Hello World With Python
In this lesson, you’ll recreate the “Hello, World!” sketch using Python.
For more information about pyFirmata and other languages that work with Arduino, check out the following resources:
00:00 In this lesson, you’ll recreate that “Hello, World!” Arduino program using Python. Up to now, you’ve primarily been using the Arduino language, even though you haven’t dove too deep into it.
00:12 You’ve seen that sketches are written in a language that’s similar to C++. They’re compiled within that IDE. They then get uploaded to the flash memory of the microcontroller.
00:23 Well, how’s it going to be different using Python with the Arduino? Well, you’re going to need a direct connection to the Arduino. Instead of uploading the sketch into its memory, you’re going to remotely control the Arduino via that USB connection.
00:39 This means that the main program will be running on a PC, using Python, then it’s going to use a serial connection—the USB cable—to communicate with the Arduino. So on the Arduino, there has to be a sketch that can read the inputs and the outputs.
00:53 This sketch is going to send input information to the PC—say from buttons or switches or different types of input controls and sensors—but also it needs to receive updates that are coming from the PC and translate those to the outputs on the Arduino. How are you going to accomplish this?
01:11 To do all that, you’re going to use Firmata. It’s a standard protocol for communication with the Arduino. It establishes a serial communication format that allows you to read those digital and analog inputs, and then allows you to send the information to the digital outputs.
01:28 And just like we were discussing in the previous slide, you upload that to the Arduino as a sketch. Let’s talk about that process of uploading it. You’d open up the Arduino IDE and then select File > Examples > Firmata and you’ll see StandardFirmata.
01:47 Let me show you what that looks like here inside the Arduino IDE. You might still have the Blink sketch open, so go ahead and close that. And then again, you’re going to go to File > Examples > Firmata, and there you’ll see StandardFirmata.
02:08 Now go ahead and plug in the USB cable to the PC
02:13 and reconnect the Arduino. You should see a little bit of flashing and the power light come on again.
02:23 And then make sure the appropriate board and port are still selected in the IDE, or re-select them just like you did in the earlier lesson. From Tools, make sure the appropriate board is selected. And also from Tools, make sure that you still have your port selected. Again, don’t forget the name of the port. You’re going to use that shortly.
02:48 That all looks great. Then, with the Firmata sketch ready to go and the USB connection established, you’ll press Upload to send that sketch over to the Arduino. You can verify that it compiles,
03:07 and then use this to upload it.
03:12 And once you press that Upload button, you’ll see a lot more activity over on the Arduino, because it’s a much larger sketch than you’ve used before.
03:18 The transmit and receive light flash for a little bit. Once all that activity is done, it should switch, only the power LED is on.
03:28 Okay, now that all that is working, there’s just one more step before you can start using Python. To work with the Firmata protocol in Python, you need to install the pyFirmata package.
03:39
That’s done using pip
. pip install pyfirmata
. Let me switch over here. I’m going to be using Visual Studio Code. Okay. In my terminal, I’ve created a virtual environment. You don’t need to do that.
03:51
I’m just going to do that because I do lots of tutorials and have lots of different setups I have to create for those tutorials. So in my case, I’m already in my environment and I’m going to run my command to install the pyFirmata, python -m pip install pyfirmata
.
04:17
And then you’re going to create a new file. I’ve created a folder, a directory called Arduino
so I can kind of keep it all together. And you’re just going to create a brand new file that’ll be a Python script, it’s going to be called blink.py
. I’m going to open it up there.
04:35
Okay. What goes into blink.py
? First off, you need to import pyfirmata
,
04:43
and you need to import something from the standard library of time
, import time
. Here, you’re going to create an object called board
.
04:52
And this is literally a connection, using pyfirmata
, to the Arduino.
04:59
And I told you this was coming up—here’s where you choose that USB connection. And mine is '/dev/usb'
—wait, I missed something—'cu.usbmodem'
and then '14601'
, which is the number of the port.
05:21
All right, again, this is going to look totally different on your machine. Just remember, it’s that thing that’s under Port. Again, that text string, if you will. Great. Now make a while
loop, and this is where you’re going to control the board. So, while this is True
, it’s going to keep looping this code around. board
—the one you created a moment ago, right? Now you can talk to a variety of things.
05:46
One of them is .digital
, so that means, “Talk to those digital pins.” Let’s talk to digital pin number 13
! That’s where that LED’s connected, right?
05:55
And you can .write()
to it. Okay. What does .write()
take? .write()
takes a parameter of a value
, and it’s a 0
or a 1
.
06:05
So 1
, you might remember, is On, or 5V. So that will send a value of 5V to .write()
. time
, we’ll have it sleep()
.
06:16
sleep()
takes a parameter of seconds
. We’ll say 1
second, just like that other sketch did. board.digital
—so stay on 1
second, and then .write()
again, put a value of 0
.
