Finding a String in a String
00:00 Now that you’ve worked out how to streamline your prints with f-strings, it’s time to find a string within a string.
00:08
One of the most useful string methods is .find()
. As its name implies, this method allows you to find the location of one string within another string, commonly referred to as a substring. To use .find()
, you tack it on the end of a variable or string literal, with the string you want to find typed between the parentheses.
00:28
The value that .find()
returns is an index of the first occurrence of the string you pass to it. Let’s take a look at that in the interactive window.
00:40
Let’s start with a phrase. So the variable phrase
is going to be assigned the string literal "the surprise is in here somewhere"
.
00:48
If you were to use the .find()
method, inside of the brackets, you choose what the substring is that you’re looking for within the string. So in this case, we’re going say, look for "surprise"
.
00:59
It will return the index of the first occurrence of the substring inside the string. So in this case, it’s the fourth index point. So here’s 0
, 1
, 2
, 3
, 4
.
01:14
I know that’s the fifth position, but again, starting from 0
—0
, 1
, 2
, 3
, 4
, that’s where this begins. Great.
01:23
What if the substring doesn’t exist within the string? You’ll get a return of -1
, meaning that it did not find it. So as a note, don’t forget that it requires that the case matches.
01:38
So if I give it a capital "S"
for "Surprise"
, it will return -1
. Let’s try another example. I’ll call it new_phrase
, and it will equal
01:51
"I put a string inside your string"
. So in this particular case, when you say new_phrase.find()
, what will you get if you type in "string"
? So in this case, it’s just the first occurrence. It did not return the second one. That returns the index only of the first occurrence starting from the beginning of the string. And note, if you had a string, let’s say of a phone number …
02:19
if you were to try to find the number 5
as, in this case, an integer, it gives you an error. It’s saying here in the traceback, it’s a TypeError
.
02:29
The value needs to be a string, not an integer that it’s going to search for. So you would require finding a literal string of "5"
, and it’s right there at the very beginning, index 0
.
02:44
Sometimes you may want to replace all the occurrences of a particular string within a string. Since .find()
returns the index of the first occurrence of a substring, you can’t easily use it to perform this operation.
02:58
But strings have a .replace()
method that replaces each instance of a substring with another string. Just like the method .find()
, you tack .replace()
onto the end of a variable or a string literal.
03:11 In this case, though, you need to put two strings inside the parentheses and separate them with a comma. The first would be the substring you want to find.
03:19 And the second is a string with which you wanna replace each occurrence of the substring. Let’s try that out in the interactive window.
03:30
So just like .find()
, you tack the method .replace()
onto the end of the variable or the string literal. Let’s try that out. Let’s say we had a variable called my_story
.
03:43
Okay. And in this case you wanted to replace using .replace()
, and you can see here, it says, put in the old, meaning the substring you’re looking for, to be replaced by the new string. So I’ll say, look for "the truth"
and replace it with "lies"
.
04:03
Kind of cool. Now what happened to my_story
itself? You might remember that strings are immutable objects, and .replace()
won’t alter the my_story
variable. And using the .replace()
method doesn’t alter the string my_story
, if you immediately type my_story
into the interactive window, you’ll see that. If you wanted to change the value of the string my_story
, you need to reassign it. So again, a bit of a refresher on this: you would say, I would like to assign my_story
to, in this case, this replacement. So you’d say, let’s say we want to do the same thing, exchanging "the truth"
for "lies"
.
04:42
So now what’s inside my_story
? You’ll see here that it’s been modified and reassigned. .replace()
will replace every single instance of the substring with the replacement text.
04:53
What if you had another string that was assigned to "some of the stuff"
? So here’s text
. And you wanted a new version of the text
, and you wanted to do a couple replacements.
05:07
Say you wanted to replace "some of"
with "all"
, but you also wanted to replace "stuff"
with "things"
. Well, you’d have to do them one at one at a time.
05:17
So new_text
says "all of the stuff"
, and you could say, okay, well, new_text
, I also want to do new_text.replace("stuff", "things")
.
05:29 So now you’ve accomplished both.
05:35
The .replace()
method replaces every instance of a particular substring with the replacement text. Now, if you want to replace multiple different substrings within a string, then you need to use .replace()
multiple times.
05:51
It’s time to challenge yourself with some new review exercises. In one line of code, display the result of trying to find the substring lowercase, "a"
in the string of "AAA"
. Note, the result should be a -1
.
06:08
Replace every occurrence of the character "s"
with "x"
in the string "Somebody said something to Samantha."
Write a program that accepts user input with input()
and then displays the result of trying to find a particular letter in that input.
06:25
Here’s another, bigger challenge for you. This one is going to require you to write a program, turning your user into elite hacker. Write a program called translate.py
that asks the user for some input.
06:38
Write the following prompt, Enter some text:
, and then use .replace()
to convert the text entered by the user into leetspeak by making the following changes to lowercase letters.
06:50
The letter a
becoming the number 4
, a b
becoming the number 8
, the letter e
becoming a 3
, the letter l
becoming a 1
, and the letter o
becoming a 0
.
