Flask by Example
Learning Path ⋅ Skills: Web Development, Flask Framework
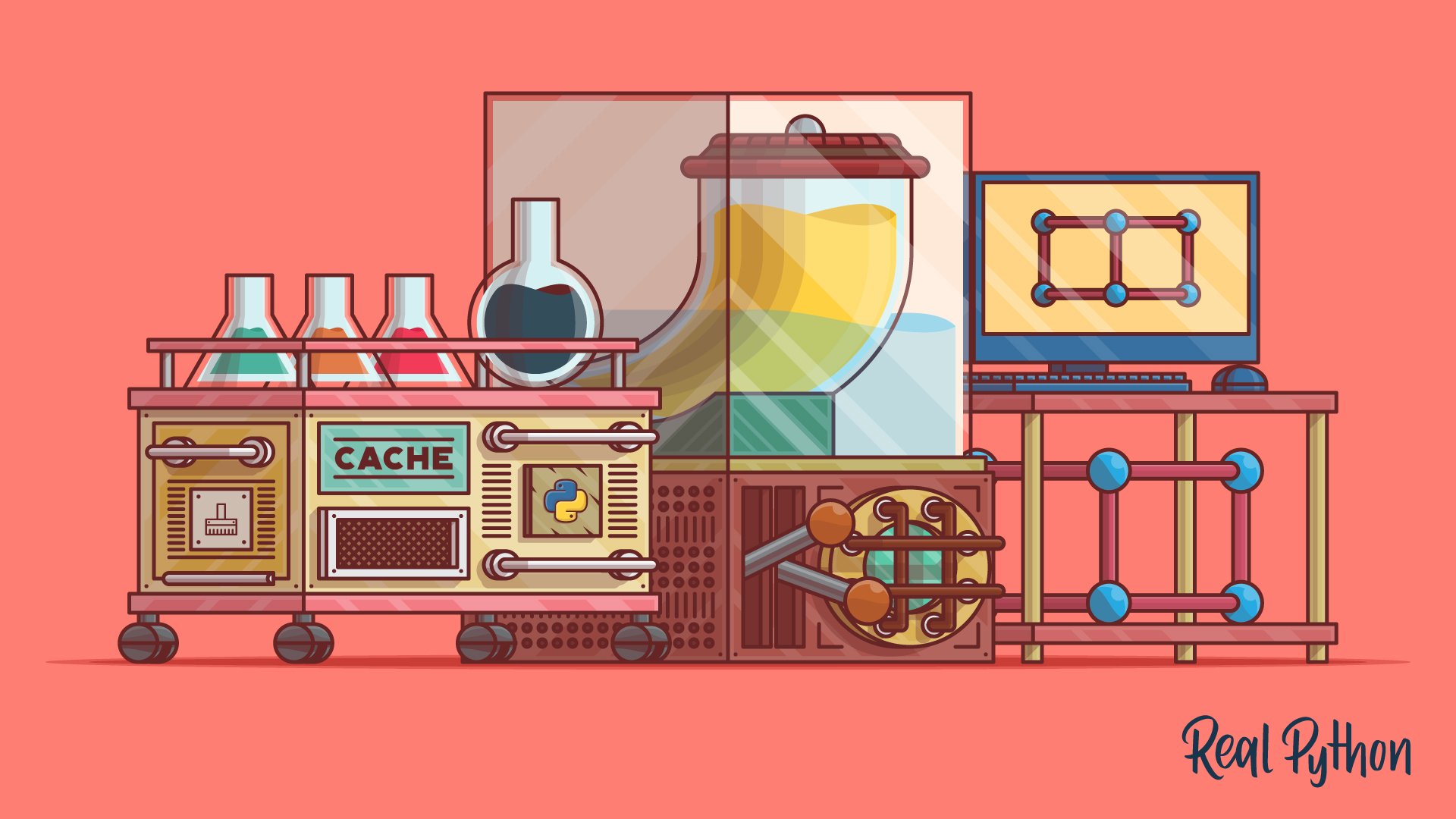
In this learning path, you’ll get started with Flask. Flask is a powerful and flexible micro web framework for Python, ideal for both small and large web projects. It provides a straightforward way to get a web application up and running, with all the features that you need to get started.
This learning path is a great starting point if you’re interested in web development with Python.
Flask by Example
Learning Path ⋅ 12 Resources
Laying the Foundation for Web Development
Before you jump into web development with Flask, it’s important to brush up on some foundational skills, like understanding HTML, CSS and Jinja templating.
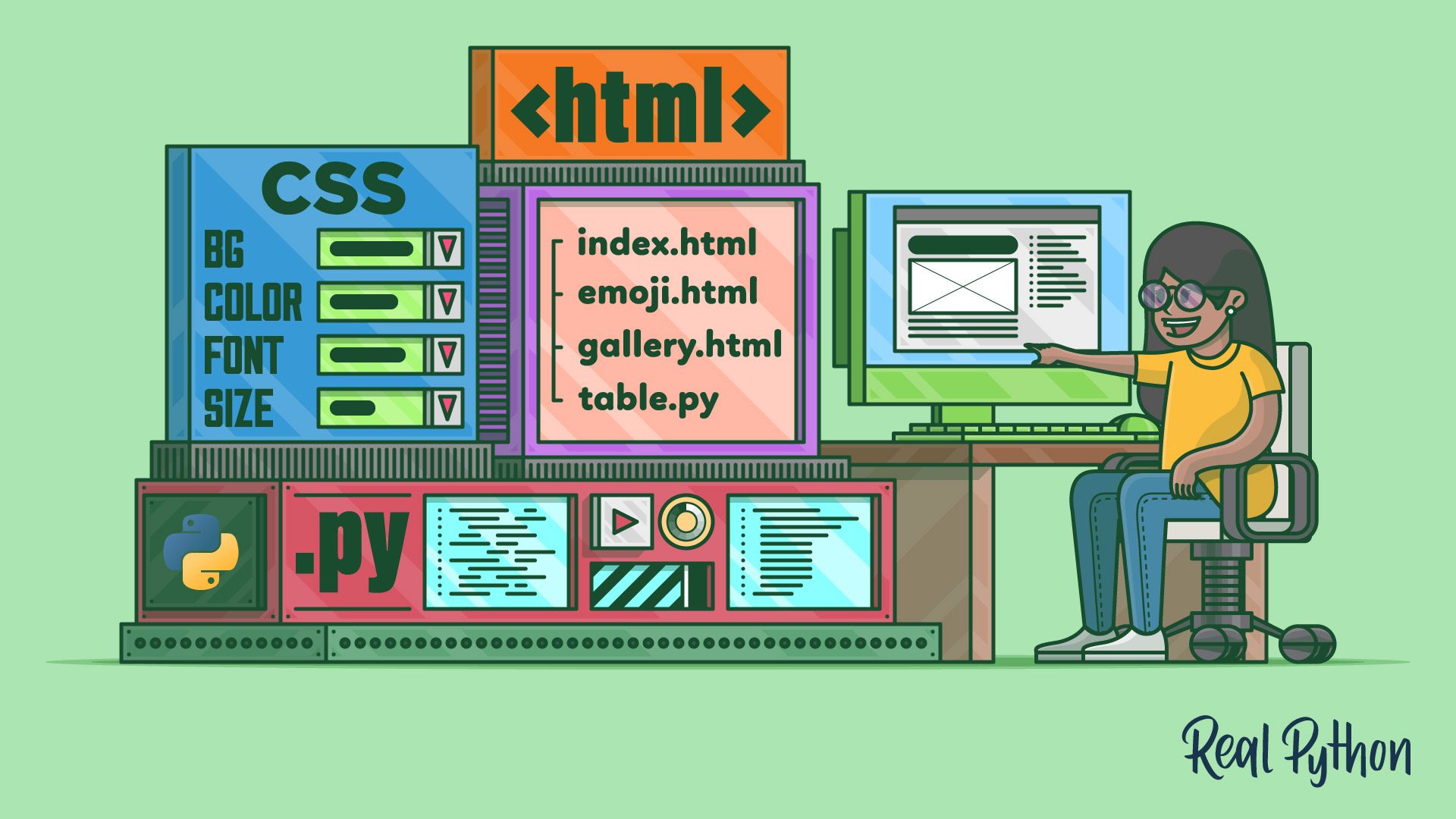
Course
HTML and CSS Foundations for Python Developers
There's no way around HTML and CSS when you want to build web apps. Even if you're not aiming to become a web developer, knowing the basics of HTML and CSS will help you understand the Web better. In this video course, you'll get an introduction to HTML and CSS for Python programmers.
