Indexing and Slicing
In this lesson, you’ll see how to access individual elements and sequences of objects within your lists. Lists elements can be accessed using a numerical index in square brackets:
>>> mylist[m]
This is the same technique that is used to access individual characters in a string. List indexing is also zero-based:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a[0]
'spam'
>>> a[2]
'bacon'
>>> a[5]
'lobster'
>>> a[len(a)-1]
'lobster'
>>> a[6]
Traceback (most recent call last):
File "<input>", line 1, in <module>
a[6]
IndexError: list index out of range
List elements can also be accessed using a negative list index, which counts from the end of the list:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a[-1]
'lobster'
>>> a[-2]
'ham'
>>> a[-5]
'egg'
>>> a[-6]
'spam'
>>> a[-len(a)]
'spam'
>>> a[-8]
Traceback (most recent call last):
File "<input>", line 1, in <module>
a[-8]
IndexError: list index out of range
Slicing is indexing syntax that extracts a portion from a list. If a
is a list, then a[m:n]
returns the portion of a
:
- Starting with postion
m
- Up to but not including
n
- Negative indexing can also be used
Here’s an example:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a[2:5]
['bacon', 'tomato', 'ham']
>>> a[-5:-2]
['egg', 'bacon', 'tomato']
>>> a[1:4]
['egg', 'bacon', 'tomato']
>>> a[-5:-2] == a[1:4]
True
Omitting the first and/or last index:
- Omitting the first index
a[:n]
starts the slice at the beginning of the list. - Omitting the last index
a[m:]
extends the slice from the first indexm
to the end of the list. - Omitting both indexes
a[:]
returns a copy of the entire list, but unlike with a string, it’s a copy, not a reference to the same object.
Here’s an example:
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a[:4]
['spam', 'egg', 'bacon', 'tomato']
>>> a[0:4]
['spam', 'egg', 'bacon', 'tomato']
>>> a[2:]
['bacon', 'tomato', 'ham', 'lobster']
>>> a[2:len(a)]
['bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a[:]
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a == a[:]
True
>>> a is a[:]
False
>>> s = 'mybacon'
>>> s[:]
'mybacon'
>>> s == s[:]
True
>>> s is s[:]
True
A stride can be added to your slice notation. Using an additional :
and a third index designates a stride (also called a step) in your slice notation. The stride can be either postive or negative:
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a[0:6:2]
['spam', 'bacon', 'ham']
>>> a[1:6:2]
['egg', 'tomato', 'lobster']
>>> a[6:0:-2]
['lobster', 'tomato', 'egg']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a[::-1]
['lobster', 'ham', 'tomato', 'bacon', 'egg', 'spam']
00:00 In this video, you’ll practice list indexing and slicing. The elements of a list can be accessed by an index. To do that, you name the list, and then inside of a pair of square brackets you use an index number, like what I’m showing right here.
00:17
That allows access to individual elements within the list. The indexing for the list is zero-based. So if you have a list such as this, with these six elements, the indices start with 0
and would go up to 5
.
00:36 Let me have you try that out. Start with a new list.
00:47
Here’s a
. As described, indexes are zero-based for lists, so a[0]
would access the first item in that list.
01:03
a[2]
would access the third one. And in this case, a[5]
would access the last. Another way to get there would be a
and the len()
(length) of a
minus 1
.
01:18
If you use an index value that’s too high, Python will raise an exception—an IndexError
saying that the list index is out of range. Negative indexing is available also.
01:33
If you want to access the last item, you’d start with -1
. So continuing to work with that list a
I’ll have you try out negative indexing.
01:44
a[-1]
will access the last item, a[-2]
, and so forth. So in this case -6
—which is also negative the length of your list—would return the first item.
02:05
If you try to access an index that’s beyond the scope, you’ll get that index out of range
also. Slicing is available too. Slicing’s an indexing syntax that’s going to extract a portion from your list. So in this example, if a
is your list, then inside your square brackets you would have your two index numbers separated by a colon (:
), and it’s going to return a portion of that a
list that will start with position m
and go up to but not include index n
.
02:38
Using that same example from before, if you took a[2:5]
, you’d get the three objects in the middle, starting at index 2
and going up to index 4
, but not including 5
.
