Different Update Methods
00:00 Let’s look at some more set modifying operations. All of the ones in this video will have a method and a corresponding augmented assignment. They will also correspond to some of the set operations—union, intersection, difference, and symmetric difference.
00:19 Starting with update, this modifies the set by adding any elements that do not already exist. It’s very similar to union, where that will create a set with all elements in all sets. So instead of creating a new set, we actually change the set to include all elements.
00:37
Here’s the method, .update()
. It takes in multiple arguments, and they need to be iterables.
00:43
Here is the augmented assignment. The operands need to be sets. This is different than the operator that you saw in previous videos because the operator creates a new set while augmented assignment modifies the existing set x1
. Let’s look at some examples.
01:02
x1
is a set with {'foo', 'bar', 'baz'}
, x2
is a set with {'foo', 'baz', 'qux'}
. We use the augmented assignment to change x1
to include all the elements of x2
. So then x1
will be {'qux', 'foo', 'bar', 'baz'}
.
01:20
Notice how 'foo'
doesn’t appear twice, as well as 'baz'
doesn’t appear twice. And it is unordered, so they just appear in some random order.
01:31
Here we can also update x1
using the method and pass in the list of ['corge', 'garply']
, and that will update x1
to now include those two strings as well as all the other elements it originally had.
01:47
Intersection update corresponds with intersection and modifies a set by retaining only elements found in both sets. .intersection_update()
takes in multiple arguments, and here is the augmented assignment, ampersand equal (&=
).
02:03
So here’s our example. We have {'foo', 'bar', 'baz'}
, we have {'foo', 'baz', 'qux'}
. We use x1 &= x2
, and that will change x1
to include only the elements found in both sets, which are 'foo'
and 'baz'
.
02:19
We can call the method and pass in a list of ['baz', 'qux']
, and we will only retain those elements that are both in x1
and in this list, which is just {'baz'}
.
02:33 Moving on to difference update, which corresponds to the difference operation. It modifies a set by keeping only elements that exist in the first set, but not any of the rest.
02:43
Here we have x1.difference_update(x2[, x3...])
, and this will modify x1
to keep only elements that exist in x1
, not x2
or x3
, et cetera. The arguments need to be iterables.
02:56
Here is the augmented assignment, -= x2
, and then we actually have union| x3 | x4
. Operands need to be sets. Note, there is the union operator between multiple operands.
03:12
This will mutate x1
by checking the union of all sets, x2
, x3
, and remove any elements from x1
that exist in the union.
03:20
x1 -= x2 - x3
et cetera won’t do this, and we’ll see that in the example.
03:28
Here we have a
is the set {1, 2, 3}
, b
is the set {2}
, c
is the set {3}
. And then we call a.difference_update(b, c)
, we remove all the elements found in b
or c
from a
, and that will give us the set {1}
. Here we reset a
. Remember, we’re using a mutation now, and so we actually have to reset a
if we want to use the set {1, 2, 3}
.
03:53
Then we do a - b | c
. It will first evaluate the union b | c
, which will be the set {2, 3}
, and then remove those elements from a
. We reset a
, and let’s try to use the -=b - c
.
04:11
That will evaluate b - c
, which will find the difference of b
and c
, which will be the set {2}
because, remember, difference finds all the elements in the first set that do not exist in the second set.
04:23
So that’s essentially doing a -= {2}
, which will remove 2
from our original set. So this was not the same as calling .difference_update(b, c)
.
04:37
Symmetric difference update will modify a set by keeping only the elements that exist in a single set, but not in multiple. x1.symmetric_difference_update(x2)
will modify x1
so that x1
only includes elements that exist in either x1
or x2
.
04:54
The argument needs to be editable, and for some reason, it only takes in one argument. Here’s the augmented assignment, ^=
. Operands need to be sets, and this will mutate x1
to include all elements found in either any of the sets, but not in multiple sets.
05:12
Here’s an example. We have a
is {1, 2, 3}
, b
is a set {3, 4, 5}
, c
is a set {1, 5, 6}
.
05:21
We call a.symmetric_difference_update(b)
. That will update a
to include only elements found in either set, but not both. That will be {1, 2, 4, 5}
.
05:34
Here we reset a
, and then we use the augmented assignment ^=
to update a
to include only elements found in one set, but not multiple.
05:45
So here, those elements would be {2, 4, 6}
, because 2
, 4
, 6
are only found in one set, but not multiple.
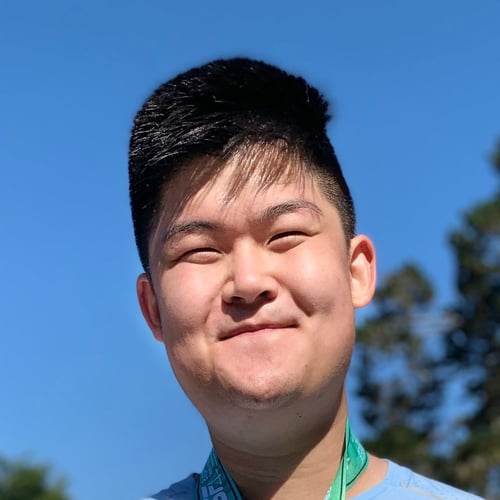
James Uejio RP Team on April 29, 2020
@Sciencificity Thank you for the comment and this is definitely tricky. So what actually happened is Python evaluated the right hand side of the expression first (b ^ c) and then modified a.
You can think of it like this:
a = {1,2,3}
b = {3,4,5}
c = {1,3,5,6}
# step 1
print(b ^ c)
{1, 4, 6}
# step 2
print(a ^ {1, 4, 6})
{2, 3, 4, 6}
# step 3
Modify a to be equal to {2, 3, 4, 6}
Victoria on March 27, 2023
The explanation in the video is not correct. A counter-example, by adding another set.
a = {1, 2, 3}
b = {3, 4, 5}
c = {1, 5, 6}
d = {1} # additional set
a ^= b ^ c ^ d
# {1, 2, 4, 6}
The ^
operations are chained and evaluated on the right first, before the final operation and assignment.
Become a Member to join the conversation.
Sciencificity on April 27, 2020
Hi James,
Here again I believe that the
symmetric_difference_update()
only works on 2 sets for a reason (hence the ^= operator too, should be used only with 2 sets)If we take the 3 set example used in the videos, and introduce a 3 in the last set we notice the weird behaviour for more than 2 sets.
Well, that’s my thinking anyway. Please let me know if I am thinking about it incorrectly?
Thanks for a really great course! It is just this concept which is confusing for me.