Migrations: SQLite
It’s very important to understand the folders and files that make up a Django project. In this lesson, you’re going to take a look at migrations and SQL databases.
Inside your projects
folder, a folder called migrations
has been generated. If you run the development server, you’ll see that there are migrations that haven’t been applied.
The database isn’t currently set up, and that’s what migrations do. They take the code you have and apply it to create or change the database that you’re working with.
00:00 Welcome back! I don’t want to dwell on this for too long, but I think it’s really important to understand what are the folders and files that make up a Django project, so we will take a look at migrations and also take a peek into the SQL database in this video. Let’s start with migrations.
00:18
You might’ve seen this folder generated here. It’s called migrations
, inside of your project. Maybe you don’t have it yet, but usually, it comes right away. You can see it’s empty right now.
00:29 So, what’s this folder about? For this, I want to show you something that we’ve blissfully ignored, so far. If I go ahead here and I run the development server…
00:45
we’re starting up our project, right? And now I already opened up the terminal far enough. You’ve probably seen this before if you ran the server. It tells me this very warning-like looking message in red: 17 unapplied migration(s).
So, here we’ve got the word popping up, migrations, and then it tells us the project may not work properly until the migrations are applied. And we see these things here: admin
, auth
, contenttypes
, sessions
.
01:12 All right. So, first of all, again, this is your friend, right? We’ve got nice error messages here in Django. It’s just giving us some information. You might have seen those before.
01:25
Let’s remember quickly where we’ve seen them: it was in our management app in settings.py
.
01:33
So, if we scroll all the way to the top where we added our first app projects
, way back in section two, I think? We read over those ones here, you see? admin
, auth
, contenttypes
, sessions
.
01:46 So, these first four of those, they apply to other installed apps. What this is telling us, essentially, it’s just that the database is currently not yet set up, and that’s what migrations do.
01:57
Migrations take the code that we have in here and apply it to create or update or change the database that we’re working with. Now, again, Django is very helpful and tells us to just run python manage.py migrate
to apply them. So remember, always read what Django tells you. It’s very helpful.
02:18
And another thing here, before we actually go ahead and try that out, I want to show you a thing in settings.py
: there’s a DATABASES
section. The default one that comes with a Django app when you start it up is just a SQLite database. We’ve got an 'ENGINE'
that tells us, “Use this connector to allow us to interact with SQLite.” We’ve got a 'NAME'
, and the default 'NAME'
is just 'db.sqlite3'
, which you might have seen around here. There it is, okay.
02:50 So, this is our database in the Django project. By default Django works with a file-based SQL database—that’s SQLite—so we don’t need to run a separate database server, but everything gets stored inside of here. And that’s just a file, so—you might already know that there’s some limitations to this, for reading and writing to the file.
03:10
You wouldn’t want to use this in production because it would be very slow, but for development, it’s perfectly fine and it takes away complications that we have with otherwise setting up databases. Eventually, you’re going to look into this more, and it’s pretty simple to change out just these settings here in the settings
file to connect to a different type of database—for example, a Postgres server that you have running somewhere.
03:34
Okay. But for now, we are going to write to this database file db.sqlite3
, and we’ve learned that our migrations are the changes that happen to the database. So inside of this folder, once we go ahead and follow Python’s tips here—I’m going to open up a new terminal tab for that.
03:54
I’ll say python manage.py
—
03:59
what is it suggesting? migrate
. So, it’s just telling us to migrate. There’s actually two steps for this. The first step is to say makemigrations
, and we can specify which app we want to makemigrations
for.
04:13 For now, I’m just going to leave it empty, it should apply to all of them.
04:18
Then, you see it tells us that it created the migrations for projects/...initial.py
: Create model Project
. So the default ones by Django already have makemigrations
applied, that’s why you can run migrate
right away. But for the new one,
04:35
our Project
model here, this just got created. So inside of our projects
app, we can now see this 0001_initial.py
migration file sitting.
04:47 You generally are not going to have to deal with this at all, but I want to just take a look so that you know what’s going on, on a very rough level. If you open up this file, there’s lots of Python code in here and we don’t need to understand this, but just know that what it does—this is the step in between translating to SQL.
05:07
So if you run makemigrations
, this migration file gets generated, and it’s, essentially, a description of what’s going to happen when this migration gets applied. Nothing has happened yet to the actual database, but this file got created. So now, we’re on the second command, the one that’s been suggested to us here.
05:29
If I say this, it’s going to take the migrations file and apply the migrations to the database. So running this: blah, blah, blah, blah, we got lots OK
s. And you see a lot of those OK
s, they don’t actually apply to our projects
app that we created, but to those other apps that Django already comes with: admin
, auth
, contenttypes
, you can see them here. Those migrations already existed before, so now they’re just getting applied—blah, blah, blah, blah—all the way down.
