Create Your Templates: Part 1
In this lesson, you’re going to revisit templates and deal with the TemplateDoesNotExist
error you saw in the previous lesson.
You’re going to make a new html
file, all_projects.html
, in your double folder structure. When you’ve created your template and made sure Django knows where to find it, it can be rendered.
00:00
Welcome to our second take on templates! Let’s go ahead and deal with this TemplateDoesNotExist
error that we were getting before, by creating the all_projects.html
template that we told Django we would be creating. Inside of here—remember the double folder structure—I’m going to make a new HTML file,
00:26
and call it all_projects.html
. Again, PyCharm already gives me some help in here by creating this basic HTML structure.
00:37 So, what’s interesting about this? We could just—again, let’s do this quickly, take a look again.
00:48
Now, our template is there and it can get rendered, so if I head over to our app and reload this—I’m still getting our TemplateDoesNotExist
! Let’s figure out why that’s the case,
01:07
And the reason is—we have to check back to the views
file, actually, because we made a little mistake here. Remember the double folder structure, right?
01:19
What we need is to also prepend this with 'projects/'
so that Django knows how to find it. Yeah? So, it’s by default only going to look inside of the templates/
folder, so if I have this double folder structure, I need to say, “Yes, you’re inside of templates/
. Now, go inside of projects/
, and then that’s where you’re going to find this HTML file.” We’re heading back over there and reloading.
01:46 We can now see hello again! so the template is found and correctly renders the HTML that I’ve written over there. No change to what we did, so far.
01:57
Now, let’s go ahead and deal with this interesting situation: we did a query to our database in the view, and we passed that forward as the name projects
with the key 'projects'
to our template. And here, we’re going to take a little dive into the Django templating language. Templating languages, in general, are ways of putting code and variables—values of code—inside of HTML.
02:23
So, Django has its own templating language that consists mostly of two things that you’re going to have to know. One is you’re going to use this {
(squiggly bracket), %
, then your code logic, and then again, to close it off %
and }
(squiggly bracket).
02:38
You can write for
loops in there, that’s what you’re mostly going to end up doing, probably, but you can write some limited subset of Python code logic inside of this special syntax.
02:51
Then, the second one is {{ }}
(double squiggly brackets), and this is, kind of, the print()
statement of your template. So, if you have variable that you’re passing over and you want it to be shown inside of your template, then you put it inside of double squiggly brackets.
03:07 And that’s what we’re going to do. So, let’s head over to the code,
03:12
and from our views
file, we know that we have access to all of our projects through a key that’s called 'projects'
. Django makes this pretty simple.
03:25
That’s just always the way you’re going to pass those things over. Inside of here, I can render them. I can say—let’s show these first. I can say {{ projects }}
.
03:38 That’s simply the name of the key of whatever we’re passing over there.
03:46 Now, when I head back over and refresh this I can see this printed out: the same thing that we got printed out earlier when I printed it to the console.
03:54
Okay? So, we’re getting a QuerySet
that has one Project
object in there. Okay, cool. So, that’s not exactly what we want to see, right?
04:04 But it’s already getting there. We’re displaying something that we’re passing from the view. So, what we want to do next is we can actually iterate over this.
04:13
This is a QuerySet
, so let’s use that second part—the code logic—to iterate over our projects
variable that we’re passing over there.
04:23
I’m going to say {% %}
and close it right away. I’m going to say for project in projects
. It looks exactly like how you would start off a Python for
loop, only with this weird syntax around it. Okay. I don’t need commas and because we’re inside of an HTML document, we have to use it in this Django templating way of writing Python.
04:51
But inside of here, I can make some more HTML. So I can say, “Give me a <p>
—give me a <p>
! And inside of that <p>
, I want to render—of my project
—the .description
.” Okay.
05:12
And one other thing you have to do, because we’re inside of the Django templating language and not actually Python, you also have to close this for
loop with a statement called endfor
.
05:26
All right? Looks good. Let’s check this out in our browser. When I reload this, I get my project description in here. Since I’m in a for
loop, if I had multiple instances of Project
in my database, I would print out all those descriptions. Now, let’s do some more. What else do we have?
05:46
We have a .title
. So I’m going to say, “Give me a .title
.”
05:52
And we have a .technology
, right? Let’s do that—print out everything that we have.
reblark on Oct. 29, 2019
I am using Pycharm. I made a change in views.py, removing project_list and replacing it with all_projects. I cannot start the server because it keeps returning an attribute err that the module projects.views has no attribute ‘project_list’. Clearly, it still sees project_list and doesn’t recognize the change in code. How do I fix this?