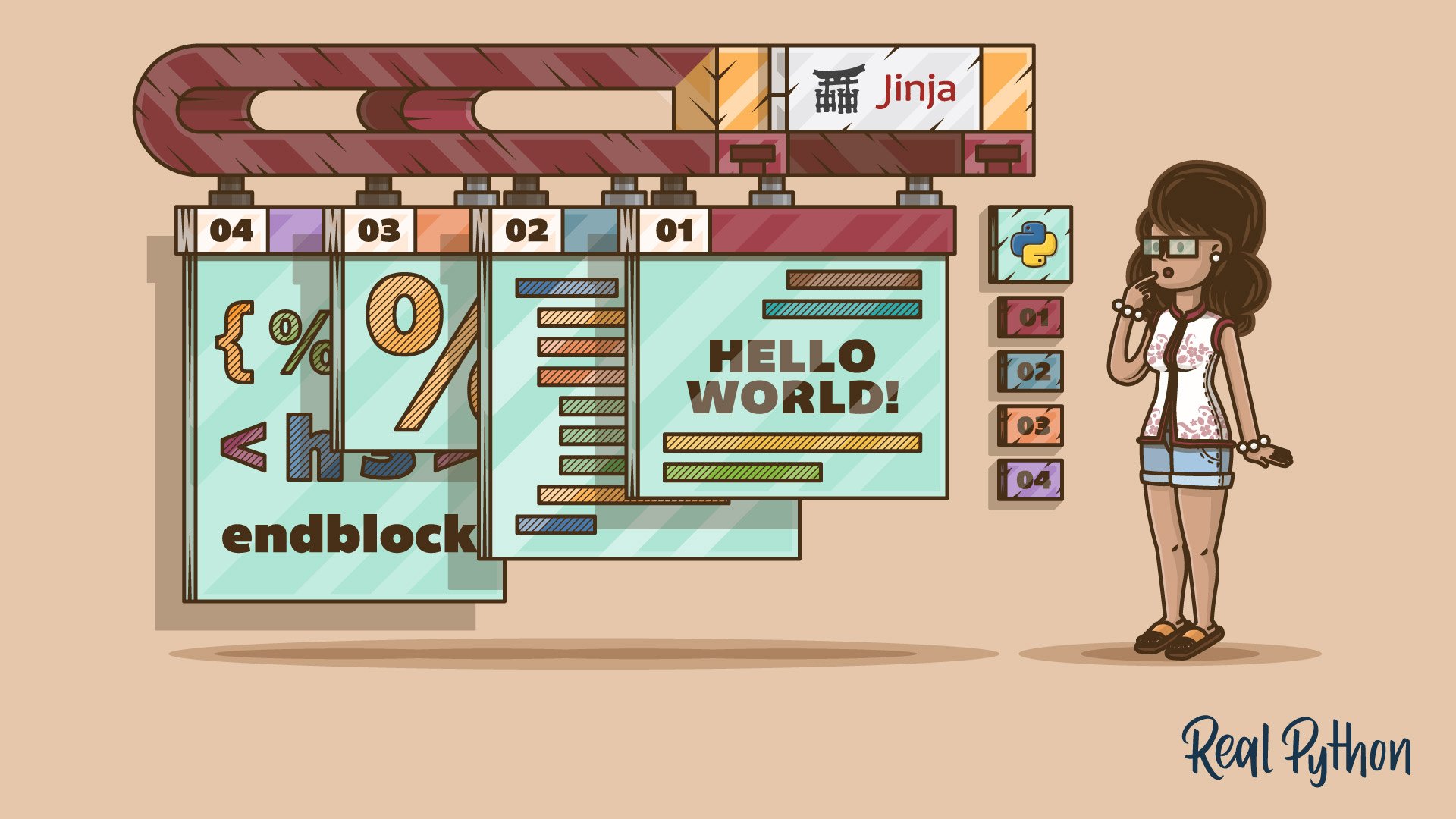
Course
Jinja Templating
With Jinja, you can build rich templates that power the front end of your web applications. But you can use Jinja without a web framework running in the background. Anytime you want to create text files with programmatic content, Jinja can help you out.