05:58
And then we have projects
here—ours. That’s exactly this file here that we created with running makemigrations
. This one also gets applied in this one migration.
06:09
Now, our database is set up. Before, we didn’t have anything in there yet. So, it’s important to run these two commands: makemigrations
if you create a new app, and then migrate
to actually apply the migrations.
06:21 And now, if we go ahead and run our server—I’m going to stop it here.
06:27 If I run the server again,
06:31 boom. Those scary, but actually helpful, messages are gone because all the migrations are applied. Sweet! Now, let’s take a look at what happens inside of a SQLite file.
06:45 Think of it as what we talked about before, a relational database, and now we’re just going to take a peek inside of that. Also, just so that you have an idea of what’s going on in there without digging too deeply. I’m back here with the finished app, just so that we can see, also, something with data.
07:03 One thing that the professional PyCharm version has included is a database viewer, so I’m going to open up that one. If you don’t have this, if you just have the community edition, there’s free tools out there—“SQLite database inspector,” just Google it—and you can find something like that.
07:21
It makes it nice because it gives you a visual way to look into your database. You see, we have it here: db.sqlite3
(database sqlite3
).
07:29
With the database viewer, I can take a look inside, so just opening up some of these things. And here you see all the tables that we have. Those are the ones that got created when we ran migrate
, just before.
07:43
Let’s take a look at one that has some interesting data in there. So, projects_project
—our Project
model that we just created before, I can now inspect it.
07:54 I’m going to look at this.
07:59 It queries our database, and now we can see in here. That’s the contents of the database.
08:07
We can see the id
, title
—so, the columns in the same structure that we’re used to seeing like a table. Because that’s all it is, like, these ones are all tables that got created. This one has the data inside of it.
08:19 Sweet. So, there’s some other cool things you can do with the database inspector. For example, we could say…
08:56 Okay. “Show me a diagram of the whole database.” So, this now includes everything, all the authentication tables that are set up by default in Django, but also the things that we’re going to create. So here’s the blog tables, here’s our project table, the one that we just created. That just gives you a nice way to inspect what’s going on in your database, if you’re interested in that side.
09:21 If you’re not, you’re lucky because you don’t really have to deal with it when you’re using Django. So, this is my little excursion inside of a SQLite file, so that you know what’s actually happening in there, and that there’s just a table structure—as with any relational database—contained within one file. And that sums it up for the database part for now.
reblark on Oct. 22, 2019
So I entered the second command. ptython manage.py migrate aand it worked. Am I OK? It did not apply projects.
reblark on Oct. 22, 2019
I am doing two parallel implementations watching the tutorial twice. In both of my attempts, the is no “projects” enter under #my apps in the settings.py file. Why? I believe I have entered everything diligently.
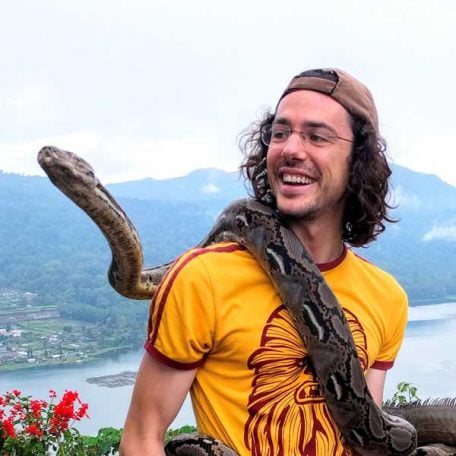
Martin Breuss RP Team on Oct. 23, 2019
Hei @reblark. Thanks for bringing this to my attention! Indeed, one of our videos seems to have accidentally gone missing. We’ll fix that asap.
As you discovered, the migrations (and a couple of other things) won’t work unless you register your app in the project’s settings.py
file.
Check out the relevant section in the Supporting Materials. You’ll have to manually type the name of each app that you add to your Django project into the INSTALLED_APPS
list in settings.py
:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'your_app_name_here', # in this case it'll be 'projects'
]
Django’s migrations also rely on the fact that the app is correctly registered, so once you’ve added it here, this part should work as well. Give it a go and let me know whether that fixes it!
reblark on Oct. 23, 2019
Thank you. The amazing thing is that I went back to that section and read the text. The text had this information in it but, as you have noted, the video did not. Thank you very much for responding.
