Integers
00:00
In this lesson, you’ll take a deep dive into one of the most common numeric types in programming, the integer data type, which Python calls int
.
00:08 If you’d like to follow along in an interactive Python interpreter session, then go ahead and start IDLE now.
00:16
An integer is a whole number with no decimal places. The quickest way to create an integer in Python is by writing an integer literal consisting of digits that appear literally in your code. For example, typing 42
in IDLE creates an integer.
00:32
You can check the type of such a literal, which Python refers to as int
. Because integers are whole numbers, they don’t come with a fractional part, so as soon as you include the decimal point in your literal, you no longer create an integer.
00:47
Even if the fractional part of your literal is equal to 0
, like in the example, the result will be a floating-point number, which you’ll learn about in the next lesson. On the other hand, an integer literal can include the -
(minus) sign.
01:01 This creates a negative integer number. Additionally, you can delimit groups of multiple digits by placing a single underscore character anywhere in your literal to make a big number easier to read.
01:13
It makes no difference to Python whether you use the underscores or not, but writing a number with them is arguably more readable for humans. So far, you’ve only seen decimal literals consisting of the familiar ten digits, 0
through 9
. However, occasionally you might want to express a number using a different numeral system.
01:33
Because computers use binary and sometimes a few other numeral systems, Python lets you create integers using those alternatives. By prefixing your integer literal with one of the few supported system bases, you can change how many digits you want to use. For example, the number 42 can be expressed as 101010
in the binary system.
01:57
To tell Python to interpret such a literal as binary digits, or bits, you can use the 0b
prefix. Notice how IDLE presents the number to you in the decimal system again.
02:10
It’s the same number, only represented in two different ways. Similarly, you can express the number 42 in the hexadecimal system using the 0x
prefix followed by digits 2a
.
02:24
You have up to sixteen digits at your disposal in the hexadecimal system, the usual ten digits from 0
to 9
, plus six Latin letters, a
to f
.
02:34 The letters can be either uppercase or lowercase.
02:38
The last numeral system supported by Python literals is the octal one, which has eight digits, from 0
to 7
. You can enable an octal literal with a 0o
prefix.
02:51 Again, you get a decimal representation of the created inger.
02:55 It’s fair to say that you will rarely need to use integer literals other than the default decimal one. Nevertheless, they can sometimes be useful. Using integer literals is best when you know your numbers up front and want to embed them in your code in a literal form. However, numbers often come as strings from the users who type them on their keyboard.
03:16
From an earlier video course, you might remember that you can convert a string to an integer by calling the built-in int()
function in Python. Notice the quotes around "42"
, which define a string literal.
03:30
What you get back is the corresponding in integer value, denoted without the quotes. Again, you can verify the type of the return value, which happens to be int
. By default, the int()
function assumes that you will supply a string consisting of decimal digits 0
to 9
.
03:47
If you’d like to choose a different base for the numeral system, then you can optionally pass a second argument to the function after a comma. In this case, you are creating an integer number, 42, from a string of binary digits, "101010"
.
04:04 The maximum value for the base supported by Python is 36.
04:09
It’s worth nothing that int()
accepts a value of any data type, not just strings. For example, you can pass a floating-point number in order to truncate its fractional part.
04:20
As you can see, the int()
function allows for converting values from other data types to integers, which you might need to perform some calculations on numbers rather than strings. When you call int()
without passing any value as an argument, it will always return 0
as the result.
04:37 The third way to create integers in Python is through expressions such as arithmetic expressions or function calls.
04:46 You’ll learn more about those in the future. In the meantime, integer literals should be completely sufficient.
04:55 All right. Now you know how to create whole numbers in Python using integer literals, which you can represent with different numeral systems, including the decimal, binary, hexadecimal, and octal systems.
05:08
In this case, they’re all different representations of the same value. You also know about the int()
function, which return 0
when you call it without any arguments. Otherwise, it will convert whatever data type you supply, such as a string literal, to the corresponding integer number.
05:26
When you call the int()
function with a floating-point number as an argument, it will truncate its fractional part and return only the whole part as an integer.
05:35
Finally, the int()
function can optionally take another argument, which is the base of the system used to interpret a string of digits, such as "101010"
, in the given base.
05:50 Next up, you’ll explore floating-point numbers, the second most important kind of numbers and python.
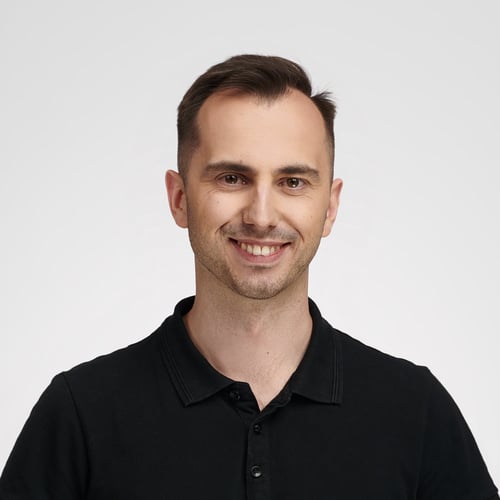
Bartosz Zaczyński RP Team on Oct. 17, 2023
@s150042028 The video courses belonging to the Python Basics learning path didn’t originally come with exercise solutions. It’s only recently that we’ve started recording them. The one for this course is currently on its way and will be published soon. Until then, you can refer to the course materials or check the solutions on our GitHub repository.
If you have specific questions, then you’re more than welcome to ask them on Real Python’s Slack community or during Office Hours, which is a live Q&A session hosted weekly.
s150042028 on Oct. 17, 2023
I don’t need exercise solutions. I will answer the exercises myself, such as the ones in the String module. Please refer to the String module by the end of the lesson. There are a lot of exercises set there.