07:01
The letter s
becomes a 5
, and the letter t
becomes a 7
.
07:08 Then your program should display that resulting string as output. Below is a sample run of what the program would look like.
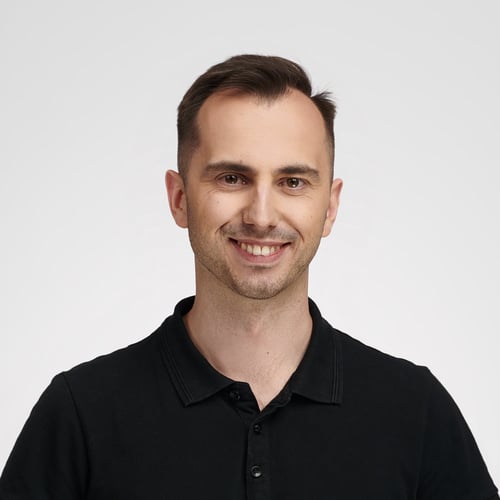
Bartosz Zaczyński RP Team on June 6, 2023
@balakrishna dhulipalla If you meant to ask about a multiline f-string expression, then you can define one by using the triple-quote:
>>> variable = "ipsum"
>>> f"""Lorem {variable} dolor sit amet,
... consectetur adipisicing elit,
... sed do eiusmod tempor incididunt
... ut labore et dolore magna aliqua."""
'Lorem ipsum dolor sit amet,\nconsectetur adipisicing elit,\nsed do eiusmod temp
or incididunt\nut labore et dolore magna aliqua.'
Jagpreet Sahi on Aug. 1, 2024
Would this syntax be acceptable for replacing multiple substrings?
# Replace text in a string
phrase2 = "I'm telling you the truth; nothing but the truth!"
phrase2 = (phrase2.replace("truth", "lie")).replace("you", "us")
print(phrase2)
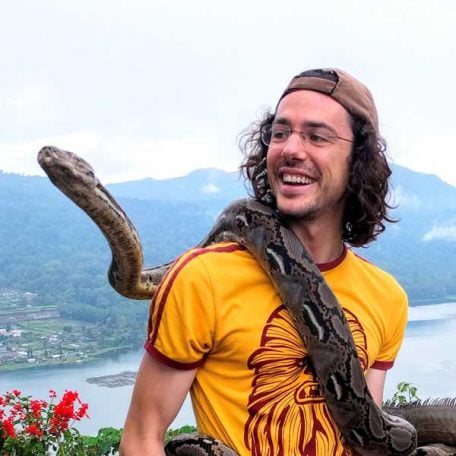
Martin Breuss RP Team on Aug. 1, 2024
@Jagpreet Sahi yes absolutely! This is called method chaining and can be a nice way to reduce code duplication. I’ve used the same approach in the solution to the challenge of the associated exercises course!
Dean Marsh on Nov. 13, 2024
I used a similar approach to Jagpreet but added the “" to keep my lines tidy. (I have not checked with the PEP 8 to see if this is against best practice, though. :-D)
user_input = input("Enter some input: ")
user_input = user_input\
.replace("a","7")\
.replace("b","8")\
.replace("e","3")\
.replace("l","1")\
.replace("o","0")\
.replace("s","5")\
.replace("t","7")
print(user_input)
bobsmithsonian90 on Jan. 9, 2025
what does the \ mean in Dean’s code please?
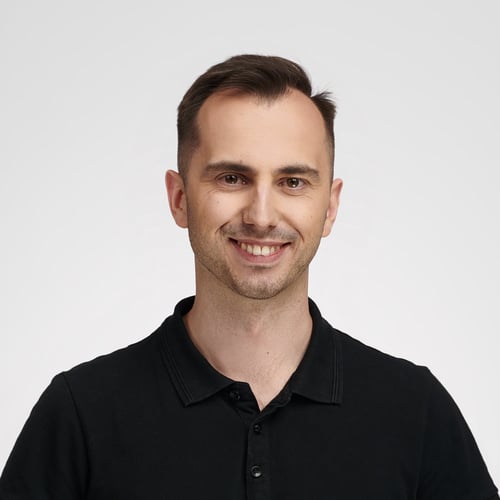
Bartosz Zaczyński RP Team on Jan. 10, 2025
@bobsmithsonian90 This is called an explicit line continuation, which is one way of splittling a long line in Python to make it more readable. Alternatively, you could rely on its implicit counterpart, e.g., by wrapping the entire expression in parentheses:
user_input = input("Enter some input: ")
user_input = (
user_input
.replace("a","7")
.replace("b","8")
.replace("e","3")
.replace("l","1")
.replace("o","0")
.replace("s","5")
.replace("t","7")
)
print(user_input)
Both methods make the code syntactically correct, which would otherwise fail to run.
Victor Ramos on April 10, 2025
Hi everybody, is not possible to use a matrix to solve the hacker problem? just to avoid writing ‘replace’ many times
Become a Member to join the conversation.
balakrishna dhulipalla on June 5, 2023
How can we write a multiline f print statement?