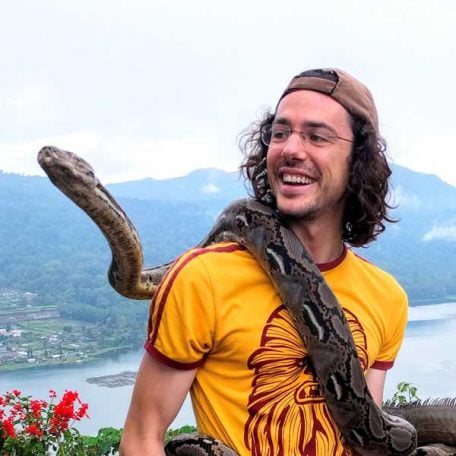
Martin Breuss RP Team on Oct. 24, 2019
Sure thing, the video was part of this course all along, just slipped through the cracks when getting the course up on the platform. It’s all set now, thanks for noticing and letting us know :)
reblark on Nov. 1, 2019
How did you get SQLLite3 in that third frame in PyCharm?
reblark on Nov. 1, 2019
If I click on db.sqlite3 in the first frame, “db” appears in the third frame, but not sqlite3 and none of the information that you show in the video.
reblark on Nov. 1, 2019
OH, and I do have the professional version of PyCharm.
reblark on Nov. 4, 2019
Aha, in going back to try to figure out where my database is, I see that I asked this question before but it didn’t get answered. So, I sent a message to Jetbrains, but it seems that you know PyCharm quite well and should understand the problem. If I can’t see the database entries, and I can’t, what do I do? I know in a later video you show the file structure and database entries in the coding section of PyCharm but, of course, I do not see those. I really need to resolve this. I am in the process of going through all the videos again. But, it seems to me that the makemigrations/migrate sequence should provide this facility (i.e. the database viewer holding sqlite3).
reblark on Nov. 4, 2019
How do you get the screen displayed a 6:58 ?
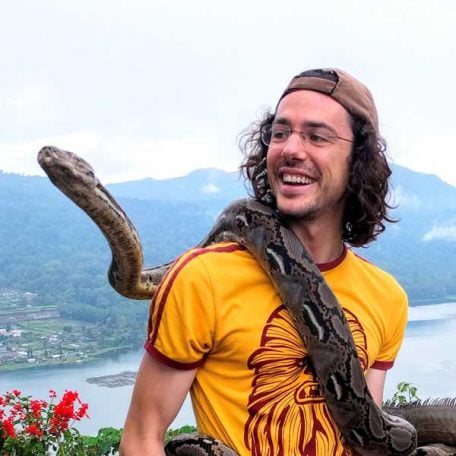
Martin Breuss RP Team on Nov. 5, 2019
Hei @reblark–I answered your question regarding the database viewer in another comment.
Another useful PyCharm tip: If you click <kbd>Shift</kbd> twice quickly in PyCharm, it opens up the Search Everywhere dialog. Any time you don’t know how to find something in PyCharm, you can use this dialog to simply type the name of the feature.
In this case that could be typing:
Database Viewer
And you should be able to open the window like that.
Google it! Also, googling for the task you want to achieve really helps. PyCharm has a great documentation of its features, so if you google something like:
How to open database viewer pycharm
you’ll find their docs on the topic that also show you how to open up that window.
I’m happy to help you out, but for your own best interest it is really crucial that you don’t get yourself stuck waiting but instead practice the habit of venturing out into the world-wild-web and taming those search terms 🤠. You got this! :)
reblark on Nov. 7, 2019
Thanks for the response. I would like for you to know that I don’t sit around waiting for a response and posting a comment is not my first reaction to a problem. Also, “errors are your friends” has made a huge difference to me and I have had many rewarding moments thanks to your advice. I appreciate the tips on PyCharm and will use then. In fact, the problem I was having with PyCharm is one of the problems I solved on my own. I will get better and better at it.
I do think there is a problem in the debugging videos as I have revisited my code so many times and even when it perfectly matches your code, the results do not perfectly match.
I noticed in a very early lesson that the video presentation regarding “base_dir” was quite different from the text presentation.
It seems like this is a bit of a work in progress which I find incredibly helpful. When you make mistakes and fix them, that is a good learning moment.
Thank you, Martin.
BTW, I think this is a bit awkward in terms of communications. One a response appears in the comment section of a lesson that I have moved on from, then it while before I see it, if ever. I think your responsiveness is great, but the mechanics of it could be improved. Cheers. Ralph
Lokman on Jan. 6, 2020
Is database language compulsory to learn? And there is others database platform that offer etc sqlite, postsquel and so on. Or are there is the same language?Thanks
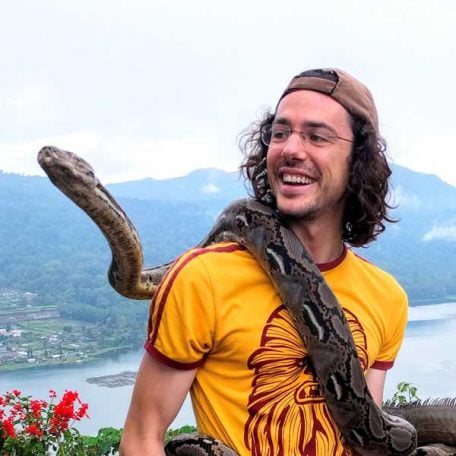
Martin Breuss RP Team on Jan. 7, 2020
A very common Database Language is SQL, which comes in a couple of slightly different flavors (e.g. SQLite, PostgreSQL, MySQL, etc.) They are all essentially the same language, yet have some small differences in their syntax.