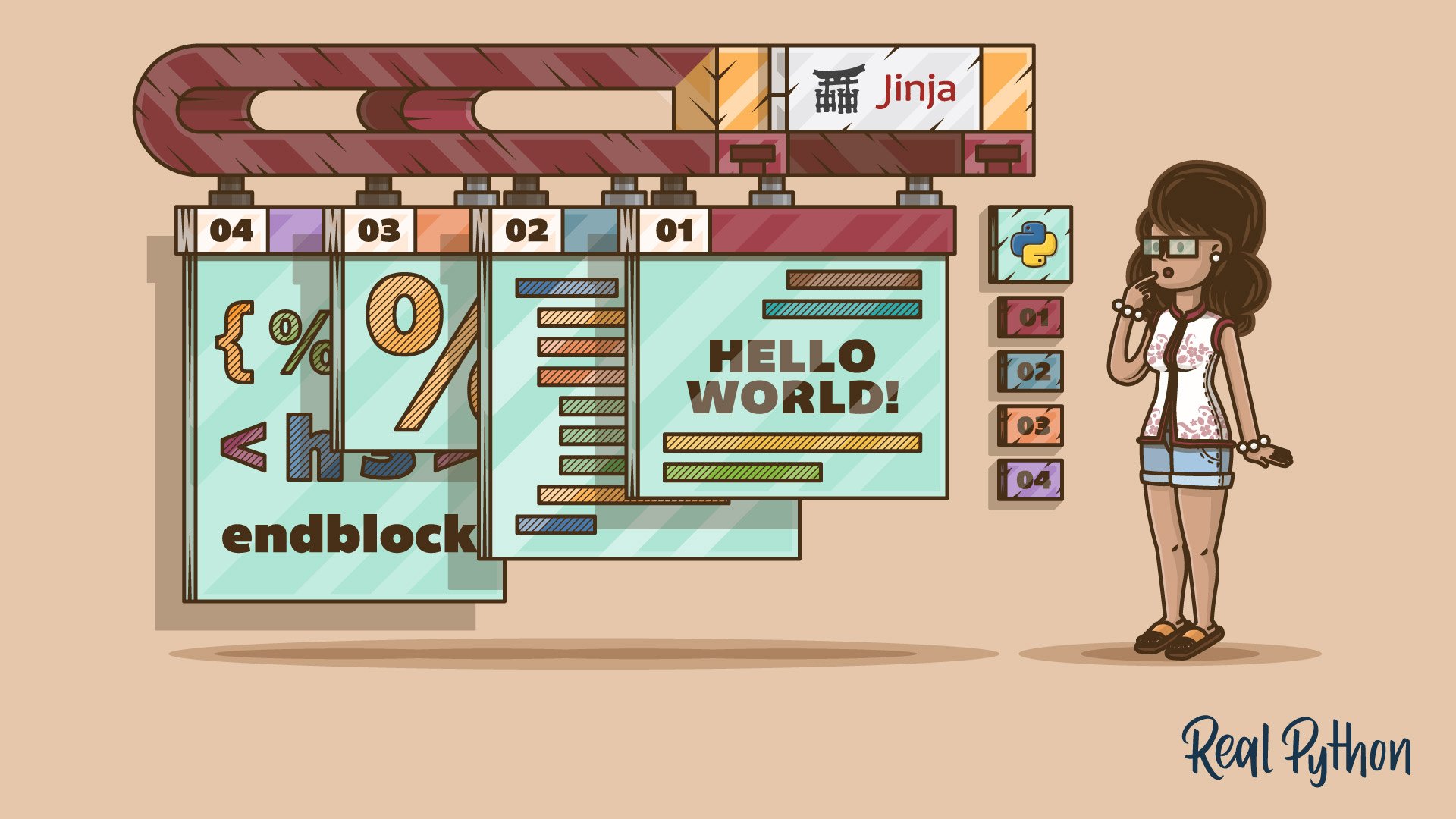
Interactive Quiz
Primer on Jinja Templating
Getting Started With Flask
Now that you’ve learned some foundational skills, you’re ready to start creating your first Flask project!
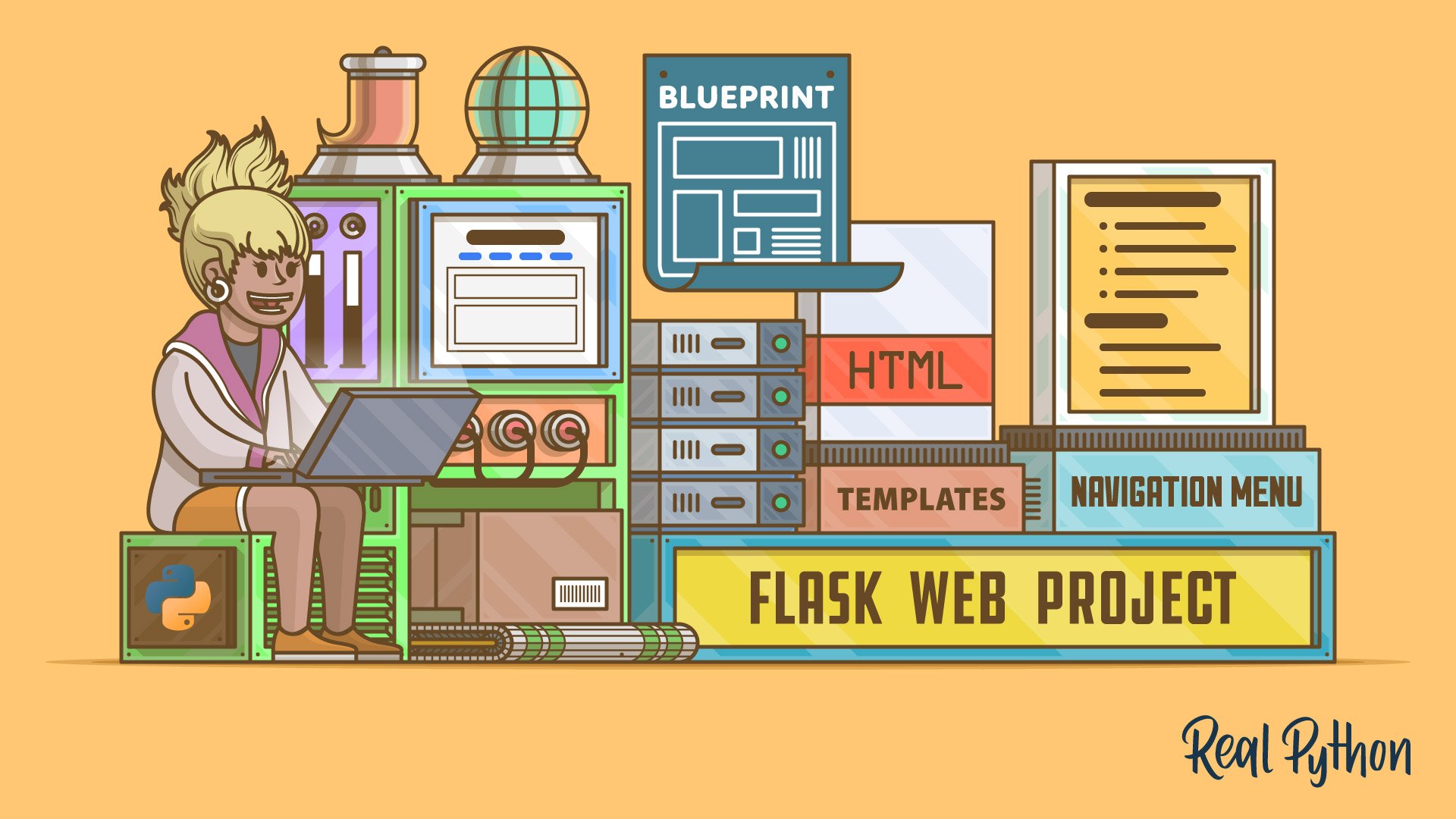
Tutorial
Build a Scalable Flask Web Project From Scratch
In this tutorial, you'll explore the process of creating a boilerplate for a Flask web project. It's a great starting point for any scalable Flask web app that you wish to develop in the future, from basic web pages to complex web applications.
