Create the Django Data Model
In this video you will be creating your data model in Django . Let’s go over what that means before we get started. I’ll provide you with a very simple explanation.
Let’s say you need to create a scheduling app for a vet office. One of the tables in your relational database is Pet. Pet has the following fields, age, gender, type, weight and owner. Your app needs to create, read, update and delete data from the Pet table. Create, read, update and delete are known as the CRUD functions.
The purpose of a database is very similar to the purpose of the object oriented programming paradigm, in that we are digitally modeling objects and ideas we find in the physical world. While this translates to tables and records in relational databases,.. in object oriented programming languages this translates to objects, typically class definitions, as is the case with Python.
Therefore when we model our data, we are basically creating a class to match our database table structure. We make a class name to match the table’s name and then make the class attributes, or member variables, match the table’s field names and data types.
The combination of field names and their associated data types, such as integer for age, string for gender and type, float for weight, and string for owner, is commonly referred to as the schema.
Once we model our database table as a class, an object instance corresponds to a current record in play from the database table. One of the benefit of programming objects is the ability and ease of defining methods to go along with our data model, such as a way to “schedule” a Pet. In that way we’ve provided nouns, adjectives, and verbs for our Pet, where the database table isn’t usually as versatile.
If you recall what I said earlier about mapping your class attributes to the schema which includes data types, you may have wondered how that’s possible given that Python is dynamically typed.
Django provides the solution to this through Object-Relational Mapping, ORM for short. In other words there are classes in the Django framework that can be used as Integer Fields, Character Fields and others that we can use as data types for our class attributes. This facilitates more friendly transitions between our programming instances and their corresponding records.
This data model is what you’re going to build for your Postgres database.
00:00 Welcome to the seventh video in the Real Python series making a location-based web app with Django and GeoDjango. In this video, you’ll be creating your data model in Django. Let’s go over what that means before we get started.
00:14
I’ll provide you with a very simple explanation. Let’s say you need to create a scheduling app for a veterinarian’s office. One of the tables in your relational database is pet
. pet
has the following fields: age
, gender
, type
, weight
, and owner
. Your app needs to create, read, update, and delete data from the pet
table. Create, read, update, and delete are commonly known as the CRUD functions.
00:41 The purpose of a database is very similar to the purpose of the object-oriented programming paradigm, in that we are digitally modeling objects and ideas we find in the physical world. While this translates to tables and records in relational databases, in object-oriented programming languages this translates to objects—typically class definitions, as is the case with Python.
01:04 Therefore, when we model our data, we are basically creating a class to match our database table structure. We make the class name to match the table’s name, and then make the class attributes or member variables match the table’s field names and data types.
01:19
The combination of field names and their associated data types—such as integer for age
, string for gender
and type
, float for weight
, string for owner
—is commonly referred to as the schema.
01:32 Once we model our database table as a class, an object instance corresponds to a current record in play from the database table. One of the benefits of programming objects is the ability and ease of defining methods to go along with our data model, such as a way to schedule a pet. In that way, we’ve provided nouns, adjectives, and verbs for our pet, where the database table isn’t usually as versatile.
01:57 If you recall what I said earlier about mapping your class attributes to the schema—which includes data types—you may have wondered how that’s possible, given that Python is dynamically typed.
02:10 Django provides a solution to this through object relational mapping, or ORM for short. In other words, there are classes in the Django framework that can be used as integer fields, character fields, and many others that we can use as data types for our class attributes.
02:26 This facilitates more friendly transitions between the data in our programming instances and their corresponding records in the database.
02:35
This data model is what you’re going to build now for your Postgres database table. “But wait!” you say, “We don’t have a table yet in our Postgres database.” Let’s take a look. In pgAdmin, expand the shops
database, then Schemas > public > Tables, and you’ll see only one spatial reference table there, added previously by the PostGIS extension. You don’t want to touch that table. Instead, you want a table for storing our shop locations.
03:02
You’ll create a table named shop
with fields for name
, location
, address
, and city
. Instead of doing this in pgAdmin, however, we’re going to take advantage of Django migrations.
03:13 Django migrations allow us to manipulate our table structure and data from just one source: Django. Migrations serve as a sort of version control for our table changes and keep everything in sync.
03:24
You’re going to start this process off by creating your Shop
data model. Open the models.py
file within your nearbyshops
app.
03:32
Because you’re going to use a special field data type called PointField
, you will need to import models
from the GeoDjango framework, so adjust the first line accordingly.
03:46 Next, add the following code to create the model beneath the comment.
03:53
Notice we get our Django field classes from the models
module, and that location
is of type PointField
, while the others are CharField
.
04:02
Good job. Your data model for your shop
table structure is complete! In order to perform the migration, we need to execute two manage.py
commands: makemigrations
and migrate
. Let’s do that now.
