Django Shell
Your next step is to put data in your database. For this, you’re going to use the Django shell to put one project in your database. Head over to your terminal and start the Django shell by typing the following:
$ python manage.py shell
This opens up a terminal, but it has the Django settings already imported, so it allows you to work directly from the root folder of a Django project.
00:00
Next, we want to put some data into our database. For this, we’re going to use the Django shell. There’s some different ways of doing that, but I want to show you this very handy tool. We’re going to get started and put one Project
into our database.
00:15
For this, I head over to my terminal, in here. I’m going to start the Django shell by typing python manage.py
—as so often—and then shell
. This opens up a terminal.
00:30
However, it has the Django settings already imported, so it allows you to work directly from the root folder of a Django project. So, if you want to do something within Django or with the database, then start your shell like this. Now, what I want to do is I want to create a Project
object and put this one into the database. For this, I’m going to use Django’s ORM. So, here is our cheatsheet.
00:57
That’s what a Project
instance is going to look like. It’s going to have a title
, description
, technology
, and image
—an image path.
01:05
We put the images already in here. So, let’s go ahead and just create one. What we need to be able to use, then, is we have to import this Project
model.
01:16
We can find this inside of—let me close this for a moment—projects/
and then models.py
. Okay, so this is where it sits. I’m going to say from projects.models import Project
, and now I can create a Project
.
01:37
So, my first project is going to be a Project
instance that has a title
.
01:45
It’s going to be "test project"
.
01:50
It’s going to have a description
…
02:03
Yada, yada, I’m just going to fill this out. Make sure that you put in the right data types. Like, it’s clear that we’re going to use text for a CharField
(character field), TextField
, and you can’t go over the max_length
or it’s going to complain, et cetera.
02:28
And for image
, I’m just going to put in
02:32
the name of the image file as the rest of the path—you remember Django knows about static/
folders in all apps, and we told it about projects/
and img/
, so we directed it from here to img/
.
02:45
All I need to put in here is, really, the name of the image file. So, I’m going to say "testproject.png"
. Okay. This is my first Project
instance. Let’s create it.
03:02
Cool. So, all done? Not quite. What we need to do first—similar to the migrations—there’s always a step in between before you actually commit something to the database. So what I need to do to put it into the database is I have to say p1.save()
.
03:22
And now, I have this one instance as a row sitting inside of my database. We can inspect this with this visual tool that I showed you before, go ahead and do that. I’m going to show you a different way of doing it, which is I can query here. So, I can say results = Project.objects.all()
.
03:43
With this, I’m retrieving all the Project
objects from the database, and now I can look at this. It’s telling us it’s a QuerySet
instance, as a result, and it contains one Project
object.
03:58
Awesome! I can say, “Give me that first one.” I can say results[0]
is going to be my p1
—let’s call it different, just to—p
, for this one.
04:13
I can say p.title
, for example, and this is 'test project'
. It’s what I just put in there—so, what I did. And that’s where we can see the power of using an object-relational mapper. Using Python, I created an object, then I simply said .save()
to put it into my relational database, and here I’m—again—using the ORM to query the database, get some results back, and then you can see, I can access them again very similar—or, in the same way that I would access a Python object.
04:47
So that’s the ORM at work, and yeah, we put a Project
inside of our database. So, let’s make sure to set up the rest that we need. We still need routes, we need views, and we need templates. Let’s make sure to set those up so that we can actually see this Project
, also on the front end.
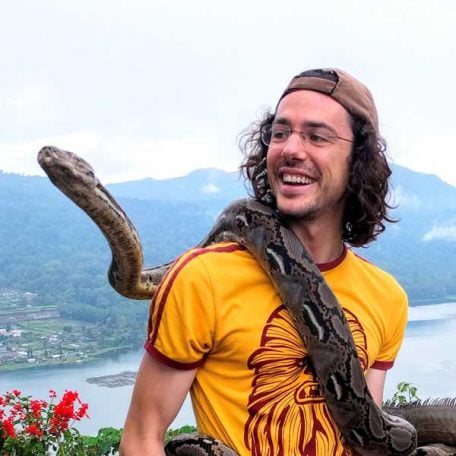
Martin Breuss RP Team on Oct. 16, 2019
Good question! As a beginner project, this assumes that you’re building a small example project and that you’ll fill up your DB as you build out your portfolio.
