String Formatting Methods
In this lesson, you’ll explore string methods that modify or enhance the format of a string:
str.center(<width>[, <fill>])
str.expandtabs(tabsize=8)
str.ljust(<width>[, <fill>])
str.rjust(<width>[, <fill>])
str.lstrip([<chars>])
str.rstrip([<chars>])
str.strip([<chars>])
str.replace(<old>, <new>[, <count>])
str.zfill(<width>)
Here’s how to use str.center()
:
>>> s = 'spam'
>>> s.center(10)
' spam '
>>> s.center(10, '-')
'---spam---'
>>> s.center(3, '-')
'spam'
Here’s how to use str.expandtabs()
:
>>> s = 'a\tb\tc'
>>> s.expandtabs()
'a b c'
>>> s.expandtabs(4)
'a b c'
Here’s how to use str.ljust()
:
>>> s = 'spam'
>>> s.ljust(10)
'spam '
>>> s.ljust(10, '-')
'spam------'
>>> s.ljust(3, '-')
'spam'
Here’s how to use str.rjust()
:
>>> s = 'spam'
>>> s.rjust(10)
' spam'
>>> s.rjust(10, '-')
'------spam'
>>> s.rjust(3, '-')
'spam'
Here’s how to use str.lstrip()
:
>>> s = ' spam bacon egg '
>>> s
' spam bacon egg '
>>> s.lstrip()
'spam bacon egg '
>>> t = ' \t \n spam \t \n egg \t \n '
>>> t
' \t \n spam \t \n egg \t \n '
>>> t.lstrip()
'spam \t \n egg \t \n '
>>> link = 'http://www.realpython.com'
>>> link.lstrip('/:pth')
'www.realpython.com'
Here’s how to use str.rstrip()
:
>>> s = ' spam bacon egg '
>>> s
' spam bacon egg '
>>> s.rstrip()
' spam bacon egg'
>>> t = ' \t \n spam \t \n egg \t \n '
>>> t
' \t \n spam \t \n egg \t \n '
>>> t.rstrip()
' \t \n spam \t \n egg'
>>> x = 'spam.$$$;'
>>> x.rstrip(';$.')
'spam'
Here’s how to use str.strip()
:
>>> s = ' spam bacon egg '
>>> s
' spam bacon egg '
>>> s.strip()
'spam bacon egg'
>>> t = ' \t \n spam \t \n egg \t \n '
>>> t
' \t \n spam \t \n egg \t \n '
>>> t.strip()
'spam \t \n egg'
>>> link = 'http://www.realpython.com'
>>> link.strip('w.moc')
'http://www.realpython'
>>> link.strip(':/pth w.moc')
'realpython'
Here’s how to use str.replace()
:
>>> s = 'spam spam spam egg bacon spam spam lobster'
>>> s.replace('spam', 'tomato')
'tomato tomato tomato egg bacon tomato tomato lobster'
>>> s.replace('spam', 'tomato', 3)
'tomato tomato tomato egg bacon spam spam lobster'
Here’s how to use str.zfill()
:
>>> s = '42'
>>> s.zfill(5)
'00042'
>>> s.zfill(10)
'0000000042'
>>> s = '+42'
>>> s.zfill(5)
'+0042'
>>> s = '-51'
>>> s.zfill(3)
'-51'
>>> s = 'spam'
>>> s.zfill(8)
'0000spam'
00:00
This video is going to cover string formatting methods. The methods in this group modify or enhance the format of a string. To start with is the method .center()
. .center()
is going to return a string consisting of the string centered in a field of width
. By default, the padding consists of the ASCII space character (' '
). As an option, the fill argument can be specified, which it will use as the padding character instead of the space. For .center()
, let’s start with a short string.
00:32
Let’s say the word 'spam'
. It’s going to return a centered string with the length of width
, and again, it can have an optional fillchar
(fill character), so let’s start here with a width
of 10
.
00:43
You can see it spaced here. In between there are three spaces, a word, and then three spaces—ten characters all together. I’ll have you try that again with the optional fillchar
.
00:52
You could use a dash ('-'
). If a word is already shorter than the value that you put into the width
, let’s say 3
, it will return just the word unchanged. For the method .expandtabs()
, it will take any tabs entered into the string as '\t'
tab characters and it will replace them with spaces (' '
). By default, the spaces are filled assuming a tab stop is equal to every eighth column, so eight spaces each, but you can specify an alternative tap stop column using the optional tabsize
argument. For .expandtabs()
, if you have tabs within your text string—like this, 'a\tb\tc'
. Again, the escape character of '\t'
is an indication of a tab.
