Advanced Techniques and Strategies
00:00 All right, welcome! Today we’re going to get into some really fun and advanced techniques and strategies for iterating through, modifying, and doing some real-world tasks with dictionary iteration.
00:12 This will mostly center around an understanding of dictionary comprehensions and how they work and how they make things easier and more elegant for us all. So, let’s get into it!
00:23 First thing we’re going to do is we’re going to just talk about a basic dictionary comprehension. You may be familiar with list comprehensions and you may also be familiar with dictionary comprehensions, so this might be a review for you.
00:34
But either way, let’s take a look. Imagine we’ve got some objects. We’ve got a 'blue'
, we’ve got an 'apple'
, we’ve got a 'dog'
.
00:43
We’ve got some disparate kinds of objects. And then we’ve got categories that these different objects belong to, 'color'
and 'fruit'
and 'pet'
and so on.
00:55
And before the idea of dictionaries or maps came around, really, this was often how you would store relational lists. You would just have two lists and each one at each index would correspond to the other. But now we have dictionaries, and so we’re going to say that a_dict = {key, value for key, value in }
—and then we use this cool little function zip()
here, which essentially just takes two iterables and puts them into one kind of parallel iterable.
01:23
There’s a whole other set of videos on exactly how to use this zip()
function. I encourage you to look around at those because it’s a really cool function.
01:29
But essentially what you need to know is that it makes these into the kind of .items()
representation of this dictionary. And then we can just use this dictionary comprehension to actually make it into a real dictionary. Of course, what I did wrong here was this needs to be a colon (:
).
01:43
A dictionary comprehension always needs to have this <key>: <value>
structure for the <key>, <value>
in something else.
01:50
So, this is one way to do a dictionary. And let’s take a look! It’s created, and so 'blue'
goes to 'color'
, 'apple'
goes to 'fruit'
, 'dog'
goes to 'pet'
. Exactly as we might imagine.
02:00 So this is really convenient and really useful because you can do a lot of the things that we talked about in the last video, like filtering and moving through dictionaries and doing things with each item, just using this dictionary comprehension structure.
02:15 So, let’s take a look again at the problem that we solved in the last video with an iterative method, which was turning keys into values and vice versa.
02:25
With this new paradigm, this becomes really, really easy. What we can do is we can just say new_dict
equals value
goes to key
for key, value in a_dict.items()
.
02:38
Do you see how clean and how readable that is? Really, all we’re doing is we’re saying we want the value
to map to the key
for each key, value
in this dictionary’s .items()
, right?
02:49
It’s so simple and it’s so clean. And there we are! We’ve got it. 'color'
maps to 'blue'
, 'fruit'
maps to 'apple'
, 'pet'
maps to 'dog'
.
02:55 It’s really pretty amazing. So, let’s go and let’s look again at how this filtering concept can really also become easy. Let’s go back to this old version.
03:07
Let’s just say num_dict
equals—this is just so that it’s really easy to see kind of what’s happening. We’ve got 'one'
goes to 1
. And you could do this with some cool dictionary comprehensions, actually, you could build this dictionary.
03:21
But I’m just going to build it by hand, just so that this is all obvious and clear. 'four'
is going to go to 4
, and so on. So we have this num_dict
, and say we want to filter these items, again so that we only take the key-value pairs whose value is greater than 2
.
03:37
So it should just be 'three'
goes to 3
, and 'four'
goes to 4
. Well, this one is really, really simple to do because we can just say… We can just again go with new_dict
. Or, you know what?
03:50
We’ll just modify num_dict
directly, because we don’t really need this other stuff. So, what you can do is you can just say key
maps to value
for key, value in num_dict.items()
, and then here at the end, you can just say if value > 2
.
04:10
Oh, sorry, that’s not what we want. But num_dict
. Great! So, see how easily that works? And it really just reads just like English. So, {key: value for key, value in num_dict.items() if value > 2}
.
04:22
So you just only include those key-value pairs which have a value of more than 2
. Let’s look again at calculations. We have this dictionary incomes
, which we made in the last video, and it has some incomes for these people.
04:36
And now what we can do is really, really simple. We can just say a sum()
—and this is something that you should learn to become comfortable with too—of [value for key, value]
—or, well, we don’t even need to say that. We just need to say for value in incomes.values()
.
04:56
So the sum()
of this list, right? Boom. So that is the sum of the incomes. And really also, you know what’s cool too is that you could do this even more simply.
05:07
You could just say sum(incomes.values())
because incomes.values()
is itself an iterable, which can be summed. So, this is getting into some really kind of advanced features of how Python works, but the core insight is the same, which is why don’t we instead of thinking iteratively start thinking in terms of comprehensions?
05:25 Something else that might be interesting to note is just that if you want to do the sum or you want to perform some calculations on large lists, you can actually instead of doing a list comprehension, you can do a generator expression.
05:41 This is actually going to do the exact same thing, it’s just going to do it in a lazy manner so that it doesn’t fetch the whole list at once, which doesn’t put perils on your memory.
