Continue From the Beginning (Solution)
00:00 Here we are back in IDLE, and I copy/pasted a short task description in there. So, let’s get going. So, I note that I’m going to need to loop over every number from one through fifty.
00:11
So, now I can do this using a for
loop. So, I’ll start with that. I’m going to say for number in range(1)
—and here’s a nice popup that tells me that the final step is not included—so I’m going to have to say (1, 51)
to loop from starting to 1
up to 50
.
00:33 Okay, let’s take a little step in between and actually run this to see what happens. We’re going to print out the number. See if I did that correctly.
00:41
Use F5 to run it. Already saved it before, so now I get a printout, starts from 1
and goes all the way to 50
. So, that’s looking good.
00:49
So, I’m able to display every number from 1
through 50
. That part is basically done, and now I have another part that says except multiples of 3
.
01:01
So, I need to put in some condition here that filters out every number that’s a multiple of three. And you can do this using the percentage sign (%
), which is also called the modulo operator.
01:11
So, it can say if number % 3 == 0:
I need something in here. What this says is that if the number is divisible by three without a remainder, so if there’s no remainder if I divide the number by three, so that means that it’s a multiple of three, then I want to not print it.
01:36
So in this case, I need to do something in here, and that’s where I use the loop keyword. I’m going to say continue
, which ends the execution of this specific round of the loop and jumps back to the beginning, goes to the next number basically. And so in this case, if this conditional expression here evaluates to True
, the Python program will use the continue
keyword, and it will not continue to run the print()
statement underneath, which means that it’s not going to print out values for numbers that are divisible by three without a remainder. Let’s try this out.
02:08 I’m going to run it again, F5.
02:12
And now we can see that 3
is missing, 6
is missing, 9
is missing, et cetera. So this worked out. Good job.
02:23
Here’s the solution. It’s using a little bit different naming. I used number
here to have it a bit more descriptive, but otherwise this is the same solution. Great.
02:32
So now you’ve trained both the break
and the continue
keyword, and you’ll be able to apply them later on in the challenge.
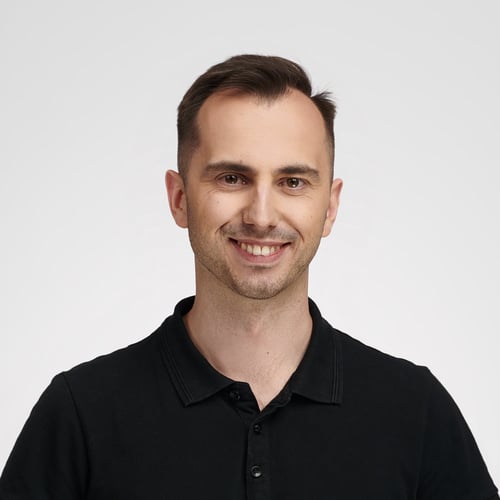
Bartosz Zaczyński RP Team on April 29, 2024
@frankpeters Using the modulo operator (%
) is a widely recognized way to check divisibility, which makes it almost like an idiom. Plus, it’s generally faster than dividing and then checking if the result is an integer. Another subtle issue that you may sometimes run into is the floating-point rounding error when you use the division operator (/
).
frankpeters on May 17, 2024
Thanks Bartosz, makes good sense! I’ll use the modulus operator moving forward!
Become a Member to join the conversation.
frankpeters on April 28, 2024
Hi, I approached this in a different way, getting to the same result:
Of course, the suggested solution using the “%” approach is better (less coding), but from a programming and learning point of view, is there any reason not to follow my approach (apart from the fact it takes more coding)?