02:50
Let me have you try it out. Okay, so here’s your list. What if you wanted to slice? Let me have you start at index 2
and then using a colon. For the second index, use 5
. From 2
up to but not including 5
.
03:05
Great! You can also use negative indexes, so you could say starting at -5
and going to -2
, which is the same as going from 1
to 4
.
03:19 And you can confirm that here
03:29 A shorthand is available. By omitting the first index, your slice is going to start at the beginning of the list and go up to the second index. If you omit the last index, it’s going to extend the slice from the first index and go all the way to the end of the list. And if you were to omit both indexes, it’s going to return a copy of the entire list.
03:54
And unlike with a string, it’s a copy—not a reference to the same object. So, what if you were to omit the first index? In that case, it will start at the beginning and go up to but not include that index. The same as from 0
to 4
.
04:12
And in the same way, removing the second index will go all the way to the end, starting with this index up to the end. Here, you can use len()
, which will return a value of 6
, and if we go from 2
up to but not include 6
, as the index.
04:35
If you were to remove both indexes and just have a [:]
, it returns the entire list. You can try this out by seeing if it is equal to a
, which is True
. But this is a copy and not a reference, whereas if you tried a is a[:]
—it’s a copy.
05:01
It’s not a reference to the original. That could be confusing because if you’ve worked with strings before, if you have a string, s
, which is 'mybacon'
, using the syntax of just the [:]
does return the entire string.
05:20
What’s interesting about it is not only is s == s
with the colon-only index, but it also is a reference to that object. Whereas with a list, it returns an entirely new object.
05:37
It’s possible to add a third index after the additional colon. That third index indicates a stride, but it’s also sometimes called a step. So in this example, if you had a slice that went from 0
to 6
, with a step of 2
, you would return the objects at index 0
, 2
, and 4
—'spam'
, 'bacon'
, and 'ham'
.
06:02
So if you went from index 0
up to 6
with a stride of 2
, you’ll see it grab the first, third, and the fifth items, skipping over with a stride of two.
06:14
If you started that at 1
instead, you’d get the other three.
06:20
And it is possible to have a negative stride, though you’d put the first index in as the highest value, and then where you want to go up to, a 0
, and in this case it’s going to go to -2
.
06:36
In fact, a simple syntax for just simply reversing your list is to leave the first two indexes blank with a colon, and then the second colon and a -1
—that will reverse your list.
06:50 Next, you’re going to practice using operators and some of Python’s built-in functions on your list.
Orlando Uribe on March 8, 2020
Thanks for this material. I guess there is a typo error (??) in one of the stride written examples (video example is OK):
Typo error:
>>> a[0:6:-2]
['lobster', 'tomato, 'egg']
the correct statement should be:
>>> a[6:0:-2]
['lobster', 'tomato, 'egg']
otherwise,
>>> a[0:6:-2]
[]
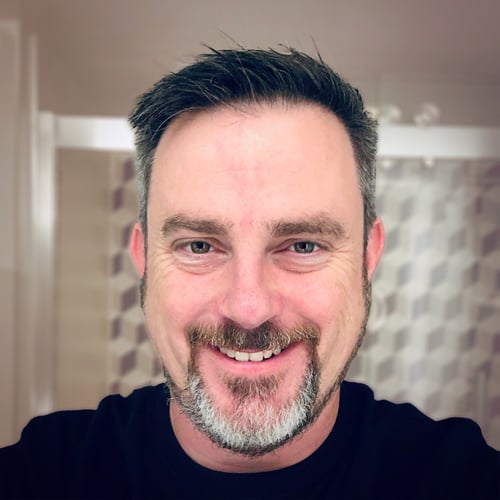
Chris Bailey RP Team on March 8, 2020
Hi @Orlando Uribe, You’re correct, I will get that changed to match the video lesson. Thanks
Ajay on June 3, 2020
@chris Video is very informative, thanks for this. When i was practising examples, got one doubt:
In [43]: a = [9, 1, 2, 4, 5, 6, 8]
In [44]: a[6:0:-2]
Out[44]: [8, 5, 2]
With negative striding why 9 is not included.
Does it exclude last value?
