Python's "with open() as" Pattern
In this lesson, you’ll learn about using the with
statement. For more on this, check out Context Managers and Python’s with
Statement as either a video course or a written tutorial.
00:00
In this lesson, I’m going to cover Python’s with open() as
pattern, otherwise known as the context manager pattern, which I think is the most important basic pattern for working with files in Python, because it’s what allows you to create and read from existing files in your filesystem.
00:18
In order to understand the with open() as
pattern, you first need to understand the open()
function, which is really quite simple.
00:26
It takes in a file_name
and a mode
and it opens the given filename—from the current directory unless otherwise specified—in the mode that you pass in.
00:36
And then once that file is open, that’s when you can do operations on that file, like write to it or read from it. So the basic file modes—and there are many more, but these are the three that I’ll show you to start with—are write mode, 'w'
, which wipes the existing file content, creating the file if it doesn’t already exist, and then allows you to write to the file.
00:55
There’s read mode, 'r'
mode, which gives you read-only access to the file’s contents, and it will throw an error if the file doesn’t exist already.
01:03
And then there is append mode, 'a'
, which writes to the end of the file, so it does not wipe the existing file content. So, those are the three basic file modes that I’ll explore throughout the rest of this lesson.
01:15
In this lesson, I’m going to use an incredibly simple sample directory. It’s just a directory called test
that’s completely empty, because I’m going to be the one creating all of these files in the Python REPL.
01:27
So let’s head over to that Python REPL. At the moment I’m in the bare terminal, just because I want to show you that, first, I’m in the test
directory, as I said I would be, and that, second, this has nothing in it, because I just executed the Bash command ls
and, as you can see, there’s no output.
01:46
And now I’m in the Python REPL, and we can change that ls
output now to have an actual text file in it. So, if you remember from the slide a second ago, what I can do is I can say something like 'test.txt'
as the file name and then I’ll open it in write mode so that I can write to it, and so that it’s created, because test.txt
does not yet exist.
02:10
And then I need to say as
, so, with open() as
, and then I give this a name. Normally I find it convenient to say something like write_file
, so that I remember what exactly it is that I’m writing to, in this case.
02:23
Then I can say something like write_file.write()
and I can just write whatever text I want to write. So, param s
(param string) of type AnyStr
(any string).
02:32
I can say something like, "Hello, I'm a file!"
And when you exit out of this, it won’t be quite obvious that anything in particular has happened, but it has been written to and you can check that by saying with open('test.txt')
in read mode as read_file
, and then I can just say print(read_file.read())
, and the .read()
function just returns all of the content of that file.
03:03
So as you can see, this is a really existing file and it does have the content "Hello, I'm a file!"
There’s one further mode that I showed you, so I’ll show you now, "test.txt"
in append mode as write_file
, and then I can say write_file.write()
,
03:23
and I’ll put a newline here so that there’s a newline in the file. And I’ll say "Here's some more text"
.
03:32 And then again, I’ll just do the exact same thing that I did here. And as you can see, I’ve appended to it. But if I were to open it again in write mode
03:48
"All the text has been deleted"
.
03:54 And you can see that this is, in fact, true because the write mode actually just writes over everything that exists in the file already, whereas append mode just appends to the existing text.
04:05
So, that’s how you can open, and read from, and write to a file in a couple of different ways. One last thing that I want to cover in this lesson is why this with open() as
pattern is important, because you can say write_file = open('test.txt')
in write mode, and then you can say write_file.write("Original text")
,
04:33
and that will actually return to you—the write_file.write()
function returns the number of characters written there. So you can say—you can do—this, but the problem is that then if you don’t say write_file.close()
, then you will be leaving this file resource open, which your operating system will interpret as needing to have that file open, and it will kind of snarl up your operating system resources.
04:59
So it’s best practice to always close the file after you open it, but this context manager with open() as read_file
and so on, this actually takes care of all that for you.
05:10
It opens it and then it closes it at the end in this block, so anything that happens in this context-managed block will automatically be wrapped with this write_file.close()
.
05:20 That may not seem that important but, really, when you’re building large applications, it’s incredibly important to manage your resources carefully. The context manager syntax allows you to do that really quickly and easily, and you don’t have to ever worry about, “Oh, did I close it here? Do I need to close it there?” Anything that’s in this block is already wrapped by that.
05:40
So, that’s how you can use the with open() as
pattern to create, and read from, and write to files. In the next lesson, I’ll cover how to get a directory listing: how to look at all of the things that are in your directory that have already been created.
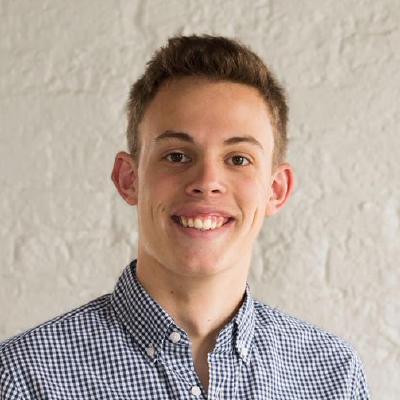
Liam Pulsifer RP Team on July 27, 2021
Hi @Gopinath Y! Thanks for watching the course. Would you mind clarifying what you expect to happen here? The .write
method of a file object writes the input string into the file, then returns the number of characters written. Your terminal is just showing you the return value of the method call. Is there something else you’re hoping to do here?
Gopinath Y on July 28, 2021
Thank you sir, (I’m a BEGINNER). I want to know that in your python REPL:
- How did you do that
...
the prompt not showing return value? - How did you change the three dots into prompt?
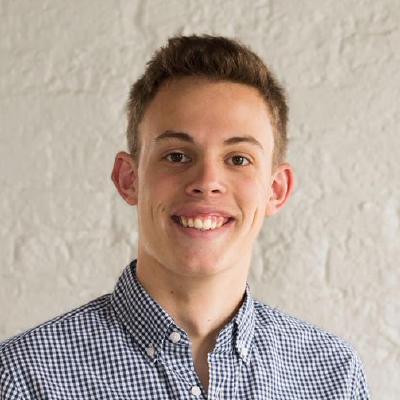
Liam Pulsifer RP Team on Aug. 12, 2021
Ah, I see. That’s just a setting on the REPL I use (ptpython, in this video).
johnsonbass17 on Nov. 9, 2021
Hi~I’m a beginner, at 02:00 of the video .
Is that mean that will creat a text.txt file under the test directory?
Become a Member to join the conversation.
Gopinath Y on July 27, 2021
Hi, even when i use…
my terminal returns number of characters … why?