Arithmetic Operators and Expressions
00:00 In this lesson, you’ll learn about the available arithmetic operators in Python and how to combine them to make mathematical expressions.
00:09
There are seven arithmetic operators in Python, which are all binary, meaning they take two arguments each. Both arguments of an operator are often called its operands. For example, the +
(plus) operator adds the left operand, 5
, to the right operand, 2
.
00:26
In Python, it’s customary to surround operators like this with a single space character on both sides. The -
(minus) operator, on the other hand, subtracts the right operand from the left one. Note that both the +
and -
operators have their unary counterparts, which take just one operand.
00:44
A unary -
flips the sign of a number, while a unary +
has no effect but exists for symmetry. You can only use the unary form of these operators as a prefix, which appears to the left of the number.
00:57
The *
(star) operator multiplies one operand by another. There’s also a unary *
operator in Python, but it has a different meaning outside the arithmetic context, so you’re not going to cover it in this course.
01:11
A **
(double star) operator represents exponentiation, which is a form of multiplication. You take the left operand and multiply it by itself the number of times specified by the right operand.
01:22
A /
(forward slash) character represents a division operation. In this case, the left operand becomes the dividend, while the right one the divisor.
01:32
The result of such a division is always a floating-point number, even when both operands are integers. If you’d like Python to automatically round the result of a division to the nearest whole number, then you can use the //
(integer division), also known as the floor division. Floor is a mathematical function, which does the rounding.
01:51
The last arithmetic operator in Python is the %
(modulus), which is closely related to division. It calculates the remainder of a division, so in this example, 5
divided by 2
has a remainder of 1
because 5
equals 2
times 2
plus 1
.
02:08 It’s worth noting that all of these operators will accept integers as well as floating-point numbers as operands. Most will also work with complex numbers, fractions, and decimals. You can even mix them together in a single operation, which will make some of them subject to promotion.
02:25 Let’s take a quick look at it in IDLE.
02:29 When you add two integers, then you also get an integer result. However, once you add an integer to a floating-point number or the other way around, then Python will first promote that integer to a float and return a floating-point result.
02:44 That’s because computers can only add numbers of compatible data types. Even if two data types, such as ints and floats, represent the same value, they can’t be manipulated directly.
02:57 Although that wouldn’t be 100 percent technically accurate, you can think of integers as a subset of floats.
03:03 It’s possible to promote an integer to a float without any loss of information, but the reverse isn’t true, because you lose the fractional part. Promotion works for all arithmetic operators, even those that are normally expected to return integers, such as the in integer division or the modulus.
03:23 In the case of the integer division, you still get a whole number as a result, but it’s represented using the float data type that has no fractional part.
03:31 The modulus, on the other hand, can sometimes give you a fractional remainder. The regular division is kind of special because it always returns a floating-point result, even when both of its operands are integers.
03:44 There’s one exception though. What happens when you try dividing a number by zero? In mathematics, such an operation is undefined. When you try this in Python, then you’ll immediately get an error, so watch out for it.
03:59
Regarding the integer division, you might be thinking that all it does is discard or truncate any fractional part of the resulting number. You can simulate this behavior by calling the built-in int()
function on the division’s result. For example, when you divide 3
by 2
, you get 1.5
.
04:18
Wrapping this in a call to the int()
function will get rid of the fractional part and leave only the whole part, which is 1
. This is virtually the same as doing an integer division of 3
by 2
.
04:29
No surprises there. However, things may become surprising when you throw negative numbers into the mix. For instance, -3
over 2
is -1.5
.
04:40
When you truncate the fractional part with int()
, you’ll get a -1
as a result. But when you use the integer division for the same operands, you’ll get a -2
.
04:52 This has to do with the floor function used for rounding behind the scenes, which behaves differently for positive and negative numbers. Note there are a few different strategies for rounding numbers in Python.
05:04 You’ll take a look at one of them in an upcoming lesson.
05:09
The **
operator needs a little explanation too. Normally, it’s a shorthand notation for multiplying a number by itself a few times. For instance, 5
raised to the power of 3
gives the same result as 5 * 5 * 5
. When you use negative exponents, the result will be the same as 1
over the corresponding positive exponent.
05:33
You can even use fractional exponents, such as 0.5
, which can be helpful in calculating roots of a number. In this case, you calculated the square root of 2
.
05:45
The %
operator is perhaps the most complicated one. As mentioned before, it calculates the remainder of the left operand divided by the right one.
05:54
For example, 5
divided by 3
has a remainder of 2
. 20
divided by 7
has a remainder of 6
.
06:03
But 16
is divisible by 8
, so it has no remainder, in which case the modulus returns 0
. Noted that because the modulus performs a division, its right operand cannot be equal to 0
, as that will give you a familiar error. Also, you might get surprising results when you start using negative operands.
06:24
The remainder of 5
divided by -3
is -1
. -5
divided by 3
has a remainder of 1
.
06:32
But when both operands become negative, you suddenly get -2
.
06:37
The formula for the %
operator in Python takes advantage of the integer division, which uses the floor function for rounding. That’s why you’re getting seemingly inconsistent results when using negative numbers as operands. Although Python comes with a floor function, you won’t cover it in this course. If you’d like to learn more about it, then you can check out the Real Python tutorial on rounding numbers in Python, which was mentioned earlier in the overview of the numeric types.
07:07 Okay, now that you know how to use the individual operators in Python, you can start making some more complicated arithmetic expressions with them. For example, you can combine addition and multiplication in a single expression.
07:20
There’s no upper limit on the number of operators you can use per line of code. The order in which Python evaluates the individual operations in an expression like this follows a well defined operator precedence. The *
operator, for example, has a higher precedence than the +
operator, so it gets evaluated before the addition, even though it appears further to the right.
