Returning Functions From Functions
This lesson covers how to return functions from functions.
The code example in the lesson uses a function called parent()
that contains two inner functions that return a string of text:
def parent(num):
def first_child():
return "Hi, I am Emma"
def second_child():
return "Call me Liam"
if num == 1:
return first_child
else:
return second_child
You’ll see how to access these inner functions and assign them to variables.
00:00
If functions can be defined inside of other functions, can a function also be returned from a function? Sure. It’s time for another example. Continuing in this idea of functions inside of functions, let me have you make a new one. This one will be named parent()
also, so start off with def parent()
. This time you’ll have an argument of num
—it will be used for selecting the child. Right away in the statement, you’re going to start with defining a new function named first_child()
that doesn’t take any arguments, and for its statement, you’ll have it return a string of text that says "Hi, I am Emma"
.
00:41
Then, define a second_child()
function. For that function, I’ll have you return text that says "Call me Liam"
. And last, before we return out of our main statement, returning from the function itself, we’ll set up a little condition.
00:56
If the argument num
is equal to 1
, return the function first_child
—not calling it, but simply returning the function itself. else
—in all other cases—return the other child, second_child
. Again, not calling it, just simply returning it as a function. And close the statement.
01:15
So in this case, if you select parent
, you’ll see that it’s a function
. If you try to access first_child
, it says that it’s not defined
. Same with second_child
.
01:29
But try this instead. Let’s set up a variable and let’s call it first
. Into first
, I’ll have you copy parent()
with the number 1
in it.
01:40
So it’s calling the parent()
function with the argument of 1
.
01:45
That means if num
is set to 1
, it should return first_child
. Okay. It looks like nothing happened. Well, let’s try a second one. Let’s set up another variable, second
, and pass the argument of 2
. So, what are these? We’ll look at first
by itself—not call it, just type in the name and Return.
02:08
It says here that first
is a function
that lives inside the local scope of parent
. Its name is first_child
.
02:19
And then its memory location. What about second
? second
is a function
. It lives inside parent
, and second_child
again is what it’s referencing inside of it. Can you call them?
02:35
Sure! If you call first()
as a function. It prints out 'Hi, I am Emma'
—well, it returns the string 'Hi, I am Emma'
. And second()
—very similar. It returns the string 'Call me Liam'
.
02:50
So these two variables first
and second
are actual functions that are referencing—from the parent()
function, inside of the scope of the parent()
—the first_child
function or the second_child
function.
03:08 And then they’re callable on the outside. Pretty neat! You’ve returned functions from within a function.
03:18 All right. I think you’re ready to talk about decorators, but first, let’s do a short review.
Rynaldo I Bama on March 24, 2019
It explains the concepts clearly and concisely.
snjy1609 on Oct. 27, 2022
def parent(num):
def first_child():
print("hi! from first_child")
def second_child():
print("hi! from second_child")``
if num == 1:
return first_child
else:
return second_child
first=parent(1)
print(first())
Hi All can someone please help me to understand if we call the first reference function why it also print None after printing “hi! from first_child”?
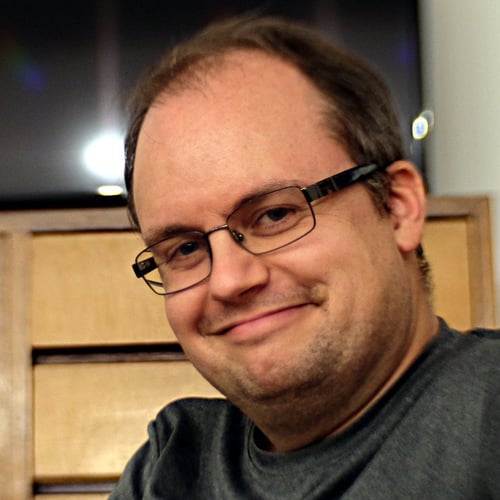
Geir Arne Hjelle RP Team on Oct. 27, 2022
Hi Sanjay.
In your example first
ends up being the inner first_child
function. So doing first()
is the same as doing first_child()
would have been if you defined first_child
as a regular function (not an inner function).
When you call first()
that triggers the print()
within the function itself. When you do print(first())
, you’re first calling first()
which does its print()
. Afterwards, you print the return value of first
. Since first_child
doesn’t have an explicit return
statement, it returns None
(see realpython.com/python-return-statement/#implicit-return-statements).
This behavior is not really related to the inner function. You can see the same thing in a simplified version of your example:
def first():
print("Hi from first")
print(first())
I hope this helps you explore this further!
snjy1609 on Oct. 27, 2022
Thank you all I understood the reason of printing None.as child function is not returning anything, Hence print function is printing None.
Become a Member to join the conversation.
Anonymous on March 20, 2019
Nice and clear explainations though the pace for me is a little faster than I would like.