Control the Flow of Your Program (Solution)
00:00 Here you’re back in an IDLE window. Now I have on the left side the interactive window, and on the right side, I have a script file open that is currently not saved yet, but just this is where we’re going to start building the program and then eventually run it to see its output.
00:14 A good way for me to start is always take a note of what the task is, maybe rephrase it a little in my own words, or just copy/paste the task description here.
00:22 Now I’m going to try to rephrase it a bit. So the idea is that I want to display whether the length of a user input
00:32 is smaller than, bigger than, or equal to five characters. This is the overall task. And what I need to start off with doing is I need to collect some user input.
00:48
Let’s do that first. You can do this using the input()
function. input()
, collect it, and then I need to assign that to a variable as well.
00:56
I’m going to call this string_to_check
,
01:02
input()
, and then I can also give a little descriptive message here so the user knows what they’re supposed to do. "Check string length: "
.
01:11
This is going to collect the user input and save it to the variable string_to_check
. Now I can go ahead and do something with this variable.
01:21 I want to do three things with it. I want to use these three different Boolean comparators to figure out how the length of the string compares to the number five.
01:30
We have the len()
function. I can pass in string_to_check
to it, and then I get as an output how long that string is. And then I just need to compare it.
01:44
I could either calculate this every time I do the check, or I could do it once for this script. Now I’m going to just do it once, as I’m going to say string_length =
len(string_to_check)
. And you could also wrap this around the input and then right away just get the length of the string.
02:03 But I want to keep the string around because maybe I want to display it back to the user or something. So now I have two variables. I have the string variable that the user inputted, and then I also have the length variable, how long it is.
02:16
Now I can do my control flow using if
and elif
statements. I’m going to say if string_length < 5:
I want to print a descriptive message, and the task was very specific about it, so I am just going to copy it from the task so I don’t have to type it out.
02:37
I’m going to print()
this. That just says "Your input is less than 5 characters long."
02:45
Let me move these windows a bit, get some more space. So here’s the first conditional check. I’m checking whether the string length is smaller than five, and I’m going to print it’s less than five characters long. And then I want to know elif string_length > 5:
then I want to print different string.
03:06
I’m going to say essentially the same, just greater than five characters. "Your input is greater than 5 characters long."
And then finally, I’m going say else
, so if it’s not less than or greater than, then it’s going to be exactly five. I’m going to say, "Your input is 5 characters long."
03:36 Okay, I think this is the script that I want. I want to display specific strings depending on the length of the user input. And I want to collect the user input first. All right, let’s save this and try it out.
03:49 I can run it with F5, and it’s asking me to save first. So I say OK …
04:02
Save. And here on the left side, now you can see that once I saved the script and ran it using F5, here in IDLE, I get the input here that I defined, Check string length:
. All right, I’m going to say hello there
.
04:18
And it gives me back the output, Your input is greater than 5 characters long.
Okay, and then it restarts it, tries it again. So I’m going to say hi
.
04:27
It’s less than five characters long. I guess I pressed F5 twice. It’s why it ran it twice. Start it again, And now 12345
. This should be exactly five characters long.
04:39 Seems to be working fine. Okay, so this is how I solved this challenge. Now, you don’t necessarily need this intermediate variable. You could also instead do a length check in here every time, but I’m using it twice, so I just thought, all right, I’ll define the variable and then reuse it down there.
05:00
And here at the else
statement, maybe it’s also interesting, like it could also write elif string_length == 5
,
05:08
and that should work as well. But in this case, it’s, nice to have this else
clause just to catch anything because the only condition that would come down here after these two, less than and bigger than, would be equals to.
05:23
So you could just catch it with the else
like I did before. And then I also left a comment that kind of, like, indicates that the else
clause in this case means that it’s exactly five characters long.
05:37 Okay, cool. This would be a possible solution. Small extra formatting. Curious if you came up with something else. Post it in the comments.
05:49
And here’s another solution that uses the len(my_input)
without the intermediate variable and a little more general "Type something: "
than the way that I solved it.
05:58 But this is another alternative solution that you could use to tackle this exercise.
