Simulate Events (Solution)
00:00
So, write a function that simulates the role of a die, and there’s some randomness involved. So you’re going to use the Python random
module.
00:08
I’m going to import random
. Then I should write a function. So, I’m going to say def roll()
because I think this is what we said it should be called. Doesn’t really need to take any input, but what it does is it should return a number from 1
to 6
.
00:24
I’m just going to say return random.randint(1, 6)
The start
and the stop
are both included in the possible results, so I can just say 1
to 6
, and that is already the solution.
00:39
Let’s give it a go. Run it. Well, okay, I guess I’ve got to print something too. I need to first call the function and then also print something. So I will print()
a call to roll.
00:54 Let’s do a couple of them to see whether the randomness works.
01:00
So, we’ll save this, run it, and we get 4
, 2
, 2
, 4
, 5
. So, these are possible outcomes of rolling the dice one, two, three, four, five times.
01:10
Great. A slightly different way of doing this is you could, instead of importing the whole random
module, we could say from random import
just the randint
function, and then you could cut it out here and just return a call to randint()
. Exactly the same otherwise.
01:27
So, let’s run it again. No, InvalidSyntax
from fron
, or fron
is not exactly the same. from
. There we are.
01:39
5
, 3
, 5
, 6
, 1
, and 4
. Looks like our die is fair. Yippee! Okay. And you practice importing randomness and then also defining function, which are both things that you’re going to need for the upcoming challenge.
01:57
Here, you’ve got the solution again, and I’ve also added a little docstring to the function that just describes what this function does. from random import
randint
and then define the roll()
function.
02:08
And then you’re essentially just returning a random number between 1
and 6
. All right, that’s it for the review exercises. Let’s do a quick recap and then afterwards move on to the challenges.
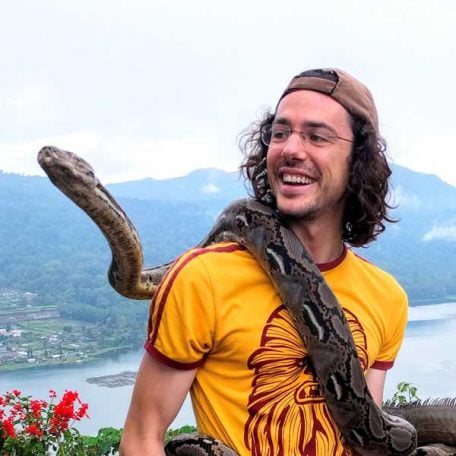
Martin Breuss RP Team on Dec. 22, 2023
@Joon that’s a fun idea for a lesson to introduce random.seed()
:D I’d encourage learners who read this to give it a try and post your seeds that make the dye roll in order below :)
We don’t go very deeply into using the random
module in the Python Basics learning path, but for learners who want more, you can check out the tutorial on random number generation.
Anonymous on Feb. 14, 2024
After looking through the random module came across the choices method, after playing around with it, decided to implement it.
from time import sleep
from random import choices
dice_probability = (1.05, 1.05, 1.05, 1.05, 0.95, 0.90)
def roll():
return choices(list(range(1, 7)), weights=dice_probability, k=1)[0]
print('Rolling die', end='')
for i in range(3):
print('.', end='')
sleep(1)
else:
print()
print(f'Dice has revealed itself:')
input('Press Enter to summon thee!')
dice_roll = roll()
print('TADA -> ', dice_roll)
print('\nBTW - Never trust a digital dice :)')
Become a Member to join the conversation.
Joon on Dec. 20, 2023
Hi, maybe it’s a bit too soon, but this exercise would be a good opportunity to introduce how random.seed works in a simple way. For example at the end of the exercise you could show the learner how to find a seed value that allows them to verify that their function
roll()
correctly returns all 6 values.Something like:
or similar.
Obviously for such a simple function like
roll()
it’s not very useful to userandom.seed()
to debug as long as you read the documentation ofrandom.randint()
(or remember the lesson introducing it), but the idea would just be to introduce whatrandom.seed()
does and how it can be useful in a simple way. Or maybe explained separately as a short single video in the lessons?That said maybe you already introduce
random.seed()
in an equally simple way later on in the path ^^, if so, disregard that, it’s just something I thought about since you chose to introducerandom.randint()
very early.Thank you for your work.
P.S. I know that at this point of the path the lists have not been introduced yet but the same thing could be shown with 6 variables or something.