Improve Your Python With:
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days:
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
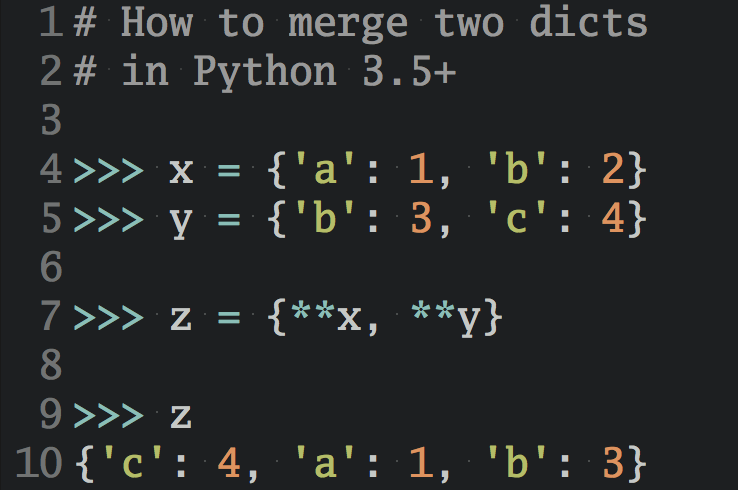
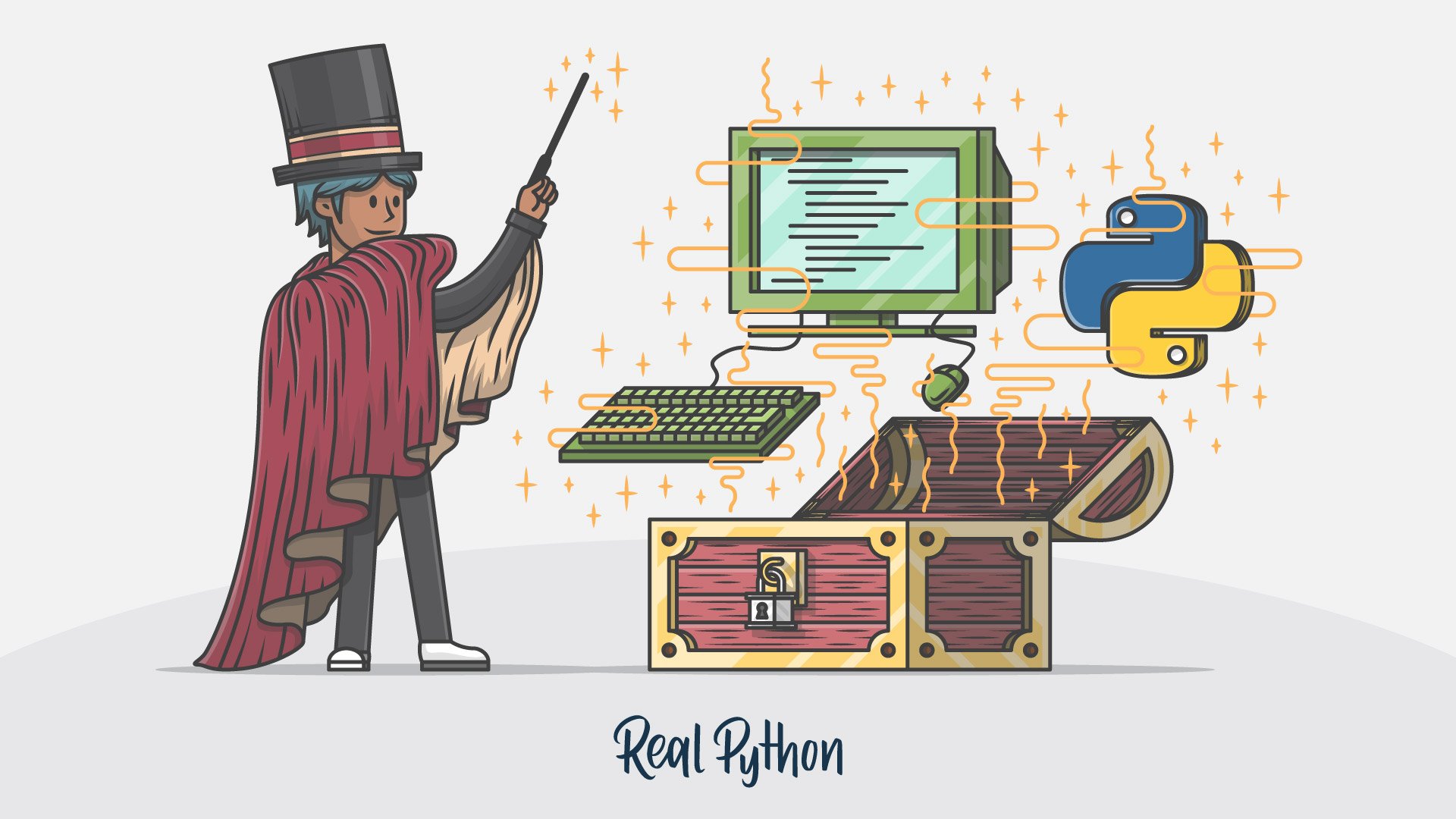
Q: What’s a Python Trick?
