Defining a Set in Python
00:00
There are two ways to define a set in Python. You can use the set()
function, which takes in a iterable and converts the iterable to a set.
00:09
So for example, we can pass the list ['foo', 'bar']
, which will convert that list into a set with those two elements. Notice how the order is not exactly the same as the list we passed in, because set are unordered, so it’ll just display in some random order.
00:25 Let’s see what happens when we pass in a dictionary. It will turn the keys of the dictionary into a set. We can also pass in a string, because that’s a iterable.
00:34 And that will split the string by characters and create a set from the resulting characters. And if there are any duplicate letters, it will only include those once because sets cannot contain duplicates.
00:45
It will also print out in a random order. For example, we could use the list()
function, which will turn a iterable into a list, and that will keep the order and the duplicates.
00:55
The second way we can define a set is by using the curly braces. So here, let’s create a set with two elements, I guess {'foo', 'bar'}
. And x
is the set {'foo', 'bar'}
.
01:07
If we want an empty set, we have to use the first method because, as we see, the second method like this is actually a dictionary and not a set. So we have to actually use set()
like this, which will be a set.
01:24
Let’s talk a little bit more about the empty set. That is a False
value, just like how empty lists and dictionaries are all False
values.
01:34
Sets are really cool because they can contain any hashable value, and the same set does not have to include the same type of value. So we can have a string, a Boolean, None
, and then a tuple—because, remember, tuples are immutable and by definition hashable—and that will work. Some other languages might not allow that, but Python does.
01:55
Let’s try to pass a not-hashable value and see what happens. Lists are not hashable, and this will error. And it actually says unhashable type: 'list'
. In the next section, you’ll learn different ways to operate on a set.
kiran on Aug. 1, 2020
Why set showing same order?
>>> people = {"Jay", "Idrish", "Archi"}
>>> people
{'Idrish', 'Archi', 'Jay'}
>>> people
{'Idrish', 'Archi', 'Jay'}
>>> people
{'Idrish', 'Archi', 'Jay'}
>>> people
{'Idrish', 'Archi', 'Jay'}
>>> people
{'Idrish', 'Archi', 'Jay'}
>>> people
{'Idrish', 'Archi', 'Jay'}
>>> people
{'Idrish', 'Archi', 'Jay'}
>>> people
{'Idrish', 'Archi', 'Jay'}
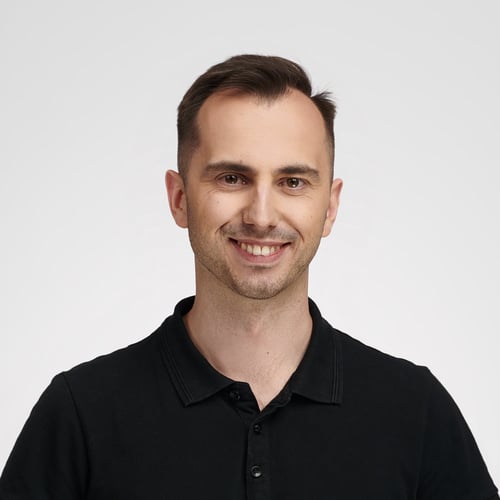
Bartosz Zaczyński RP Team on Aug. 3, 2020
It’s an implementation detail. You should assume that elements of a set can appear in any order.
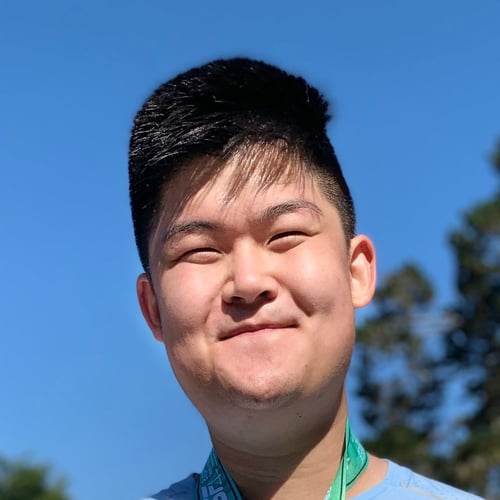
James Uejio RP Team on Aug. 4, 2020
Weirdly enough it comes out in a different order on my computer…
In [5]: {'Idrish', 'Archi', 'Jay'}
Out[5]: {'Archi', 'Idrish', 'Jay'}
Also on Python 2.7
>>> {'Archi', 'Idrish', 'Jay'}
set(['Idrish', 'Archi', 'Jay'])
I would think it would be consistent but it seems to not. Would love to know if any RP staff or others have any ideas. Anyways clearly we cannot assume the ordering of sets at all.
