Testing Yourself With a Challenge
00:00
In this special lesson, I have a challenge for you so you can test your knowledge that you gained during this video course. Here is the challenge. Create an empty dictionary named captains
.
00:14
Then enter the following data into the dictionary, one item at a time. The data is "Enterprise"
→ "Picard"
"Voyager"
→ "Janeway"
"Defiant"
→ "Sisko"
. All of these are strings, so don’t forget the quotation marks at the beginning and end of them.
00:34
Once you’ve added data, write two if
statements to check if "Enterprise"
and "Discovery"
exist as keys in the dictionary.
00:44
Set their values to "unknown"
if the key does not exist. Again, "unknown"
is a string here, so don’t forget the quotation marks.
00:54
Next, I want you to write a for
loop to display the ship and captain names contained in the dictionary. So for example, you could print a string, "The Enterprise is captained by Picard"
. And finally, delete the key "Discovery"
from the dictionary.
01:13 If you’re unsure about how to solve some steps of this challenge, you can revisit some lessons of this video course. I would recommend try solving it first on your own, but if you don’t know how to solve it after trying things, just ask in the comments below, and the community will help you out.
01:32 Once you solve the challenge, hop to the next lesson, where I will summarize the video course for you and give you some resources to learn more about dictionaries.
Corbin Nexus on July 15, 2023
May I suggest that Real Python include a solution video for the challenge they create so that we easy can check if we get stuck. To say that we should ask here is not that smart since it can take over a month to get a reply like in this case.
Vassi07, im sure you have solved it by now, but here is a solution that worked for me.
captains = {
'Enterprise': 'Picard',
'Voyager': 'Janeway',
'Defiant': 'Sisko',
}
# List of keys to check if they are in the dictionary
ships_to_check = ['Enterprise', 'Discovery']
# Iterate over each key
for ships in ships_to_check:
# Check if key is in the dictionary. If not, add it with the value 'unknown'
if ships not in captains:
captains[ships] = 'unknown'
print(captains)
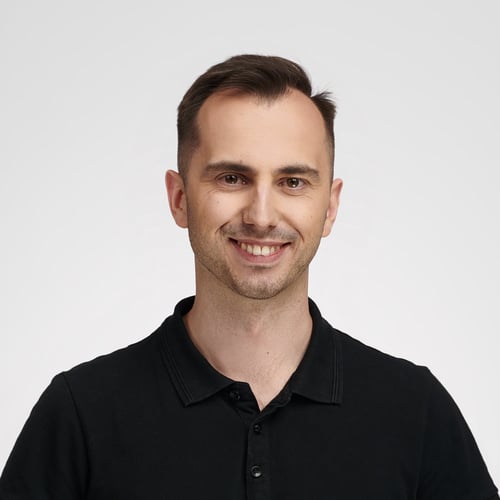
Bartosz Zaczyński RP Team on July 17, 2023
@Corbin Nexus Thanks for the comment. You’re not the first to voice a similar suggestion, so we’ve listened and are working on the solution videos as we speak.
If you still have questions, don’t hesitate to put them here. Someone will usually respond within a business day, as we regularly keep an eye on new comments.
JEX on July 19, 2023
Hi, if you stuck, guess that’s the right sollution.
# 1 - 2
captains = {
'Enterprise': 'Picard',
'Voyager': 'Janeway',
'Defiant': 'Sisko',
}
# 3
if 'Enterprise' not in captains.keys():
captains['Enterprise'] = 'unknown'
if 'Discovery' not in captains.keys():
captains['Discovery'] = 'unknown'
# 4
for ship,captain in captains.items():
print(f"The {ship} is captained by {captain} ")
# 5
del captains['Discovery']
alphafox28js on Nov. 15, 2023
I was not sure how the comparators would work with the .keys method, but it seems to have do the job.
print("----Starship Commanders Method 1----")
import time
start_time = time.time()
captains = {
'Enterprise': "Tiberius",
'Voyager': "Janeway",
'Defiant': "Sisko",
}
if "Enterprise" == captains.keys():
captains['Enterprise'] = "Unknown"
if "Discovery" != captains.keys(): #can also us if 'str' <not in> class.keys(): in replacement of <!= or ==>
captains['Discovery'] = 'Unknown'
for ship,captain in captains.items():
print(f"The {ship} is Captained by {captain}.")
del captains['Discovery']
print("Process Finished --- %s seconds ---" % (time.time() - start_time))
Steinar Martinussen on Dec. 13, 2023
I see most suggestions here use <dict_name>.keys() for interacting with the dictionary in the if statements. It works without as well. Is it considered best practise to use the dicttionary method like .keys() ?
