Setting up the Game Loop
In this lesson, you’ll add the game loop to your code. Every game uses a game loop to control gameplay. The game loop does four very important things:
- Processes user input
- Updates the state of all game objects
- Updates the display and audio output
- Maintains the speed of the game
You will create the event loop just below your current code, starting from line 27:
27# Variable to keep the main loop running
28running = True
29
30# Main loop
31while running:
32 # Look at every event in the queue
33 for event in pygame.event.get():
34 # Did the user hit a key?
35 if event.type == KEYDOWN:
36 # Was it the Escape key? If so, stop the loop.
37 if event.key == K_ESCAPE:
38 running = False
39
40 # Did the user click the window close button? If so, stop the loop.
41 elif event.type == QUIT:
42 running = False
For more information about the pygame
event loop, check out the following resources
from the pygame
documentation:
00:00 In this lesson, you’ll learn about setting up the game loop. Every game that you’ve ever played—from Pong to Fortnite—uses a game loop to control its game play. The game loop does four very important things.
00:13 It processes user input, it updates the state of all the game objects, it updates the display and your audio output, and it also maintains the speed of the game.
00:25 Each cycle of the game loop is called a frame. How quick that you can do everything in each cycle—and that includes the efficiency of your code and how fast your computer runs—will determine how fast your game’s going to run.
00:40 Each of these frames continue to process until some condition to exit the game is met. With this design, you’re going to look for two different conditions.
00:50 The first is that the player collides with an obstacle.
00:56 The other is that the player closes the window.
01:03 That takes us to the actual loop itself, in how it processes events. Events are going to be placed into what’s called the event queue. Every event that you’ll see coming in has an event type. You’ve learned about a couple already.
01:17
Key presses are going to be that event type of KEYDOWN
. Closing the window is the event type QUIT
. To access this list of active events as they’re coming in, you’re going to use this method pygame.event.get()
, and that’s going to pull out the latest event. Okay, it’s time to build that game loop in code.
01:42
We’ll start on line 27, and this is where we’re going to build the game loop. But first you need a variable to keep the main loop running, so create a variable named running
—it’s a very simple Boolean object that’s set to a default of True
. Eventually, you can change it to False
.
02:00
And here at line 30 it starts the main loop. It’s a while
loop, so here you’re using while running
, since it’s set to True
, and then you’re putting your colon (:
) in.
02:14
And here, you’re going to look at every event in the queue using a for
loop, in pygame.event.get()
. The first thing you’re looking for is # Did the user hit a key?
On line 35, if the event.type
is of KEYDOWN
, which we were talking about before, you’re going to do an extra check.
02:46
Here, if event.key
equals that other constant that you imported of K_ESCAPE
, you’re going to flip running
to False
so that next time it hits the while
loop, it will exit. Take your indentation back a little bit.
03:02 On line 40, you’re going to do another check.
03:11
And here on line 40, # Did the user click the window close button?
And then if so, stop the loop. So, it’s an elif
building off the if
from here. Again, you’re looking at another event.type
, and this is the event.type
if it was equal to QUIT
. Again, you’ll turn running
to False
.
03:33
So this is really just setting up the skeleton of the loop with two important tools, because if these are not there, then this will run infinitely. So, one is to check if the keypress in event.type
is of the Escape key—set it to False
.
03:54
And the other is if the player clicks on the window close button, then set it to False
also. Go ahead and save, and now run it again—python sky_dodge.py
—and we should see the window that you created earlier.
04:12 And again, very simple, just a blank window right now. But I could either press the Escape key or press the close button here. I’m going to try the Escape key. Yeah, it looks like it worked just fine. Great!
realneutron on Dec. 20, 2020
I realized what was happening in my case. This step of the tutorial does not specify that pygame.quit()
is needed after the while loop. Otherwise, the loop terminates, but the window and program continues to execute.
I added the pygame.quit()
call and now it works as in the video. The code for this step should be updated and the description needs an errata. Thanks! Great course so far!! Loving it.
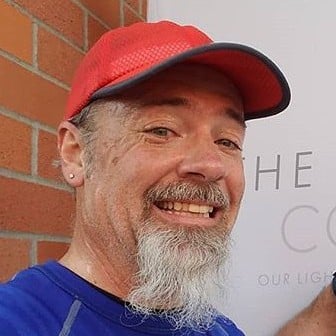
Jon Fincher RP Team on Dec. 21, 2020
Hi realneutron:
I wrote the original article on which this course is based, and I took a look at the issue you reported. This looks like an issue specific to Thonny. I was unable to reproduce it from a shell prompt or within VS Code, but I was able to reproduce it using the latest Thonny installation. I haven’t seen it in any other environment yet.
I can update the article on which the course is based to reflect this for Thonny, and will work with Chris on the proper solution for the course as well.
Thanks for the error report!
–Jon
Become a Member to join the conversation.
realneutron on Dec. 20, 2020
HI! Using Python 3.7.9 on Windows 10 in the Thonny IDE. Detection of the close window button, or the ESCAPE key press is not happening.
My IDE throws out warnings similar to:
Module ‘pygame.locals’ has no attribute ‘K_ESCAPE’
I’m currently googling and not finding anything immediately helpful. I’m curious what your solution is. I’m thinking it has something to do with how the Pygame packaged was installed through Thonny, but I’m not certain. It appears to be installed correctly. I was able to verify through PIP that pygame is installed.