However, Django does have capabilities to integrate with an existing database, and since it’s all Python code, you could also write a custom script that fetches the data from your source and funnels it into your project’s DB.
reblark on Oct. 24, 2019
Wait. You copied image files from your own server, which, of course, I can’t do. How can I get copies of those? It is really important to me that I can replicate what your are demonstrating.
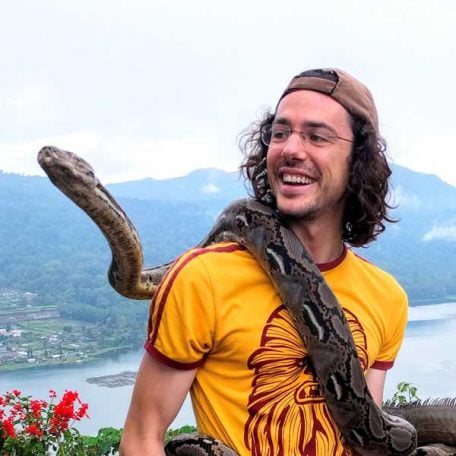
Martin Breuss RP Team on Oct. 24, 2019
@reblark: I took some screenshots right on my computer and moved those files into the Django folder structure that you see above. The path that you see points to the relative path in the Django project. This could be any image file that you have locally, you just need to make the names match.
reblark on Nov. 4, 2019
OK. I don’t see the screenshots or the structure and I apologize for not being able to keep my comments in synch but it is difficult only being able to communicate through a specific session. It’s 11/4 at 11:00 AM PST, and I just wanted you to know that I worked through the PyCharm database problem. On the one hand, it is very frustrating. On the other hand, figuring things out is very rewarding. It’s all effing crazy.
Gaurav yadav on Dec. 7, 2019
Are there any ways by which we can enter data through frontend interface?
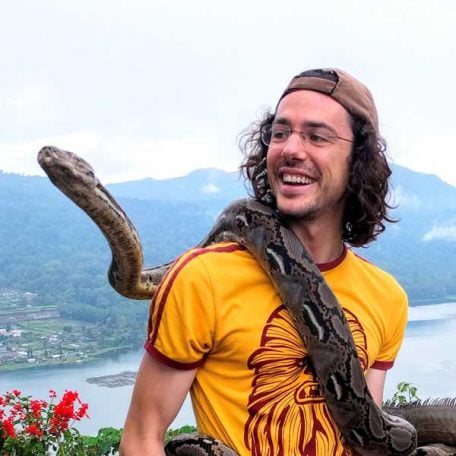
Martin Breuss RP Team on Dec. 7, 2019
Yes, you can use the Admin Interface that comes provided with a Django app.
Otherwise, you’ll have to built your own Django Forms to collect user input.
Siggi Berg on Dec. 29, 2019
The database doesn’t work in my project. This is how the Django shell looks:
from projects.models import Project p1 = Project(title=”test project”,description = “This is a test, 1234”,technologi=”Django”, image=”F-01.jpg”) p1.save <bound method Model.save of <Project: Project object (None)>>
Her somtething seems to have gone wrong. Anyway I continued. But nothing seems to have been inserted into the database: *>>> results = Project.objects.all()
results <QuerySet []>
*
Siggi Berg on Dec. 29, 2019
I’m sorry! I found that I had spaces in the “project.models” command. Also I forgot the () after the p1.save. Now everything is working fine.
koutsellisthemistoklis on March 1, 2020
1) When you write p1 = Projects(title=’test project’, ....)
where is the model.Models argument of Projects class?
Also, another question.
2) In Projects class declaration, variables: title, escription, technology, image shouldn’t be self.title, self.escription, self.technology, self.image instead?
