Recover From Errors (Solution)
00:00
Here we are. Let’s give it a go. To start off by asking the user to input an integer, I’m going to say users_integer = input("Enter an integer: ")
.
00:19
This is going to be a string so far, right? But I do want it to be an integer. So what I can generally do is I can convert this to an integer, so I’m going to say int()
, use the int()
function here to convert this to an integer.
00:33 But now if the user inputs something that isn’t an integer, then the program’s going to break. Let’s try it out. I already saved this so I can run it. I’m going to say F5.
00:42
I enter an integer, so if I enter an integer here, 3
, then no problem. But let’s try it again. If I enter something that can’t be converted to an integer, like e
, then I get this traceback ValueError
because Python can’t convert the e
to an integer.
01:00
So this is the error that I want to catch and do something instead of quitting the program like this. So, what I’ll do is I’ll wrap this inside of a try
… except
block.
01:10
I’m going to say try to convert the user’s input to an integer, and then I want to print it, users_integer
. And now I’m going say except
I run into this ValueError
, and then I want to do this again, right? So I want to collect another input.
01:31
So, an easier way to do this is wrap the whole thing inside of a loop. So I can say while True:
01:40
try to collect and convert the users_input
to an integer. But if I run into a ValueError
, then I’m just going to tell them to try again, "Please try again!"
01:53 And in this case, I don’t have to do anything. So now I’m repeating the process until the user has entered an integer. Let me just move all of this up there.
02:03
But now I still need to get out of this infinite loop somehow. So, for this, you can use the break
keyword that you’ve practiced before, and we put it into the condition where you actually achieved what you want to achieve, right?
02:15
So in this case, I’m going to say break
if we did not run into a ValueError
, if it worked successfully to convert the user’s input to an integer and then print it back out to them, then we’re done with the program. Then I want to break out of this infinite loop.
02:30
Otherwise, if we keep running into this ValueError
, then we are going to just keep looping back to the beginning and trying again. Give it a go. Save this, try it out, enter an integer.
02:43 I’m going to say not an integer, try again.
02:48 Such a polite program too. And then if I add an integer, then it prints it back out and the program quits, and we never run into an exception. Great!
03:01
And here’s that solution: while True
, try to collect the user input and print it out. In this case, you’re doing the integer conversion, not right on the input, but then afterwards, which comes down to the same because it’s both still in the same part of the try
… except
block. So, it’ll still throw the ValueError
and then catch it down here, and otherwise the solution is the same.
03:23 Did you solve the challenge differently? Then post it in the comments.
Anonymous on Feb. 14, 2024
Here is an alternative solution by using built-in string methods
while True:
number = input('Enter a number: ')
if number.isdecimal():
number = int(number)
print(f'You entered: {number}')
break
print('You entered an invalid number. Try again!\n')
RV on Oct. 19, 2024
Hello, here is my solution below. But I would like to know are there any guidelines on how many lines of code it is considered acceptable to have in the “try”-block of code. Can an all relevant logic be put inside the “try”-block regardless of number of lines of the code that it takes or should it be split so that some small part is located inside the “try”-block and the rest is only after the “except”-block.
while True:
try:
user_input = int(input("Enter an integer: "))
except ValueError:
print("Try again!")
continue
print(f"You've entered an integer {user_input}")
break
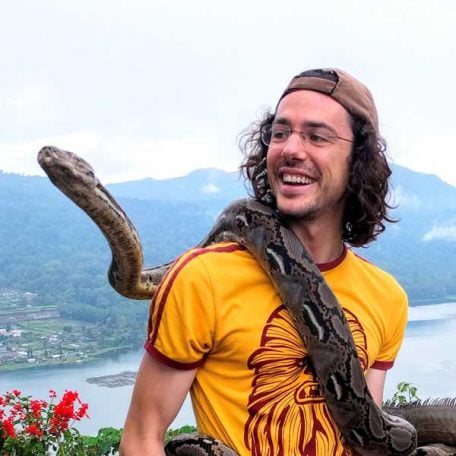
Martin Breuss RP Team on Oct. 21, 2024
@RV There are no strict guidelines or limitations on the number of lines of code that should be inside a try...except
block. However, there are best practices to consider when deciding how much logic to include within a try block:
-
Keep try Blocks as Small as Reasonable: It’s generally a good idea to keep try blocks as small and focused as possible. Only include the lines of code that you expect might raise the exception you’re handling. This makes it easier to understand what part of the code might fail and improves the readability of the error handling logic.
-
Limit Unintended Exception Capture: If you include too many lines in a try block, you might unintentionally catch exceptions from other parts of the code that you don’t expect to fail. This can mask bugs or make it harder to debug. The goal is to catch exceptions specifically where they are likely to occur.
-
Separation of Concerns: Consider splitting the logic so that the try block handles the risky code (e.g., file I/O, network requests) and subsequent logic is outside the try block. This ensures that only the necessary parts of the code are under the umbrella of error handling, and any unrelated logic doesn’t get caught in the same try block.
-
Avoid Using try for Flow Control: Try not to overuse try blocks as a general flow control mechanism. Exceptions should represent actual exceptional situations, not routine events.
Sometimes a longer try block is necessary if multiple related operations depend on one another and could fail together, or if you’re handling complex transactions, for example some database operations.
Generally it’s better to err on the side of keeping the try block small and focused. However, if splitting the logic outside the try block doesn’t make sense or leads to code that’s harder to understand, you can include more lines in the try block.
RV on Oct. 31, 2024
Martin, thank you for your comprehensive reply!
Become a Member to join the conversation.
MarkYoung on Sept. 29, 2023
Could ask the user to enter a float to test the program too. Just an idea.