Length, Membership, and Iteration
00:00
Let’s talk about different ways to operate on a set, starting with length. len(x)
will compute the length of a set. The argument needs to be an iterable, and because set are iterables, len()
of a set works.
00:14
But this is actually how you get the length of any iterable, so len()
of a list or len()
of a tuple would also get the length of those corresponding data structures. Let’s look at an example.
00:24
a
is a set of {1, 1, 2}
. What is the length? Well, it’s actually 2
because sets cannot contain duplicates. So when we define the set with two 1
values, our resulting set is actually just the set of {1, 2}
.
00:40
We can get the length of the empty set, which is 0
. And here, let’s look at a little more complicated example. b
is a set with a tuple, {(1, 2), 2}
.
00:51
The length is 2
because we’re looking at how many elements the set b
has in it. We’re not looking at how many elements those elements have.
01:01
We just look at how many elements are in b
and there’s only two, there’s a tuple and the number 2
.
01:08
Let’s move on to checking membership in a set. Checking membership is another way of saying “checking if an element exists in the set.” We use the in
syntax, so <elem> in x
and it returns a Boolean which indicates if an element exists in a set.
01:24
x
just has to be an iterable, and just like len()
, this will work on any iterable, and because sets are iterables, this will work. Here we have the set {'foo', 'bar'}
.
01:35
We check 'foo' in x
, the string 'foo'
—that’s True
. We check 'baz' in x
—that’s False
.
01:43
We check 'baz' not in x
. That will say “Is the element 'baz'
not in our set?” That is True
, because 'baz'
is not in x
.
01:52
not 'baz' in x
will evaluate “Is 'baz' in x
?” And then not
that value, which is also True
because 'baz' in x
returns False
, and not False
is True
.
02:04
I prefer the 'baz' not in x
, just because sort of from an English standpoint, it makes more sense to me to just say 'baz' not in x
. That’s True
.
02:14
So, to iterate or loop over a set, use the same syntax as you would a list or tuple, or any iterable for that matter. But note, you cannot slice or index sets because they’re not ordered, so that doesn’t really make sense to slice some of them or get the zeroth index or something like that. So, here’s an example. We have a set with {'foo', 'bar', 'baz'}
.
02:36
We say for elem in x:
you print the elem
, and it’ll just print out like that. Also note that they are in a unordered fashion, so you cannot assume any sort of ordering to them. But this is useful if you want to just print out all the elements or maybe loop through and compare them with something else. Here, we try to index. It errors, because it says 'set' is not subscriptable
.
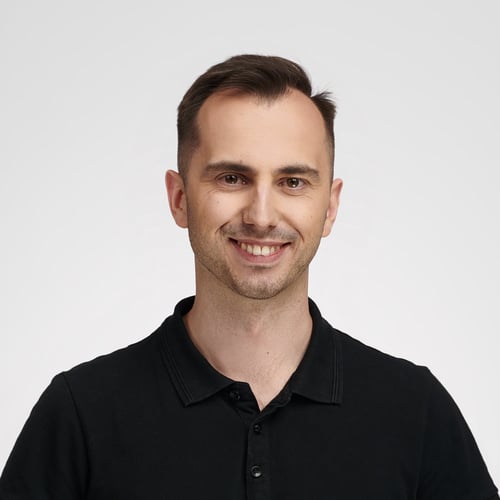
Bartosz Zaczyński RP Team on March 6, 2023
@tonypy You can use Python’s collections.Counter
as a multiset: realpython.com/python-counter/#using-counter-instances-as-multisets
tonypy on March 6, 2023
OK, not heard of multisets, thx for the feedback, I’ll take the course. In the meantime it would be useful to update the course to add a comment about mutisets and refer students to the course you suggest.
Become a Member to join the conversation.
tonypy on March 5, 2023
As sets cannot have allow duplicate elements, how can you define a set a = {1, 1, 2} which has duplicates?