Modifying Strings
Strings are immutable, meaning they can’t be modified. In this lesson, you’ll learn how to accomplish changing strings by generating a copy of the original string instead:
>>> s = 'mybacon'
>>> s[2] = 'f'
Traceback (most recent call last):
File "<input>", line 1, in <module>
s[2] = 'f'
TypeError: 'str' object does not support item assignment
Here’s how to copy a string:
>>> s = 'mybacon'
>>> s = s[:2] + 'f' + s[3:]
>>> s
'myfacon'
>>> s = 'mybacon'
>>> s = s.replace('b', 'f')
>>> s
'myfacon'
If you’d like to learn more, then check out How to Replace a String in Python.
00:00 So, how about modifying strings? Well, basically, you can’t. Strings are immutable.
00:08
Many of the data types you’ve probably seen so far are immutable. Let’s look at this and practice. So, how about a statement like this. Create a string—stick with the string you used earlier, 'mybacon'
.
00:20
What if you went to one of the indexes, like let’s say index 2
, which would be the letter 'b'
, and you tried to change it to an 'f'
?
00:30 You’ll see that string objects don’t support item assignment. You can’t change the contents—in this case, the single character of the string. It’s immutable. You’d get an error.
00:41 So what if you do want to change it? One of the best answers is making a copy instead. So, to try this out, you’re going to generate a copy of the original string that has the desired change in place. And there’s many ways to do this.
00:56
Here’s one way. If you were to take and reassign s
, and you could change up to 2
, but not including 2
, add a new letter you wanted to add,
01:11 and then tack on the rest of the string, you’d get the same result. In fact, there’s a much simpler way to do this with one of the built-in string methods.
01:20
It’s known as .replace()
. If you wanted to change it to 'myfacon'
, you would just say s = s.replace()
and then choose a letter—in this case, 'b'
is going to change to the letter 'f'
. So, you’re not changing the original string.
01:39
You’re returning from a method a copy of the original object with some of it altered, and assigning it to a new object. In this case, you’re reusing the s
object name, but it is a new object.
01:53 The entirety of Section 2 is all about methods that can be applied to strings, and an overview is next.
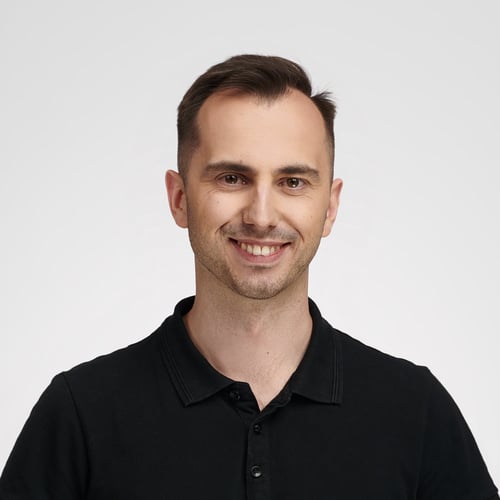
Bartosz Zaczyński RP Team on Jan. 28, 2021
@ugur It’s a long-standing tradition in Python to use names such as spam, ham, egg, or bacon for metasyntactic variables instead of the boring ones like foobar. It’s a reference to a Monty Python comedy sketch entitled Spam. So, I suggest that you start liking bacon 😉
Become a Member to join the conversation.
Uğur on Jan. 27, 2021
It seems that you are big fan of bacon. Don’t you? I strongly suggest you that use a different word something sound nice.