01:44
So, s
looks like this, but s.expandtabs()
will return a copy automatically. And again, by default, it’ll use tab stops of every eighth column, but you can override that by putting a number in, let’s say 4
, and you get tab stops of four, instead.
02:00
.ljust()
is for left-justifying a string. Like .center()
, you can enter in a value of width
that will return a string left-justified in a field of that width
. By default, the padding will be consisting of the ASCII space character again, and you can enter in an optional fill character if you desire.
02:20
Let’s say you have a string with 'spam'
in it again. .ljust()
will left-justify a string within the field, so set the width
to 10
, and you could see it returned 10-spaced left-justified string. Nice.
02:36
Again, if you want, as you can see there’s an optional fillchar
.
02:42
Let’s use that dash again. Nice. And just like before that, if the width
is shorter than the actual string itself, it will return the original string unchanged.
02:56
.rjust()
right-justifies a string in a field. Again, the width
can be specified and an optional fill character can be put instead of using spaces. So, what does .rjust()
do?
03:10 It does the same thing, just putting it on the right side, and similarly putting in a character.
03:18 And also, it behaves the same way
03:23
if you have a short string and the width
is shorter than that. .lstrip()
trims leading characters from a string. It will return a copy with any whitespace characters removed from the left end.
03:36
There’s an optional chars
argument. That argument will specify a set of characters to be removed.
03:44
Let’s say you had a string that has several spaces in the beginning, and we’ll add some at the end also. Here’s your string. So .lstrip()
will return a copy of the string with the leading whitespace removed, so everything on the left. And you can see it removed there.
04:07
In fact, let’s make a version called t
that has spaces and tabs and line returns with the escape characters.
04:23
Okay, here’s t
. So, what would happen with t
if you were to .lstrip()
it? Again, it’s going to remove all of these front characters, because each of those characters is a form of whitespace.
04:34
Now you can also specify—let’s say we create a link
and it’s 'http://ww.realpython.com'
. What if you were to take that link
and .lstrip()
it? This time, as you can see, you can specify a set of characters. I’ll specify a '/:pth'
, and there you can see the link
has been changed.
04:56
You’ve probably noticed my typo, I should have had triple 'w'
. So I’m going to reuse that and I’m going to re-enter it in, 'http://www.realpython.com'
,
05:14
and try it one more time. There we go. .rstrip()
is the opposite of .lstrip()
. It trims the trailing characters from the string, so everything off the right side.
05:26
It will return a copy of the string with any whitespace characters removed from the right end. It also has an optional chars
argument to include a specific set of characters that you want removed. For .rstrip()
, you still have the strings s
and t
. Let’s try .rstrip()
on each. So s.rstrip()
is going to return a copy of the string with all the trailing whitespace removed.
05:50
As you can see, it does that. What would it do to t
? Again, removing not only the spaces that are in there, but the tabs and the line returns. Great! And similarly, if you had a string—let’s call it x
—
06:06
and it had these odd characters in it at the end, if you were to take x.rstrip()
, as long as these are at the end and you were to put in, say, “Oh, I want to get rid of semicolons (';'
), any dollar signs ('$'
), and any periods ('.'
),” it would remove those from the right side.
06:25
The method .strip()
is essentially equivalent to invoking .lstrip()
and .rstrip()
in succession. It strips all characters from the left and right ends of a string. And again, without any character argument, it removes all leading and trailing whitespace. But as you’ve seen before, the optional chars
argument specifies what set of characters you’d like to have removed.
06:47
We have string s
, string t
. What if you were to use simply .strip()
? That will remove all leading and trailing whitespace. Great!
07:00
Okay. And what would happen to t
? Again, only the leading and trailing whitespace characters have been removed—the ones in the middle have not.
07:12
you might remember using link
before. So what if you were to use .strip()
on it and you were to remove the characters 'w'
, and '.'
, and 'moc'
? Again, since it’s not at the beginning, you wouldn’t remove the 'w'
or the '.'
there, but it would remove the 'm'
, 'o'
, 'c'
and the '.'
from the end here, on the right side.
07:33 Let’s see. What if you were to,
07:40
in this case, remove ':/pth '
and 'w'
, '.'
, and the letters 'moc'
? And you’re just left with the center of 'realpython'
. Pretty neat! .strip()
is very powerful when you carefully use the optional chars
argument.