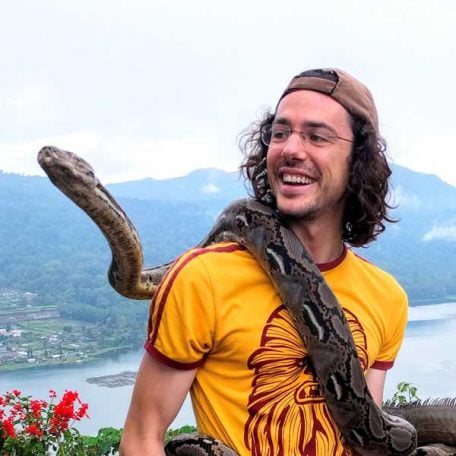
Martin Breuss RP Team on Oct. 30, 2019
You can always access your virtual environment by moving into the folder that contains your virtual environment folder, and then activating it with the following command:
source your_env_name/bin/activate
You should see the name of your venv pop up on the left side of your command prompt. That means that you’ve successfully activated it.
Changes inviews.py
It’s difficult to say what exactly is happening in your case. There could be many reasons for this and it’s difficult to diagnose without seeing your code. However, here are a couple of tips of what you can check up on:
- Double-check your
projects/views.py
file. Did you edit this file, or maybe accidentally another one? - Check in your
urls.py
file whether you are still pointing to a function calledproject_list
in your URLs - If neither of those resolves it, head over and google your error
Hope this helps resolving it
Siggi Berg on Dec. 30, 2019
Hi there. Exciting stuff Django but I have a problem with having the database object to show up in the browser. * <QuerySet [<Project: Project object (1)>]> (no datbase object) The django code in all_projects.html may be wrong but I can´t figure it out. This is how it looks like: * <body> {{ projects }}\n {% for project in projects %}\n \t<p>{{ project.description }}</p>\n {% endfor %}
grinsekatz007 on April 19, 2020
do i need to activate django templating language somewhere ? because in my case, its being ignored. Im using Pycharm
kind regards
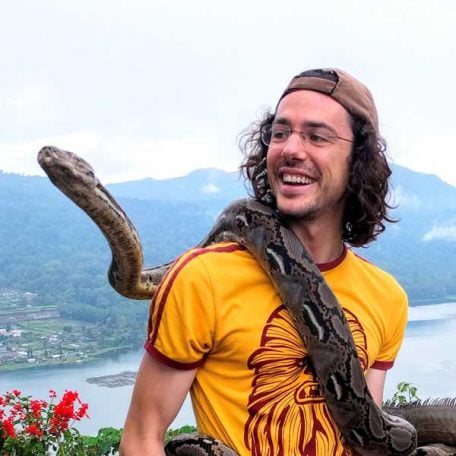
Martin Breuss RP Team on April 19, 2020
Hi @Siggi Berg, totally missed your comment here. Did you manage to figure this out? I assume your code in the template looked like this:
<body>
{{ projects }}
{% for project in projects %}
<p>{{ project.description }}</p>
{% endfor %}
</body>
The code looks fine as long as you are passing a QuerySet
object containing all your Project objects to the template:
{{ projects }}
will display a string of theQuerySet
object - this might look like the string you posted in your question:<QuerySet [<Project: Project object (1)>]>
(= aQuerySet
object containing oneProject
object). You don’t need to display this collection. Instead you do what you did below with the{% for item in collection %}
. This will iterate over all theProject
objects inside of yourQuerySet
object, and create a paragraph containing thedescription
of eachProject
So I’d need to know in more detail what is being displayed in the template when it renders, and what you are passing in. Maybe these hints can help someone else running into a similar issue.
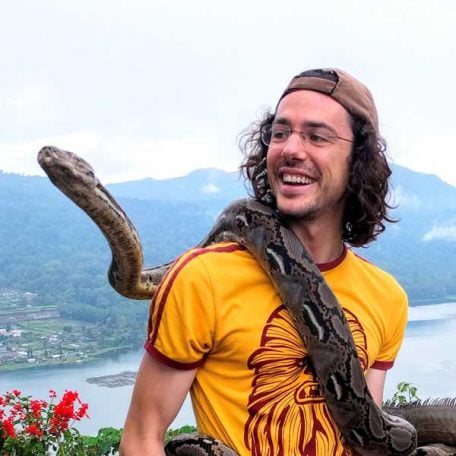
Martin Breuss RP Team on April 19, 2020
Hello @grinsekatz007. There’s no need to activate the Django templating language anywhere. If you are working with the file within a Django project context, and the file has a .html
ending, then Django templating language should work.