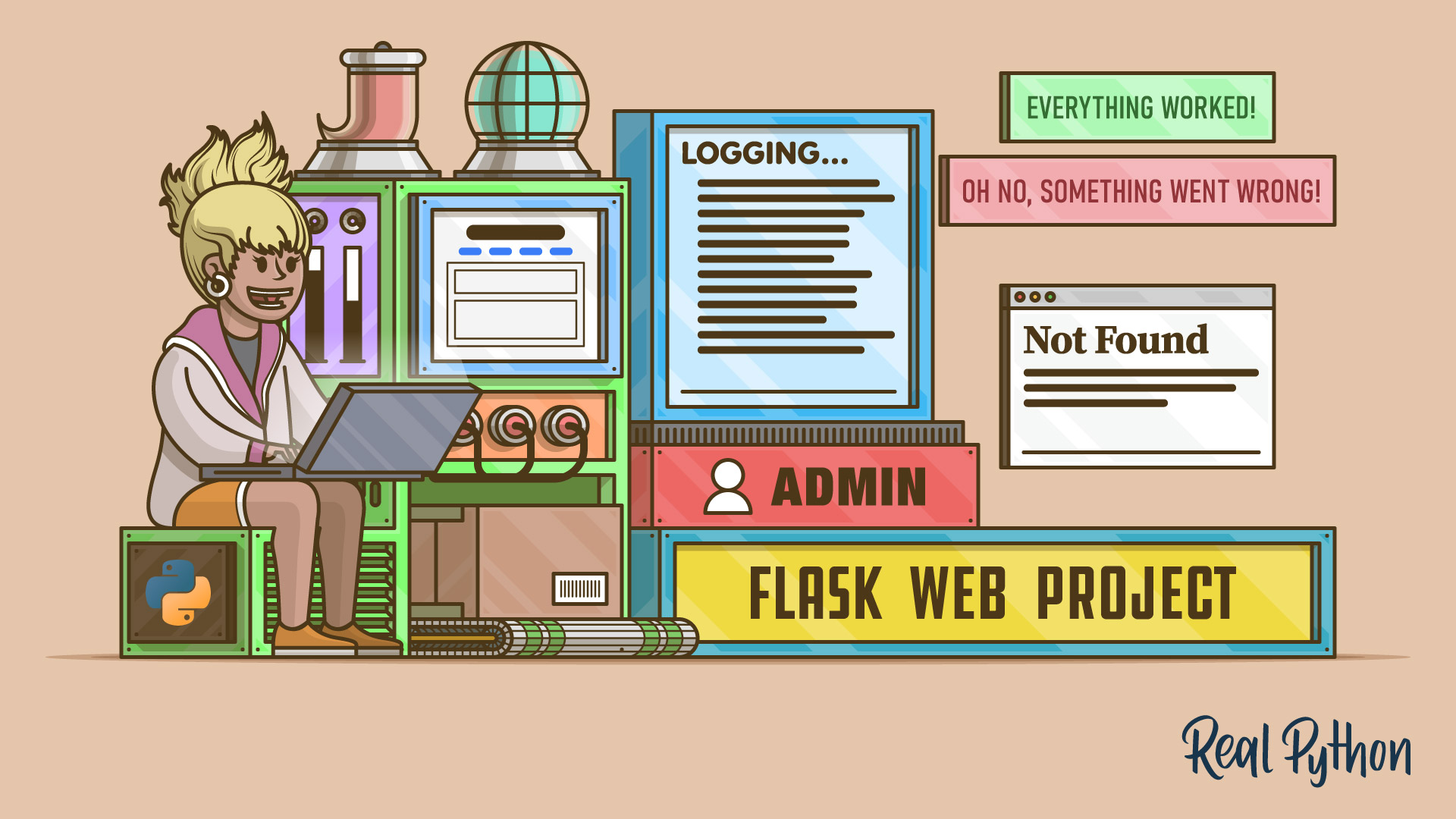
Tutorial
Add Logging and Notification Messages to Flask Web Projects
After you implement the main functionality of a web project, it's good to understand how your users interact with your app and where they may run into errors. In this tutorial, you'll enhance your Flask project by creating error pages and logging messages.
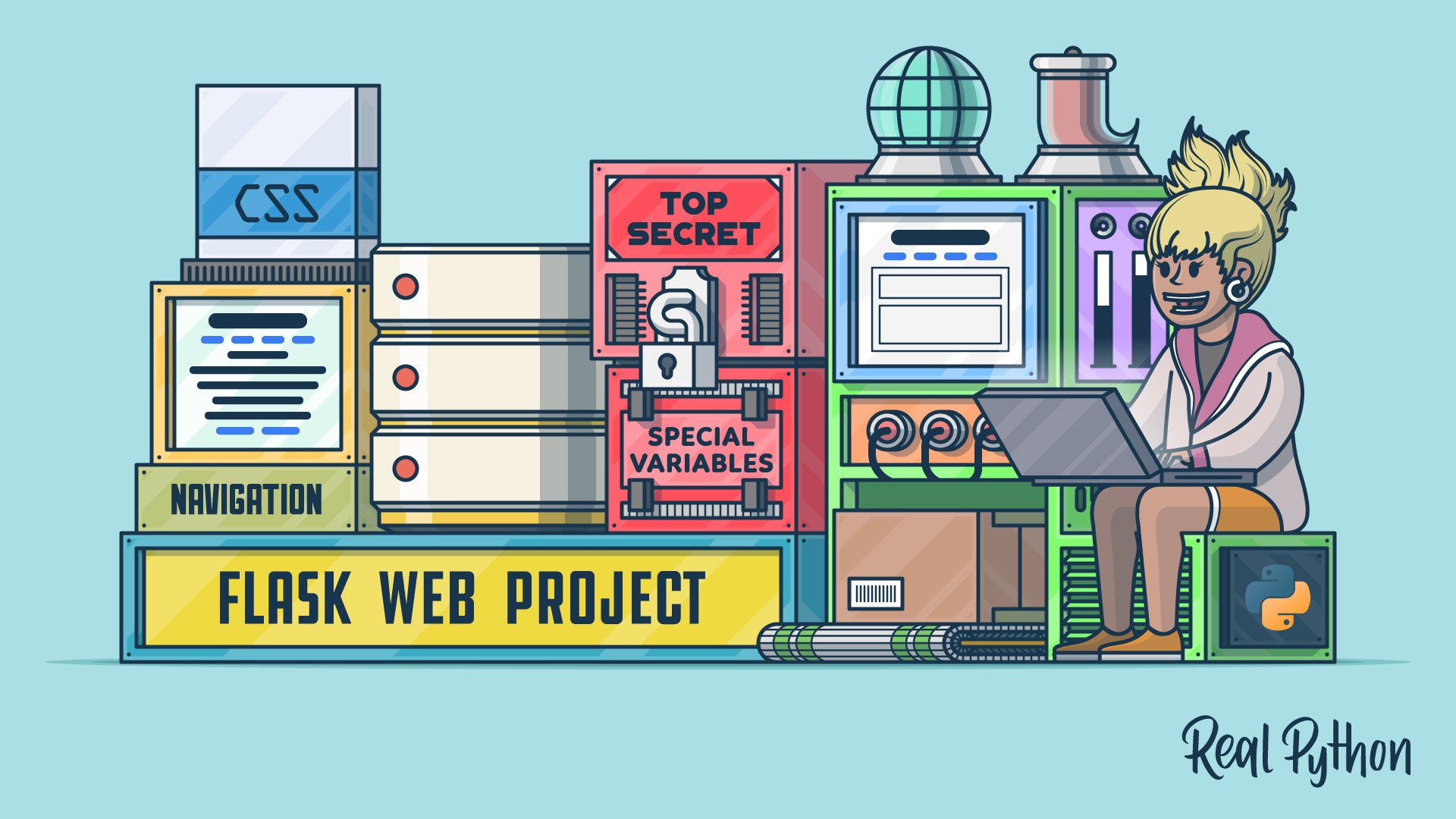
Tutorial
Enhance Your Flask Web Project With a Database
Adding a database to your Flask project elevates your web app to the next level. In this tutorial, you'll learn how to connect your Flask app to a database and how to receive and store posts from users.
Building a REST API
The lightweight nature of Flask makes the framework a valid option for building a RESTful API. While working through a multi-part project, you’ll explore HTTP requests and databases.
