List Methods
In this lesson, you’ll learn about the built-in methods that you can use to modify lists. List methods are different from string methods. Because strings are immutable, the methods applied return a new string object. The list methods shown here modify the target list in place and don’t have a return value.
Here’s an example of a string method:
>>> s = 'mybacon'
>>> s.upper()
'MYBACON'
>>> s
'mybacon'
>>> t = s.upper()
>>> t
'MYBACON'
>>> s
'mybacon'
.append()
appends an object to a list:
>>> a = ['a', 'b']
>>> a
['a', 'b']
>>> a.append(123)
>>> a
['a', 'b', 123]
>>> a = ['a', 'b']
>>> a
['a', 'b']
>>> x = a.append(123)
>>> x
>>> print(x)
None
>>> type(x)
>class 'NoneType'>
>>> a
['a', 'b', 123]
>>> a = ['a', 'b']
>>> a + [1, 2, 3]
['a', 'b', 1, 2, 3]
>>> a
['a', 'b']
>>> a.append([1, 2, 3])
>>> a
['a', 'b', [1, 2, 3]]
>>> a = ['a', 'b']
>>> a
['a', 'b']
>>> a.append('hello')
>>> a
['a', 'b', 'hello']
.extend()
axtends a list by appending elements from the iterable:
>>> a = ['a', 'b']
>>> a
['a', 'b']
>>> a.extend([1, 2, 3])
>>> a
['a', 'b', 1, 2, 3]
>>> a = ['a', 'b']
>>> a
['a', 'b']
>>> a += [1, 2, 3]
>>> a
['a', 'b', 1, 2, 3]
.insert(<index>, <obj>)
inserts the object <obj>
into the list at the specified <index>
, pushing the remaining list elements to the right:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.insert(3, 3.14159)
>>> a[3]
3.14159
>>> a
['spam', 'egg', 'bacon', 3.14159, 'tomato', 'ham', 'lobster']
.remove(<obj>)
removes the first occurence of the value <obj>
and raises a ValueError
exception if the value is not present:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.remove('egg')
>>> a
['spam', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.remove('egg')
Traceback (most recent call last):
File "<input>", line 1, in <module>
a.remove('egg')
ValueError: list.remove(x): x not in list
.clear()
removes all items from the list:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.clear
>>> a
[]
.sort(<key=None>, <reverse=False>)
sorts the list items in ascending order. An optional function can be used as a key. The optional reverse flag allows to reverse to descending order. For more details on this method, check out How to Use sorted()
and .sort()
in Python.
Here’s an example:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.sort()
>>> a
['bacon', 'egg', 'ham', 'lobster', 'spam', 'tomato']
>>> a += ['Apple', 'Zebra']
>>> a
['bacon', 'egg', 'ham', 'lobster', 'spam', 'tomato', 'Apple', 'Zebra']
>>> a.sort()
>>> a
['Apple', 'Zebra', 'bacon', 'egg', 'ham', 'lobster', 'spam', 'tomato']
>>> a.sort(key=str.upper)
>>> a
['Apple', 'bacon', 'egg', 'ham', 'lobster', 'spam', 'tomato', 'Zebra']
>>> a.sort(key=str.upper, reverse=True)
>>> a
['Zebra', 'tomato', 'spam', 'lobster', 'ham', 'egg', 'bacon', 'Apple']
>>> b = [1, 77, 98, 34]
>>> b
[1, 77, 98, 34]
>>> b.sort()
>>> b
[1, 34, 77, 98]
>>> b += ['apple']
>>> b
[1, 34, 77, 98, 'apple']
>>> b.sort()
Traceback (most recent call last):
File "<input>", line 1, in <module>
b.sort()
TypeError: '<' not supported between instances of 'str' and 'int'
.reverse()
reverses the list in place:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.reverse()
>>> a
['lobster', 'ham', 'tomato', 'bacon', 'egg', 'spam']
>>> a.reverse()
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a[::-1]
['lobster', 'ham', 'tomato', 'bacon', 'egg', 'spam']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a = a[::-1]
>>> a
['lobster', 'ham', 'tomato', 'bacon', 'egg', 'spam']
00:00 This video is to show you the methods that are available to your list objects. There’s one big difference between how list methods work compared to string methods. In another course I have here on Real Python about String and Character Data, we work with a large number of string methods.
00:17 And the thing about string methods is that they return a new string object that is modified, but they leave the original string unchanged. But unlike a string, the methods that you apply to a list modify the target list in place, changing the original, and they don’t return a new list at all. In fact, they don’t return anything typically. Now there’s a couple of exceptions to that, which I’ll show you as we go through here.
00:41
I’m going to have you practice with a couple string methods quickly here. Create a string s
. By placing the dot (.
) after your string, you can see the large number of string methods. And as you can see, if I were to apply .upper()
, which will basically convert the whole thing to uppercase, it returns that, but s
is not modified.
01:00
I’d have to actually create a new object t
and then assign s.upper()
into it, so t
would be saved versus s
. Just to reiterate, one of the big differences between lists and strings: lists are going to modify in place and actually not return anything when you apply these methods.
