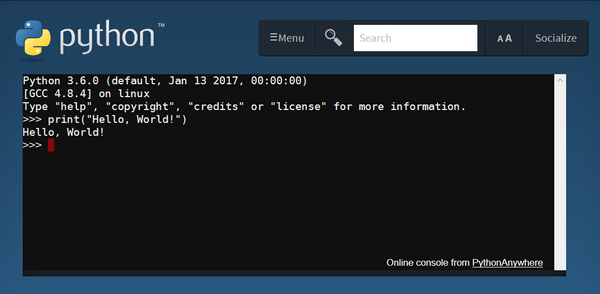
The familiar >>>
prompt shows you that you are talking to the Python interpreter.
Here are a few other sites that provide Python REPL:
Conclusion
Larger applications are typically contained in script files that are passed to the Python interpreter for execution.
But one of the advantages of an interpreted language is that you can run the interpreter and execute commands interactively. Python is easy to use in this manner, and it is a great way to get your feet wet learning how the language works.
The examples throughout this tutorial have been produced by direct interaction with the Python interpreter, but if you choose to use IDLE or some other available IDE, the examples should still work just fine.
Continue to the next section, where you will start to explore the elements of the Python language itself.