Catching Exceptions With try and except
00:00
So far, you’ve learned how to raise exceptions in different ways. In this lesson, you’re going to learn to use the try
… except
block to catch your exceptions—so to prevent your program from quitting if an exception gets raised. And you can do this using the try
and the except
keywords in the way that you create an indented code block where you try to run some code, and if an exception occurs, you catch it and you execute some code when the exception occurs instead of quitting your program. Now, if you head back to the code, you can see that here I did a bit of refactoring that you can also do if you want to.
00:41
You can pause this and just write the function in the way that I did here. You will see that it’s quite similar to what you had before. It’s just the check of if not
, the platform is "linux"
, then you want to raise the custom WrongOsError
that you wrote at the beginning.
00:58
And all I did is just make the message that comes out a little bit more meaningful, and then also simulate some sort of work that would happen on the Linux system afterwards. In this case, it’s just the print statement, but you could have your platform-specific code happening down here and the windows_interaction()
and the macos_interaction()
functions up here, they’re exactly the same.
01:23 So if you go ahead and you run this code, I’m going to show you what the output is, and you will see it’s familiar to before.
01:32
You can see that the code raises the WrongOsError
, and now you get a little bit more descriptive message, but otherwise it’s the same. Any code that would happen here…
01:47
is not going to execute because your program runs into an error before getting there. And you’re not handling this exception. The exception gets raised, so that means your program quits. Now, you don’t want that, so you want to make sure that this "happens after"
runs also if you’re running into an exception. And to do this, you can wrap the call to linux_interaction()
into a try
… except
block.
02:12
So you want to try to run this linux_interaction()
, and if an exception occurs, except
, then you want to say,
02:23
"oops!"
. But your program won’t quit, and so you will still see this happens after printout even if you run into an exception. Let’s give that a spin.
02:40
So, you see that you tried to run the linux_interaction()
function. You know that this ran into your custom WrongOsError
, and your except
statement here caught it, and then instead of quitting the program, it printed out "oops!"
and then your program continued, the script continued running, and printed out this "happens after"
as well.
03:03
Now, this is actually an anti-pattern that you shouldn’t do. You shouldn’t just have a plain except
statement, but you always want to be explicit with the exceptions that you’re catching.
03:12
So you know that this one is your custom WrongOsError
, and so you want to catch the WrongOsError
and then print "oops!"
.
03:22
And you can also assign the error to a variable and then print it or use it inside of that block. So I’m going to say error
and "oops!"
.
03:32
Or let’s make it more logical and go the other way around: "oops!"
, error
. So this is going to print the error message. Now, if you run this code again, you can see that, again, the WrongOsError
gets raised.
03:48
It gets caught here in the except
block. It gets assigned to a variable, and then you’re printing out "oops!"
and the value of this variable, which is going to be the message of the WrongOsError
. And your program doesn’t quit, but instead continues and then prints the next line as well.
04:07
Now, if you switch that, and let’s instead of trying to run the linux_interaction()
, I’m going to run the macos_interaction()
.
04:18
Since I am on a Darwin system, this one is going to pass, and then the except
block is not going to print, so nothing in here is going to execute.
04:33
And you can see that it instead prints out the Doing important macOS work
placeholder for whatever system-specific code you would want to run, and then continues afterwards and skips this whole except
block because the WrongOsError
didn’t get raised.
04:50
So, this is how you can use try
… except
blocks, and it’s also good to know that you can use multiple except
blocks. You can be specific and catch a couple of errors, you can just continue writing them down here. except AnotherError
—this one doesn’t doesn’t exist, ha. That’s not a built-in one.
05:06 And then open up another block and do your code in here.
05:13
This is the way how you can catch exceptions in Python, so any exception that gets raised, whether it’s your own custom exception or a built-in exception that Python raises for you, you can catch it with an except
statement and then do some alternative code execution rather than just quitting the program.
05:31 And that’s all! What happens, however, if no exception gets raised? You could also want to execute some code only in the case that no exception got raised, and that’s a keyword that exists as well that you can use, and you will learn about it in the next lesson.
torrepreciado on Dec. 31, 2021
Is a bare except always discouraged or could it make sense if you don’t expect any exception to be raised?
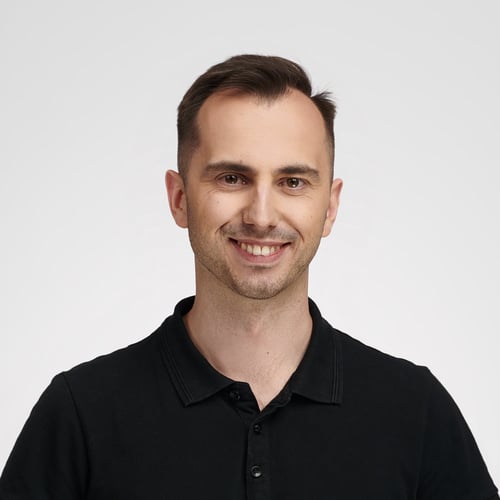
Bartosz Zaczyński RP Team on Jan. 3, 2022
@freemti You meant the No Entry traffic sign, which is this emoji. You can obtain it in Python if you know its Unicode codepoint:
>>> ord("⛔")
9940
>>> chr(9940)
'⛔'
>>> hex(9940)
'0x26d4'
>>> "\u26d4"
'⛔'
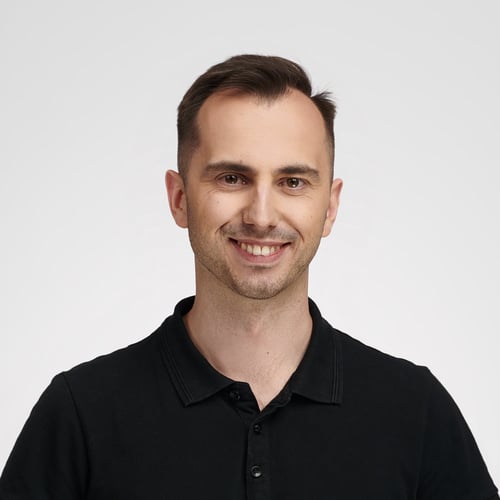
Bartosz Zaczyński RP Team on Jan. 3, 2022
@torrepreciado StackOverflow seems to have a good answer to your question:
Bare
except
will catch exceptions you almost certainly don’t want to catch, includingKeyboardInterrupt
(the user hitting Ctrl+C) and Python-raised errors likeSystemExit
.If you don’t have a specific exception you’re expecting, at least
except Exception
, which is the base type for all “Regular” exceptions.That being said: you use
except
blocks to recover from known failure states. An unknown failure state is usually irrecoverable, and it is proper behavior to fatally exit in those states, which is what the Python interpreter does naturally with an uncaught exception.Catch everything you know how to handle, and let the rest propagate up the call stack to see if something else can handle it. In this case the error you’re expecting (per the docs) is
pyautogui.ImageNotFoundException
. (Source)
Become a Member to join the conversation.
freemti on Dec. 26, 2021
Off on a tangent here, but how do you insert the stop sign symbol?