01:20
So, let’s try out some of these lists methods. The first method you’re going to explore is .append()
. In .append()
, it appends that object to the end of the list. One note: it appends it as a singular object, so if you append an integer or a string, that will come in as a single object.
01:37 But also, if you append a list or another iterable, it will append that as a singular object also. Create a simple list.
01:48
Here’s a
. And again if you use the dot notation, you can see here in bpython
, it’s showing you all the different ones that we’re going to cover, and append
being the first one. For .append()
it says here it appends an object to the end of the list. Okay, so in this case, 123
as an integer, a.append()
didn’t return anything but as soon as I press a
by itself, you can see that it has been appended.
02:10
So it’s modifying the target list in place, not creating something new. Let me have you practice it one more time. Here’s a
again with its two objects.
02:20
Let’s create x
and append 123
to it. So, this looks a little weird, especially since you just did something with strings that, this worked perfectly.
02:31
But here we’re saying a
, append 123
to it, and then assign that to x
. This would normally assign what’s being returned from this to x
. Well, in that case if you type x
by itself and press Return in the REPL, it doesn’t have anything. It exists, but if you were to print(x)
it would say None
or you were to type(x)
it’s a NoneType
. a.append()
didn’t return anything into x
.
02:58
a
got modified in place by using this method on it. So again, behavior very different. Now, the concatenation operator behaves a little differently from this method .append()
. So go back to a = ['a', 'b']
.
03:16
If you were to take a
and concatenate [1, 2, 3]
, now this isn’t going to modify a
, it’s just going to return this, so a
is still the same.
03:28
But I wanted to show you that if you use that operator for concatenation, it will take a list into it and break it out into the individual items and add them here. .append()
is going to behave differently. a
is still ['a', 'b']
.
03:41
What if you did a.append()
and then put in the list [1, 2, 3]
? Again, square brackets inside those parentheses. Again, returning nothing.
03:50
It appended it, but it appended that as a sublist. So a single object into that spot at the end of your existing list a
—be aware that .append()
behaves differently than concatenation did.
04:09
take back a
to just being ['a', 'b']
. And then if you did a.append()
, what if you put a string into it? So in this case, you’re appending 'hello'
.
04:17
Well, in that case, it doesn’t break it apart into individual characters the way the concatenation operator did. It appends it as a singular object. Now, if you needed to append an iterable, there’s a separate method called .extend()
, which I’ll show you next.
04:34
The method .extend()
takes an iterable as an argument into it, in contrast to the method .append()
, which took a single object and behaves a lot more like the concatenation operator did. For .extend()
, let’s reset a
here.
04:50
What if you want to extend a
? You can see here it takes an iterable and it’s going to extend that list by appending all the elements from the iterable. So in this case, if you were to give it a list of [1, 2, 3]
inside the parentheses—very different behavior.
05:04
You can see those integers being added to the end of a
list. Again, behaving much more like if a += [1, 2, 3]
, using concatenation.
05:20
The method .insert()
inserts the object into a list at the specified index. Remaining elements are pushed to the right. Here’s our old list from before.
05:33
In this case, if you’d said a.insert()
it says here, okay, you need to enter an index
and then the object
. Let me have you put index 3
, and then the object—
05:48
in this case, a float. So what’s at a[3]
now? There it is. And you can see that it truly did insert it in the middle of the existing list. The object will be inserted and the remaining list elements are going to be pushed to the right.
06:04
The method .remove()
will remove an object from a list. If the object isn’t contained within the list, an exception will be raised. Let me hit the Up arrow a couple of times here to retrieve the original a
list.
06:16
For .remove()
, it will remove the very first occurrence of the value. So let’s say we want to get rid of 'egg'
. Again, it returned nothing and you can see that it has been removed from list. Well, what if you tried it again? In this case, an exception will be raised, ValueError
, that you can’t remove that from there because that object x
isn’t in the list.
06:41
.clear()
empties the list completely. Again, we’ll hit the Up arrow a couple times to get back our original a
list. So here’s a
again. What if we use .clear()
?
06:56
Well, that one’s pretty straightforward. It’s going remove all the items from the list. So a
is now an empty list. And you can see a.clear()
didn’t return anything.
07:05 It just blew out the whole list, clearing it completely. Pretty straightforward.
07:12
One of the more advanced features that’s unique to lists is the method .sort()
. The default behavior is to sort your items in your list in an ascending order.
07:23
But the method also has two optional arguments: one being a key
, which is a function that you can apply when sorting, and the other is to reverse the order from ascending to descending.
07:35 The ability to specify a function within the method is pretty powerful, and I’m not going to go into too much depth here, but there is a Real Python article that discusses this in detail, and I’ll have a link to it in the description text below this video.
07:52
I’ll have you start with the familiar list here of a
. If you put .sort()
in, if you put no arguments, you can see here it’s done that alphabetically.
08:02 Now, there’s still a problem that we mentioned before, where it’s if you have elements in your list that start with uppercase letters,
08:14
that you might get a little odd return here. .sort()
placed the words with the uppercase first letters at the front of the list. Those, again, have a lower ordinal value—these two letters do—so everything lowercase is going to be after.