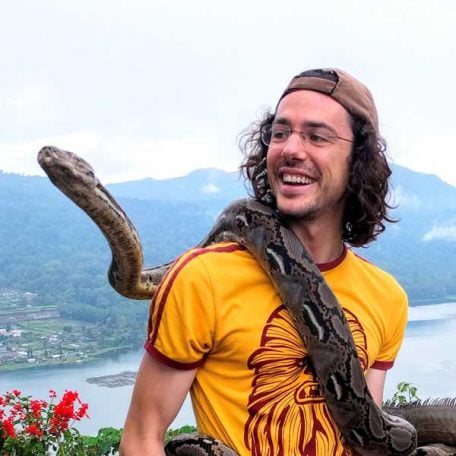
Martin Breuss RP Team on March 1, 2020
Hi @koutsellisthemistoklis!
1) Inheritance
What you are doing with the below code:
from django.db import models
class Project(models.Model):
# your code
is that you are inheriting properties from Django’s Model
class to the new model you are creating. Therefore, models.Model
is not an argument that you would need to pass when creating an instance of a class. Classes work differently in this than functions do. Check out our article on Inheritance and Composition: A Python OOP Guide and the section in our associated course.
2) Missing self
in Django Models
The very stripped-down and somewhat unusual way of defining a class in a Django model is due to the fact that Django has metaclasses that actually take on the work of creating your class.
This allows the class definition to be so clean and easy. Note that you also don’t have to write an explicit __init__()
method for a model class.
All this is thanks to metaclasses and Django’s gargantuous workload behind the scenes that aims to make webdev easier for you :)
okorobright13 on April 12, 2020
(InteractiveConsole)
from projects.models import project Traceback (most recent call last): File “<console>”, line 1, in <module> ImportError: cannot import name ‘project’ from ‘projects.models’ (C:\Users\Dell\PycharmProjects\django-portfol io\projects\models.py)
please how can i resovle this thanks to progress
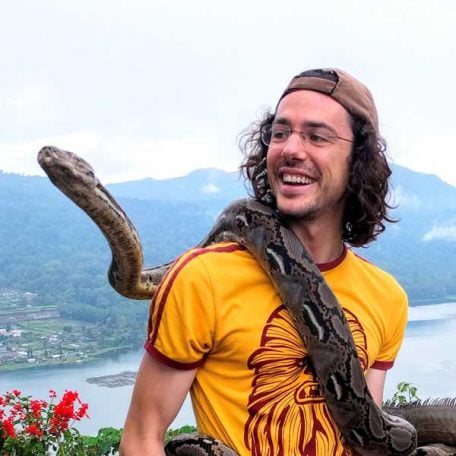
Martin Breuss RP Team on April 13, 2020
Hi @okorobright13!
Looks like this might be a typo. Your Project
model should be capitalized instead of in lowercase.
You want to import the Project
class that you defined in projects/models.py
, therefore your import statement needs to make sure it can actually find it. Computers are very literal with spelling and capitalization, so it’ll only find it if it matches exactly :) Hope that fixes it!
okorobright13 on April 13, 2020
Martin Breuss,thanks alot you are right is working now and i’m in progress for now,hahahahaha message error is our friend!!!!!1
jacob6 on April 20, 2020
>>> p1 = Project(title="test project", description="this is a test 1234!", technology="Django", image="avatar.jpg"
... p1.save()
File "<console>", line 2
p1.save()
^
SyntaxError: invalid syntax
What is going on there? I’ve been staring at it for a long time, and I can’t find the error.
jacob6 on April 20, 2020
Nevermind. I found the error. It turns out you have to be very precise with parenthesis and notation! =oP
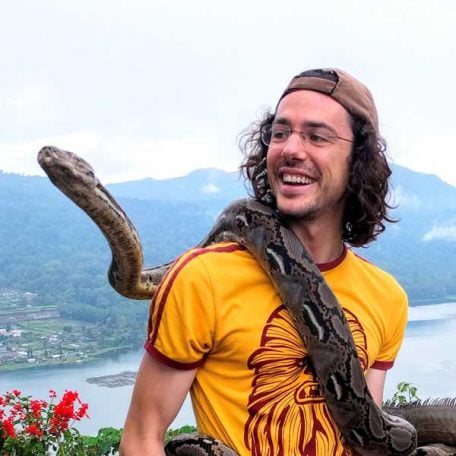
Martin Breuss RP Team on April 20, 2020
It’s a tricky one, because the arrow doesn’t point in the right place here.