When you are using Django, you (theoretically) never need to write any SQL directly. You’re instead using Django’s ORM to interact with the Database, and Django’s ORM works in a (+/-) pythonic manner. That brings some advantages:
- you won’t need to know SQL to interact with a SQL Database
- you won’t have to write different flavors of SQL when interacting with different SQL backends
- you are even able to interact with non-SQL databases while still using the same syntax
Django does the heavy-lifting for you and all you need to know is how to use Django’s ORM.
However: SQL Is Very Common!
When you’re applying for a job as a web developer, it is extremely likely that your future employer will require you to know some SQL. It is a common language for interacting with databases, so learning some SQL is worth the effort and can be fun :)
Lokman on Jan. 8, 2020
Thanks @Martin after go through your course at debugging session. I start to understand about ORM.
bluebe55yPie on Feb. 9, 2020
Hello, I’m using PyCharm community so am not able to easily connect to a database. I’ve installed SQLite onto my computer, but how do I then connect that to PyCharm as you haven’t mentioned these steps. Thank you
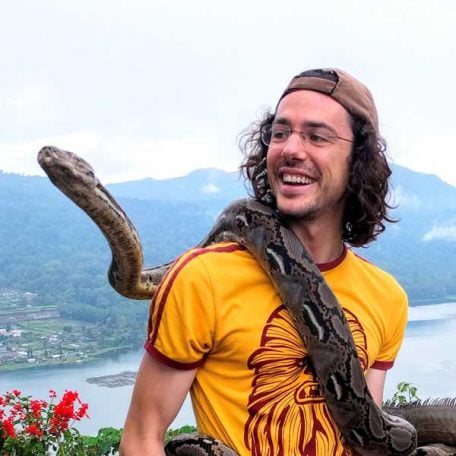
Martin Breuss RP Team on Feb. 10, 2020
Hi @bluebe55yPie! SQLite is a file-based database and when using Django, there’s nothing additional that you need to install. You can already interact with your SQLite database out of the box.
GUI: Viewing Content of a SQLite Database
In order to get a nice visual representation of the contents of your SQLite file, you can use the free DB Browser for SQLite. You can open the SQLite file that Django creates when you migrate for the first time with this program and get the same nice view of your database that you get with PyCharm’s Pro version.
CLI: Viewing Content of a SQLite Database
Another way to inspect the contents of your database file is through using the command-line. First, open the SQLite file using:
sqlite3 path/to/your/django/project/db.sqlite3
Now you can interact with the database through SQL commands, as well as some SQLite-specific dot-commands. E.g. you can list all available tables like so:
sqlite> .tables
To further inspect the content of a specific table, you’d have to write some raw SQL, e.g.:
sqlite> SELECT * FROM table_name;
NOTE: the sqlite>
part of these commands just indicates that you’re successfully connected to your database file. You should see that prompt when typing the above commands, but you don’t need to type it out yourself.
Inspecting your SQLite database in that way can be a nice SQL-practice, if you’re into that kind of thing. Otherwise, using the GUI DB Browser for SQLite is a good option as well.
bluebe55yPie on Feb. 15, 2020
Amazing, thank you :)
reblark on Feb. 23, 2020
After running migrations, I looked at the tables in the database and none of the blog tables are in the “main” folder as shown in the video. I know the application needs them. How do I get them in there?
reblark on Feb. 23, 2020
First, where do those “blog” tables come from? Could I dump the migrations directory and redo the makemigrations and migrate commands?
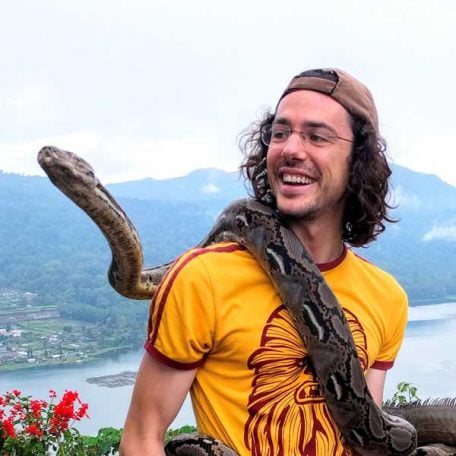
Martin Breuss RP Team on Feb. 24, 2020
Dumping Your Migrations
Yes, you can do that. Django will figure out what the right structure for your database is according to how you’ve set up the models. Therefore it will recreate the structure of your database again after you delete your migrations/
folder.