Can you describe in more detail what you are doing and what is/isn’t working? You could also link to a GitHub repo with your code, that makes it easier to check and refer to what’s going on.
tomislavm021 on July 20, 2020
I keep getting the error : Class ‘Project’ has no ‘objects’ member
What can i do?
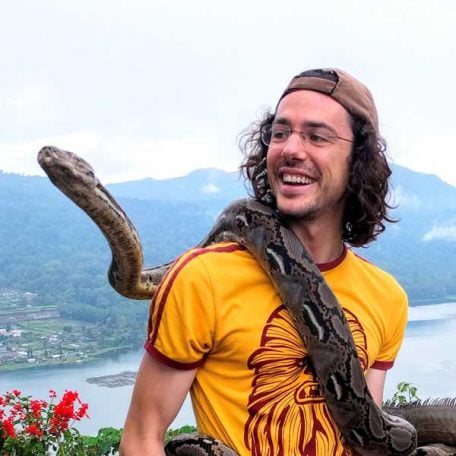
Martin Breuss RP Team on July 21, 2020
Hi @tomislavm021 - are you receiving this as an error or as a warning? If it is a warning, which means your code still works as expected, then it might be related to some linter tool that you have installed on your text editor. Check out this StackOverflow answer for more details.
If it’s actually an error, meaning that your app doesn’t work because of it, then please share a link to your code repo so I can take a look. The most likely place to check on for possible errors would be models.py
- e.g. is your model inheriting from Django’s models.Model
?
Hope this helps!
mathv87 on July 28, 2020
I had a similar problem with this task. However I found out that it is very important to not confuse the {{ }} with {% %}. It generates the error message “could not parse ..” when you would for example write something like {{% %}}.
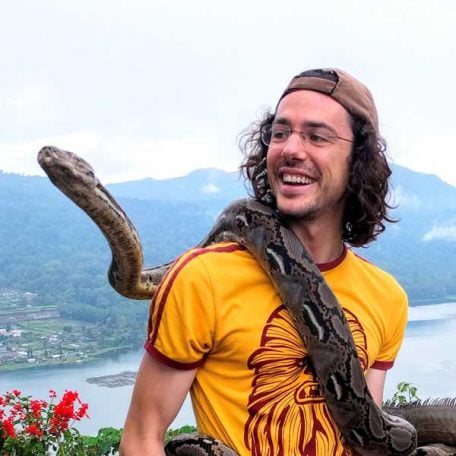
Martin Breuss RP Team on July 30, 2020
Thanks for sharing @mathv87. :) Yes, in Django’s templating language there’s a difference to keep in mind between:
- Template Variables: which are used to display the value of variables present in your code, and which use the
{{ }}
syntax, and - Template Logic: which is used to write code logic inside your template, that Django will execute. Code blocks use the
{% %}
syntax
As you mentioned, Django will tell you if something’s wrong in that department with an error message similar to “Could not parse” if you try to use syntax aside from those two. That’s good, because you get helpful feedback :)
Jesse F on July 17, 2024
Running into an issue where Quarry set is coming back empty.
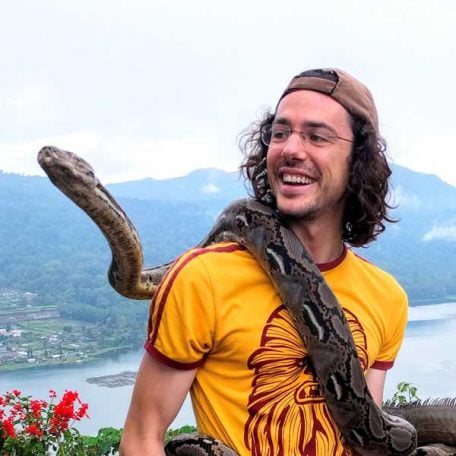
Martin Breuss RP Team on July 18, 2024
@Jesse F please share more information (e.g. which error are you getting, if any, which query set, etc.) and the relevant code, otherwise I can’t help you figure out what might be going wrong.
Jesse F on July 21, 2024
@Martin Breuss, thank you greatly for responding so quickly. I have learned so much the past few days and I’ve made plenty of new friends, too! I ran into the issue due to not properly saving the Db entry when using the Django shell. I forgot to add the () when typing p1.save. Happy to report I am moving on to the remainder of the course! :)
Become a Member to join the conversation.
reblark on Oct. 29, 2019
When I shut everything down and then return, how do I get back into the virtual environment in the directory where my app is?