08:29
I’ve included that link in the text below this video to the Real Python article about .sort()
and sorted()
, so I’m not going to dive too deep into it, but that’s what this key
is about. You can say key=
and then you could say something like str.
—let’s make everything be uppercase for the .sort()
. And in that case, the result is that everything will be sorted as if all of these words were an uppercase. So, .sort()
is pretty powerful.
08:59
It’s a unique thing to lists, compared to other iterables, because it does sort it in place. There is the ability to reverse, so we could say key=upper
and then reverse=
, instead of it being defaulted to False
, we could say True
.
09:16 And that would give you a reverse list. One issue, though, is that if you have a list where you’ve mixed things—
09:27
well, let’s start with unmixed. Okay, so here’s b
. b.sort()
would put them in order but if b
had a string item in there such as 'apple'
, if you were to try to attempt to sort that, it’s going to have a problem with the default method that’s being used here.
09:46
It’s going to give you an exception and say that—in a similar way to min()
and max()
—that instances of string and integer can’t be compared with this operator.
09:58
The next method is .reverse()
, which reverses the order of the items in your list. Okay. So here’s that simple list of a
. If you were to try .reverse()
, it’s pretty straightforward. It’s just going to reverse the items in place.
10:12 In fact, if I do it again here, it will put them back in the original order.
10:17 If you were to do it slicing with a negative stride,
10:24
it would reverse it. Now, in this case that didn’t reverse a
, right? It just simply returned that as a slice. So if you wanted to, you would have to reassign it. That would be reversed.
10:36
So probably .reverse()
as a method is a little faster, depending on how you’re typing it.
10:43 The list methods that you covered so far didn’t have any kind of return values, but there are a few that do. That’s what you’re going to cover in the next video.
kiran on July 24, 2020
>>> a = ['a', 'b']
>>> a += 'c'
>>> a
['a', 'b', 'c']
# a += 'c' means a = a + 'c' but i try like this a = a + 'd' it showing error.
>>> a = a + 'd'
Traceback (most recent call last):
File "<pyshell#40>", line 1, in <module>
a = a + 'd'
TypeError: can only concatenate list (not "str") to list
can you explain why it perform like this?
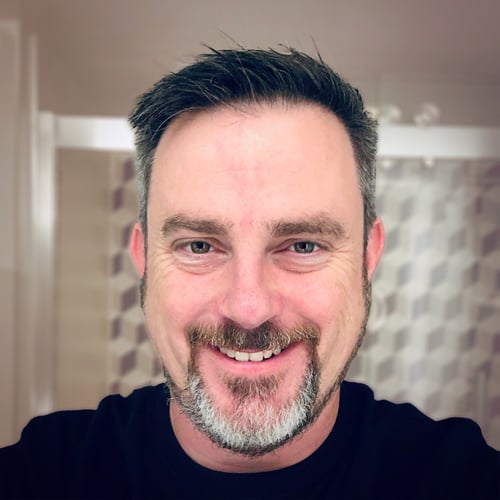
Chris Bailey RP Team on July 24, 2020
Hi @manupanduworld,
The +
operator will only concatenate lists together. It will not concatenate objects of different types. Hence the error you are getting.
TypeError: can only concatenate list (not "str") to list
Two ways to accomplish the end result would be: Using concatenation
>>> a = ['a', 'b']
>>> a += ['c'] # adding a new list with the 'c' str object
>>> a
['a', 'b', 'c']
or using the .append()
method
>>> a = ['a', 'b']
>>> a.append('c')
>>> a
['a', 'b', 'c']
DoubleA on Jan. 31, 2021
Hi Chris! I haven’t found any reference to the article / course on how to use .sort()
method or sorted()
function you referred to in this lesson. Is this the course you referred to?
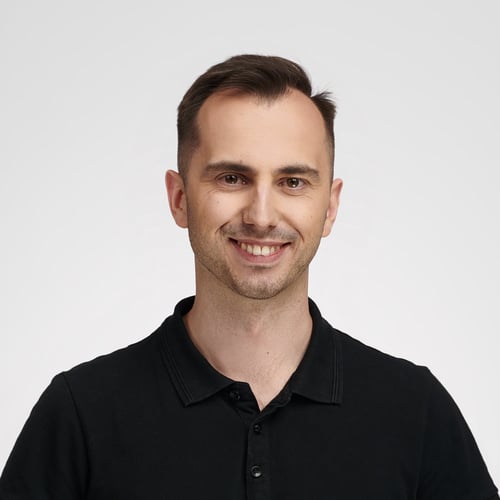
Bartosz Zaczyński RP Team on Jan. 31, 2021
@DoubleA Check out this tutorial: How to Use sorted() and sort() in Python.
DoubleA on Jan. 31, 2021
@Bartosz thanks mate! Going to check it out!
Become a Member to join the conversation.
kiran on July 24, 2020
a + = 'c'
meansa = a + 'c'
but i can return like this it showing errorWhy like this?