04:28
We appear to have a successful migration. Let’s use pgAdmin to see what happened in our shops
database. In pgAdmin, expand the shops
database, then Schemas > public > Tables.
04:42 You may need to do a refresh in order to see the changes.
04:48
Wow, your first migration added quite a few tables. Many of these are administrative tables Django added, but if you look towards the bottom of the list, you’ll see a reference to our nearbyshops_shop
table.
05:01 If you expand it, you’ll see the field column names.
05:08 In the next video, we’ll switch gears briefly to set up our Django administration with a superuser account. See you there.
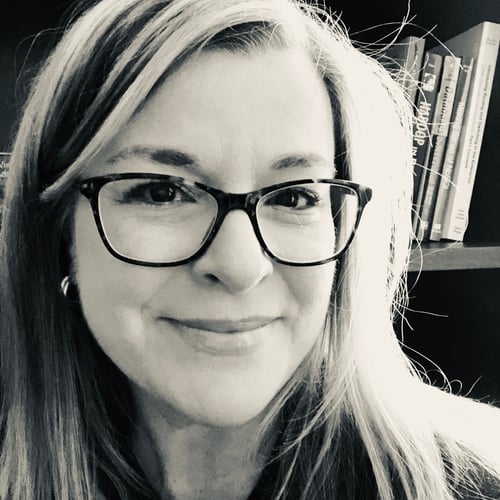
Jackie Wilson RP Team on May 9, 2019
Did you have any problems installing QGIS? Also, if you’re on Windows you’ll likely have to reboot after the QGIS install.
bultita on May 9, 2019
Thanks Jackie for the swift response. I have no problem installing QGIS, followed exactly as described in the video and I did reboot after installation. I even repeated video 1 to 6 to see if I missed any thing in the process. Thanks.
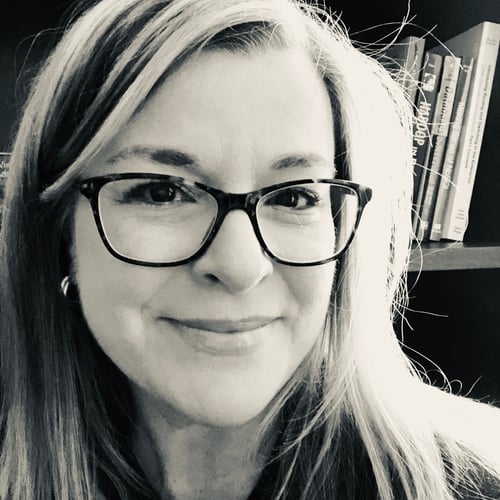
Jackie Wilson RP Team on May 10, 2019
Are you installing this on Windows? Sorry for all the questions - it helps me pinpoint what’s going on. I mean, it appears GeoDjango can’t find GDAL driver in your path, but it’s good to eliminate some other possibilities. If you launch QGIS, does it work? Or do you get a Python 3.6 error.
Kevin M on May 13, 2019
I’ve got the same bug, and I had to add the path into the settings: GDAL_LIBRARY_PATH = r’C:\OSGeo4W\bin\gdal204.dll’ After too long trying to figuring out what’s wrong in windows
iamfarzaad on July 6, 2019
This post Helps for all who is trying in windows.
brandsinbloom on Oct. 7, 2019
Thanks iamfarzaad. That helped me get this working in Windows for me :)
GeoMeteoMe on March 19, 2020
@iamfarzaad thanks for the link - it helped to solve a bunch of errors, however I am still not able to import data from the jason file to database even though migration is completed without anny error. The database is empty.
Dr Andrea Kocsis on Feb. 28, 2023
It didn’t let me execute migration involving creating the postgis extension unless already being a superuser. So following suggestions here (stackoverflow.com/questions/16527806/cannot-create-extension-without-superuser-role/51395480#51395480?newreg=d82ed2d6afb54ed1bce3d3e9a06605df), I first created the extension in terminal, and then it worked.
Thierry Gagné on Oct. 2, 2024
Hi everyone, in case someone else has this problem: I could not run “python manage.py migrate” in the current setup. I had to modify the pgAdmin4 query editor:
CREATE USER shopadmin WITH PASSWORD ‘shoppass’; GRANT ALL PRIVILEGES ON DATABASE shops to shopadmin; GRANT CREATE ON SCHEMA public TO shopadmin;
The last bit made the migration work.
Become a Member to join the conversation.
bultita on May 9, 2019
Hi, There is error when I run the server - python manage.py runserver : “django.core.exceptions.ImproperlyConfigured: Could not find the GDAL library (tried “gdal203”, “gdal202”, “gdal201”, “gdal20”, “gdal111”). Is GDAL installed? If it is, try setting GDAL_LIBRARY_PATH in your settings.”
Appreciate your help.
Thanks, Assaye