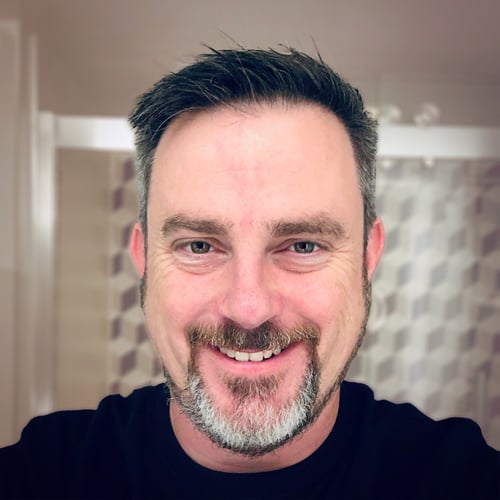
Chris Bailey RP Team on June 3, 2020
Hi @Ajay,
Thanks for watching the course! The slice you have created would start at index 6 and end at index 1 and not include index 0, which is why you are not getting index 0 of 9. If you were to use:
>>> a = [9, 1, 2, 4, 5, 6, 8]
>>> a[6::-2]
[8, 5, 2, 9]
That would include index 0. I hope this helps to explain what’s happening. Reiterating from the text below the lesson.
Slicing is indexing syntax that extracts a portion from a list. If
a
is a list, thena[m:n]
returns the portion ofa
:
- Starting with postion
m
- Up to but not including
n
Ajay on June 4, 2020
@chris thanks for clarification.
kiran on Aug. 11, 2020
>>> a[ : -1:-1]
''
Why it returns empty string? I am too much confusing while handling mixing of positive & negative indexes. Can you give me best clarity regarding this?
>>> 'hello world'[-2:-4]
''
kiran on Aug. 11, 2020
The value will be 'hello world'
.
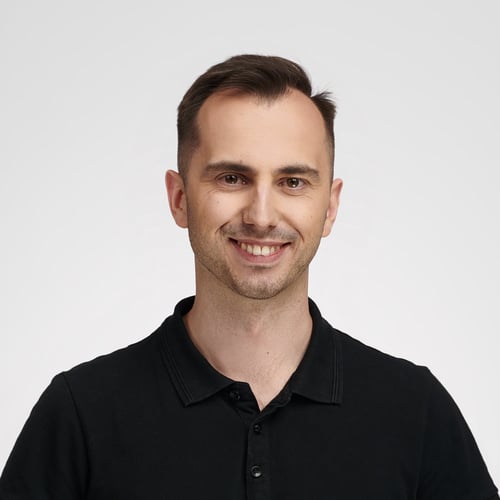
Bartosz Zaczyński RP Team on Aug. 12, 2020
Think of negative indices as a shorthand notation for subtracting from the given string length:
>>> name = 'Elizabeth'
>>> name[-1] == name[len(name)-1] == name[8] == 'h'
True
The negative indices in your “hello world” example would translate to this:
>>> x = 'hello world'
>>> len(x)
11
>>> x[-2:-4] == x[len(x)-2:len(x)-4] == x[9:7]
True
You’re getting an empty string because the left index is higher than the right one, while you haven’t indicated a reversed order with the third “step” argument. If you did, here’s you’d get:
>>> x = 'hello world'
>>> x[-2:-4:-1]
'lr'
You can get the resulting indices used by the underlying loop like this:
>>> slice(-2, -4, -1).indices(len('hello world'))
(9, 7, -1)
It returns a tuple that you can unpack and pass to the range()
function:
>>> list(range(9, 7, -1))
[9, 8]
>>> for i in [9, 8]:
... print('hello world'[i])
...
l
r
These are the indices used by the square bracket syntax.
kiran on Aug. 12, 2020
Brilliant @Bartosz Zaczyński. Thanks.
mo on Dec. 21, 2021
I happily started to use bpython; thanks for the tip. A try out all things. And more :) Doing that I ran into this:
>>> lst = ['AA', 'CC']
>>> lst
['AA', 'CC']
>>> lst[1:1] = 'BB'
>>> lst
['AA', 'B', 'B', 'CC']
I was expecting ['AA', 'BB', 'CC']
. How should I insert a string into a list?
mo on Dec. 21, 2021
Ah I found out already from 3 lessons later in this course … next time I will be more patient :)
Roman Gerasimov on April 11, 2024
Shorter version is a[::2]
. In this case, we do not even need to know the length of the list. Very useful.
Become a Member to join the conversation.
JulianV on Dec. 13, 2019
Nice!