Python Basics: Dictionaries (Quiz)
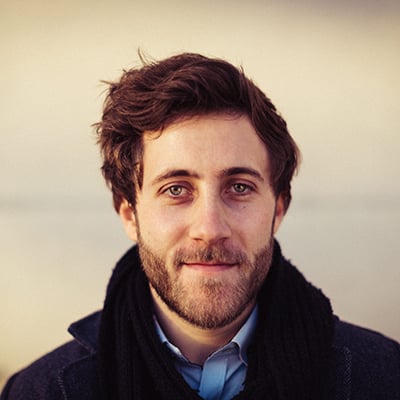
Philipp Acsany RP Team on Feb. 21, 2023
Thanks for pointing the trailing comma out, @9relpyn53! 🙂
In Python dictionaries, you can do both:
- Omit the comma on the last item
- Add a comma to the last item
Both are valid options. I tend to use the trailing comma, because then I don’t need to remember to add the comma when I manually add a new item.
That being said, I should have mentioned this in the course. Sorry for the confusion 🤗
tonypy on Feb. 21, 2023
Excellent, many thanks for clarifying.
Mark Pearson on Dec. 23, 2023
After completing the challenge, I had a question about the FOR statement. I don’t understand how Python knows what “ship” is when it isn’t defined anywhere? If I had used the word “spacecraft” instead of “ship” would Python know I meant the same thing? Thanks.
captains = {
"Enterprise": "Picard",
"Voyager": "Janeway",
"Defiant": "Sisko",
}
if 'Enterprise' not in captains.keys():
captains['Enterprise'] = 'unknown'
if 'Discovery' not in captains.keys():
captains['Discovery'] = 'unknown'
for ship,captain in captains.items():
print(f"The {ship} is captained by {captain} ")
del captains['Discovery']
print(captains)
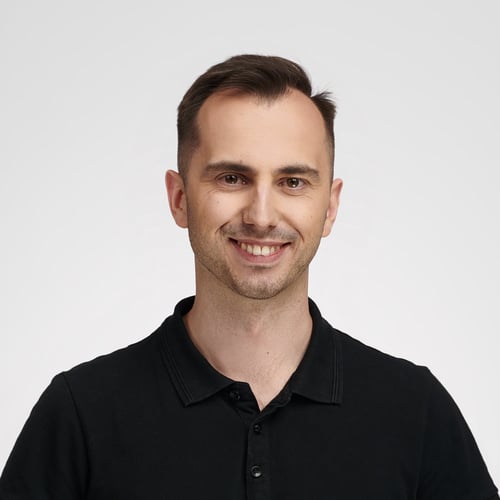
Bartosz Zaczyński RP Team on Jan. 2, 2024
@Mark Pearson In Python, when using a for
loop, the names you assign to the items being iterated over, like ship
and captain
in your example, are essentially variable names and can be any valid Python identifier. Python doesn’t inherently know what ship
or captain
refers to. It’s just following the structure of the for
loop, where ship
and captain
are temporary variable names assigned to the key-value pairs of the captains
dictionary as the for
loop iterates through it.
In other words, when you call for ship, captain in captains.items():
, Python is assigning each key in the captains
dictionary to ship
and each value to captain
in each iteration of the loop.
If you replaced the word “ship” with “spacecraft”, Python would be perfectly fine with it. Your modified loop would look like this:
for spacecraft, captain in captains.items():
print(f"The {spacecraft} is captained by {captain} ")
And it would work the same way as before, just with a different temporary variable name. Instead of ship
, you’re now using spacecraft
to refer to each key.
Become a Member to join the conversation.
tonypy on Feb. 20, 2023
Can you clarify why some of the example dictionaries include a trailing comma after the last entry and others don’t? As an example:
This has a comma after the last key-value pair
"hungry": True
However, if we look at these nested dictionaries
None of the entries after the key-value pair for
flower
have a trailing comma. Why the difference?