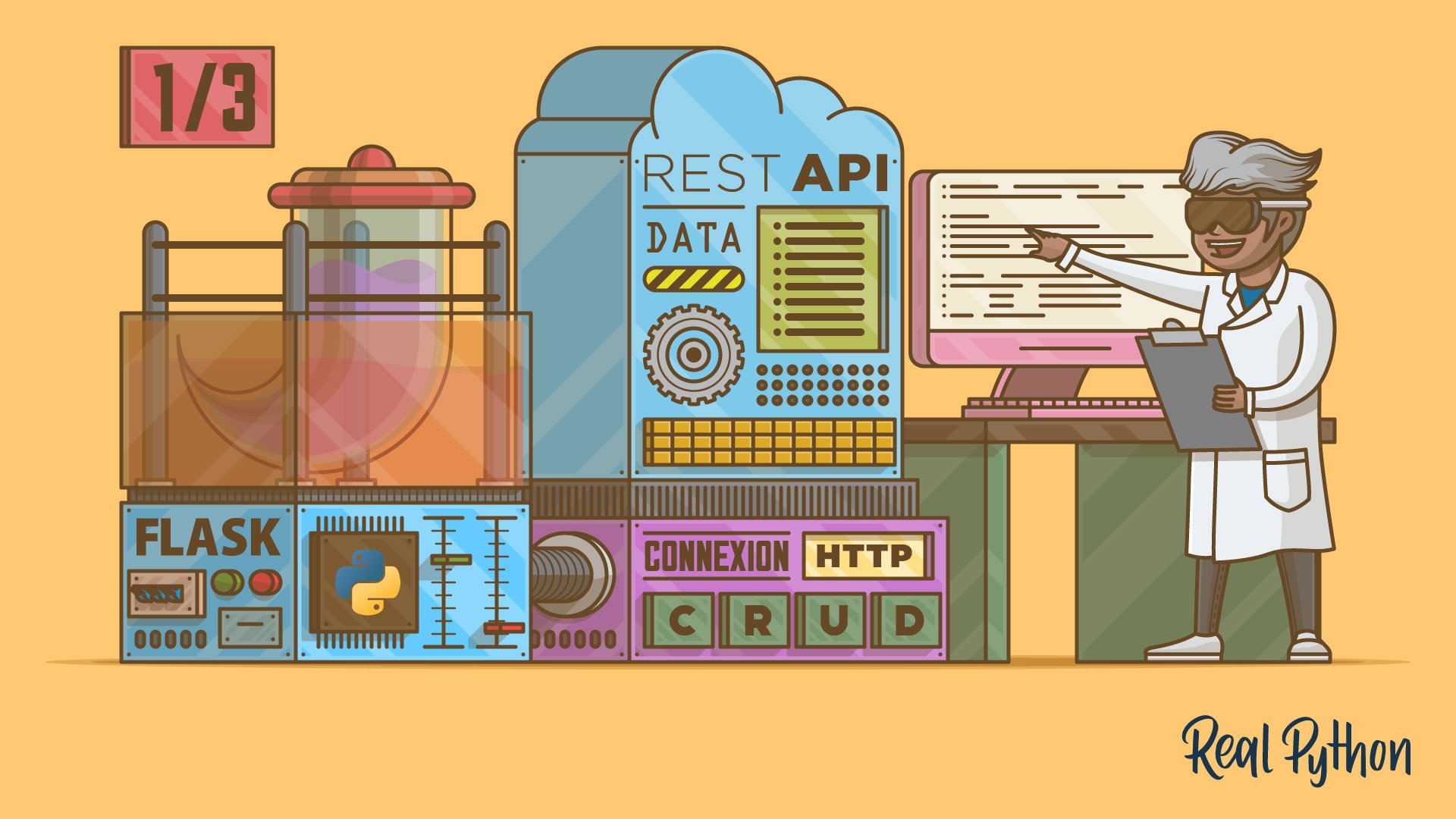
Tutorial
Python REST APIs With Flask, Connexion, and SQLAlchemy – Part 1
In this three-part tutorial series, you'll create a RESTful API from scratch to keep track of people and notes using the Flask web framework. You'll also test your API with Swagger UI API documentation. In part one, you'll build the foundation of your note-keeping app.
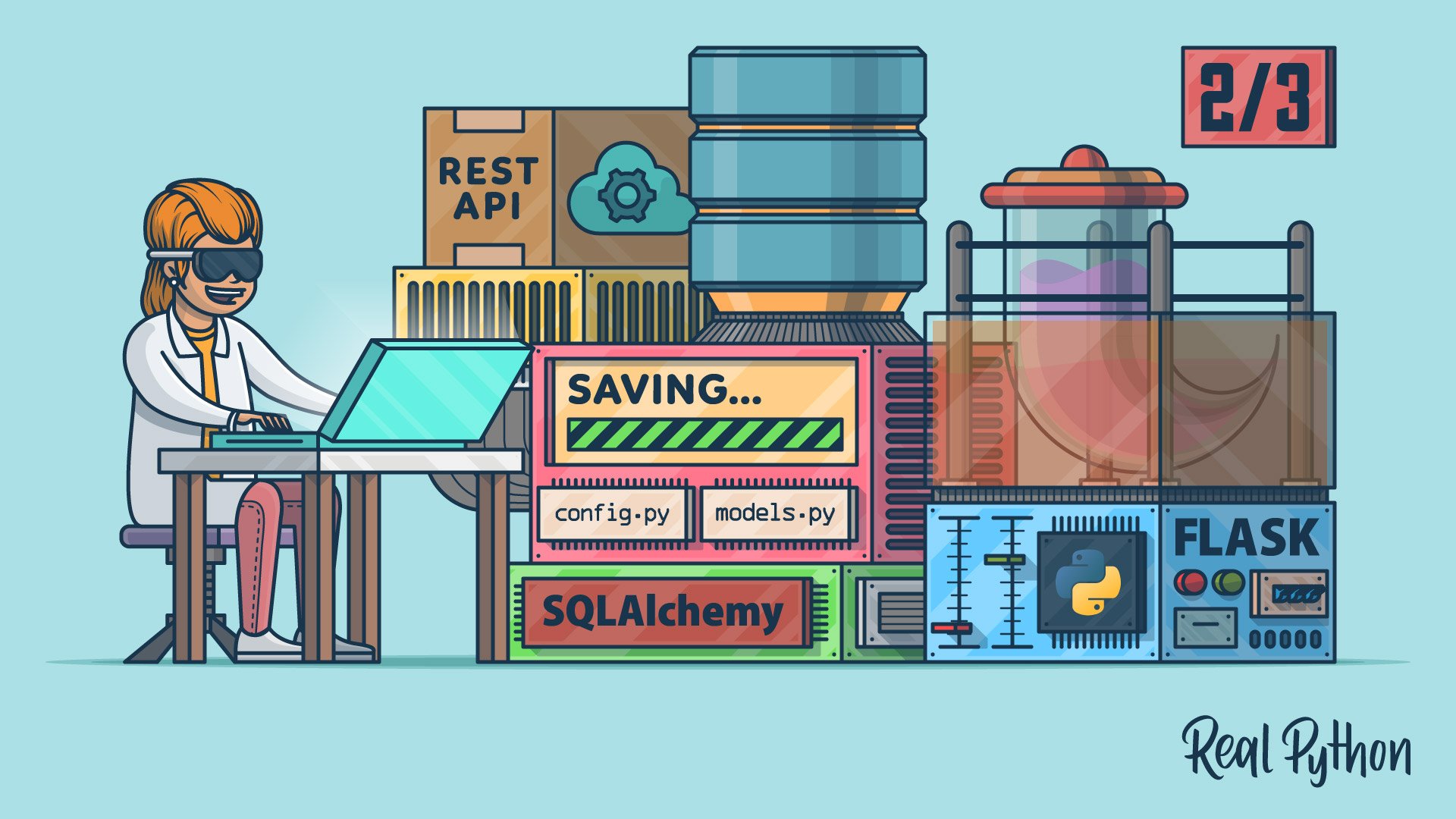
Tutorial
Python REST APIs With Flask, Connexion, and SQLAlchemy – Part 2
In part two of the series, you'll implement a SQLite database to permanently store the data of your note-keeping app.