The idea of keeping the migration files around is essentially one of version control. You want to keep track of the changes that were applied to your database, so that:
- someone you are working with remotely can get the database in the same shape as yours
- you can roll back to older versions of your database structure
Missing Migrations
Did the other migrations get applied correctly? If it is missing for one specific app, you might have to run it for that app specifically:
python manage.py makemigrations blog
python manage.py migrate blog
It is possible that the app is not automatically included if e.g. you forgot to add it to the INSTALLED_APP
in your management app’s settings.py
file.
reblark on Feb. 24, 2020
After migrations, my db viewer does not show any collations but yours shows 3 collations. Where might mine be?
reblark on Feb. 24, 2020
There is just something wrong with whatever I did with migrations. I don’t have the blog tables, I don’t have 3 collations, and the viewer didn’t work the your did. Is there someway to erase the migrations and then try it again?
reblark on Feb. 24, 2020
sorry. I found the collations but have not found the blog tables.
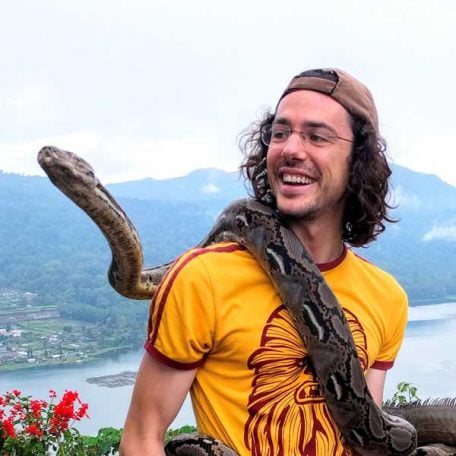
Martin Breuss RP Team on Feb. 24, 2020
What did you get when trying to run it specifically for blog
as I suggested in the comment above?
And did you verify that you have the blog
app added to INSTALLED_APPS
in settings.py
?
reblark on Feb. 24, 2020
Whoa! this is interesting. “blog” is not an app that we install per se during the video, yes? but when I run makemigrations blog, I get the message that there is not an installed app named “blog.” I can see that in the settings file. My app is there and django.contrib.admin,auth,contenttypes,sessions,messages, staticfiles are all there. No ‘blog’ file. When I do “visualization” it all looks fine except for the blog files. Amazing. Is the “blog” file just a gift from the heavens?
reblark on Feb. 24, 2020
BTW, your exceptional responsiveness really makes this fun.
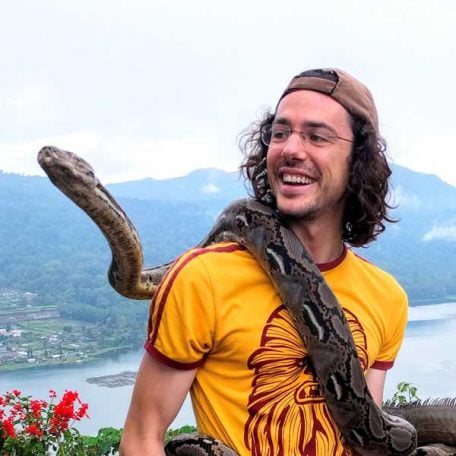
Martin Breuss RP Team on Feb. 24, 2020
That’s correct! Well spotted 🔎!
In this course, we are not building out the blog
app. Instead we’re focusing on the projects
app to get a handle on Django’s basic concepts and make some new friends :)
The tutorial the course is based on goes over building out the blog
app as well, and the code I am sometimes working with when I show the finished project therefore also includes a blog
app.
If you never ran python manage.py startapp blog
and didn’t set up the models, nor add it to settings.py
(and all the other fluff that needs to be done), then there will be no blog
tables that descend upon you from the heavens. Ever. While Django might sometimes seems a little transcendent 🎩, it is after all just a bunch of code on a pile 😜
reblark on Feb. 25, 2020
Was the blog app originally in the video then dropped?
reblark on Feb. 25, 2020
So I ran “python manage.py startapp blog
No errors, but where do I find the blog app then? I looked in “installed_apps” in my pycharm and in your pycharm. I don’t see “blog” in your structure in the migrations video, but I have seen it in other videos. It was in the “install_apps” and there were three entries.
reblark on Feb. 26, 2020
Keeping comments in each video has its confusing results. I can’t find my long comment about “structure,” so that I could tell you that I have worked it out. It took a little time but I found the error. Sometimes you don’t get error messages, you just get no results. I like error messages much, much better. Anyway, I have not seen a response from you. That comment I sent ended in the Danish word for ‘comprendo?’ Hope you got it.