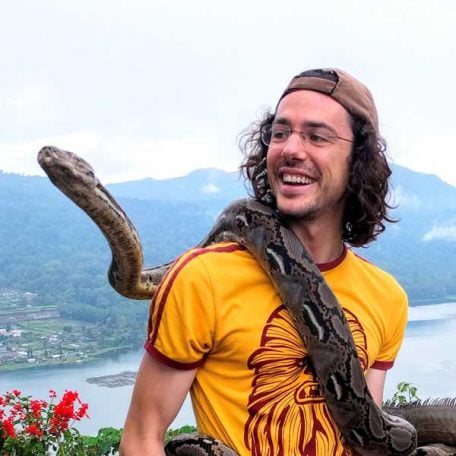
Martin Breuss RP Team on Oct. 18, 2023
@s150042028 here you go, some exercises for you to preview and work through. You’ll see them introduced and solved in the upcoming exercises course as well:
Creating Integers and Floating-Point Numbers
-
Write a program that creates two variables,
num1
andnum2
. Bothnum1
andnum2
should be assigned the integer literal25000000
, one written with underscores and one without. Printnum1
andnum2
on two separate lines. -
Write a program that assigns the floating-point literal
175000.0
to the variablenum
using E notation and then printsnum
in the interactive window. -
In IDLE’s interactive window, try to find the smallest exponent
N
for which2e<N>
, where<N>
is replaced with your number, returnsinf
.
Formatting Numbers as Strings
-
Print the result of the calculation
3 ** .125
as a fixed-point number with three decimal places. -
Print the number
150000
as currency with the thousands grouped with commas. Currency should be displayed with two decimal places. -
Print the result of
2 / 10
as a percentage with no decimal places. The output should look like20%
.
Getting Numbers From the User
Write a program called exponent.py
that receives two numbers from the user and displays the first number raised to the power of the second number.
Here’s sample run of what the program should look like, including example input from the user:
Enter a base: 1.2
Enter an exponent: 3
1.2 to the power of 3 = 1.7279999999999998
Keep the following in mind:
- Before you can do anything with the user’s input, you’ll have to assign both calls to
input()
to new variables. input()
returns a string, so you’ll need to convert the user’s input into numbers to do arithmetic.- You can use an f-string to print the result.
- You can assume that the user will enter actual numbers as input.
Using Math Functions and Number Methods
- Write a program that asks the user to input a number and then displays that number rounded to two decimal places. When run, your program should look like this:
Enter a number: 5.432
5.432 rounded to 2 decimal places is 5.43
- Write a program that asks the user to input a number and then displays the absolute value of that number. When run, your program should look like this:
Enter a number: -10
The absolute value of -10 is 10.0
- Write a program that asks the user to input two numbers by using
input()
twice, then displays whether the difference between those two numbers is an integer.
When run, your program should look like this:
Enter a number: 1.5
Enter another number: .5
The difference between 1.5 and .5 is an integer? True!
If the user inputs two numbers whose difference is not integral, then the output should look like this:
Enter a number: 1.5
Enter another number: 1.0
The difference between 1.5 and 1.0 is an integer? False!
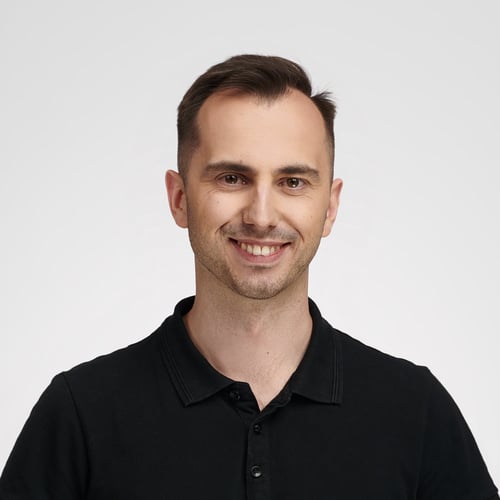
Bartosz Zaczyński RP Team on Nov. 7, 2023
@s150042028 Here’s the promised video course with the exercises: realpython.com/courses/numbers-and-math-exercises/
Janos Antal on Dec. 19, 2023
I second this, this was the most boring course ever. This course makes absolutely no sense without real life examples, use cases and exercises.
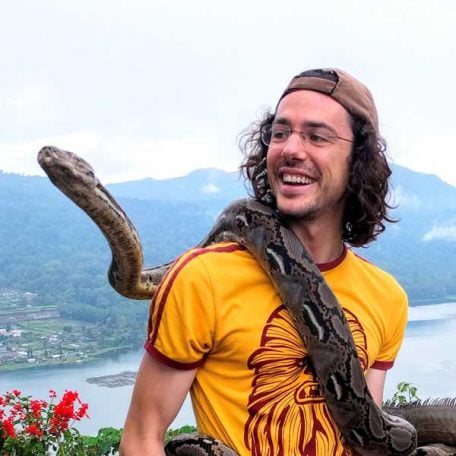
Martin Breuss RP Team on Dec. 20, 2023
@Janos Anta sorry to hear that you didn’t like the course. Did you get a chance to try the exercises in the dedicated exercises course?
For what it’s worth, I think that Bartosz did a great job at explaining numbers and how computers handle them in this course—but if I understand you correctly, you felt that it was too abstract?
Can you think of a good real life use case for this topic that you would have liked to see?
Become a Member to join the conversation.
s150042028 on Oct. 16, 2023
Where is the exercise like the previous chapter? Just watching the video is not a useful way to practice. Very bad!