A short Python code snippet meant as a teaching tool. A Python Trick either teaches an aspect of Python with a simple illustration, or serves as a motivating example to dig deeper and develop an intuitive understanding.
Here are a few examples of the kinds of tricks you’ll receive:
# How to merge two dictionaries
# in Python 3.5+:
>>> x = {'a': 1, 'b': 2}
>>> y = {'b': 3, 'c': 4}
>>> z = {**x, **y}
>>> z
{'c': 4, 'a': 1, 'b': 3}
# In Python 2.x you could use this:
>>> z = dict(x, **y)
>>> z
{'a': 1, 'c': 4, 'b': 3}
# Why Python Is Great:
# Function argument unpacking
def myfunc(x, y, z):
print(x, y, z)
tuple_vec = (1, 0, 1)
dict_vec = {'x': 1, 'y': 0, 'z': 1}
>>> myfunc(*tuple_vec)
1, 0, 1
>>> myfunc(**dict_vec)
1, 0, 1
# The lambda keyword in Python provides a
# shortcut for declaring small and
# anonymous functions:
>>> add = lambda x, y: x + y
>>> add(5, 3)
8
# You could declare the same add()
# function with the def keyword:
>>> def add(x, y):
... return x + y
>>> add(5, 3)
8
# So what's the big fuss about?
# Lambdas are *function expressions*:
>>> (lambda x, y: x + y)(5, 3)
8
# → Lambda functions are single-expression
# functions that are not necessarily bound
# to a name (they can be anonymous).
# → Lambda functions can't use regular
# Python statements and always include an
# implicit `return` statement.
Improve Your Python Skills, Bit by Bit:
🐍 Python Tricks 💌
Get a short & sweet Python Trick delivered to your inbox every couple of days. No spam ever. Unsubscribe any time. Curated by the Real Python team.
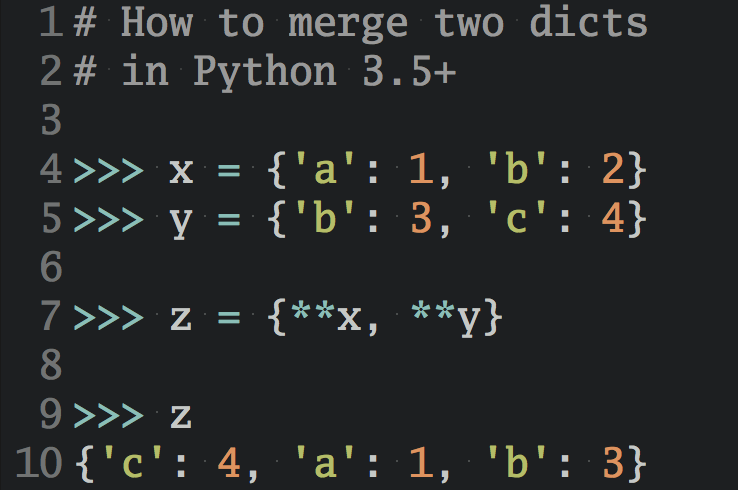
What Python Devs Are Saying:
The PyTricks newsletter by @realpython is a fantastic example of how newsletter should look like. Short, clear and straight to the point!
— Oras Al-Kubaisi (@orask) May 30, 2018
These are so good. The "dicts to emulate switch/case statements" was 🔥😎🔥. https://t.co/U2J7IOPmJN
— Ben Love 🐍💻⚒ (@thebenlove) March 11, 2017
Dare I say, that looks Pythonic to me. https://t.co/qEajq6GNl8
— Talk Python Podcast (@TalkPython) March 9, 2017
Recommended! https://t.co/Mc0dX7gJjn
— Carlos Abdalla (@carlosabdalla) March 11, 2017
The regular emails from @realpython are a real treat and highly recommended for anyone interested in Python.
— Matthias Miltenberger (@MattMilten) October 7, 2018love the work you’ve done, are doing, and continue to do!
— Kenneth ☤ Reitz, Creator of the Requests library
“Python Tricks” curated by Dan Bader and the Real Python team.