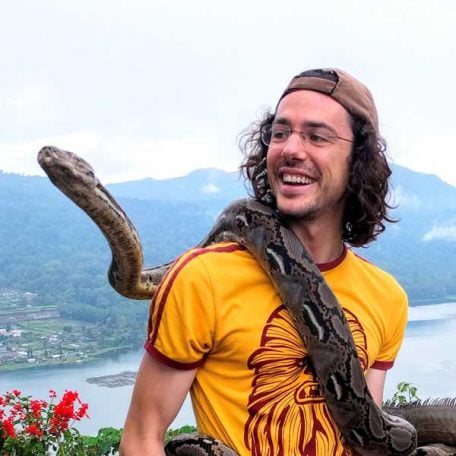
Martin Breuss RP Team on Sept. 29, 2023
DimaG nice, thanks for sharing! Here’s a link for others who are interested to learn more about the walrus operator :=)
Learner on Nov. 25, 2023
This is my version of this exercise.
#I'm an absolute beginner to the Programming and I love these exercises :)
text = str(input("Enter your input text: "))
length = len(text)
if length < 5:
print(f"Your input {text=} entered is less than 5 characters long")
elif length > 5:
print(f"Your input {text=} entered is more than 5 characters long")
elif length == 5:
print(f"Your input {text=} entered is 5 characters long")
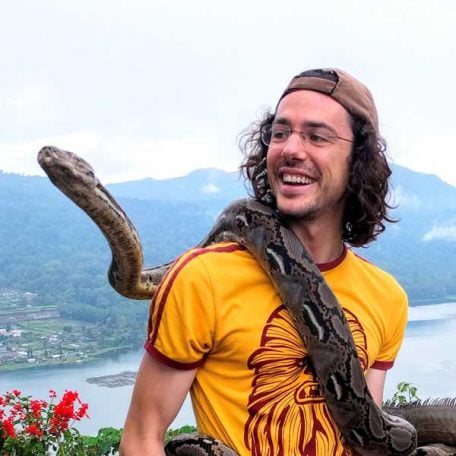
Martin Breuss RP Team on Dec. 6, 2023
@Learner yay, glad to hear that you’re enjoying the exercises and good work coming up with a solution! 🙌 :D
Becs on Jan. 22, 2024
user_input = input("Enter a word/s: ")
if len(user_input) < 5:
print("Your input is less than 5 characters long")
elif len(user_input) == 5:
print("Your input is 5 characters long")
else:
print("Your input is greater than 5 characters long")
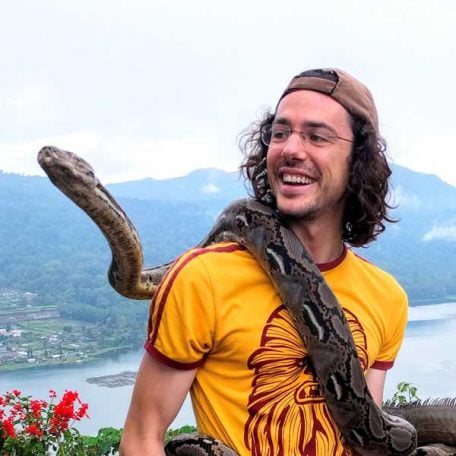
Martin Breuss RP Team on Jan. 24, 2024
Nice @Becs! If it’s not less than or exactly 5
, then it’s gotta be more than 5
:)
Anonymous on Feb. 14, 2024
I wanted to do it more in a manual way without using the len()
function, and I think that way you make it more challenging but also allows you think think in a different way, and even though the built-in functions are already optimized, choosing not to use the naive approach allows you to make use of various python features.
word = input('Word: ')
target_length = 5
current_length = 0
for idx, _ in enumerate(word, 1):
current_length = idx
# if idx is longer than the target no need to check further
# This also makes sure we can exit the loop avoiding unnecessary looping
if current_length > target_length:
print('Your input is greater than 5 characters long')
break
else:
if current_length < target_length:
print('Your input is less than 5 characters long')
elif current_length == target_length:
print('Your input is 5 characters long')
JVargas on Jan. 24, 2025
This is my solution knowledge acquire mainly Real Python, assistant chatGPT using VSCode.
def prompt() -> str:
"""Display a message to the user, then wait for a response."""
user_input = input("Enter a word: ")
return user_input
def is_to_long(user_input) -> str:
"""Determine if the input is too long."""
set_length = 5
if len(user_input) < set_length:
return "Your input is less than 5 charecters long."
if len(user_input) > set_length:
return "Your input is greater than 5 characters long."
#else:
return "Your input is 5 characters long."
def main() -> None:
"""Run the main program."""
prompt_input = prompt()
print(is_to_long(prompt_input))
if __name__ == "__main__":
main()
Become a Member to join the conversation.
DimaG on Sept. 27, 2023
This is my version of the code for Control Flow of Your Program using walrus operator: