Break Out of the Pattern (Solution)
00:00
Here we are back in IDLE in a script. I made a new script and put in a short comment just to keep the context for me what I’m supposed to do here. I’m going to run an infinite loop until they use a types "q"
or "Q"
.
00:13
while True
is a good way to do an infinite loop, and then to get out of it, I’m going to need something and then a break
keyword at some point. All right, so the task is that I want to collect user input.
00:27
So I’m going to say user_input =
again with the input()
function, and I can put in a little message here.
00:37
So what am I going to tell the user? I’m going to tell them that if they want to get out of this, that they need to type either "q"
or "Q"
.
00:43
So let’s just be explicit and tell them that: "Type
00:48
'q' or 'Q' to quit: "
. That gives them the instructions. And then I need to compare their input. So I’m going to say if user_input ==
"q"
. I need to make sure that it’s both lowercase and uppercase. In this case, I’m going to just say .lower()
, use the string method on it to say if the user input that comes in, lowercased, is equals to a lowercase "q"
, that would catch both lowercase and uppercase "Q"
inputs from the user, then I want to break out of the loop.
01:25
So this is where I’m going to use the break
keyword now, and make sure to not forget the colon. And this needs to be nested under your conditional statement.
01:34
So here, you’re using a conditional statement and a Boolean comparator, and then you use the break
keyword to break out of the infinite loop, the loop that’s otherwise infinite.
01:45
Users can keep inputting anything until they put in either lowercase or uppercase "Q"
. Only then, the program’s going to break out of the loop and end in this case.
01:54 So, let’s try it out. I’ll save and run it.
02:05
And over here, you see the input comes up, "Type 'Q' or 'q' to quit: "
. So, let’s try something else first. You can see the infinite loop in action.
02:13
I can type in anything, and it just keeps asking me until I type either an uppercase Q
—well, there was a couple of spaces in the Q
, so if it’s just a Q
, then this should get me out of there.
02:25
And then same should work with a lowercase q
. There we are. It takes me back out. Great. So this is working,
02:35
and here’s one of the possible solutions to this challenge. The code again that I just wrote. In this case, we’re doing .upper()
and comparing to an uppercase "Q"
. So both of these work.
02:45
When I wrote it out, I wrote .lower()
and compared it to a lowercase "q"
, but otherwise the code is the same.
02:53 Now just for fun, because I keep saying that there’s always different solutions for it, and also trying to show that to to you. Yeah, you don’t have to worry about if you get a different solution, as long as it has the same functionality, right?
03:04
So I want to show you something here that’s another way of handling this. Python 3.8 introduced something called an assignment expression. So I could take this and instead of using while True
, I’m going to say while user_input
and then use the assignment expression, which is also dubbed the walrus operator (:=
) because it’s this little sign looks a little like a walrus with its eyes and tusks. All right?
03:30 And I’m wrapping this into some parentheses, so
03:35
I’m just making this a little smaller so it’s easier to read. "type: "
.
03:40
So here I’m going to say while this .lower() != "q":
03:54
And then the funny thing is I don’t need anything in here. In this while
loop, I’ve moved the whole logic into just the first line of code, and then I’m using an ellipsis here, which is similar to pass
. Well, actually, maybe this is better.
04:08
I just need something down here so that the loop doesn’t produce a SyntaxError
, but I’m actually putting the whole logic into this first line.
04:16
Let’s try this out. If I run this code, I should have the same functionality as before. So it tells me "type:"
. I can type anything, and it keeps
04:28
working, right? And if I type Q
, then it quits. And the same should work with a lowercase q
. Let’s run it again.
04:42
What happened here? Okay, we’re back to normal, and the lower, just the lowercase q
quits the program. So, this is a strange and much less readable alternative solution that uses the pattern 3.8 walrus operator, or assignment expression.
05:00
Let’s unpack this. So what’s happening here is you’re collecting the user input just as before. And then in this case, you are assigning it to user_input
right here.
05:10
And then this whole thing that you wrapped inside of parentheses, you’re lowercasing it and then comparing it to the lowercase "q"
, and it’s saying if it’s not equal to "q"
.
05:22
So essentially, this whole thing is going to be True
as long as the user doesn’t input "q"
, and it keeps evaluating in here. And so you’re essentially creating this while True
loop with a very, very not well-readable line of code up here, but it’s going to evaluate every round, and once the user inputs a "q"
, this whole expression is going to be false, and the loop is going to stop.
05:48 So you don’t even need any code in the loop body for this solution. Okay? But this is just a weird sidenote, and I don’t expect you to read or write this type of code because it’s much less readable than the other solution.
06:03 The most important thing in writing code is that it’s readable and understandable for other humans.
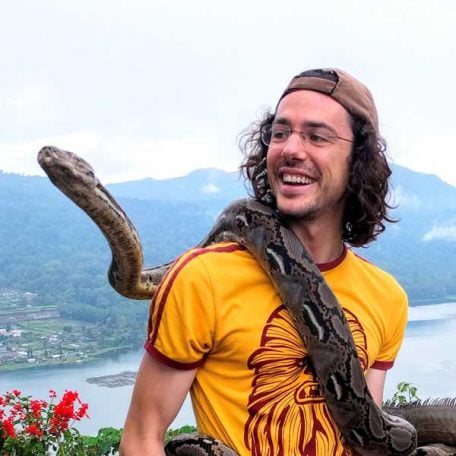
Martin Breuss RP Team on Dec. 6, 2023
@Learner nice, this works! However, your else
block isn’t necessary because a while True
loop will continue to loop anyways, so you only need to define the break
condition.
Anonymous on Feb. 14, 2024
Here is my version, albeit a little bit long, instead of the break, i decided to make the exit condition as a boolean expression for the while loop
exit_prompts = ('q', 'Q')
message = 'You are trapped, find a way to exit!'
tries = 1
max_tries = 6
print(f'{message}\nYou have {max_tries} tries, use it wisely!')
while (answer := input('>>> ') not in exit_prompts):
max_tries -= 1
if tries < 3:
print('\nnu-huh, keep trying!\tTries left:', max_tries)
elif tries < 6:
print('\nYou can do it!\tTries left:', max_tries)
else:
print('\nThe secret untrap me prompt was \'q\' or \'Q\'')
print('You are now untrapped :)')
break
tries += 1
else:
print('GOOD JOB! Here is a cookie :D')
ajackson54 on July 1, 2024
Here is my code.
letter_added = True
while letter_added == True:
user_input = input("Enter a letter: ")
if user_input != "Q" and user_input != "q":
letter_added == True
else:
letter_added == False
break
I learned a few things from this exercise. First, I originally had letter_added == True
in which I got a name undefined
error. Then I had
if user_input != "Q" or "q"
instead of above, and it didn’t the program prompted for a letter even "q"
and "Q"
.
ajackson54 on July 1, 2024
I don’t know how my code was formatted this way – this is not the way it looked when I posted it. Sorry.
ajackson54 on July 1, 2024
I tried to enter the code again, manually, but I got the same format as above. What am I doing wrong?
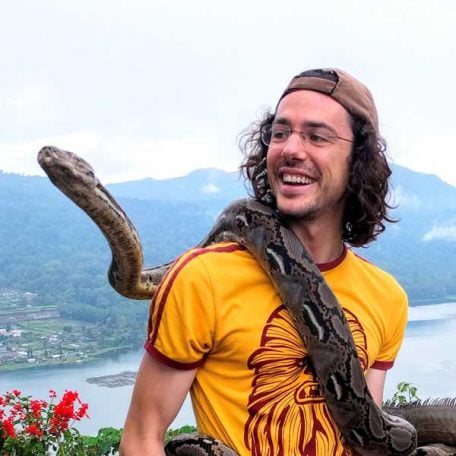
Martin Breuss RP Team on July 2, 2024
Hi @ajackson54, I edited your code formatting so that it looks like you intended. You can create such fenced code blocks using backticks, and you can even add syntax highlighting.
As for your code solution, I think it’s mostly a well readable solution that makes use of a flag via the letter_added
variable. I’d still suggest to slim it down a bit. You don’t need to continue setting letter_added = True
in the case that the user didn’t choose the exit condition. In that case, it’s still True
!
letter_added = True
while letter_added == True:
user_input = input("Enter a letter: ")
if user_input == "Q" or user_input == "q":
break
I also changed your comparison operators from !=
to ==
, and your boolean logic from and
to or
. Do these changes make sense to you? Why do you think I applied them?
ajackson54 on July 2, 2024
Yes, your code is a lot cleaner. Also, I don’t really need the “==True” after letter_added. As for changing from != to ==, I would guess it would be faster in the loop.
Become a Member to join the conversation.
Learner on Nov. 25, 2023
Here’s my version of this exercise: