Math Functions and Number Methods
00:00 In this lesson, you’ll learn about the most common functions that let you work with numbers in Python. You’ll also discover some useful behaviors exhibited by integers and floating-point numbers. Without further ado, let’s dive right into it.
00:15
The first function that you may come across when crunching numbers in Python is the round()
function. By default, it takes a floating-point number and returns the nearest integer.
00:26
For example, passing 2.3
as an argument will round the number down and return 2
. On the other hand, a number like 2.7
will get rounded up.
00:36
Things get more unexpected when your number ends in digit 5
. This is known as a tie because the distance to the nearest whole number is exactly the same whether you round up or down.
00:47
Python follows a strategy in which ties like this are resolved by rounding to the nearest even number. In this case, that number is 2
. However, in the case of 3.5
, the closest even number is 4
.
01:02 This is a choice by design to minimize the impact that rounding has on operations involving lots of floating-point numbers.
01:10
Another interesting fact about the round()
function in Python is that you can optionally pass a second argument to it, specifying how many decimal digits you want to round to. For instance, if you have an approximation of pi, then you can round it to say, three decimal places.
01:27
Notice that you’re getting a floating-point number as a result this time. Because 5
was the last digit in this example, it means there’s a tie. Instead of rounding to the nearest even integer, however, Python will look at the last but one digit to decide about the rounding direction. In this case, 2
is the closest even number. However, this doesn’t always work right.
01:50
When you try rounding a number like 2.675
to two decimal places, you’d expect to get 2.68
as the result because 8
is the closest even number. But when you actually run this code, you’ll notice that Python rounds down instead of up.
02:07
It’s not a bug in the round()
function itself, but rather the result of that floating-point representation error you learned in the previous lesson. Okay.
02:18
The next function related to numbers in Python that you may want to use is called abs()
, which is short for the absolute value. It takes a number and returns its size without the sign. For example, the absolute value of 42
is 42
.
02:33
At the same time, the absolute value of -42
is also 42
. You can call the abs()
function on integers as well as floating-point numbers.
02:43
Either way, you’ll always get the positive value of a number. However, when you call the abs()
function on a complex number, then you’ll get the so-called magnitude of a complex number, which is calculated using the corresponding formula.
02:57
You’ll take a closer at complex numbers in one of the upcoming lessons. These are the most important use cases for the abs()
function in Python.
03:08
Finally, the third and last function that you’ll learn about in this lesson is the pow()
function. It provides a more explicit way of raising a number to the given power when compared to the **
(exponentiation) operator you learned about before.
03:22
The pow()
function takes two arguments. The first one is the base, or the number to be raised to a power, and the second argument is the exponent, or the power to which the number is to be raised. As with the **
operator, you can use negative or fractional exponents and bases.
03:42
While both methods of calculating the power of a number in Python work mostly the same, the pow()
function accepts an optional third argument as long as all three arguments are in integers.
03:54
It’s a shorthand notation for the %
operator on the result of a power, such as this.
04:00 You can write this more compactly, passing the third value as an optional argument. Before closing this lesson, it’s worthwhile to mention that numbers in Python expose a few useful methods, just like strings do, which you might remember from an earlier course.
04:17
For example, you can check if a floating-point number contains an integer value with no fractional part by calling its .is_integer()
method.
04:26
Notice however that in order to call a method in Python, you need to use the .
(dot) operator, which also happens to be the decimal-point and floating-point literals.
04:35 To avoid this ambiguity, you can wrap your numeric literal in parentheses, but this method is only available for the floating-point data type. When you try to call it on an integer value, then you’ll get an error.
04:49
Remember that a numeric literal without a decimal point represents an integer. Alternatively, if you want to be more explicit, then you can assign your number to a variable and call the method on the variable rather than the literal. In this case, 3.14
is not an integer, but 3.0
is.
05:10
Another useful method available on floats is .as_integer_ratio()
, which returns a pair of integers whose ratio is exactly equal to the original float. In this case, the numbers are rather big, but you can try something more like three-quarters to get a ratio comprised of smaller numbers.
05:31
Integers also have a couple of helpful methods. One of them can tell you how many bits you need to represent a number in the binary system. For example, a bit string consisting of binary digits 101010
represents the decimal number 42
, so you need at least six bits to faithfully express the same number in the binary system.
05:53
You can confirm this by calling the .bit_length()
method on the number 42
. There’s also a similarly named .bit_count()
method, which will tell you how many of these bits are actually turned on.
06:05
The original bit string has three ones and three zeros, so the return value is 3
.
06:13
To recap what you’ve just learned, Python comes with a few built-in math functions for working with numbers. It has the round()
function, which rounds a floating-point number to either the nearest whole integer or up to the given decimal places, which you can specify as the second argument of the function.
06:30 Ties are resolved by rounding to the nearest even number, but the floating-point representation errors sometimes interferes with the expected rounding direction.
06:39
The abs()
function returns an absolute value of a number or the magnitude of a complex number. The pow()
function performs largely the same mathematical operation as the **
operator in Python, but it also provides a shorthand notation for calculating the modulo of the resulting power.
06:58
You also learned that floating-point numbers in Python expose a few methods, such as the .is_integer()
method, which checks whether the value is a whole number or not.
07:07
The .as_integer_ratio()
, on the other hand, returns a pair of integers whose ratio is equal to the floating-point number. Integers also have their methods. For example, they can tell you how many bits you need to represent them in the binary system or how many of those bits are lit up.
07:27 Next up, you are going to learn how to format numbers as strings when you want to display them along with other text to user.
robertprzydatekcl on Aug. 1, 2023
You can find some description here: docs.python.org/3/library/functions.html#round
Python uses “bankers rounding” stackoverflow.com/questions/45223778/is-bankers-rounding-really-more-numerically-stable
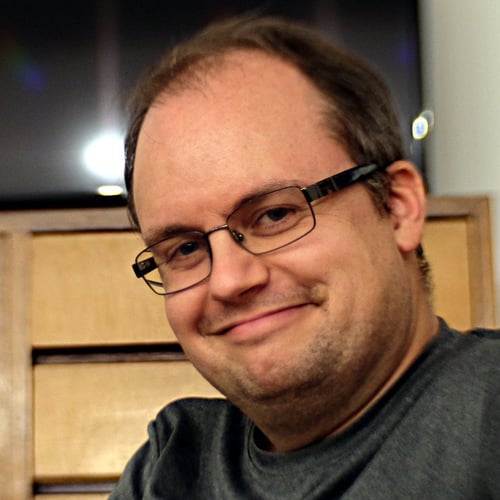
Geir Arne Hjelle RP Team on Aug. 1, 2023
There are much more detail about why rounding in Python is sometimes unintuitive in our dedicated tutorial: realpython.com/python-rounding/
Gokul Thiruvengadam Rajagopalan on Dec. 1, 2023
When I try using .bit_count()
, following is the error I am getting:
>>> (42).bit_count()
Traceback (most recent call last):
File "/usr/lib/python3.9/idlelib/run.py", line 559, in runcode
exec(code, self.locals)
File "<pyshell#39>", line 1, in <module>
AttributeError: 'int' object has no attribute 'bit_count'
Python version details:
>>> import sys
>>> print(sys.version)
3.9.5 (default, Nov 23 2021, 15:27:38)
[GCC 9.3.0]
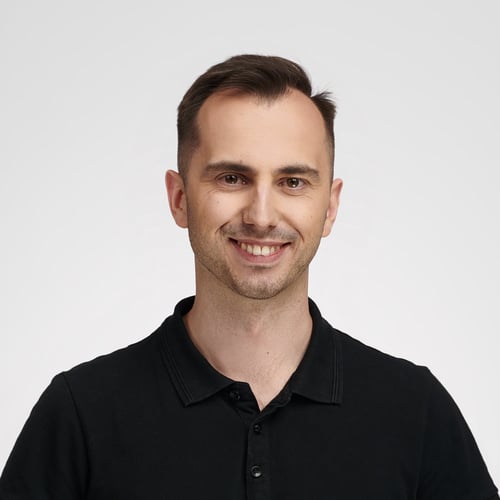
Bartosz Zaczyński RP Team on Dec. 1, 2023
@Gokul Thiruvengadam Rajagopalan The .bit_count()
method is available since Python 3.10, as noted in the Python documentation I linked.
Become a Member to join the conversation.
Jesús Pineda on July 31, 2023
Why does the error occur when using the round() function? It’s supposed to round up to the nearest number, but it doesn’t always work like this, for example: round(1.5) 2 round(2.5) 2 round(3.5) 4 round(4.5) 4 round(5.5) 6 round(6.5) 6 round(7.5) 8 round(8.5) 8 Is this a python bug? or does it only happen when we use this editor?