The Python Boolean Type
00:00 It wasn’t until 2002 that the Boolean class was added, and if you’re interested in knowing what the thought process was when adding the class, check out PEP 285.
00:12 One of the main reasons why the Boolean class was added was to standardize the process of deciding when a condition is either true or false. Remember, a lot of your code is all about making decisions and then taking the appropriate action.
00:27 The Boolean class is implemented as a subclass of the integer class, and so it inherits all of the mathematical operations that exist in the integer class.
00:38
The constructor function to the Boolean class is called bool()
, and bool()
can therefore be used to transform an object to a Boolean data type.
00:48 Of course, unless the object that you’re trying to transform is a Python built-in object, your class has to define a way to transform the object into a Boolean type.
00:59 We’ll discuss this in a future lesson.
01:03
There are only two possible values of an instance of the Boolean data type—either True
or False
. True
and False
are also keywords, which means that you can’t use them as variable names or identifier names in your programs. When typecasted into integer objects, True
evaluates to 1
and False
evaluates to 0
.
01:26 So since the Boolean class is a subclass of the integer class, you can do things like this.
01:33 Now, this may not seem all that useful, but we’ll look at an example where you may actually want to take advantage of this. All right, let’s take a look at some examples.
01:44
Let’s start by taking a look at the help()
documentation for the Boolean class.
01:49
The constructor to the Boolean class is called bool()
and if the input to the Boolean constructor is x
and x
has a value or evaluates to a value of True
, then the constructor returns True
and otherwise it’s going to return False
. In an upcoming lesson, we’ll talk about how Python decides when an object should evaluate to True
and when it should evaluate to False
.
02:15
The two built-in constants True
and False
are the only two instances of the Boolean class, and the Boolean class is a subclass of the integer class.
02:26
Now, since the Boolean class is a subclass of the integer class, it inherits all of the mathematical operations that exist in the integer class. Now, in addition to these operators, the two most important operators that are particular to the Boolean class are and
and or
, and we’ll talk about these in-depth later on in a video.
02:49
Now, for good measure, let’s verify that the Boolean class is indeed a subclass of the integer class. So is Boolean a subclass of int
? And we get True
.
03:00
Let’s also verify that True
and False
are instances of the Boolean class. So is True
an instance of bool
?
03:07
We get True
. And is False
an instance of bool
? And we also get True
. Now, even though that True
evaluates to the integer 1
when typecast into an integer, the integer 1
is not an instance of the Boolean class, so we get False
in this case.
03:25
And if we try the same thing with the 0
integer, we’re also going to get False
.
03:31
Let’s verify that True
and False
are built-in keywords, so let’s import the keyword
module. The keyword
module contains a list of all of the keywords in Python.
03:43
The list is called kwlist
, or keyword list.
03:49
And at the very top of this list, along with the None
keyword, is False
and True
.
03:58
Let’s now see an example that demonstrates how True
and False
behave when they’re integers. If you go ahead and typecast True
as an integer, you’re going to get 1
. And then if you do the same thing for False
, you’re going to get the integer 0
. So that means, for example, if you were to say what’s True
plus True
plus, let’s say, False
and then maybe you want to add one more True
, you’re going to get 3
, right?
04:26
So True + True + False + True
is 3
. This may not seem all that useful, but you can actually take advantage of this when you want to determine the number of occurrences of a Boolean condition in a list. Let me show you an example.
04:43
So, here’s your problem. You have a list of integers—say, stored in a list called codes
, and maybe these integers represent some sort of code—and you want to know how many of these integers are divisible by, say, 3
.
04:58
Let’s write down a few more and let’s introduce a new variable, N
. So N = 3
. So again, the problem is how many of these integers are divisible by 3
?
05:11
Why don’t you use a list comprehension? You loop over the integers of the codes
list, apply the modulo operator (%
), and whenever you get a 0
, you know that you have a multiple of 3
.
05:23
Start off by writing [for c in codes]
,
05:28
and you want to apply the modulo operator to c
,
05:35
and you know that you’re going to get an integer, and whenever you get 0
, you have a multiple of 3
. So use the equality operator (==
). This is a Boolean operator, which we’ll discuss later on in a future lesson. But for the moment, what this will do is whenever we get a 0
, then that’s a multiple of 3
, and so this comparison operator, this equality operator, will return True
exactly when we have a multiple of 3
. I’m certain you’ve probably used this operator before, but we’ll talk about it later on in a future lesson.
06:10
So, what happens when you run this? Well, you get a list of True
and False
values, or a Boolean list. And you know that when you sum up Booleans, True
evaluates to 1
and False
evaluates to 0
. So if you go ahead and sum this list, you’re going to get 4
.
06:29
So this tells you that four of those integers are divisible by 3
or a multiple of 3
. And in this case, you can see them exactly.
06:36
It’s the integer one in the list, the third one, the fourth, and the fifth one. So, this is a nice, clean way of determining how many occurrences of some sort of Boolean expression—in this case, how many of the integers are a multiple of 3
—using the fact that True
evaluates to 1
and False
evaluates to 0
and then summing up this list of these True
and False
values.
07:03
All right! Well, that ends the first lesson on the Boolean data type built into Python. In the next lesson, we’ll take a look at the Boolean operators, and in particular, we’ll focus on the operators not
, and
, and or
that are built into Python and are by far the most widely-used operators.
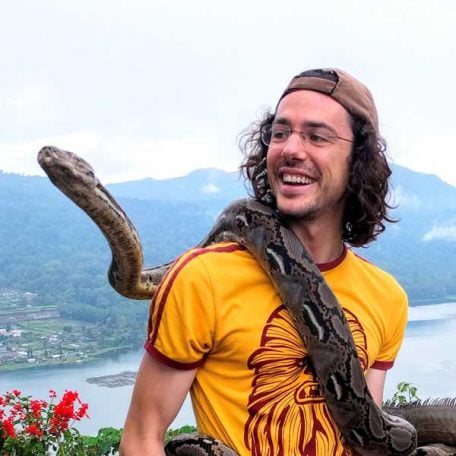
Martin Breuss RP Team on Feb. 2, 2023
@jmflannery82 thanks for the feedback. Which learning path did you follow where you got to this course?
If you want to follow a beginner learning path that starts from scratch and introduces you to Python step by step, then you can check out the Python Basics Learning Path.
Become a Member to join the conversation.
jmflannery82 on Feb. 1, 2023
Please remember that at this point in the learning path, we have not learned what a “comprehension” is.