06:29
So that’s going to change the pin to 0
, turning the voltage off, or 0V, and make it sleep one more time for 1
second. So again: on, sleep for a second, off, sleep for a second, on. So, blinking, right? Go ahead and save.
06:48
Down here, I just need to run the script and then we can change and look at what happens as you send that information over to the Arduino. Running python blink.py
.
07:03 It has a little bit of flashing of the LED light and the transmit light, but then you can see it receiving the message to turn on pin 13, and light up the LED once a second, and receive a message, turn it off.
07:18
So there’s your blink
Python script. If you halt the script using Control + C like I just did there, you’ll see the LED stay on, because that was the last command that it received, was for it to be on.
07:32 In the next lesson, now that you’ve done a little bit of output from pyFirmata, I’m going to show you how to read digital inputs.
erniej55 on May 24, 2020
I am unable to import pyfirmta and import time and anything beyond the pip install of pyfirmata. I am using python 3.7. I attempted to create the sketch in terminal but getting no module found errors for pyfirmata
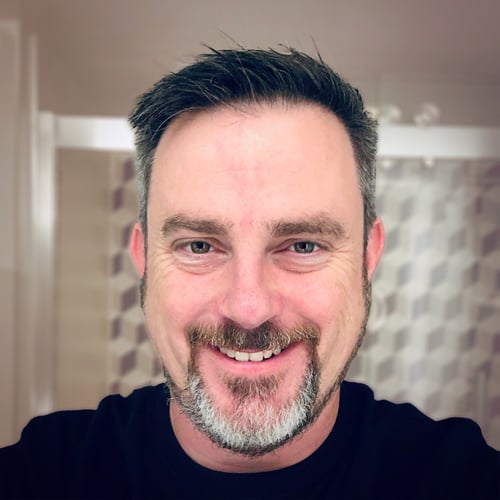
Chris Bailey RP Team on May 25, 2020
Hi @erniej55, I’m a little confused by your question. You say that you have Python 3.7. What computer platform are you one, and what Operating System?
You mention that you are unable import time
, that one is strange as it is a built in module, and you should normally be able to import it without any additional installation.
You note that you are creating the sketch
in the terminal. I don’t understand this. What are you using to write the Python script? Is it a text editor, or IDE, or tool like Thonny?
You can test your that your installation of pyfirmata
worked properly in your terminal, by starting a Python REPL session and attempting this:
import pyfirmata
Are you using Thonny? It would require different steps to install the package, using Thonny’s built in package manager.
Sorry if I’m leaving with more questions than answers, but I want to make sure I can give more directed advice and help.
erniej55 on May 29, 2020
Thanks for responding, I am using Macos Catalina and Jupyter Notebook with Python 3.7. I am new to this, my issue was the path to my board for this exercise. Once I got that corrected I was able to get thru the Hello World with Python.
R-4360 on Aug. 15, 2020
I am lost at the point where you install pyfirmata
R-4360 on Aug. 17, 2020
Have installed Firmata How and where do I install $ Python -m pip install pipfirmata ?? Your instructions are easy to follow except at Point 3:40 minutes.
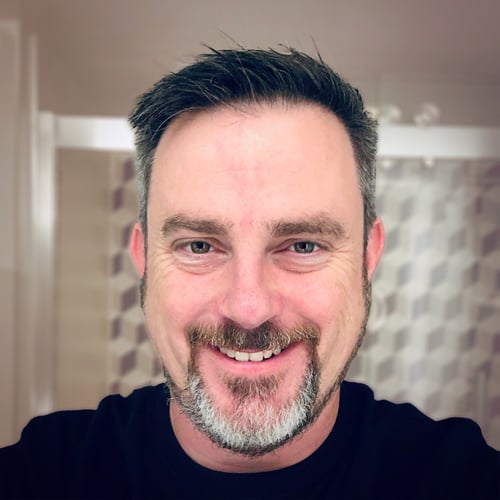
Chris Bailey RP Team on Aug. 17, 2020
Hi @R-4360,
To install pyfirmata, you should be able to use pip
. Things may be a little different depending on your Operating system. The best command to use if you are on a Python 3.6 or higher installation would be:
python3 -m pip install pyfirmata
You need to be in a terminal, I’m using VSCode in my tutorial. To open a terminal window in VSCode I use the key command of holding the control key and pressing the tilde key (~).
If that doesn’t work, can you tell me a bit more about your setup? What computer, Operating System, Python version, and editor are you using?
R-4360 on Aug. 19, 2020
I am 99% electronic/computer hardware person, so loading of software other than user apps can sometimes be a problem. Where do I find VSCode terminal and hove do I install it? Only terminal I know is CMD on windows. I am using Windows 7, as the Arduino IDE gets installed with unrecognizable letters, changing the language has no effect. I have tried multiple versions of the IDE and they all act the same way. My computer spec are as follows: Windows 10\7 Dual boot, AMD FX 9590 Processor, 16GB Memory, 500GB SSD, 3TB Data storage.
