Add, Remove, Discard, Pop, and Clear
00:00
Now you’ll learn how to modify a set. We’ll first start with add. Add uses the method .add()
and it will add an element to a set. The <elem>
must be hashable or else it’ll throw an error.
00:15
This is because sets can only contain hashable objects, so we can only add hashable objects. Here we have a set with {'foo', 'bar', 'baz'}
. We add 'qux'
, now our new set becomes {'bar', 'baz', 'foo', 'qux'}
.
00:30
Notice how this actually changed x
. Then let’s try to add a set of {'quix'}
, and this errors because we cannot add a set to a set because sets are not hashable.
00:46
Now let’s say we want to remove an element from the set—just a single element. We use x.remove(<elem>)
, and <elem>
must exist in the set or else it will throw an error. Here we have the set {'foo', 'bar', 'baz'}
, and when we remove 'baz'
, x
becomes the set {'bar', 'foo'}
.
01:06
Let’s try to remove 'qux'
. It will say KeyError: 'qux'
.
01:12
Discard will also remove an element from a set using the .discard()
syntax. But if the <elem>
does not exist, it will do nothing, instead of raising an error like .remove()
. Here we have the set {'foo', 'bar', 'baz'}
.
01:27
We call .discard('baz')
, and we get {'bar', 'foo'}
because we removed 'baz'
. Then we call x.discard('qux')
and it does nothing.
01:38
So x
stays the same and is {'bar', 'foo'}
.
01:42
Pop will remove and return a random element from the set. The syntax is x.pop()
, and if x
is an empty set, then it will raise an error. Here’s our set {'foo', 'bar', 'baz'}
.
01:55
We call x.pop()
. That will return the element. Then x
now doesn’t have that element anymore. We call x.pop()
again, we get 'baz'
.
02:08
We call x.pop()
again, we get 'foo'
. We look at x
, it’s now the empty set because we’ve called .pop()
three times. And now we try to pop, and it raises an error.
02:20
Clear is a handy method to remove all elements from the set. We use .clear()
. Here is our set {'foo', 'bar', 'baz'}
. We see that it’s {'foo', 'bar', 'baz'}
. Then we call x.clear()
, and now x
is the empty set.
02:36
We can clear an empty set and it’ll just do nothing and x
will still be an empty set.
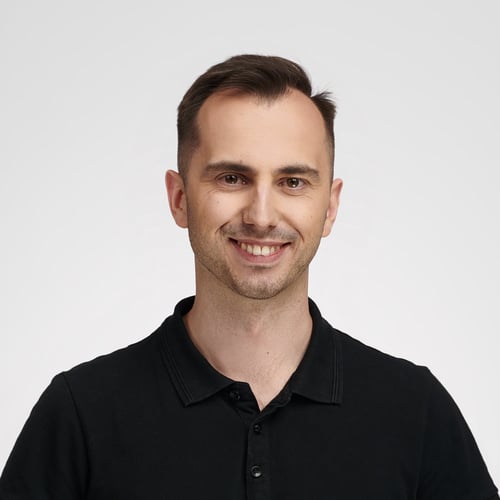
Bartosz Zaczyński RP Team on Feb. 9, 2021
@DoubleA One example that comes to mind is implementing a quiz. You could populate your set with questions and pop them until the set becomes empty:
questions = {"How are you?", "What's your name?"}
while questions:
answer = input(questions.pop())
DoubleA on Feb. 9, 2021
@Bartosz, thanks for the example. Having reflected a bit more, I think, there might potential uses in situations where you want your code to process a task / value from a set of jobs / values, but the order is not essential. Combined with the advantage in the computing speed sets offer this can be indeed quite useful.
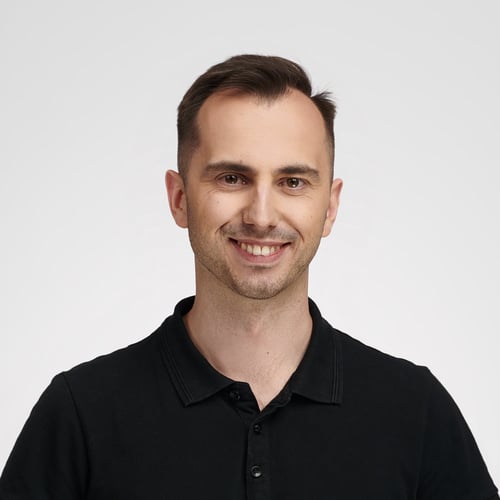
Bartosz Zaczyński RP Team on Feb. 9, 2021
@DoubleA Yes, exactly! In fact, that was my first thought of a real-world application, but figured that there might be an even simpler example. That said, you’re better off using one of the built-in queues for background task processing.
Become a Member to join the conversation.
DoubleA on Feb. 5, 2021
Hi James, thanks for the great tutorial on Pythons sets. Super clear, no fluff, to the point. It is perhaps worth mentioning that all the methods you discussed here are in-place.
Also, on a practical side, what would be a real-life use of the
.pop()
method in the context of sets? Asking, because it removes an arbitrary element from a given set and does not take any arguments. Thanks.