The and Operator
00:00
The and
operator is one of only two binary Boolean operators in Python. Binary means that the operator takes in two inputs. In Python, to apply the and
operator to the inputs x
and y
, you simply type x and y
.
00:17
and
returns True
only when both operands, or both inputs, evaluate to True
.
00:24
Here is the truth table to the and
operator.
00:29
There are only four possible inputs to the and
operator, and the and
operator will return only True
when both of the inputs have a value of True
.
00:41
In all other cases, and
will return False
.
00:46
There’s another way to think about how the and
operator works, and we can divide those into two cases. The first case corresponds to when the first input to the and
operator is True
.
00:59
These are two cases, or two sub-cases. In these two cases, the return value of the and
operator is completely determined by the value of the second input. So notice that in this case, the value of the output of the and
operator is just simply the value to the second input. Again, this is provided that the first input has a value of True
. Then, the output to and
is just simply the value of the second input.
01:31
The other main case is when the first input to the and
operator has a value of False
. In these two sub-cases, the output to the and
operator is False
. So in these two sub-cases, the value of the second input plays no role.
01:50
These two cases correspond to and
’s short-circuiting feature. The idea is that when and
sees that the first input is False
, it is going to immediately return False
and it won’t matter what the second input is. In the upcoming examples, you’ll see how you can take advantage of this short-circuiting feature of the and
operator.
02:14
Although Boolean operators are, by definition, supposed to return Boolean values, Python’s and
operator returns the value of one of its operands.
02:26
The return value of the and
operator is determined by and
’s truth table. So when Python sees the expression x and y
, the first input x
is evaluated. If the Boolean value of x
is False
, or if x
is falsy, then the value of x
is returned.
02:46
This case corresponds to the short-circuiting feature of and
, and corresponds to the last two rows of the truth table to the and
operator.
02:56
However, if the first input x
is True
, then the second input y
is evaluated and the resulting value of y
is returned. This case corresponds to the first two rows of the truth table, and so this is the case where the value of the and
operator is completely determined by the second input—in this case, y
. You’ll see how the short-circuiting feature of the and
operator can be used to shorten your code or to set default values.
03:27
It can also be used to make your program more computationally efficient. If in an and
operator expression the first input is False
, then the second input doesn’t have to be evaluated and you can save some computational time.
03:41 You’ll see an example of this in the upcoming code.
03:46
Let’s start off by generating the truth table of the and
operator. But instead of doing this manually, let’s use the product()
function in the itertools
module.
03:56
So go ahead and import from the itertools
module the product()
function.
04:02
If you’re not familiar with the product()
function, I’ll let you look it up but we’re going to use it to generate all four inputs to the and
operator.
04:12
Go ahead and type for x, y in
and then we’re going to have the product()
of the list that contains both of the Boolean values, True
and False
.
04:24
And then we’re going to pass on a keyword argument of repeat
, and so what this will do is generate the Cartesian product of the list containing both True
and False
.
04:35
Now you want to print what the operator will do, so go ahead and type something like f"{x} and {y}"
and this is going to equal… So here, go ahead and type what the actual and
operator will do to the inputs x
and y
.
04:52
And so remember, x
and y
are going to be taking on the values of True
and False
generated by the product()
function. Go ahead and run that. And you know what?
05:01
Before you do that, put in this tab (\t
) just to make it a little bit nicer. When you print it, it’ll add a little bit more space. Go ahead and run it.
05:10
So, those are the four possible outputs to the and
operator. The only time that the and
operator returns True
is when both operands are True
, and otherwise it’s going to return False
.
05:24
Let’s take a look at an example that demonstrates the short-circuiting feature of the and
operator. Go ahead and define a function that we’ll call print_and_return()
. It takes one input, say, x
.
05:39
It’s going to print a simple statement that just says f"I am returning {x}"
. And then it’s just simply going to return the value x
.
05:52
And let’s add a little bit of space before the "I"
, just so that when the print statement is returned, we get a little bit of space there. Okay.
06:02
Now let’s use this function with the and
operator. Go ahead and type True and
, the function, and let’s pass into the function the value of False
.
06:17
So what’s going to happen here is that the and
operator will first evaluate the first input, which is in this case True
. Then it will pass on and take a look at the second expression, or the second input. It’s going to evaluate that, which is this function, and then it’s going to return the value returned by the function.
06:38
So, in this case, we have this side effect generated by the function that prints the statement, the function returns False
, and so that means that the whole and
operator returns False
.
06:49
If we change this to True
, then in this case the and
operator will return True
. Now, the way that you define this function, we can also pass in, say, 2/3
.
07:05
And so Python will do that computation and return it. And if you did something like this, say 1/0
, then of course you’re going to get a division error.
07:17
Let’s now try this in the case that the first input to the and
operator is False
. Go ahead and type False and
, and then we’ll use the same function, and we’ll pass into the function the value of True
.
07:30
And so what’s going to happen here is that the and
operator evaluates the first input, the first input has a value of False
, and so the and
operator short-circuits. Notice that the side effect of the function, or the function itself, is never evaluated.
07:48
So it doesn’t matter what the second expression is because the first input is False
, the and
operator short-circuits and simply returns that value of False
. That means that, for instance, if you were to actually write in here 1/0
, that never returns a ZeroDivisionError
because that function is never evaluated. All right, this ends this lesson on the and
operator. In the next lesson, we’ll take a look at the or
operator.
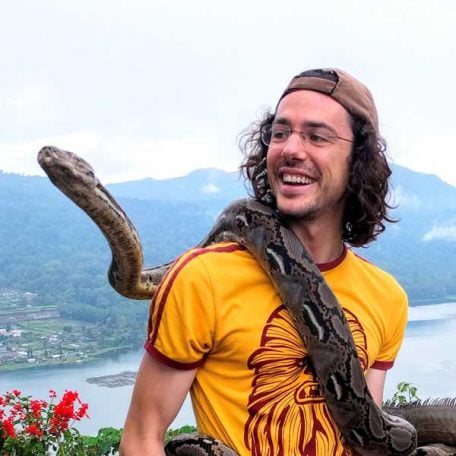
Martin Breuss RP Team on Feb. 2, 2023
@jmaflannery82 see also my other comment.
Become a Member to join the conversation.
jmflannery82 on Feb. 2, 2023
This video uses concepts and constructs that we have not yet learned at this stage of the “Learning Path”.