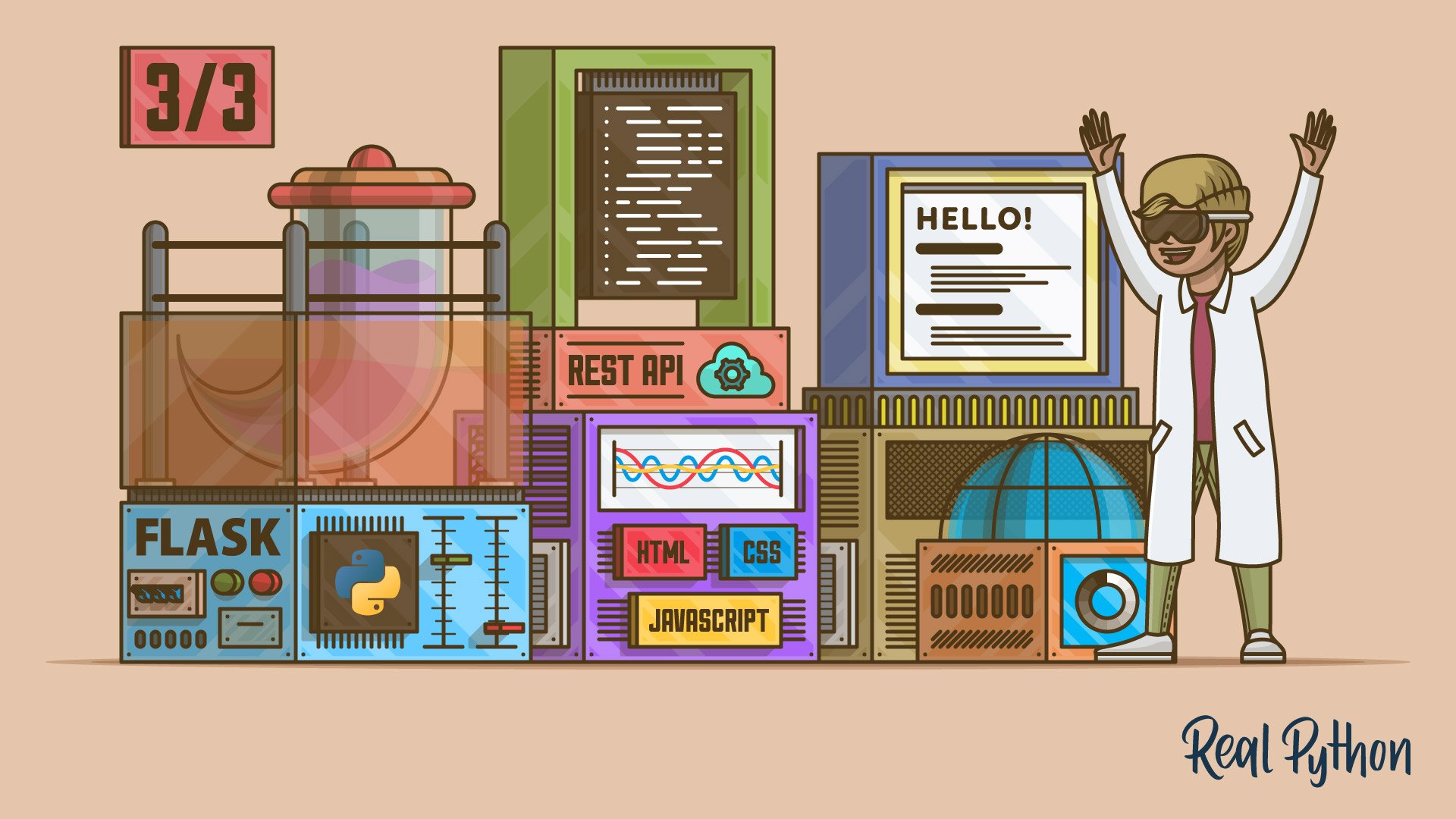
Tutorial
Python REST APIs With Flask, Connexion, and SQLAlchemy – Part 3
In part three, you'll use SQLAlchemy to provide the functionality to add notes to a person in your Flask note-keeping app.
Working on the Frontend
The frontend of your web application is the part that your users interact with. You already learned about HTML and CSS–now it’s time to put JavaScript into the mix.
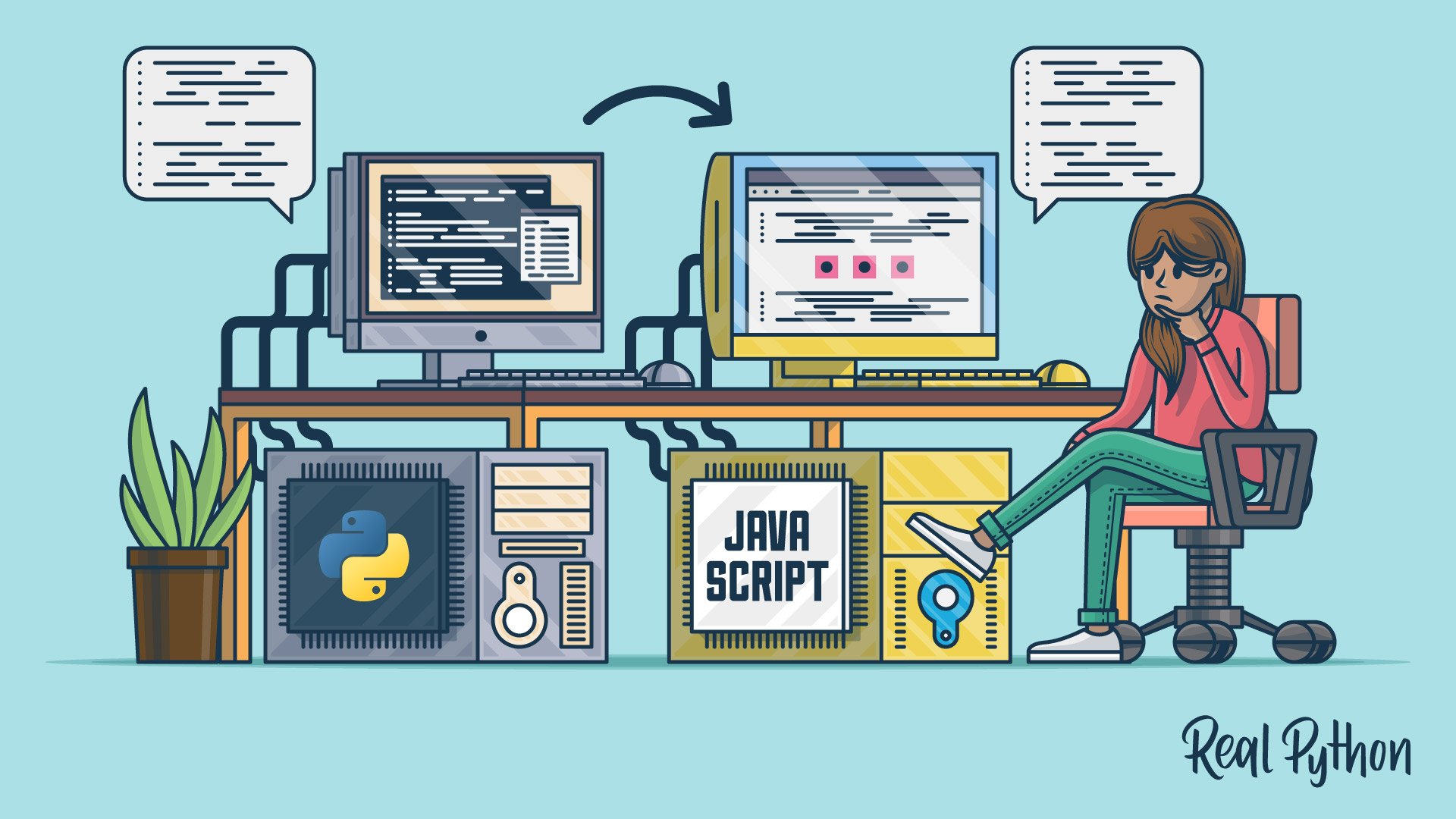
Course
Python vs JavaScript for Python Developers
Python and JavaScript are two of the most popular programming languages in the world. In this course, you'll take a deep dive into the JavaScript ecosystem by comparing Python vs JavaScript. You'll learn the jargon, language history, and best practices from a Pythonista's perspective.