05:52
So that’s just something to think about. Again, we’re getting into some really advanced and fun and interesting stuff. There are many other different things that you can do with this dictionary comprehension paradigm. For example, what you could do is we could have incomes
again, and then if you wanted a sorted version of incomes
, so if you wanted the keys in sorted order, for example, you could iterate through—you could say, you know, for key in sorted(incomes)
. You could do that. But what you can also do is you could, if you wanted just a sorted_incomes
dictionary—excuse me, there we go. sorted_incomes
.
06:31
Then you can again do this with a dictionary comprehension. You can say k
goes to incomes
at k
—and k
just stands for key, of course—for k in sorted(incomes)
.
06:45
So again, this is just a way to leverage—sorry, sorted_incomes
. A way to leverage the comprehension style of Python to really get some concise, clear code which still does exactly what you want it to do.
06:59 So that’s really, really cool. And you can also sort by values, and this is a little bit more complex because it requires you to use some functions, which I hope you’re familiar with but you may not be.
07:13
So what we’re going to do, we’re going to define a by_value()
function on a dictionary item, which will just return the item at its first index.
07:22
And so this is going to be convenient because then we can say for k, v in sorted(incomes.items(), key=by_value)
.
07:32
This will sort everything by by_value()
. print(k, v)
.
07:38
Um. I’m sorry, what have I done? I forgot to put parentheses after the .items
, which does tend to happen sometimes when you code and when you’re working.
07:50
Don’t worry about it, just find the little error and keep on moving. So now, as you can see, it’s sorted by the value instead of by the key, like we sorted it above in sorted_incomes
.
07:59 So, those are just a small sample of the really awesome things you can do with dictionary iteration using comprehensions and using that style. It really becomes very, very simple. So, thank you for watching.
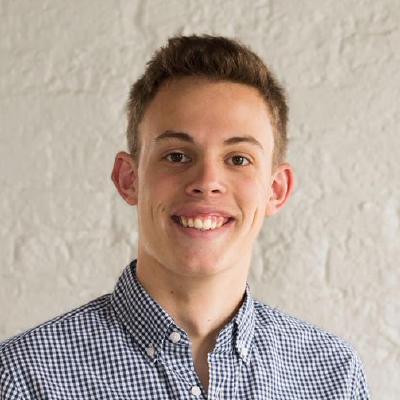
Liam Pulsifer RP Team on April 3, 2020
Hmm, @Dattaram did you make sure to define by_value as follows?
def by_value(item):
return item[1]
Vedang Joshi on April 13, 2020
Do Python dictionaries always maintain the order in which the elements were added?
For Example: The method shown in the video:
>>> veggies
{'celery': 5, 'cabbage': 3, 'broccoli': 11}
>>> sorted_veggies = {k: veggies[k] for k in sorted(veggies)}
>>> sorted_veggies
{'celery': 5, 'cabbage': 3, 'broccoli': 11}
>>>
>>> for k in sorted(veggies):
... print(k, ' -> ', veggies[k])
...
('broccoli', ' -> ', 11)
('cabbage', ' -> ', 3)
('celery', ' -> ', 5)
>>>
The order of items added is NOT maintained. However, if we use OrderedDict(), it is. @Liam Am I missing something?
>>> from collections import OrderedDict
>>>
>>> sorted_veggies = OrderedDict()
>>> for k in sorted(veggies):
... sorted_veggies[k] = veggies[k]
...
>>> sorted_veggies
OrderedDict([('broccoli', 11), ('cabbage', 3), ('celery', 5)])
>>>
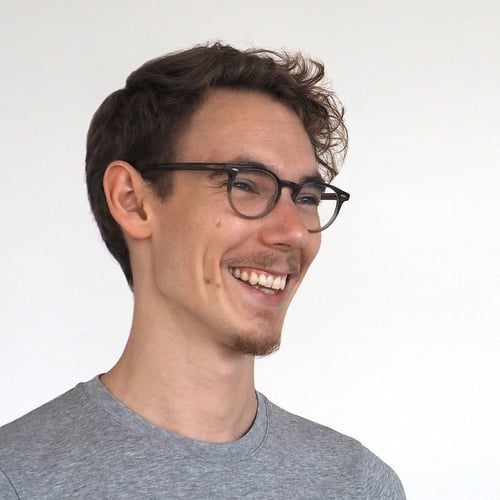
Dan Bader RP Team on April 14, 2020
Python dictionaries started retaining the insertion order with Python 3.6 (and above). More info here in this tutorial: realpython.com/python-dicts/#dictionary-keys-vs-list-indices
Vedang Joshi on April 14, 2020
Got it. Thanks
Liza Olariu on June 13, 2020
Hi Liam! Thanks for the great tutorials. For the last example, I think we might use the example below as well.
sorted_incomes = {key: value for key, value in sorted(incomes.items(), key=lambda item: item[1])}
Become a Member to join the conversation.
Dattaram on April 1, 2020
For the last example, by_value is throwing error as undefined; NameError by_value is undefined