Check the end of the line above where the ^
is pointing to, there’s a little thing missing…
You can notice that there’s something wrong by how the prompt starts the line where you’re writing p1.save()
, it should start with a >>>
, but insteads starts with a ...
. The thee dots mean that it’s continuing the line above.
Hope that helps you find it! :)
c4inmypants on July 27, 2020
Hey! So I have a weird question. So in the part after p1.save() I did results = project.objects.all and when I did >>>results I got this : <bound method BaseManager.all of <django.db.models.manager.Manager object at 0x03B899D0>>
I realise my mistake was not adding the () at the end and everything was fine. So my question is what does the () tell python to do? I tried googling what the shell spit out the time I did the command without () but I couldn’t find anything too helpful. Could someone explain to me I am just very curious.
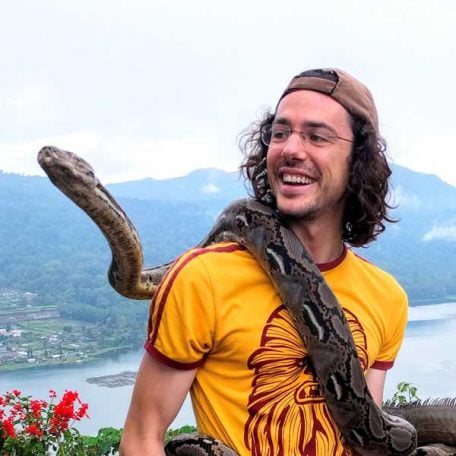
Martin Breuss RP Team on July 27, 2020
Hi @c4inmypants and nice job figuring it out and staying curious :)
The short answer to your question is that in Python, the ()
execute a function or a method.
Functions And Methods
Functions and methods have some, well, functionality encoded in them that can be accessed by calling them. That’s why using the ()
at the end of their name is called a Function Call. In your case, to be all the way precise, you’d have to talk about a “method” instead of “function” because the code that does the work is dependent on a specific object. 🙄 Don’t worry about that too much, however, for this situation, that’s mostly terminology. But if you want, you can read more about Difference Between a Method And a Function.
Your Example
So when you read that the ()
execute the method, it might make you think that there needs to be something to execute. That is the object that you are referring to and that contains the code logic. Let’s look at your example, stripped away from the variable assignment:
project.objects.all
Here you are referencing the all
method on your projects.objects
object, but you are not calling it. For all that Python knows, you want to access the method, and not to execute it. So it does your bidding and tells you what you got:
<bound method BaseManager.all of <django.db.models.manager.Manager object at 0x03B899D0>>
You can dive deeper into this output and learn a fair bit from it, but the main take-aways for this example are:
- Reference, not execution: When leaving out the brackets, Python gives you access to the
bound method BaseManager.all
of some object that lives in your computer’s memory - Execution: If you want to make the method do its job, you need to call it, which you do by adding the
()
at the end, e.g.:
projects.objects.all()
redxking on Sept. 1, 2020
The instructor set the query to p1 so after running:
p1 = Project(title="test project", description="this is a test 1234!", technology="Django", image="testproject.png")
Run: p1.save()
Then: p1
alazejha on Nov. 16, 2020
The error message is:
from projects.models import Projects
Traceback (most recent call last):
File "<console>", line 1, in <module>
ImportError: cannot import name 'Projects'
What I’m doing wrong?
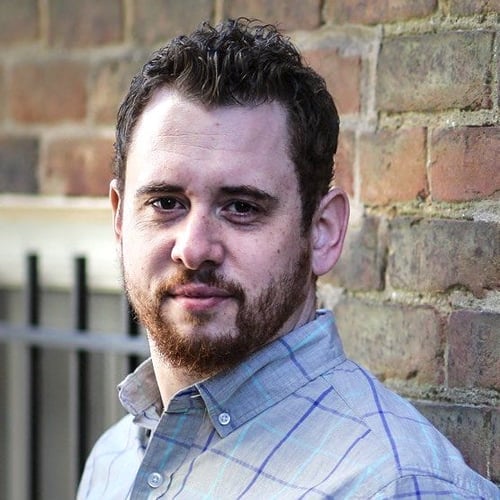
Ricky White RP Team on Nov. 16, 2020
@alazejha Hi. Without seeing your code, it’s hard to tell, but it might be a simple typo error. Are you importing the class Project
or Projects
?