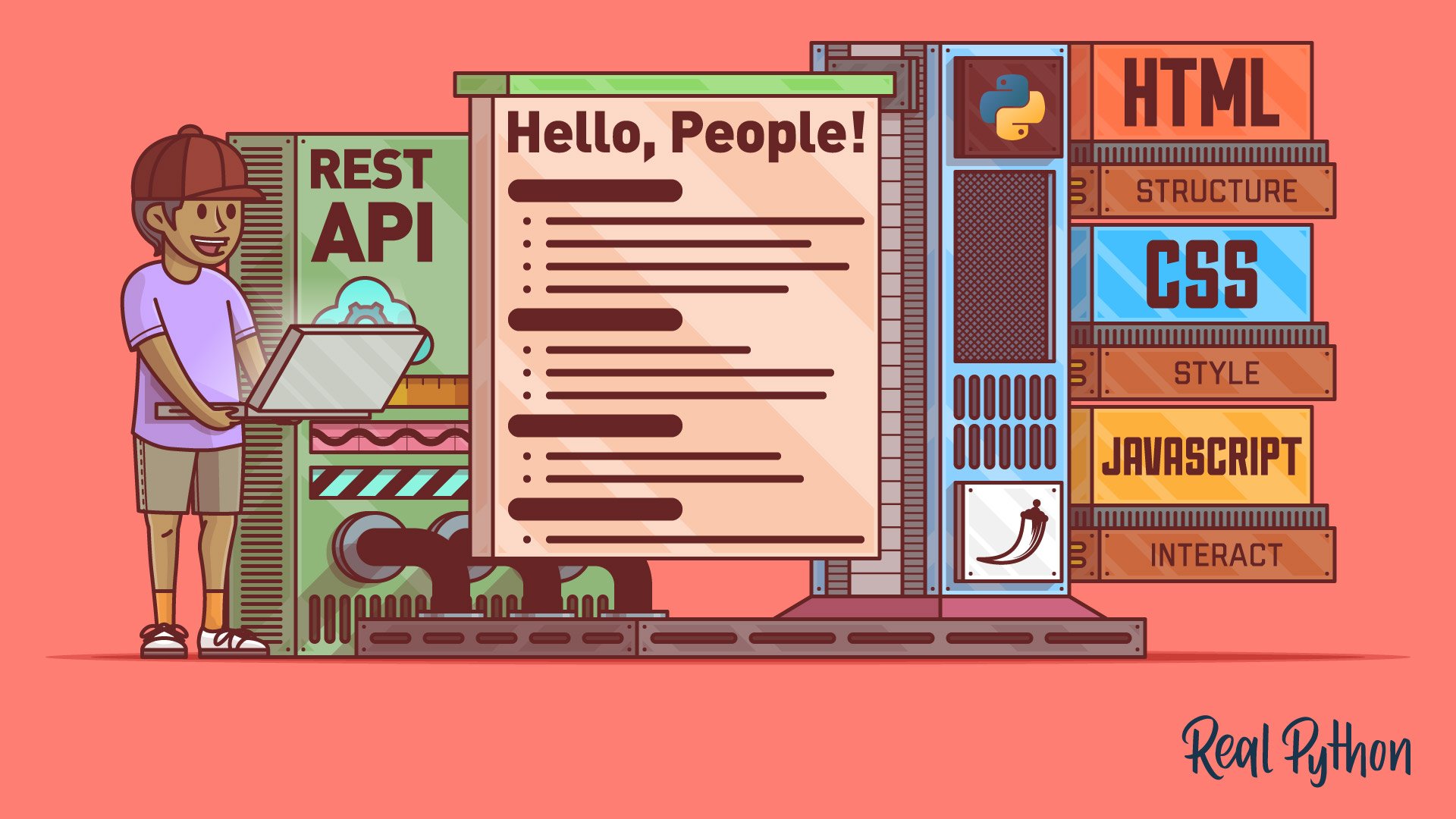
Tutorial
Build a JavaScript Front End for a Flask API
Most modern websites are powered by a REST API. That way, you can separate the front-end code from the back-end logic, and users can interact with the interface dynamically. In this step-by-step tutorial, you'll learn how to build a single-page Flask web application with HTML, CSS, and JavaScript.
Deploying Your Projects
You must put your Flask projects online to make them available to everyone. This process is called deployment, and it’s the cherry on top of working with Flask.