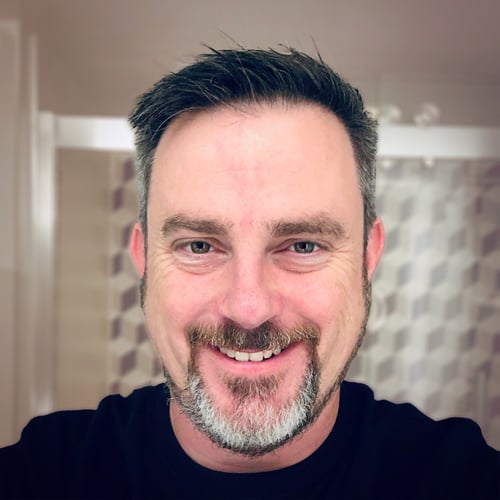
Chris Bailey RP Team on Aug. 19, 2020
Hi @R-4360, Microsoft Visual Studio Code (VSCode) is a free Integrated Development Environment (IDE). You can install it from this website.
Here is a link to another video course on setting it up. And this is a link to the article that course is based on.
How are you currently writing Python scripts (what editor)? What version of Python is installed on your machine?
I’m not sure I understand your comment about:
“Arduino IDE gets installed with unrecognizable letters”.
Were you unable to install the Arduino IDE? Can you run the example “sketch” from the previous lesson? You will need to send the firmata
sketch to the board before you can try to talk to the board using Python.
Maybe the Forums could be a quicker way to get solutions as this is such a slow way to get support for anything beyond a simple question.
R-4360 on Aug. 19, 2020
Regarding “Arduino IDE gets installed with unrecognizable letters” Just under the Arduino 1.8.13 is a line of unrecognizable Characters. It should say “File Edit Sketch Tools Help” Selecting any of the items produces more unrecognizable Characters. This only happens on Windows 10, windows 7 works fine.
lefferson on Dec. 8, 2021
I am confused about where to type python3 -m pip install pyfirmata. You speak of your environment. I don’t know what that means. Do I need SV code to install pyfirmata?
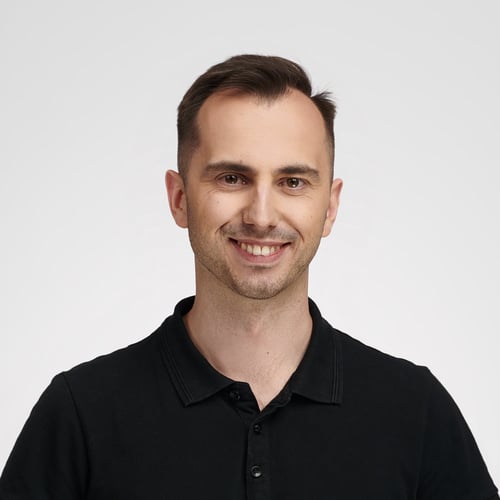
Bartosz Zaczyński RP Team on Dec. 9, 2021
@lefferson Sooner or later, you’re gonna need to learn about virtual environments in Python. Feel free to check out our written tutorial that covers them.
In a nutshell, a virtual environment is a per-project copy of the Python interpreter so that you can install dependencies, such as pyFirmata, independently across your projects without worrying for version conflicts. To use a new virtual environment, you need to create and then activate it in your current terminal session:
$ python3 -m venv venv/
$ source venv/bin/activate
This will activate the virtual environment, making the python and pip commands point to your copy:
(venv) $ python -m pip install pyfirmata
lefferson on Dec. 9, 2021
Still confused. Typing into the IDLE I get -
>>> $ python -m venv venv/
SyntaxError: invalid syntax
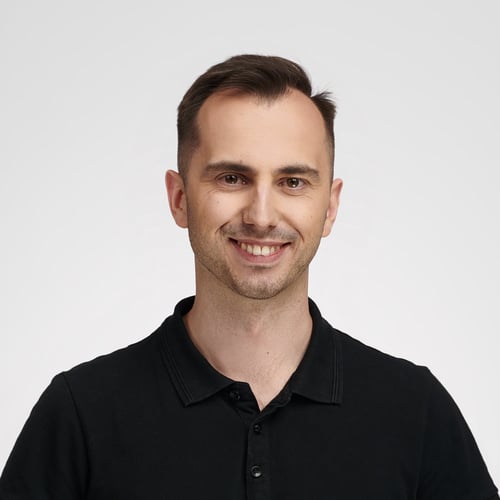
Bartosz Zaczyński RP Team on Dec. 10, 2021
@lefferson IDLE expects Python instructions, whereas you gave it a shell command instead. To create a new virtual environment, you must open the command line, make sure that the python
command is in the PATH environment variable, and type python -m venv venv/
without the dollar sign, which is just a certain convention on Unix-like operating systems.
Become a Member to join the conversation.
nitishnit2016 on April 18, 2020
sir can you explain the process to install python 2.7.9 in windows and how to add pyfirmata lib in it