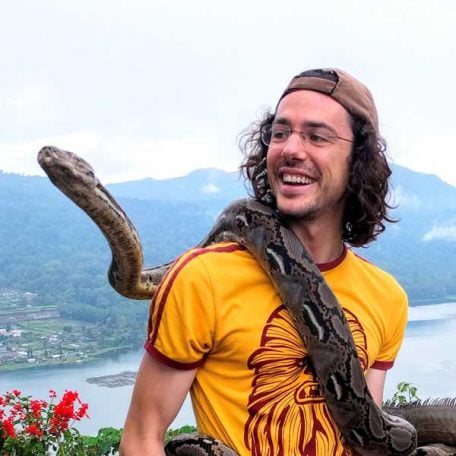
Martin Breuss RP Team on Feb. 26, 2020
Hi @reblark!
I initially considered building the blog
app as well, but the course was too long already with just projects
. We do have Jasmine’s article that walks you through building it out as well.
Creating And Registering A Django App
Check your directory structure. What Django does when running:
python manage.py startapp your_app_name
is that it creates a new Django app with all the files associated in your project’s base directory. You should have a folder called blog
sitting next to your projects
folder.
Running this command does not register the app, so if you want to use the new app it in your project, you have to add it manually in settings.py
. The process is exactly the same one as what you went through for the projects
app, so you can go back through those early videos for creating an app and registering an app.
Comments
Yes, the structure of these courses and the comments are not really set out for mentoring learners through the course, but more to add notes and… well comments to the individual lessons :)
I believe you mean this comment here?
You could post your solution under there, that would make it helpful for future learners going through the course :)
reblark on March 9, 2020
Back to the question of the “blog” app. How do you install it? where does it come from? Surely you don’t just type the word blog in the “Installed Apps” section.
reblark on March 9, 2020
In this video, when you “migrate” and then use the db viewer on projects_project, there are three records already in the database. How did they get there?
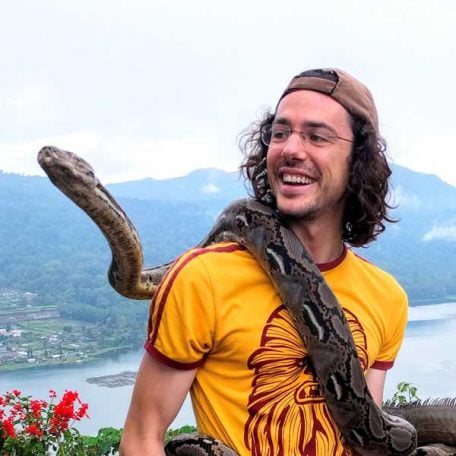
Martin Breuss RP Team on March 10, 2020
Hei @reblark. Check my comment just above. You create an app in Django as I described it in Creating And Registering A Django App. You’d need to run the command with the name blog
to create the basic structure of a Django app, then build it out following the article to make it functional. Lmk in case I misunderstood your question.
reblark on March 10, 2020
I had the impression that the blog app is a real app that is already built and that can be used in the projects project. It sounds like you saying that you just name an app and register it. It’s nothing more that a holding place and could be named blag for all anybody cares.
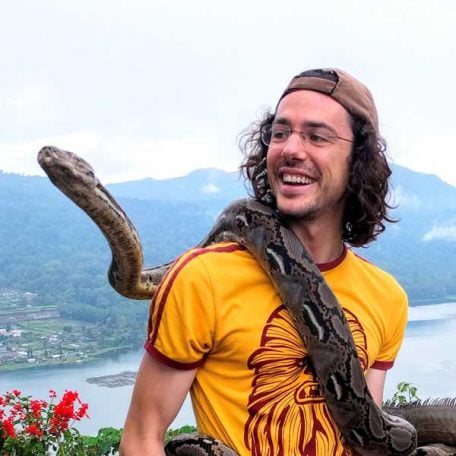
Martin Breuss RP Team on March 10, 2020
Yes, it’s not a Django app that already exists, but one that you can build yourself following the written tutorial. As you say, it could be named anything, as it’s just a new app you are creating.
Muthukumar Kusalavan on May 2, 2020
Hello Mr.Martin, I could not able to view the database view.
I did as you mentioned search tool: “Action: Database”. but it did not listed any schema or table. i did all the changes that you mentioned earlier.
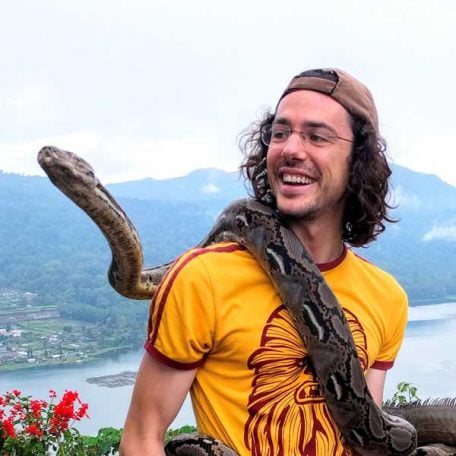
Martin Breuss RP Team on May 2, 2020
Hello Muthukumar. The database tool is only available in PyCharm’s Premium version, not in the Community Edition.