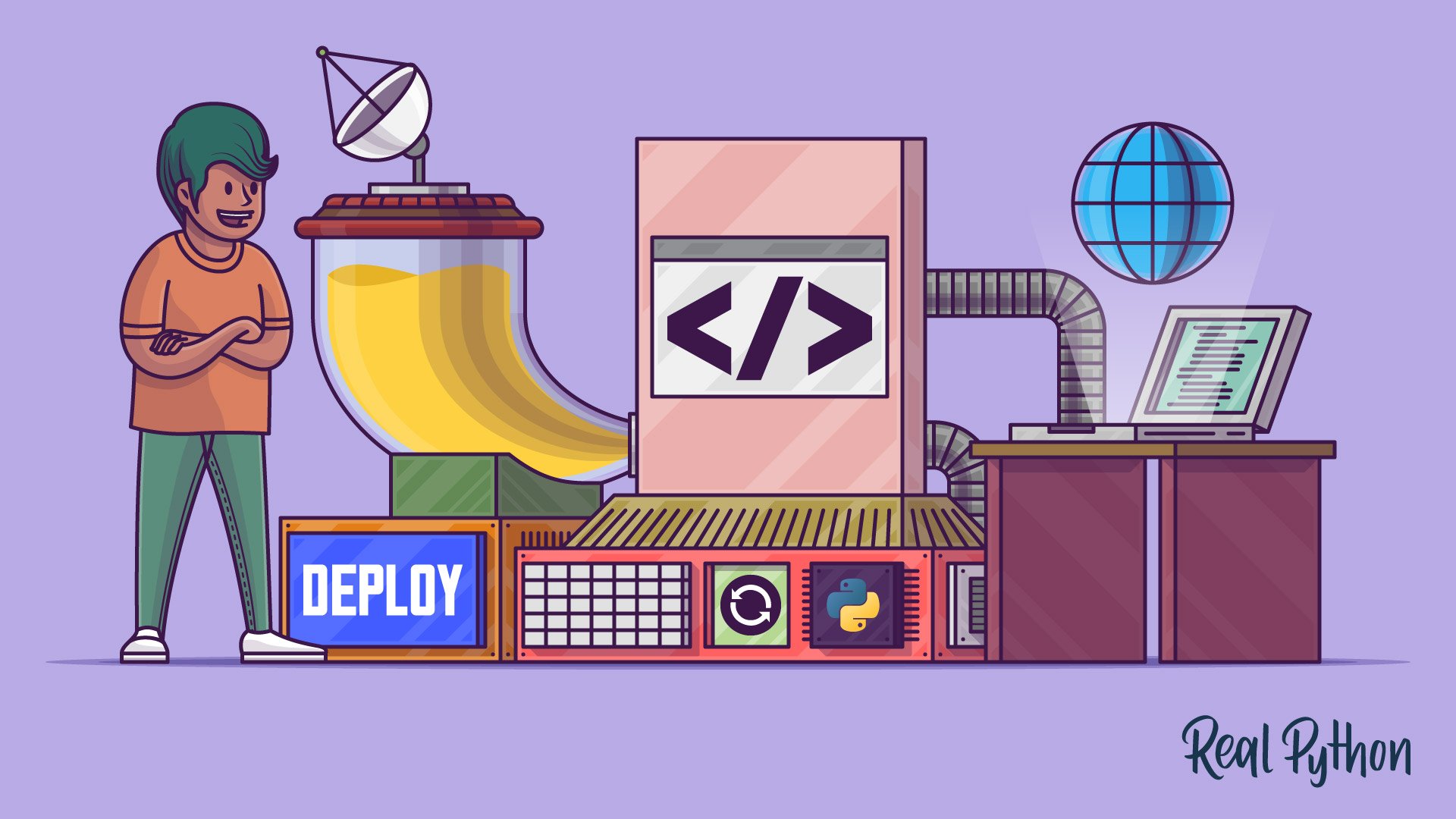
Course
Deploy Your Python Script on the Web With Flask
In this course, you’ll learn how to go from a local Python script to a fully deployed Flask web application that you can share with the world.
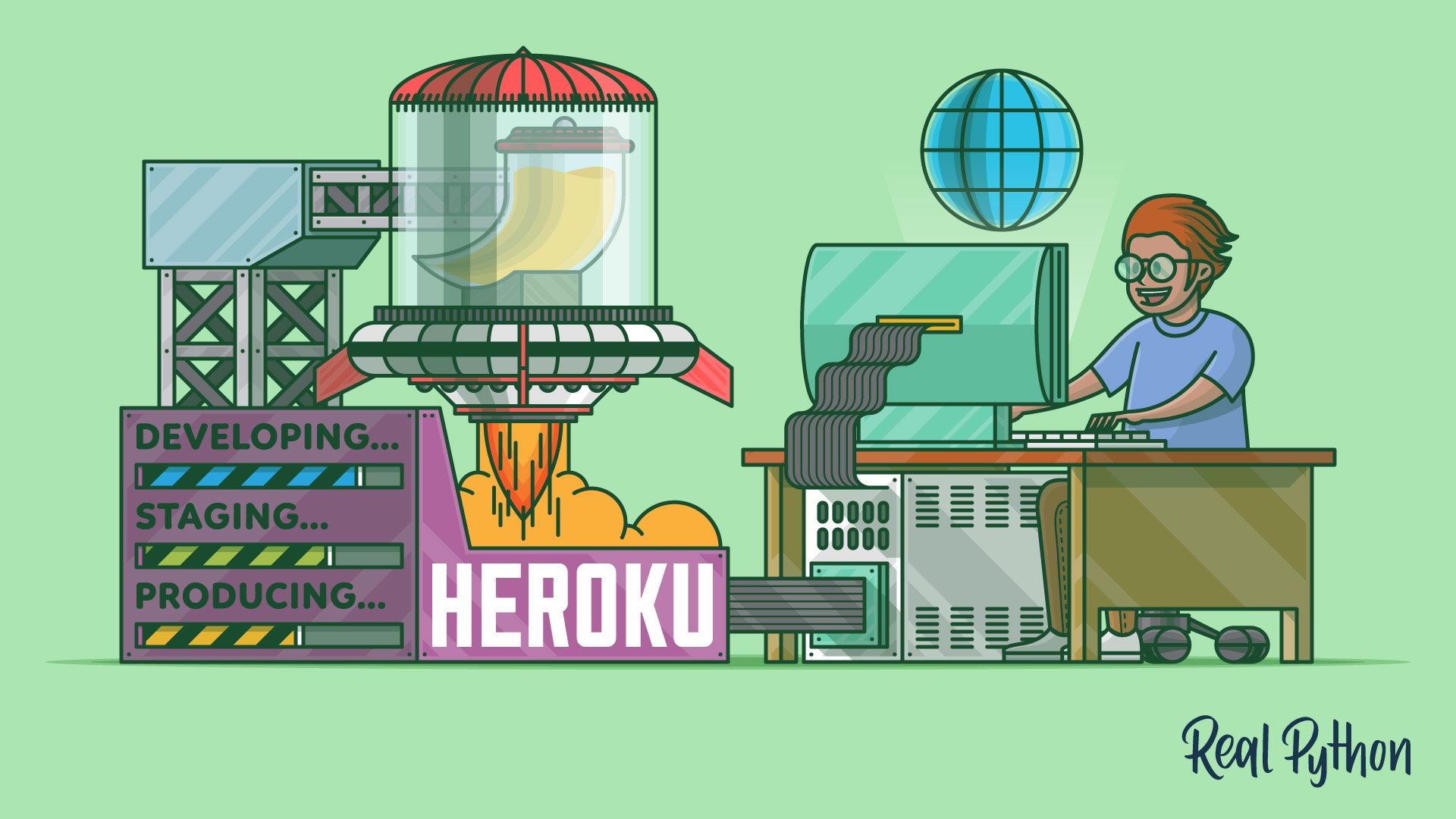
Course
Deploying a Flask Application Using Heroku
Learn how to create a Python Flask example web application and deploy it using Heroku. You’ll also use Git to track changes to the code, and you’ll configure a deployment workflow with different environments for staging and production.
Congratulations on completing this learning path! If you’d like to continue to develop your web development skills, then check out the web development topic on Real Python.
Or maybe you’d like to explore different ways to interact with the web. In that case, these learning paths have got you covered:
Got feedback on this learning path?
Looking for real-time conversation? Visit the Real Python Community Chat or join the next “Office Hours” Live Q&A Session. Happy Pythoning!