Using .blit() and .flip()
In this lesson, you’ll use .blit()
and .flip()
to get your newly created Surface
to display on the screen. The term blit
stands for Block Transfer, and .blit()
is how you copy the contents of one Surface
to another. You can only .blit()
from one Surface
to another, but since the screen is just another Surface
, that’s not a problem.
Here’s how you draw surf
on the screen:
54# This line says "Draw surf onto the screen at the center"
55screen.blit(surf, (SCREEN_WIDTH/2, SCREEN_HEIGHT/2))
56pygame.display.flip()
The reason why the image looks off-center is that .blit()
puts the top-left corner of surf
at the location given. If you want surf
to be centered, you would update the code like below:
54# Put the center of surf at the center of the display
55surf_center = (
56 (SCREEN_WIDTH-surf.get_width())/2,
57 (SCREEN_HEIGHT-surf.get_height())/2
58)
59
60# Draw surf at the new coordinates
61screen.blit(surf, surf_center)
62pygame.display.flip()
For more information about the .blit()
and .flip()
, check out the following resources from the pygame
documentation:
00:00
In this lesson, you’ll start using .blit()
and .flip()
. How do you get your new Surface
that you just created to appear? The method .blit()
—blit
stands for Block Transfer—and it’s going to copy the contents of one Surface
onto another Surface
.
00:17
The two surfaces in question are the screen
that you created and the new Surface
. So, .blit()
will take that rectangular Surface
and put it on top of the screen. The method takes two arguments.
00:31
One is the Surface
that you want to draw, and then the other is the location at which you want to draw it on the source Surface
. And along with block transfer, using .blit()
, how are you going to get it to draw those changes onto the screen itself?
00:48
For that one, you’re going to use pygame.display.flip()
. .flip()
updates the entire screen, and you’re going to put it inside of the game loop.
00:57
That basically means, at this point, update the whole screen, including everything that’s been drawn since the previous .flip()
. Okay, it’s time to add those two new features.
01:10
At line 54, again within the while
loop of the main game loop…
01:25
you’ll use screen.blit()
, and then again, you’re going to say, “What are you drawing onto the screen?” surf
, the object you created a moment ago.
01:32
And then, “Where?” Well, you’re going to use SCREEN_WIDTH
, but you’re going to divide it by 2
and then SCREEN_HEIGHT
, and divide it by 2
, therefore putting it in the middle of the screen, right? Again, that’s a tuple.
01:44
So that will block-transfer the one surface onto the other, so then the next step is to do pygame.display.flip()
. Great. Now down here in the terminal,
01:57
if you run sky_dodge
again, from python sky_dodge.py
, you’ll see here that it’s drawn your new object surf
02:09 onto there. Huh, though it’s not quite in the center, is it? Okay, so to quit, you can use the button or Escape. I’m going to press Escape. Let’s fix that. It’s a little off-center. So what you could do—I’m going to add a couple lines—
02:32
you’re going to create a new variable called surf_center
.
02:36
It starts with the SCREEN_WIDTH
again, but this time you’re going to subtract the Surface
width, and there’s a method called .get_width()
. When you do that and then divide that number by 2
, you’ll get the actual center of the screen, as far as the width.
02:53
Now, do the same for the height. So again, subtracting the .get_height()
from SCREEN_HEIGHT
—so again, it was like, 50
, so that’s going to subtract that 50
from that number to give you the more accurate value. Divide that by 2
.
03:10
So that will be a new tuple that you can then use here on line 61. So, erase this and put surf_center
instead. Go ahead and save, so again, it’s going to .blit()
that on there—surf
onto screen
at surf_center
. And then here, you’re going to .flip()
to update. Okay, running one more time—oh, make sure I’m saved. Make sure you’re saved.
03:34 Cool. There! That looks much more centered. Awesome! Congratulations! You finished Section 2 and you’re ready to move on to Section 3, where you’re going to get a chance to start working with sprites and you’ll start putting lots of things on the screen.
shevachp on April 22, 2020
Hi Chris, Am really enjoying this tutorial. I was just wondering is there any reason to make the black rectangle another surface instead of just pygame.draw.rectangle(....)?
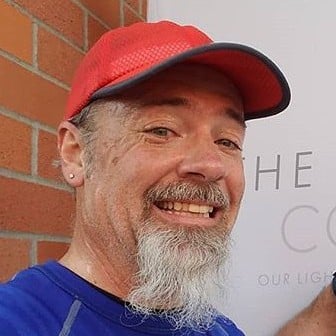
Jon Fincher RP Team on April 22, 2020
shevachp, you will be using the rect in a later step to draw an image on the screen. This step prepares for the next.
David Alvarez on July 18, 2020
I have to complete the whole tutorial but just wondering right now why you create all this objects inside the game loop. Doesn’t this make things way more inefficient? I understand is an introductory tutorial, that I’m enjoying a lot, but performance is key in game programming so better has it present from the very beginning. Maybe I’m missing something anyway, so please let me know if I’m wrong here.
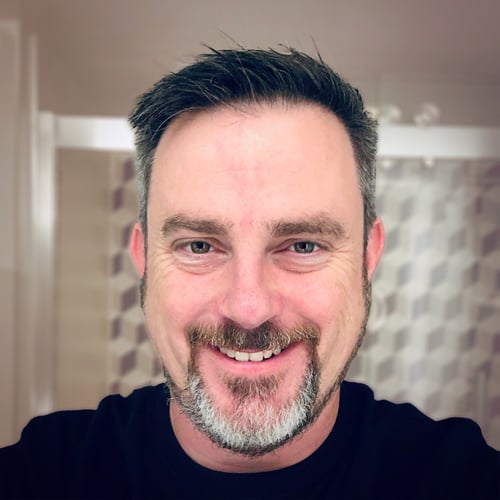
Chris Bailey RP Team on July 20, 2020
Hi @David, You are correct. As the tutorial progresses you will start to create the objects outside the loop, and only call to update various objects within the loop.
David Alvarez on July 25, 2020
Thank you @Chris I was kind of impatience. Thank you for your answer.
Become a Member to join the conversation.
John Berliner on March 23, 2020
Just curious why you introduced get_rect() here. I assume you’ll be using it later, e.g. to determine the coordinates of sprite on the screen, but unless I’m missing something, it’s not being used yet? Great tutorial BTW.