07:44 Python follows the rules of everyday arithmetic that you may remember from school. If you’d like to change the default order or make it more explicit, then you can group a given subexpression using parentheses. Now, the expression has been evaluated to a different result because you changed the order of calculations.
08:02
Python calculated the operation inside the parentheses first, despite a lower precedence of the +
operator.
08:13 You can visit the official Python language reference to learn about its operators and their precedence. When you do, you’ll find that Python has many kinds of operators, not just the arithmetic ones, but at this stage you can ignore most of them while only remembering those that you already know. As you can see, the exponentiation operator has the highest precedence, while the addition and subtraction ones have the lowest precedence.
08:39 Some operators appear on the same row in a table because they share equal precedence, in which case Python will evaluate them left to right. To change the default order, you can group the operators using parentheses, which always take precedence.
08:57 Knowing about Python’s math operators and their precedence lets you use IDLE as a rudimentary calculator. However, unlike a pocket calculator that you might be used to, Python can sometimes lie to you due to the representation error of floating-point numbers, which you’ll learn about in the next lesson.
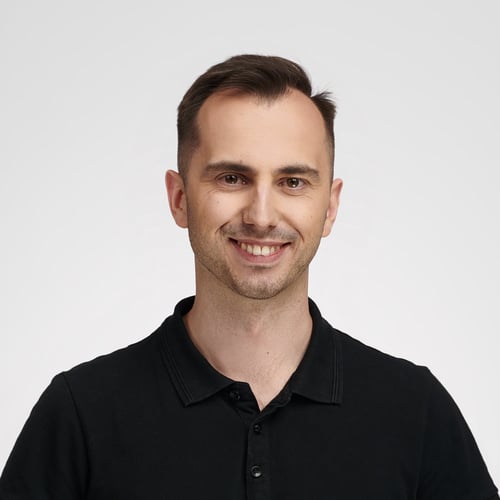
Bartosz Zaczyński RP Team on Oct. 25, 2023
@s150042028 In Python, both the plus (+
) and minus (-
) operators have binary and unary versions. The word “binary” in this context means that the operator takes two operands or arguments, while “unary” implies only one argument.
When used with two operands, the plus operator adds them together, which can mean different things depending on the types of the operands:
>>> 5 + 2
7
>>> "bat" + "man"
'batman'
When used with numbers, the plus operator performs the addition. For strings, on the other hand, the plus operator is defined as the concatenation operation.
The unary version of the plus operator does nothing, so numeric literals written with or without it are considered equal:
>>> +2
2
>>> +2 == 2
True
The minus operator is only defined for numbers. More specifically, the binary minus operator performs the subtraction of two numbers, whereas the unary minus operator flips the sign of the number, or more generally, the arithmetic expression that follows:
>>> 5 - 2
3
>>> -2
-2
>>> -(5 - 2)
-3
Let me know if that clears it up for you!
s150042028 on Nov. 2, 2023
Hello,
I am still confused, but I will read it again. However, I am not feeling comfortable with this structure of the course. I mean, there are no projects or exercises. Watching videos is not a useful way for me. There is a 90% chance that I will cancel my membership due to this poor method of teaching. There should be an interactive environment with videos, examples, and exercises. Do you have any suggestions, please?
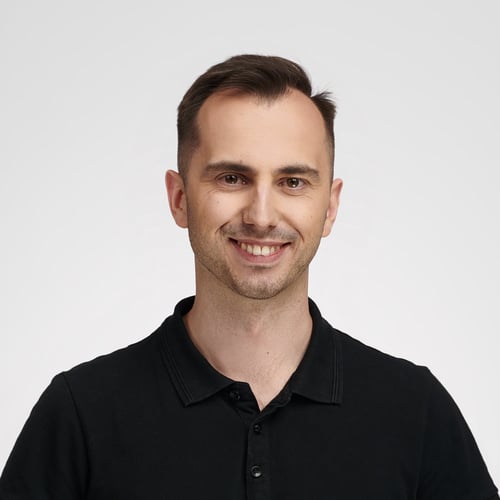
Bartosz Zaczyński RP Team on Nov. 3, 2023
@s150042028 Thank you for your feedback. We appreciate you taking the time to share it with us, as we’re continually working to improve our course offerings. Your comments are immensely beneficial in that process!
Please note that this video course is part of a series based on the Python Basics book. We’re working hard to eventually accompany each course in this series with a separate hands-on course that contains practical exercises. We’re almost done with recording all of them, but some will require a little bit of patience. The exercises course for this particular one will come out next Tuesday, November 7.
Once released, you’ll have a chance to solve the accompanying exercises yourself and then confront your solutions with our walkthrough, where we explain each step in detail and the thought process behind it.
In the meantime, I’d highly encourage you to take advantage of other benefits that Real Python offers to its members. If you haven’t already, you can ask for help on our Slack community, or you can come to Office Hours where we can address your doubts in real time. It’s a weekly webinar where we often talk about various Python-related concepts, including solving actual programming challenges that people face at work through screen sharing. This would be a perfect opportunity for you to get some hands-on experience and interact with our team on a personal level. We hope to see you there and look forward to helping enhance your learning experience with us.
Davide70 on Nov. 18, 2023
Difficile da capire un pò, ma solo per chi è agli inizi, ovviamente. Basta rileggere varie volte è diventa molto più chiaro. Fin’ora ottimi videi. Complimenti.
Become a Member to join the conversation.
s150042028 on Oct. 24, 2023
What do you mean by unary +2 in the second example? 5 - 2 = 3. I can’t understand unary. Please explain it.