James on Jan. 12, 2021
Just curious if there’s an easy way to see everything in the query object. So in this lesson you could do this:
>>> res = Project.objects.all()
>>> print(f'title={res[0].title}, description={res[0].description}, technology={res[0].technology}, image={res[0].image}')
That’s pretty tedious though. The only thing I can think of is to peek inside the object and filter out private attributes:
>>> {k: v for k, v in res[0].__dict__.items() if not k.startswith('_')}
Is there a better way or if you want to peek inside the database you should a database tool instead?
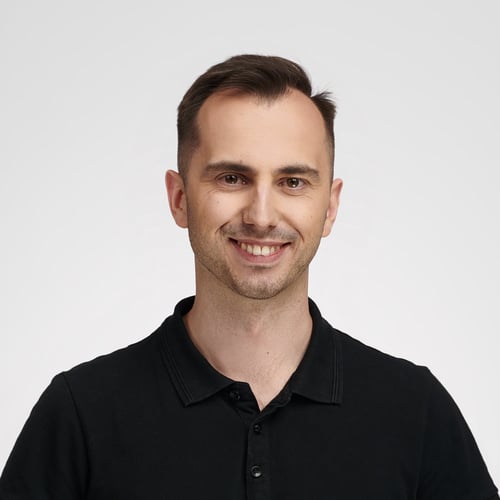
Bartosz Zaczyński RP Team on Jan. 13, 2021
@jamesrsmall Normally you’d use a dataclass, which implements the string representation out of the box:
>>> from dataclasses import dataclass
>>> @dataclass
... class Project:
... name: str
... age: int
...
>>> Project("foobar", 42)
Project(name='foobar', age=42)
Since Django models use descriptors, they aren’t compatible with dataclasses. However, you could try whipping up a custom mixin class to encapsulate your logic:
class ReprMixin:
def __repr__(self):
attrs = ", ".join([
f'{name}="{getattr(self, name)}"'
for name in vars(self) if not name.startswith("_")
])
return f"{self.__class__.__name__}({attrs})"
class Project(ReprMixin, models.Model):
name = models.CharField(max_length=20)
age = models.IntegerField()
Then, in your Django shell:
>>> from projects.models import Project
>>> Project.objects.first()
Project(name="foobar", age="42")
Note that this code snippet is super simple and doesn’t account for recursive traversal or even handling different data types. I used to have a thorough implementation of this in one of my projects, but can’t find it anymore.
There are plenty of third-party libraries on PyPI that may do what you’re looking for. Try searching for “repr” or “str”.
James on Jan. 14, 2021
@Bartek - very cool, thanks! I appreciate the example and links.
gburbano92 on Jan. 26, 2021
Hi, I’m facing some difficulties having the “daily”, “test project”, “todo” images appear in the img file. I’ve completed as instructed the double file projects>img but no images, through my desktop I can see the files are present in my django portfolio>projects>static>projects>img file so not sure what’s causing the no show in my virtual environment.
crispineda34 on March 23, 2021
For this command I keep getting this error. I’m not exactly sure on how to proceed. “Model class projects.models.Project doesn’t declare an explicit app_label and isn’t in an application in INSTALLED_APPS.”