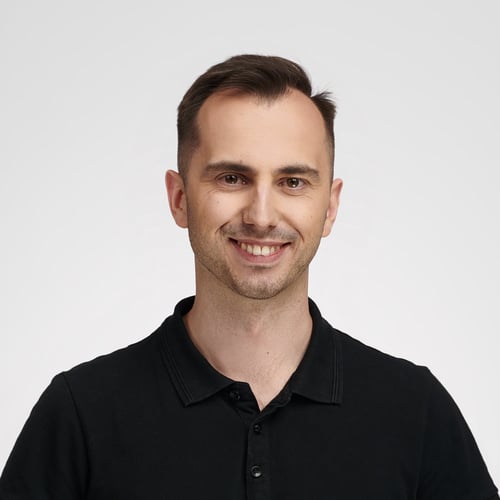
Bartosz Zaczyński RP Team on Aug. 4, 2020
Sets don’t make any promises about the ordering of their members. They use hashing, which itself is an implementation detail of the Python interpreter and depends on multiple factors.
To avoid hash collisions, which is a well-known security exploit, Python chooses a random seed for the hash function every time it starts. As a result, the same object will produce different and seemingly random hash values in separate interpreter sessions:
$ python
>>> hash('Archi')
-863196821531266440
$ python
>>> hash('Archi')
4042034065582253111
$ python
>>> hash('Archi')
7069460679375787308
These numbers determine the order of your set members. If you’re aware of the risks involved, you can disable this mechanism, for example, by setting an environment variable:
$ PYTHONHASHSEED=123 python
>>> hash('Archi')
1934745164520175339
$ PYTHONHASHSEED=123 python
>>> hash('Archi')
1934745164520175339
$ PYTHONHASHSEED=123 python
>>> hash('Archi')
1934745164520175339
Then, you should be able to get a predictable order of set members. However, if you need them to retain insertion order, you’re better off using a different collection such as OrderedSet:
$ pip install orderedset
$ python
>>> from orderedset import OrderedSet
>>> oset = OrderedSet([1, 2, 3])
OrderedSet([1, 2, 3])
kiran on Aug. 4, 2020
@Bartosz Zaczyński killing thanks.
kiran on Aug. 6, 2020
A set itself may be modified. can you give example for this?
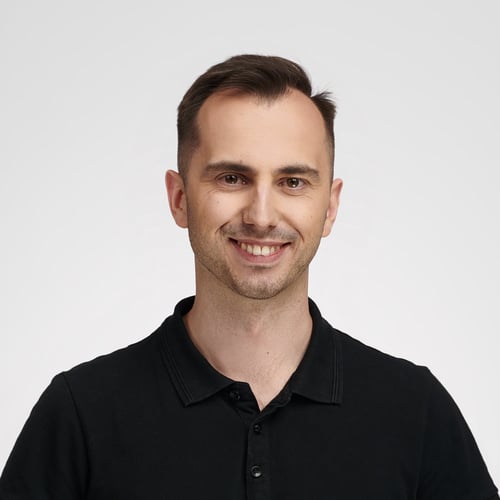
Bartosz Zaczyński RP Team on Aug. 6, 2020
Sets are mutable in Python. You can add or remove elements from them:
>>> fruits = {'apple', 'banana', 'orange'}
>>> fruits.add('peach')
>>> fruits
{'apple', 'orange', 'banana', 'peach'}
>>> fruits.remove('banana')
>>> fruits
{'apple', 'orange', 'peach'}
Become a Member to join the conversation.
Levi on March 14, 2020
Other tutorials I’ve watched on lists/sets never mentioned anything about hashes. Thanks for covering them!