08:01
The method .replace()
was covered briefly before. The .replace()
method replaces occurrences of a substring within a string. There’s an optional count
argument that can be specified for a maximum number of replacements. By default, it will replace all copies.
08:20
Let’s say you had a much longer 'spam'
filled string of s
and inside of it, you wanted to do .replace()
. So, what are you replacing? Well, let’s say you’re replacing this string 'spam'
and you wanted to replace it with 'tomato'
instead.
08:38
Great. But you might notice that .replace()
also has not only an old
and a new
, but a count
. So, what if you were to put the count
of 3
in there? In that case, it’s only going to replace the first three and not the last two.
08:54
So note, the default value is -1
, which actually in this case would mean replacing all of the occurrences. If the optional argument count
is given, it starts at the beginning and only those first occurrences are replaced. Nice.
09:09
The last string format method you’re going to cover is .zfill()
. .zfill()
pads a string on the left with zeros. In it, you specify width
, and it returns a copy of the string left-padded with '0'
characters up to the specified width
. If the string contains a leading sign, like a negative sign or a positive sign, it remains at the left edge of the resulting string after the zeros are inserted. For .zfill()
, let’s start with a number '42'
, and if you were to .zfill()
with 5
, again, it’s going to pad a numeric string with zeros on the left side to fill a field to the given width
. The string will not be truncated. So, there you go!
09:52
I’ve used .zfill()
many times over the last couple of years as a data analyst. It’s very handy for creating indexes. Let’s say it was 10
. Nice.
10:03 If the number you’re beginning with has a positive or negative sign at the beginning of it, that sign will be encapsulated in the length of the fill.
10:17
If you had only 3
, there’d be no zeros added in the case of '-51'
. And .zfill()
isn’t too picky. If you were to try to .zfill()
a string of alphabetic characters instead of numbers, it’ll add zeros to the front of it also.
10:35 It’s just adding up to the length, filling on the left-hand side with zeros. All right! Next up is to talk about converting between strings and lists.
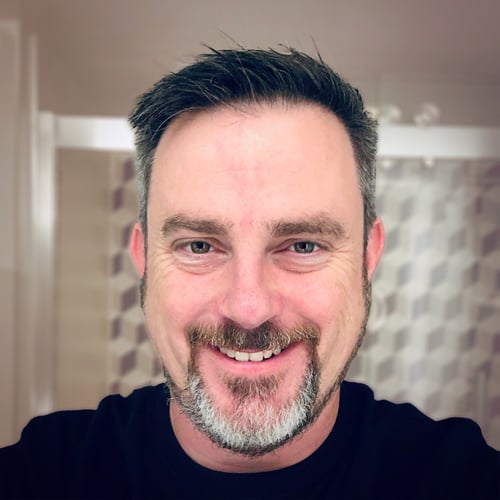
Chris Bailey RP Team on April 20, 2020
Hi @keyurratanghayra,
In your link example with link='www.python.com
the ‘p’ from python will be removed as it is one of the characters to be stripped from either side. In the example in the lesson, the link was slightly different.
link = 'http:\\www.realpython.com'
and the characters to be stripped from left and right side were defined as
link.strip(':/pth w.moc') # this would remove the colon, slash, period
# and the letters p, t, h, w, m, o, and c
# from either side.
'realpython'
The extra letters from ‘real’ stop the “stripping” on the left side as the letter “r” is not one of the characters. Where as in your example the letter “p” is one of the letters to be stripped. In fact you do not need the repeated “t” and “/” as only one instance needs to specified for it to strip the 2 t's and 2 /'s
. I made a slight mistake in my wording during the lesson, saying it was going to remove the “path”, but what I should have said is it is going to remove those specific characters (/:pth
) and I could have put them in a different order, like (p:t/h
).
kiran on July 23, 2020
can you provide any best resource to know more about(deep dive) for strip() in python.
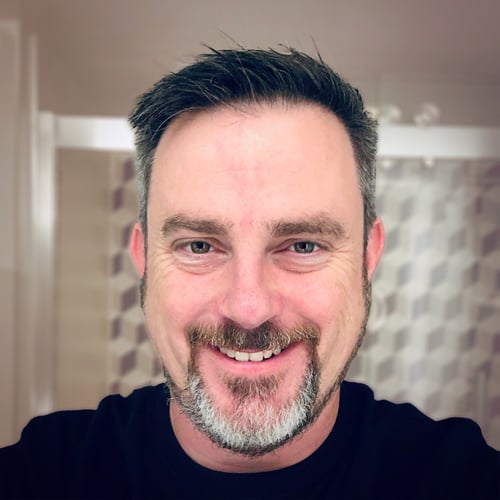
Chris Bailey RP Team on July 23, 2020
Hi @manupanduworld, I don’t know of a specific deep dive, but I will give you some resources that I think may help you.
