Create and Inspect a Variable
For more information on concepts covered in this lesson, you can check out:
00:00 Now that you’ve seen variables briefly in the last lesson, it’s time to dive in much deeper into talking about them.
00:08 In Python, variables are names that can be assigned a value and then used to refer to them later on throughout your code. And that’s the really crucial idea of a variable is that you can assign it and give it a value, but then if you want to reference that value anytime later on in the program, you can call up the name of the variable and use it again, or potentially give it a different value.
00:35 Variables are really fundamental to programming, and there’s two major reasons. One, it keeps the values that you’re trying to get to inside your program accessible. For example, you can assign the result of some time-consuming operation or expression to a variable so that your program doesn’t have to perform the operation each time you need to use the result.
01:00 It can just access that value right out of the variable.
01:04
The other reason is variables can give you context. When you have the number twenty-eight, it could mean several different things. It could be the number of students at class, it could be the amount of times someone has accessed a website or gone somewhere, and so on. Giving the value twenty-eight a name like number_students
makes the meaning of that value clear. In this lesson, you’re going to learn how to use variables in your code and some of the rules and style guidelines behind that. To get there though, to create a variable, you have to learn about something called the assignment operator.
01:43
So let’s start with the second word, operator. An operator is a symbol, such as +
(plus) or -
(minus), that performs an operation on one or more values. You used one earlier of like 2 + 2
.
01:58
And it performs addition in that case. If it was 2 - 2
, it would perform subtraction.
02:04
In the case of assignment, values are assigned to variable names using a special symbol called the assignment operator, which is a single equal sign (=
). And this can be a little confusing.
02:17 You’ll get to learn more about how this assignment operator works and how it’s not necessarily comparing two things and saying they’re equal to each other.
02:26 It’s actually giving the value and assigning it to that variable’s name. The operator takes the value that’s on the right side of the operator, and it assigns it to the name on the left. Let me have you look at that in the interactive window.
02:43
Type this. You’re going to make a variable. Its name is greeting
. To assign it a value, you use the equal sign, the assignment operator. You’re going to give it the string literal of "Hello, World"
.
03:01
If you were to type print()
and then, as the value, choose to use the variable name greeting
, you would get the same output as if you were to have it print the literal itself: Hello, World
. If you were to type greeting
by itself,
03:22
you would see what it’s assigned to: the string literal "Hello, World"
. What if you were to make a typo when you went to print and you chose capital Greeting
? This is valid code again.
03:36 It shows that as far as the syntax is concerned, this works, but when I press Return,
03:43
you can see that I got a RuntimeError
of a NameError
. The name Greeting
is not defined. And then again, this is an advantage of Python 3.10, where it’s actually giving you a little better idea of the context of what’s happening, and it says, Did you mean: 'greeting'?
because there is a variable named greeting
in here, which is really nice.
04:05 This is an update. You might not see this in an earlier version of Python. So yeah, the case that you’re using in a variable’s name is important, and they are completely different.
04:18 You might have learned in other programming contexts that maybe capitalization didn’t matter, but in the case of Python, it does.
04:29
Now there are rules for valid variable names inside of Python, again to avoid things like a SyntaxError
. Variable names can contain uppercase and lowercase letters.
04:41
Any of those are fine. It can include digits. It can include underscores (_
). And the one trick there though, is it can’t begin with a number, can’t begin with a digit.
04:55
So let me give you a couple examples. So a valid Python name could be string1
or "_a1p4a"
, or list_of_names
. Some things that would not be valid would be starting with a number, so 9_lives
or 99_balloons
or 2beOrNot2Be
. So just keep that in mind: you can’t start with a number, can’t start with a digit, okay?
05:22 In addition to the alphabetic characters that we just talked about and the digits and the underscore, Python variable names can contain Unicode characters. Unicode, if you’re not familiar with it, it’s a standard for digitally representing characters.
05:38 And they’re used in most of the world’s writing systems. So let me give you a couple of examples of things you might’ve seen. So decorated letters in French or Spanish or German, where you have like e with an accent on it (é), or u with an umlaut (ü), you know, different things like that, that are decorated—you can use those characters and Chinese, Japanese, or Arabic symbols, but not every system that you run your program in can display these characters.
06:06 So it’s something to be careful with and it may work better to use the ones that we specified a little earlier. You’re going learn a lot more about Unicode in a much later course, but I’ll also include some resources if you want to jump ahead.
06:19 There’s a few resources on Real Python about Unicode that you can dive into much deeper. Choosing a good name for a variable is difficult. There’s a few memes out there that show someone thinking really hard of, “How do I come up with a name?” And there are some guidelines that you may want to follow.
06:38
The first one that I want to share with you is keeping things descriptive can be much better than keeping it super short. A variable named s
is kind of difficult to know your intention of what that variable is, especially if it was something like seconds. That might be much more useful, or even more explicit and descriptive would be seconds_per_hour
. That may seem to you like a lot to type.
07:08 What you’ll learn is that there are lots of tools when you’re working with an editor that can help you complete the names of things so you don’t have to worry so much about keeping things super tight and short.
07:20 It can be much more useful for you going forward to have a descriptive name than just a simple, short name. And a good rule of thumb is to maybe limit the variable name to three or four words at maximum. All right, so I said that you could use lowercase or uppercase letters. Well, where should you use them?
07:41 The case that you should use can vary depending on what you’re wanting to do, but there are some guidelines. Let’s talk about different ways this can be done.
07:50 One term that’s used is mixedCase, where you start with, say, lowercase letters, and then every individual word that you’re adding inside of that variable name, you make it uppercase for that first letter of it. So here’s some examples.
