Scripts, Modules, Packages, and Libraries
Python uses some terms that you may not be familiar with if you’re coming from a different language. Among these are scripts, modules, packages, and libraries.
A script is a Python file that’s intended to be run directly. When you run it, it should do something. This means that scripts will often contain code written outside the scope of any classes or functions.
A module is a Python file that’s intended to be imported into scripts or other modules. It often defines members like classes, functions, and variables intended to be used in other files that import it.
A package is a collection of related modules that work together to provide certain functionality. These modules are contained within a folder and can be imported just like any other modules. This folder will often contain a special __init__
file that tells Python it’s a package, potentially containing more modules nested within subfolders
A library is an umbrella term that loosely means “a bundle of code.” These can have tens or even hundreds of individual modules that can provide a wide range of functionality. Matplotlib is a plotting library. The Python Standard Library contains hundreds of modules for performing common tasks, like sending emails or reading JSON data. What’s special about the Standard Library is that it comes bundled with your installation of Python, so you can use its modules without having to download them from anywhere.
These are not strict definitions. Many people feel these terms are somewhat open to interpretation. Script and module are terms that you may hear used interchangeably.
You can learn more about packages in Python Modules and Packages: An Introduction.
00:00 Python uses a lot of fancy terminology that you may not be familiar with if you’re new to programming or if you come from a different language. Before Python, my background was primarily in C# and Microsoft’s .NET framework.
00:16 I remember trying to learn Python but being kind of confused by all of these buzzwords. My goal in this course is to clear up some of this so that it’s not confusing to you later on.
00:30 A Python script is best defined as a Python file that contains commands in logical order. For this to be considered a script, the program should do something—really, anything—when it’s run through the Python interpreter. To better understand this, take a look at these two programs.
00:51 Both programs contain a function that will return the sum of two values. However, only the program on the right will actually call that function and print its result. The file on the right is intended to be run directly, so we can say that it’s a Python script. On the other hand, the program on the left doesn’t look like it’s actually meant to do anything.
01:16 Running that file will not produce any sort of output, since the function is never being called. It’s not intended to be run directly. The file on the left is better classified as a module. That’s because we can import it into other files to utilize its classes, functions, variables, and other members it’s defined.
01:40
I’m going to change the program on the right so that instead of defining the add()
function, we import it from the module on the left. This has the effect of making the addition function on the left accessible to the script on the right.
01:58 So, long story short: scripts are intended to be run directly, whereas modules are meant to be imported. Scripts often contain statements outside of the scope of any class or function, whereas modules define classes, functions, variables, and other members for use in scripts that import it.
02:22 The words script and module are often used interchangeably, so be aware that you might see other people use them in ways that don’t strictly follow these definitions.
02:35
Packages are a collection of related modules. A package can be an individual Python module, but they’re most often a collection of multiple related modules that come bundled together. This here is the dateutil
package, which allows for performing advanced date-related operations.
02:58
It’s bundled in a folder called dateutil
. Inside there are a bunch of individual modules as well as folders containing other modules, which can be imported into your Python scripts.
03:13 These are modules because they don’t do anything interesting on their own. When you import one into your own script, nothing really happens. You have to use something defined within the module, such as a class, function, or a variable.
03:31
This special __init__
file tells Python that this is a package, potentially containing more modules nested within subfolders.
03:42 We can’t talk about libraries without mentioning the Python standard library. The Python standard library is a large collection of modules and packages that come bundled with your installation of Python.
03:58
That means that you don’t have to download them from anywhere—you can just import them into your scripts and start using them right away. dateutil
is an example of a standard library package, but there are also others, like email
and json
.
04:16 The key takeaway here is that a lot of this is, to some extent, subjective. Scripts are runnable Python programs that do something when executed. Modules are Python files that are intended to be imported into scripts and other modules so that their defined members—like classes and functions—can be used.
04:41 Packages are a collection of related modules that aim to achieve a common goal. Finally, the Python standard library is a collection of packages and modules that can be used to access built-in functionality. In an ideal world, you’d import any necessary modules into your Python scripts without any issues.
05:04 Unfortunately, that’s often not the case. In the next video, I’ll show you what to do when Python can’t find the module you’ve just installed. Then, you’ll learn how to actually install them with Python’s package manager.
Pradeep Kumar on March 8, 2022
Is pandas, sklearn and many others are library or package? It seems they contain __init__.py
but everywhere they are mentioned like library. I am confused. Can you help out, or is it something where there’s no clear line between package or library in Python.
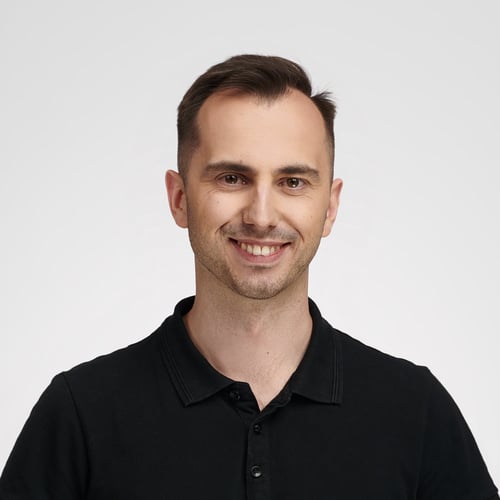
Bartosz Zaczyński RP Team on March 9, 2022
@Pradeep Kumar They’re all libraries that provide a corresponding Python package to import in your code. For example, when you install the NumPy library, you’ll be able to import the numpy
package.
chris-k on July 12, 2022
03:47 dateutil is not a standard library in the slides. please correct me if I am wrong.
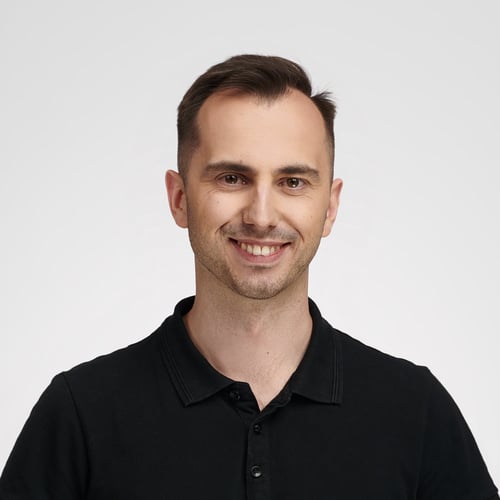
Bartosz Zaczyński RP Team on July 12, 2022
@chris-k You’re right, there’s a typo. It should’ve been datetime
module, which is present in the standard library:
>>> import datetime
Become a Member to join the conversation.
Andrew on May 26, 2020
Excellent explanation!