print() and breakpoint()
In this lesson, you’ll learn how to debug in Python using print()
and breakpoint()
.
breakpoint()
is an alias that works in Python 3.7 and newer for import pdb; pdb.set_trace()
, which works in all Python versions. pdb
is Python’s built in debugger and helps you step line by line through your code. If you want to learn more, check out Python Debugging With pdb.
In the video, you saw float("inf")
, which is a concise way to find the largest number (infinity). You can also use -float("inf")
, which finds the smallest possible number. Check out the Python Built-in Documentation to learn more.
00:00
Let’s look at two different ways to debug in Python. First, let’s look at print statements. Let’s write a function max()
that takes in our list and returns the max number without using the built-in max()
function. Let’s do this iteratively.
00:14
Let’s define a max_num
number to be set at 0
, loop through the numbers in our list, and then check if num > max_num
,
00:25
max_num = num
, and then return num
. You might have already seen the bug here, but let’s say it’s an interview. You’re under pressure, you’re trying to work fast, and you miss the bug.
00:36
What do you do? Well, let’s write a test real quick. 1
, -2
, 4
—so, we’re just going to find the max of 1
, -2
, 4
, and then run our code. 4
. So, it worked there.
00:50
Let’s say the interviewer gives you another test, or even better, you come up with better edge case tests to really test your code. Let’s try all negative numbers. -4
.
01:03
That doesn’t seem right, because the max of -1
, -2
, and -4
is -1
. So, something’s wrong here. I actually can’t spot it right away, so I’m actually going to debug. max_num
—oops.
01:17
Put this into the for
loop. Save it, run it. -1
, -2
, -4
. Why did we return -4
? Oh! return num
, here.
01:30
So, that actually wasn’t the bug I intended. There’s another one, but you can see that with printing, we were able to see that num
should really be max_num
.
01:39
Let’s save it, run it.-1 0
, -2 0
, -4 0
, and 0
. Okay, so now we’re returning 0
as the max_num
.
01:46
Why is that happening? Well, max_num
is never changing. We can verify that by printing a string here, like 'entered if statement'
, saving it, running it, and noticing it never enters the if statement.
02:00
That’s because negative numbers are always less than zero. So, what do we need to do? Well, we need to change max_num
to be instantiated to -float('inf')
(negative float 'inf'
).
02:11
Or, you could import the math
module and find the smallest number that way—I think there’s some variable there, but I like float('inf')
. It’s a little bit more explicit. Save it, run it, and we got -1
. So that worked using print statements.
02:25
I like using print statements in for
loops because you see multiple lines of output with just one click. But now, let’s try to use the built-in debugger in Python, which is pdb
.
02:35
So instead of printing, just write breakpoint()
and it’s a function, so you have to call it. And then, what will happen is as it’s executing the code, once it hits the breakpoint()
it will stop immediately there, and then you can print out variables and do all sorts of stuff.
02:51
Running it, it says line 5, if num > max_num:
. It’s right about to execute this code, but it hasn’t yet. You can print out num
, max_num
, or lst
—stuff like that, and then you can type n
to go to the next line—line 6.
03:09
It’s about to execute line 6. max_num
, num
, n
—now, it is about to execute the next iteration in our list. max_num
is now -1
and num
is -1
.
03:27
Now, num
changed to -2
, because we’re on the next element, and max_num
is still -1
, et cetera, like this.
03:35
And then, just press n
a bunch, and we’re at the return statement.
03:41
So, using breakpoint()
is very useful when there are many variables, or if there is very complicated logic, or if you’re really not sure—and so you just put the breakpoint()
in multiple spots in the code and just try to print stuff out—instead of the print statement, where you sort of have to know what you’re looking for.
03:58
One more note is the word breakpoint()
only works in Python 3.7, I believe—and newer. For older versions, you have to type out explicitly import pdb
, pdb.set_trace()
.
04:11
breakpoint()
just calls this—it’s just a wraparound, so you don’t have to type—I don’t know—20 characters? But this is what you would use in Python 2 or any version before Python 3.7.
04:22
I will link a Real Python article on pdb
, which will have all the commands and go way in-depth. I just wanted to expose you all to this great debugging tool. Also, if you’re doing a phone interview on a online code editor like HackerRank, they probably don’t have pdb
.
04:37
So, pdb
is really only if you’re doing it on your computer and they’re screen-sharing, or if you’re on an onsite and you’re using one of their computers. But a lot of times, interviewers may ask, “How would you debug this code?” and you can mention pdb
. In the next video, you will learn about f-strings, which are a new feature in Python 3.8 and newer.
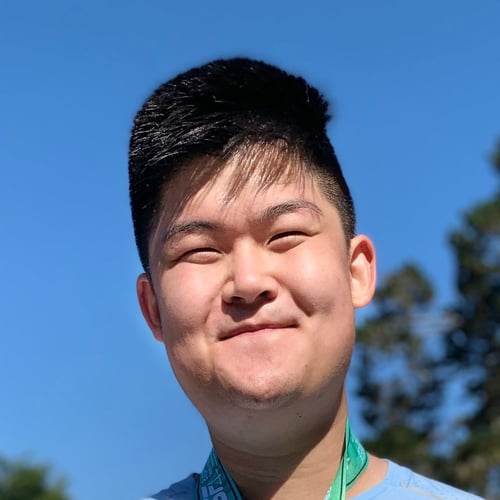
James Uejio RP Team on April 26, 2020
@Piotr thank you for the clarification. I will add a comment to the f-Strings video
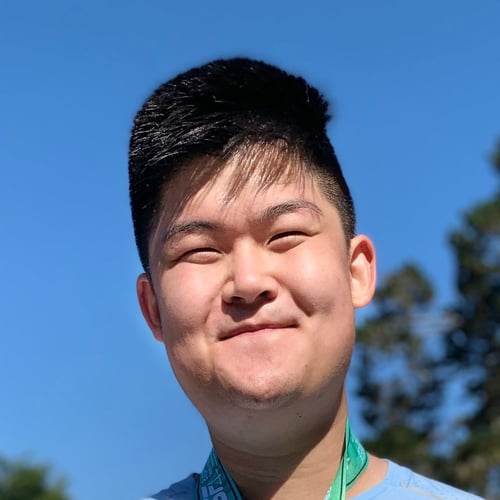
James Uejio RP Team on April 27, 2020
If you want to learn more, here is a Real Python walkthrough video on pdb debugging: Python Debugging With pdb
Also in the video, I use float("inf")
which is a very concise way to find the largest number (infinity). You can also do -float("inf")
which finds the smallest possible number. See the Python Built-in Documentation for more.
Ben Keyes on July 2, 2020
Ranodm question. Why start with -inf when you could just start with the first elelment from the list? I couldn’t think of an edge case where this would break but maybe there is one?
Walid on Jan. 25, 2021
Love the float(inf)
for removing the guess work and not thinking clearly about constraints when comparing.
Become a Member to join the conversation.
Piotr on April 25, 2020
Actually, f-strings were added in 3.6.