08:05
So like number_of_students
could be numbStudents
or listsOfNames
and so forth. So that’s called mixedCase. The other way to do it is to use underscores, keeping everything lowercase, and then using an underscore in between each one of these.
08:22 This is also referred to as snake_case. That’s what those variables would look like in snake_case, or lowercase with underscores. In Python, it is more common to write variable names in that style.
08:35 It’s not prohibited to use mixedCase, but it typically is more in the style of Python generally to do that style. In fact, there’s a whole topic about this, and it’s referred to as PEP 8.
08:51 PEP 8 is widely regarded as the official style guide for writing Python: how things are named, how things should be formatted, how things should look. And you can learn a lot more about it by looking at this website, pep8.org.
09:05 Someone has created this site to kind of give you a tour of all the features in a nice, formatted way. There’s more information. I’ll include a link in the description. You can learn more about it, too, on Real Python.
09:17 Following the standards outlined and PEP 8 will ensure that your Python code is readable code to most Python programmers. It’ll look in the style of other Python users.
09:28 As far as the name PEP, it stands for Python Enhancement Protocol. A PEP is a design document. It’s used by the Python community to propose new features or changes to the language. When working with variables, it’s nice to be able to inspect the values, and you can do that inside the interactive window. To inspect the value that a variable holds right now, you can type the name of the variable by itself at the prompt.
09:55
Just typing the name by itself at the prompt will often display something different than what is displayed compared to using print()
. You can also learn about what type of variable it is by putting the name of the variable in type()
. Let me show you what those look like.
10:13
Let’s say you created a variable of x
, and x
is going to be assigned it the value of 2
. If I type x
and inspect the variable, you can see that its value is 2
.
10:27
And if I were to print x
, I get the same output. Now it might look a little differently if I were to, say, assign y
to the value of a string literal "2"
.
10:42
So this is text instead of an actual numeric value. So in that case, if I type y
, you’ll see that it outputs something slightly different versus if I were to print it.
10:56 So that can be a little confusing. And what you’ll learn is that these are different types, and they’re interpreted differently inside of Python. And this is a big subject that you’re going to dive into in much later courses.
11:08
One thing you can check is you can say type()
. You can see here that it’ll ask for the object or name. And then it’s going to return—that’s what the arrow is indicating—the object’s type. And this is just a preview. So try it out with x
.
11:25
It says that it is a class of integer. Now versus type of y
…
11:34
y
is the class of a string. And they’re being represented differently when you simply just enter in the name of the variable. And keep this in mind that variable inspection like this, it only works in this interactive window inside of a REPL. For example, try running the following program from the editor window. So here, let’s say we start by defining greeting
and say it equals "Hello, World"
.
12:05
And then on the next line, simply type greeting
.
12:09
What’s it going to output when you run this program? As you can see when it ran it, it didn’t output anything. That type of inspection of a variable only works here in the interactive window. As a review, here’s a couple exercises you can do: using the interactive window, display some text using print()
, and then, using the interactive window, assign a string literal to a variable.
12:34
Then print the contents of the variable using the print()
function. Repeat these first two exercises using the editor window now, with a file.
12:45 All right, up next: how can you leave yourself notes inside of your code?
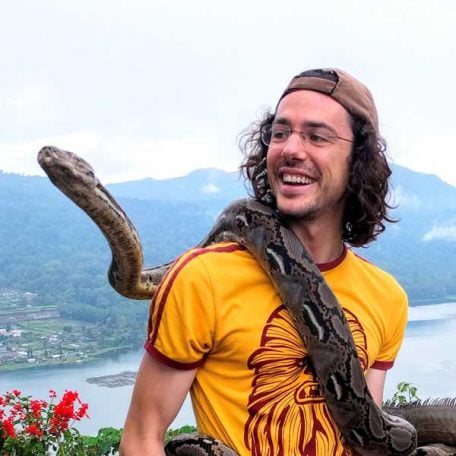
Martin Breuss RP Team on Nov. 24, 2022
@pmkdatar you can use the forward (→) and backward (←) arrow keys to scrub through the video 5 seconds at a time.
Learner on Nov. 9, 2023
Greetings!!
Can we know the use cases of type() function that we use the inspect the type of a variable?
Thanks in advance.
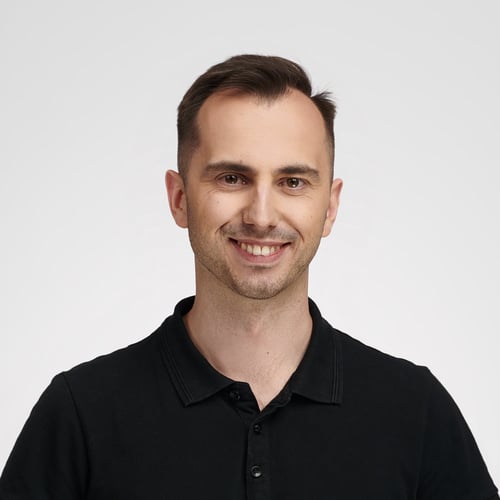
Bartosz Zaczyński RP Team on Nov. 10, 2023
It’s hard to pin specific use cases because the type()
function is one of the fundamental tools in Python. Personally, I tend to use it the most when I want to find out the type of value returned by a function from a library that I’m trying out.
Become a Member to join the conversation.
pmkdatar on Nov. 24, 2022
Excellent. But could you please add rewind 15 seconds and forward 15 seconds button to all videos of this website?