For me this works equally well:
captains = {}
captains.update({"Enterprise": "Picard"})
captains.update({"Voyager": "Janeway"})
captains.update({"Defiant": "Sisko"})
if "Enterprise" not in captains:
captains.update({"Enterprise": "unknown"})
if "Discovery" != captains:
captains.update({"Discovery": "unknown"})
I also like to see the different variations that creates the same results, but I’ve stuck to the actual challenge tasks i.e start with creating an empty list, then add entries one by one.
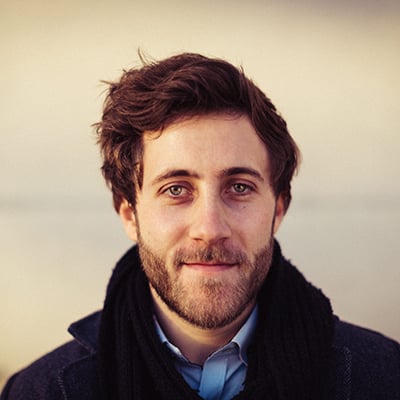
Philipp Acsany RP Team on Dec. 14, 2023
Hi Steinar! Usually, you’d check for the existence of a key in a dictionary without the .keys()
method as it is a little bit more performant.
But if your dictionary is not too big, I personally like to use .keys()
because it’s more explicit.
If you really want to dive deep into the topic, then you can check out How to Iterate Through a Dictionary where similar questions are answered.
Thanks for also sharing your code. Using the .update()
method multiple times is a reasonable way to have control about what you add to a dictionary.
Note however, that you’re not checking for the existence of a key in this if
statement:
if "Discovery" != captains:
captains.update({"Discovery": "unknown"})
In this code, you’re checking if the string "Discovery"
is not equal to the captains
dictionary. The conditional statement returns False
, too. That’s why the code inside the if
statement is executed. But the condition is a “false positive” and can have unexpected outcomes:
captains = {
"Defiant": "Sisko",
}
if "Defiant" != captains:
captains.update({"Defiant": "unknown"})
print(captains)
Can you guess how captains
looks in the end?
Steinar Martinussen on Dec. 15, 2023
Thanks for your quick replies Philipp. The not equal operator was something I saw in a different suggestion. And I now understand that it worked for me because I didn’t have the key ;) My own solution was using the ‘not in’ check. Will go through the lesson you suggest to delve deeper into the Dictionary use.
Mark Pearson on Dec. 23, 2023
As a newbie, even having done the course on IF statements, I was pretty much stuck from the beginning. While I appreciate everyone’s suggestions here, it would be great if the RP team could include the “best practice” solution to the challenge.
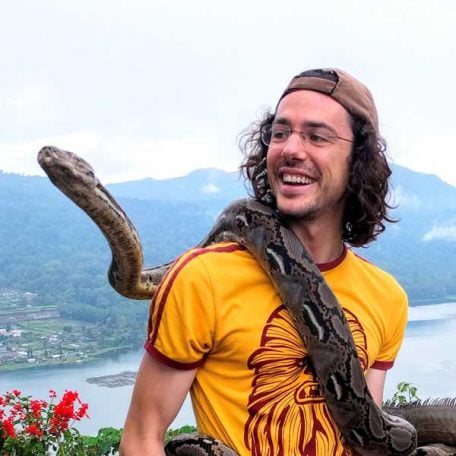
Martin Breuss RP Team on Jan. 3, 2024
@Mark Pearson we have a complete exercises course coming out soon. It’ll go over all the exercises and offer possible solutions so you can work alongside the instructor to solve them. The course is in recording at the moment and should come out soon.
Bebop on Jan. 14, 2024
Great exercise to help hammer home the lessons! Here is what I came up with, with my notes too where I know I messed up. I feel like I did the “set to unknown” part wrong, any thoughts? Code works so I guess it’s right
# Real python dictionary challenge
captains = {
"Enterprise": "Picard",
"Voyager": "Janeway",
"Defiant": "Sisko"
}
if "Enterprise" not in captains:
captains["Enterprise"] = "unknown"
elif "Discovery" not in captains:
captains["Discovery"] = "unknown"
for ship, captain in captains.items(): # This line gives the keys the value of ship, and the values the value of captain
print(f'The {ship} is captained by {captain}')
del captains["Discovery"]
captains
Bebop on Jan. 14, 2024
Terribly sorry I’m not sure how to format my code to share on forums and comments sections like this. I’m looking into it.
# Real python dictionary challenge
captains = {
"Enterprise": "Picard",
"Voyager": "Janeway",
"Defiant": "Sisko"
}
if "Enterprise" not in captains:
captains["Enterprise"] = "unknown"
elif "Discovery" not in captains:
captains["Discovery"] = "unknown"
for ship, captain in captains.items(): # This line gives the keys the value of ship, and the values the value of captain
print(f'The {ship} is captained by {captain}')
del captains["Discovery"]
captains
Figured it out! sorry for the spam
Become a Member to join the conversation.
vassi07 on June 8, 2023
Hi there did someone solve this challenge?