How to Use .popitem()
00:00
All right! So, in this video, we’re going to look at just a very small subset of some cool things to learn in Python dictionaries. The first of these is the .popitem()
function.
00:13 So, let’s see. I’ve got a dictionary here. And this dictionary, it just has some strings which map to one another. Kind of, you know, objects to the categories they might belong to.
00:25
And so let’s see what happens—just as a way to get started here—if we just call .popitem()
on this dictionary. What do we get? Well, we get this tuple, an item, of ('dog', 'pet')
.
00:39 And I’m just going to tell you right now that this is pretty much totally random, so there’s no way of knowing which key-value pair you’re going to get.
00:45
You get an arbitrary key-value pair. And then let’s look and see what happens to a_dict
. Well, this 'dog'
and 'pet'
item has been removed from the dictionary.
00:54
So, it’s probably pretty clear to you—let’s do it again, just so we can see here what happens. Now we get ('apple', 'fruit')
and a_dict
is reduced in size by one yet again, and this pair has been removed.
01:06 So, what you can probably tell is that what this does is it iterates destructively through a dictionary. And that means that it’s going to return and remove an item for you from the dictionary. So, you might be thinking, “Well, why is this useful?
01:22 I’m probably never going to need to use this.” Well, it could be very useful in some limited circumstances. For example, if you have a dictionary of items for which the ordering doesn’t really matter, and you know you’re not going to need to use it again.
01:36
And what this will allow you to do is if you have these conditions satisfied, then you can just iterate using .popitem()
, and then you are reducing the amount of memory you need to store stuff because if you’re just doing calculations on the things in your dictionary, then you can just pop all the items and not worry about it.
01:54
So, just to give you a quick final example, we’re going to pop an item and we’ll get this 'color'
, and then we get a_dict
is just nothing.
02:03
What happens if we try to pop an item again? Well, we get a KeyError
, because this dictionary was empty when we popped it. So, let’s redefine a_dict
. And this time, I’m just going to say, 'a'
goes to 10
, just for simplicity’s sake and ease of writing for me, 'b'
goes to 20
, 'c'
goes to 30
.
02:27
And this could continue, of course, but… So, we refilled a_dict
full of items, and I’ll show you a kind of a paradigm that’s useful for when you’re working with this .popitem()
function. What you can do is use a while True:
loop, which just continues to evaluate until you break out of it.
02:44
And then you can also use a try
/except
block, and you can say item = a_dict.popitem()
, and we’ll just print it just for the heck of it.
02:55
And then probably what you’ll do is you’ll do some cool stuff with this item
and then…Right. So, that’s good. And then we want to except the case that we get a KeyError
because of .popitem()
being called an empty dictionary.
03:14
And then we’ll just print out "The dictionary is empty"
.
03:19
And then finally, of course, we’ll break. So, this is a paradigm that’s really useful when you’re dealing with this .popitem()
, just because it gives you a nice way to iterate through destructively so that at the end you’ll have an empty dictionary, which will take up very little space, and then you’ll also have done all of the things you need to do with these items. So as you can see, we popped out here this, this, and then this.
03:42
And so the key things to remember with .popitem()
is that it’s a destructive iterator; that the exact items you get are not guaranteed, so be careful with assuming that there’s some kind of order that there really isn’t; and then you need to be careful at the end, because if you try to pop from an empty dictionary you’ll get a KeyError
.
03:58 So. That’s just one cool function that Python dictionaries offer. Thanks for watching.
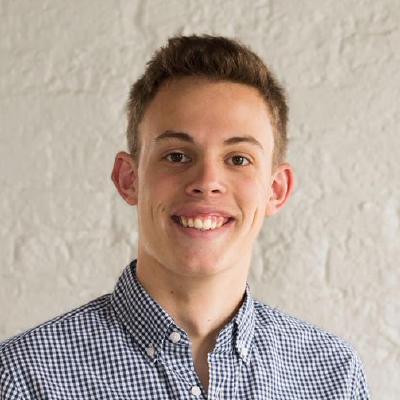
Liam Pulsifer RP Team on Dec. 29, 2019
Thanks for the comment @swannbouviermuller. I generally agree with you. In this case, the while True:
loop is intended to showcase the behavior of .popitem()
when called on an empty dictionary (i.e. that it generates a KeyError
), so it’s more of an illustrative example of a concept rather than an example of how you would structure a loop in practice. In the wild, your proposed loop condition is perfect!
cmansfield on Jan. 3, 2020
I’m not sure which version of Python you are using for this tutorial but from 3.7 popitem() removes the item last added to the list rather than a random key-value pair. I got suspicious after I kept getting the items returned / removed in this order and checked the documentation…
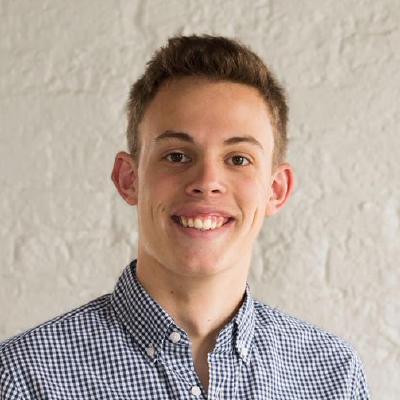
Liam Pulsifer RP Team on Jan. 3, 2020
Very nice catch @cmansfield. You are correct that in Python versions <= 3.6, .popitem()
returned arbitrary items, but in 3.7 it was updated to return items in LIFO
order. I’ll edit this video accordingly. Thanks again for the catch!
fjavanderspek on April 19, 2020
@swannbouviermuller we can even use
while a_dict:
print(a_dict.popitem())
as an empty dictionary will evaluate to False
Become a Member to join the conversation.
swannbouviermuller on Dec. 29, 2019
I find while True very ugly beacause we can’t understand up front when the loop will end. We have to read all the loop statements to find break(s) line.
I find “while len(a_dict) > 0:” much better.