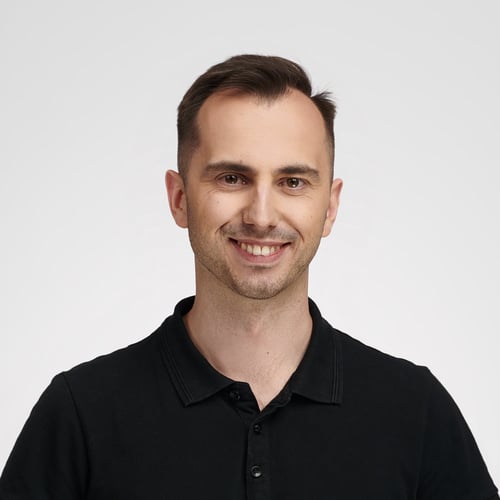
Bartosz Zaczyński RP Team on March 23, 2021
@crispineda34 If you google that error message and follow the first search result, you’ll land on a StackOverflow question, which seems to have the right answer for you:
Are you missing putting in your application name into the settings file? (…)
applejfl on Nov. 28, 2021
I can’t get pass this:
from projects.models import Project
>>> p1 = Project(title='test project', description='this is a test, 1234!', technology='Django', image='testproject.png')
>>> p1.save()
Traceback (most recent call last):
File "C:\users\apple\documents\django-portfolio\.env\lib\site-packages\django\db\backends\utils.py", line 84, in _execute
return self.cursor.execute(sql, params)
File "C:\users\apple\documents\django-portfolio\.env\lib\site-packages\django\db\backends\sqlite3\base.py", line 423, in e
xecute
return Database.Cursor.execute(self, query, params)
sqlite3.OperationalError: no such table: projects_project
# models.py
class Project(models.Model):
title = models.CharField(max_length=100)
description = models.TextField()
technology = models.CharField(max_length=20)
image = models.FilePathField(path='/projects/img')
Thanks!
applejfl on Nov. 28, 2021
Nevermind, I found the problem
mochibeepboop on Sept. 9, 2023
Wonderful course :) I have a question: Why is the path to the image in models.py not
projects/static/projects/img
And instead is
/projects/img
Here is the entire code in projects/models.py, I am referring to the last line:
from django.db import models
class Project(models.Model):
title = models.CharField(max_length=100)
description = models.TextField()
technology = models.CharField(max_length=20)
image = models.FilePathField(path="/projects/img")
Thank you!
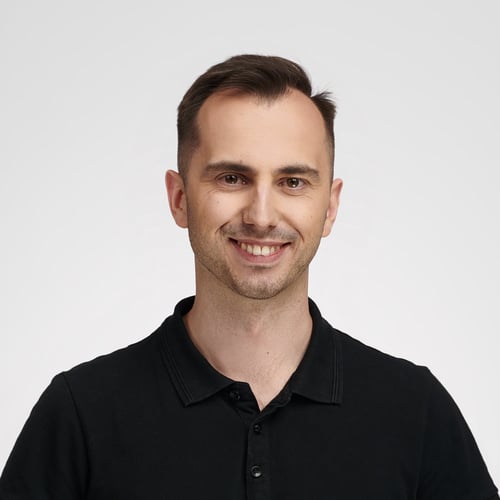
Bartosz Zaczyński RP Team on Sept. 11, 2023
@mochibeepboop Great question! It’s because Django automatically looks for static files in each application’s static/
folder, so you only need to provide a path relative to it.
mochibeepboop on Sept. 11, 2023
Thank you for your response @Bartosz Zaczyński! So do we essentially get ‘projects/static/’ for ‘free’? Meaning Django prepends this part of the path in the background?
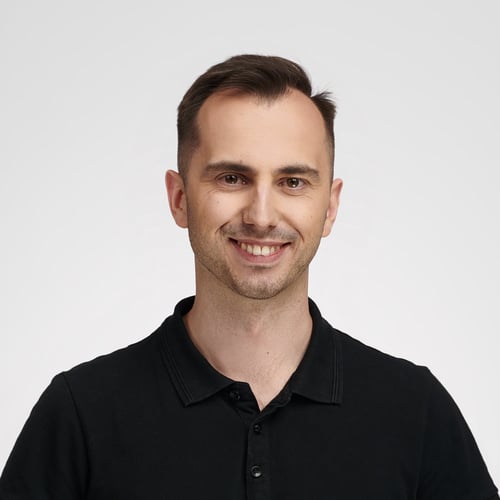
Bartosz Zaczyński RP Team on Sept. 11, 2023
@mochibeepboop Yes, that’s exactly right.
Become a Member to join the conversation.
Pygator on Oct. 13, 2019
But how would you practically populate your data base if you had 1000s or millions of sample rows?