The first is the article this course is based on.
The second is the Python3 documentation for .strip()
The third is a proposal for the new version of Python, Python 3.9 that adds new methods that work more specifically on removing text from the beginning or ending of a string. Which has been an area some students get stuck on. It is PEP 616 - and it calls for adding 2 methods named, .removeprefix()
and removesuffix()
. Here is an excerpt from the PEP (Python Enhancement Proposal).
The main opportunity for user confusion will be the conflation of lstrip/rstrip with removeprefix/removesuffix. It may therefore be helpful to emphasize (as the documentation will) the following differences between the methods:
(l/r)strip: The argument is interpreted as a character set. The characters are repeatedly removed from the appropriate end of the string. remove(prefix/suffix): The argument is interpreted as an unbroken substring. Only at most one copy of the prefix/suffix is removed.
I hope this helps to explain it a bit further.
Alain Rouleau on July 29, 2020
I like the zfill()
method. As you mentioned, very handy for indexes. Good for sorting properly as well. You know, ‘07’, ‘08’, ‘10’ as opposed to ‘10’, ‘7’, ‘8’. Will be using that one more often. I knew about it before but completely forgot, so, thanks.
marciolazaijunior on Aug. 17, 2020
Hello, can you tell me which IDE are you using?
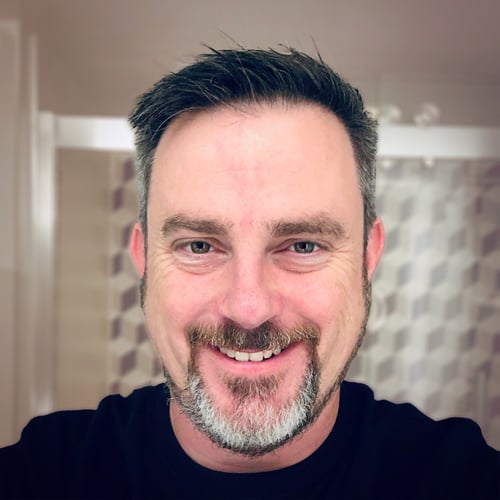
Chris Bailey RP Team on Aug. 18, 2020
Hi @Marciolazaijunior, I’m using VSCode. I have a color theme installed called Dainty - Material Theme Ocean. I use a separate tool for interactions in the REPL called bPython. You can find more information and links at the bottom of the 2nd lesson in this course.
subhrass2017 on April 2, 2022
link = "http://www.realpython.com"
When I use this: link.lstrip('/:pt')
It returns: 'http://www.realpython.com'
, without deleting '/,:,p,t'
But if I use this : link.lstrip('/:pth')
it returns www.realpython.com
Why there is a difference in output?
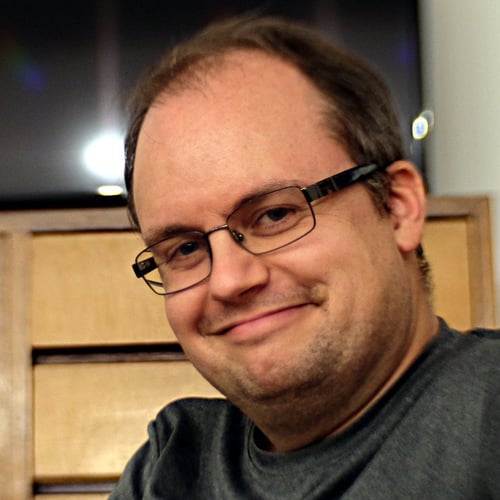
Geir Arne Hjelle RP Team on April 2, 2022
Hi subhrass2017,
.lstrip()
deletes characters from the left side of the string. The h
that is first in http://www.realpython.com
“protects” the other characters since it’s not one of the characters '/:pt'
. Your second example includes h
so there all characters until r
are stripped.
realpython.com/python39-new-features/#string-prefix-and-suffix talks a bit about .strip()
and friends, as well as a few similar methods.
Become a Member to join the conversation.
keyurratanghayra on April 20, 2020
Thanks for this wonderful tutorial.
While I tried string stripping with the following example:
The output was
ython
and notpython
.