Interpolating Variables Into a String
With Python version 3.6, a new string formatting mechanism was introduced. This feature is named the Formatted String Literal or f-string. In this lesson, you’ll learn how to do variable interpolation with f-strings. With this feature, you can specify a variable name directly within an f-string literal, and Python will replace the name with the corresponding value:
>>> n =20
>>> m = 25
>>> prod = n * m
>>> prod
500
>>> print('The product of', n, 'and', m, 'is', prod)
The product of 20 and 25 is 500
>>> print(f'The product of {n} and {m} is {prod}')
The product of 20 and 25 is 500
>>> print(f'The product of {n} and {m} is {n * m}')
The product of 20 and 25 is 500
>>> f''
''
>>> F''
''
>>> var = 'Bark'
>>> print(f'A dog says {var}!')
A dog says Bark!
>>> print(F"A dog says {var}!")
A dog says Bark!
>>> print(f'''A dog says {var}!''')
A dog says Bark!
>>> print(f'A dog says {var}!")
File "<input>", line 1
print(f'A dog says {var}!")
^
SyntaxError: EOL while scanning string literal
Here are some resources on f-strings:
00:00 This video covers interpolating variables into a string. For this, you can use a feature that was introduced in Python 3.6 called a formatted string literal. It’s also nicknamed the f-string.
00:14 If you’re interested, it’s covered in much more depth in the course Python 3’s f-Strings: An Improved String Formatting Syntax. I’ve provided links below this video to the video course and to the original article on Real Python that it’s based upon.
00:30 Let me provide you with a quick preview of the feature using variable interpolation. Back here in a REPL, I’ll have you try out variable interpolation. You can specify a variable’s name directly in an f-string literal, and Python will replace the name with its corresponding value. As an example, you want to display the result of an arithmetic calculation.
00:52
You can do this with a straightforward print()
statement, and then inside of it, you’d be going between the different strings and the values.
01:00
So let’s look at that. Let’s say you had a variable n
, which was 20
, and m
, which was 25
, and then you wanted to create an object called prod
, which was n * m
. Great.
01:13
So to print out this, you could say 'The product of'
, then you need to put in a comma (,
), closing your string just before that comma, then we could put an n
, put a comma, start and end another string, and then go back to m
, comma, and go back and forth here between all these. And each time, you would need to close the string, put in a comma, put in your variable, and so forth. Okay. So what would that look like? Okay.
01:40
It says The product of 20 and 25 is 500
. It’s a little confusing to write out. So one of the features that is provided by f-strings is you can do the same thing, but you would start your string with the letter f
at the beginning.
01:55
you would type out f'The product of'
, and then in curly braces {}
put each of your variables.
02:06 And then close your f-string at the end. So, it looks similar but avoids some confusion in, again, opening and closing your strings and all those commas. In fact, one additional feature of it is you could do the product right inside there.
02:20 So f-strings would actually interpret that for you, which is pretty neat. So again, f-strings are covered in a lot more detail in additional tutorials, but I wanted to give you a heads up on them.
02:30
They’re very useful for interpolating variables into strings, as you can see. A couple of notes, you can create an f-string with either a lowercase f
or a capital F
.
02:41
You can also use not only a single quote ('
), but a double quote ("
), or you can also use the longer string format that uses the three quotation marks ("""
) for a longer string. Let’s say you had a variable 'Bark'
. So whatever that variable gets turned into, it will insert it in there.
03:00 So you could do the same exact statement with a double quote,
03:10 or three single quotes, ending your string the same way.
03:15 You just can’t mix them, meaning that if you start with a single style quote, you can’t close it with that style. You need the same style. You’ll see f-strings throughout Real Python tutorials and articles.
03:27 They’re a very handy and modern feature for building strings and interpolating variables into the strings. For the next video, it’s about modifying strings.
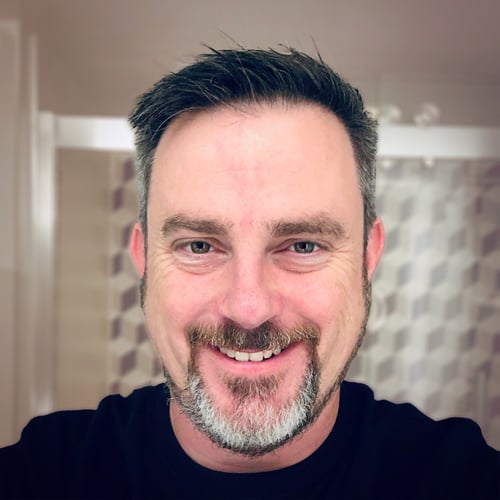
Chris Bailey RP Team on March 2, 2020
Hi @Minh Pham,
I’m using Bpython, and I put a set of links to resources for it below the video of the second lesson in the series. String Operators It is something you usually can simply pip install
, but the resources there will walk you through if you have any issues.
Become a Member to join the conversation.
Minh Pham on March 2, 2020
Hi Christopher,
I would like to know how to get the Python Shell you are using in this video to teach. I got Python from MS Appstore, try to configure IDLE but could not get the “hints” poping up every time I type something.
Thanks Minh