Check out this free tool called DB Browser for SQLite. You can inspect your SQLite file with it via a GUI :)
Muthukumar Kusalavan on May 3, 2020
@Martin Breuss, Thanks a lot, Mr.Martin. i plan to create an e-commerce website using Django/python and place it in AWS, could you suggest me some tips. i am just begineer.
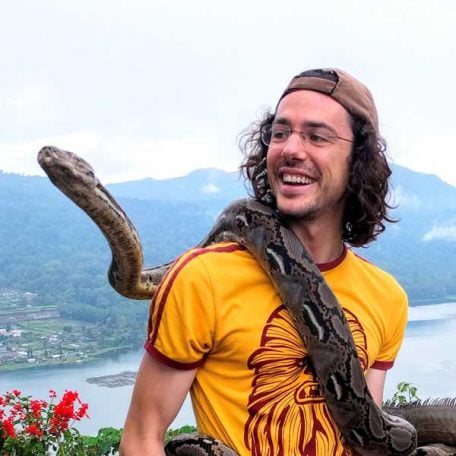
Martin Breuss RP Team on May 4, 2020
That’s a bunch of work, but Django/Python is a good choice for it. :)
If you just want to get it done, then I would suggest that you look for ready-made e-commerce projects on GitHub. I am sure you can find some maintained projects that were built with Django.
If you are doing it to learn Python/Django, then look for tutorials and go step-by-step. There are great free tutorials out there, e.g. MDN’s LocalLibrary App tutorial. Build out one, two, three tutorials, then try to apply what you learned to your own app idea. It’s about training and synthesizing your knowledge.
You can do it! There’s great resources out there and in the end it’s about your grit and how much you stick with it. :)
And if you’re interested in a more ready-made curriculum that walks you through all the steps (including deployment!) with a mentor, then you can check out my new Django course that’s going to launch in just a week or so over at CodingNomads.
Hope that helps and stick with it, Django’s a great framework for web development!
Muthukumar Kusalavan on May 7, 2020
Thank you very much Mr.Martin for your tutorials. Thank you very much that you mentioned about the tutorials like e.g. MDN’s LocalLibrary App tutorial and CodingNomads, i will definitely go through it and update you when i created something. thanks and cheers :D
bluebe55yPie on May 13, 2020
Hi Martin, thank you so much for a great tutorial. I’ve learnt so much :) I’ve decided to change the look and feel of the portfolio using Bootstrap, HTML, CSS and JavaScript… But I’ve run into a little issue, which I was wondering you might be able to help me with?
I have a few SVG images on my portfolio page, which I’ve made interactive using JavaScript, but when I view this on IE 11 it’s all over the place and looks awful! I would like to say something like “if IE 11 then show SVG files as static images” but I’m not sure where in Django this would go…when looking online some people are saying in the HTML file, others are saying in the Manager area, or Views…so now I’m confused…and if I do need to put something in Views do I need to write a function for IE?
I have a feeling that might be the thing I need to do… but then would I need to create a separate HTML template specifically for IE11? Bit lost really…any help would be greatly appreciated! Thank you! :)
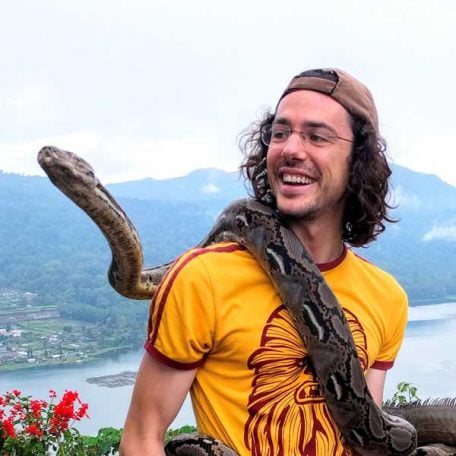
Martin Breuss RP Team on May 14, 2020
Hi @bluebe55yPie and welcome to the (horrible) world of cross-browser inconsistencies… 😱
One thing upfront: from all I know this is not a Django issue to fix, but something that you will have to deal with in your HTML+CSS. Cross-browser styling lives in the realms of Front-End Web Development 😌
Here are two links that I found that you could look into if you want to go down that path. Just keep in mind that it might be a tricky task to get it all done. Internet Explorer is a known … challenge in the front-end web development world!
Hope this helps and gets you on the way!
bluebe55yPie on May 14, 2020
Hi Martin, Thank you so much for your quick reply :) Good to know that it’s a Front End Web Development, so it narrows it down a bit to just HTML and CSS I need to deal with! I think I may have got myself confused as I thought it was something I need to change in Django and I made it all complicated for myself :( Hahaha, yes, I’ve noticed that Internet Explorer can be a nightmare from all the things I’ve read online, so I’m bracing myself for the challenge! Thank so much for the links and the help! It’s massively appreciated! I can now move forward in the right direction. :) :)
santhoshmallojwala on Sept. 10, 2020
Hi Martin,
I am just wondering how the sample data got inserted into projects_project table. Because we have not inserted any rows or test data into it rather we just created the table using the models object.
