Iterating Over Dictionaries
00:00
You may already know how to loop over lists and tuples. Just like lists and tuples, dictionaries are iterable too, so that means you can loop over them, for example with a for
loop.
00:11 In this lesson, I will show you how to loop over dictionaries.
00:17
Let’s use the dictionary of US capitals again. When you loop over a dictionary with a for
loop, you iterate over the dictionary keys, so for state in capitals:
00:35
Then indent. print(state)
will print "California"
, "New York"
, and "Texas"
. What if you also want to print the city? Well, you already know how to access a value if you know its key, so you can adjust the print()
call and write state, capitals
01:01
and then the state
in square brackets ([]
).
01:08
So when you run your code, you also print the capitals. So California
Sacramento
New York Albany
and Texas Austin
.
01:16
That works, but let me show you another way of accomplishing the same. This other way you will see more often in Python code. And that’s using the .items()
dictionary method.
01:29
The .items()
dictionary method returns a list-like object containing tuples of key-value pairs. That means you can loop over the keys and the values simultaneously.
01:41
Instead of having for state in capitals
in line 7, you can now write for state, city in capitals.items():
print(state
and now instead of capitals
and [state]
, you can print the city
. When you save and run it, then the output is exactly the same like before, but this code is considered more Pythonic.
02:14
Let me explain what happens under the hood. When you loop over capitals.items()
, each iteration of the loop produces a tuple containing the state name and the corresponding capital city name.
02:27
By assigning this tuple to state, city
in your for
loop statement, you ensure that the components are unpacked into the two variables state
and city
. And that’s how you loop over a dictionary.
moandbah on Sept. 4, 2023
How does python know what state and city are?
Thanks!
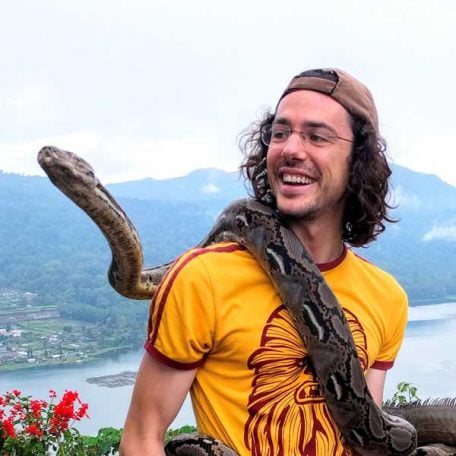
Martin Breuss RP Team on Sept. 4, 2023
Hi @arthur55 and @moandbah. In this example, state
is what’s called a loop variable:
capitals = {
"California": "Sacramento",
"New York": "Albany",
"Texas": "Austin",
}
for state in capitals:
print(state, capitals[state])
While looping over the dictionary keys, Python assigns one key after another to the loop variable. In this case, Philipp called it state
, but you could name it anything else.
Check out the detailed explanation in the lesson on while
and for
loops.
Steinar Martinussen on Dec. 13, 2023
Thanks for the answer Martin. Just wanted to add my comment here as well that it had been the hardest part to understand in this lesson. How the loop variables ‘know’ what is key and what is value.
for ship, captain in captains.items():
print(ship, captain)
Here I create two loop variables, and ask to get them printed for every loop. I just cannot seem to understand why the loop knows what is key, and what is value and that it gets assigned and printed correctly OTHER than it’s just how it is / this just works.
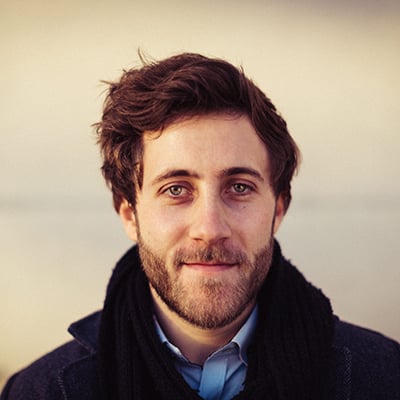
Philipp Acsany RP Team on Dec. 14, 2023
Hi Steinar! That’s such a great question, as there are indeed quite a few things happening here :)
You can investigate the .items()
dictionary method by using it without the for
loop. Here is an example with a different dictionary:
>>> user = {"name": "Steinar", "location": "Real Python"}
>>> user.items()
dict_items([('name', 'Steinar'), ('location', 'Real Python')])
The user
dictionary has the keys "name"
and "location"
, and the values "Steinar"
and "Real Python
”. When you run user.items()
, then Python returns a dict_items
object. You can think of this dict_items
object as a list.
Now, have a closer look at the content of dict_items
:
[('name', 'Steinar'), ('location', 'Real Python')]
Generally speaking, it’s an iterable with two tuples where the first item is the key and the second item is the value of the user
dictionary.
Since .items()
returns an iterable, you can loop over it. Coming back to your example, you could loop over captains.items()
like this:
for captains_data in captains.items():
print(captains_data[0], captains_data[1])
Just like Martin mentioned above, you can name the variable in the for
loop however you like. Note, that I’m just using one variable named captains_data
in this example. That means, that captains_data
remains a tuple with the ship on index 0
(key) and the captain at index 1
(value).
Since every step of the for
loop returns a tuple, you can unpack the tuple right away. And that’s what you commonly do when you use .items()
. That way, you can save yourself an extra step:
for ship, captain in captains.items():
print(ship, captain)
When you unpack the tuple, the order of your variable naming is important. The first variable is always the key and the second variable is always the value. That’s why ship
matches with the keys of your captains
dictionary and captain
matches with the values when you print them.
Become a Member to join the conversation.
arthur55 on Aug. 30, 2023
Hello,
How does Python know what state means when you iterate over the dictionary? It’s the first time the word has been used in the programme (it’s not been assigned any variable so far) and I assume it’s not in Python’s built-in scope either.
Thanks in advance.