For example, I am using MySQL and able to connect to it from Django app. Also, I inserted few records in the table manually. How can I read those records in application.
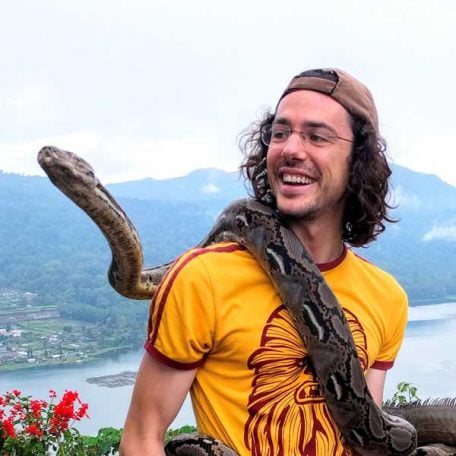
Martin Breuss RP Team on Sept. 10, 2020
Hi @santhoshmallojwala. Yes, you won’t see any data in your tables yet, because we haven’t put anything in there yet. If you double-check around this time of the video, I mention that here we’re looking at the finished project, so that you can actually see some data in the database file :)
You’ll be adding data to your database in just a bit, in the Django Shell video that’s coming up.
santhoshmallojwala on Sept. 14, 2020
Thank you Martin :)
iwatts on Sept. 22, 2020
Hi - Just in case anyone else is having the same problem:
- Problem: I have run the migrations which all seemed to work succesfully but when I open up the database in the Database Viewer (Professional Edition) there is no schema or anything - just completely empty
- Solution: When I clicked on Data Source Properties it told me I had missing drivers - once I installed them and refreshed everything appeared
Hope that helps someone.
Vasanth Baskaran on Aug. 20, 2021
Yipee, Going through all the comments itself is a great learning experience…(giving myself a pat on my back)
Thanks @Martin for the wonderful byte sized yet powerful course videos!
hillchristop on Dec. 1, 2023
When I typed python manage.py makemigrations
, I got a response that says “No changes detected.
Note: the following is what I have in the settings.py
file:
"""
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
# my apps
'projects',
]
"""
How can I fix this problem? Thanks!
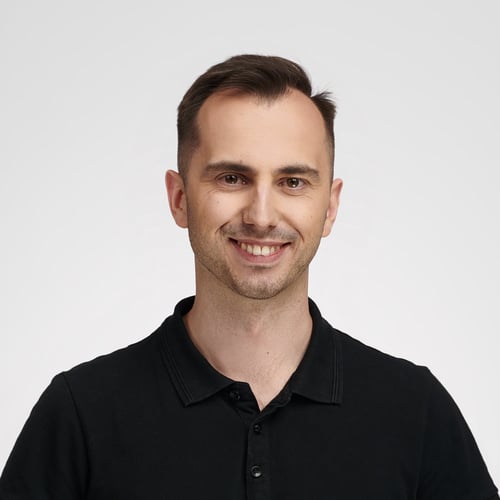
Bartosz Zaczyński RP Team on Dec. 5, 2023
@hillchristop There could be a couple of reasons. Maybe you’ve already run the migrations? Did you spell the project name correctly? Are the models defined in the right place? What’s the current state of your database? These are just a few questions, but there could be more.
The quickest fix would be to delete the migration scripts generated by Django and try again. If that doesn’t help, then something must be wrong with the code. You can always download the supporting materials to compare it with your project.
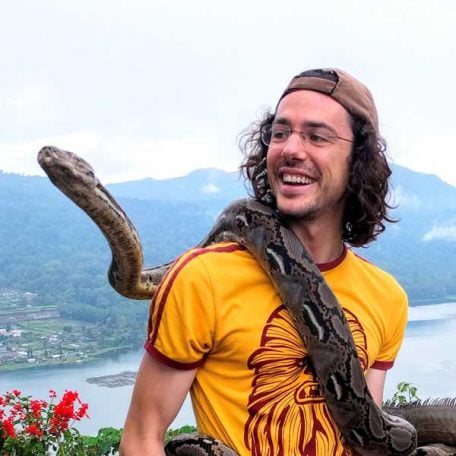
Martin Breuss RP Team on Dec. 6, 2023
@hillchristop also from the code you shared, it seems like you commented-out the INSTALLED_APPS
? I assume that this is just a formatting hiccup when posting, but if it’s also wrapped in triple-double-quotes in your code, then that would cause the migrations not to work.
Become a Member to join the conversation.
reblark on Oct. 22, 2019
when I typed in python manage.py makemigrations I get a response that says. No changes detected How do I fix this. The is no 0001_initial.py file in migrations.