List Methods With Return Values
In this lesson, you’ll learn about the additional built-in methods that, unlike the ones in the previous lesson, have return values.
.pop(<index=-1>)
removes an element from the list at the specified index. The item that was removed is returned. The default is to remove the last item in the list <index=-1>
if no index is specified:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.pop()
'lobster'
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham']
>>> b = a.pop()
>>> b
'ham'
>>> a
['spam', 'egg', 'bacon', 'tomato']
>>> a.pop(1)
'egg'
>>> a
['spam', 'bacon', 'tomato']
>>> a.pop(-2)
'bacon'
>>> a
['spam', 'tomato']
.index(<obj>[,<start>[, <end>]])
returns the first index of the value <obj>
in the list. Optional start and end indexes can be used. It raises an exception if the value <obj>
is not present:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.index('tomato')
3
>>> a[3]
'tomato'
>>> a.index('egg', 0, 4)
1
>>> a.index('egg', 2, 5)
Traceback (most recent call last):
File "<input>", line 1, in <module>
a.index('egg', 2, 5)
ValueError: 'egg' is not in list
.count(<obj>)
returns the number of occurrences of the value <obj>
in the list:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.count('ham')
1
>>> b = [2, 4, 6, 8, 6, 4, 6, 2, 100]
>>> b
[2, 4, 6, 8, 6, 4, 6, 2, 100]
>>> b.count(6)
3
>>> b.count(0)
0
>>> b.count(77)
0
mylist.copy()
returns a shallow copy of the list. For more details on shallow vs deep copying, check out Shallow vs Deep Copying of Python Objects:
>>> a = ['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> a.copy()
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> b = a.copy()
>>> a == b
True
>>> a is b
False
>>> a[0] = 'trees'
>>> a
['trees', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> b
['spam', 'egg', 'bacon', 'tomato', 'ham', 'lobster']
>>> x = ['a', ['bb', ['ccc', 'ddd'], 'ee', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> x
['a', ['bb', ['ccc', 'ddd'], 'ee', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> x[1]
['bb', ['ccc', 'ddd'], 'ee', 'ff']
>>> x[1][1]
['ccc', 'ddd']
>>> x
['a', ['bb', ['ccc', 'ddd'], 'ee', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> y = x.copy()
>>> y
['a', ['bb', ['ccc', 'ddd'], 'ee', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> x
['a', ['bb', ['ccc', 'ddd'], 'ee', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> y == x
True
>>> y is x
False
>>> y[1][1]
['ccc', 'ddd']
>>> y[1][2]
'ee'
>>> y[1][2] = 'zz'
>>> y
['a', ['bb', ['ccc', 'ddd'], 'zz', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> x
['a', ['bb', ['ccc', 'ddd'], 'zz', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> y[2] = 'Bob'
>>> y
['a', ['bb', ['ccc', 'ddd'], 'zz', 'ff'], 'Bob', ['hh', 'ii'], 'j']
>>> x
['a', ['bb', ['ccc', 'ddd'], 'zz', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> z = x[:]
>>> z
['a', ['bb', ['ccc', 'ddd'], 'zz', 'ff'], 'g', ['hh', 'ii'], 'j']
>>> z[3][1]
'ii'
>>> z[3][1] = 'Jerry'
>>> z
['a', ['bb', ['ccc', 'ddd'], 'zz', 'ff'], 'g', ['hh', 'Jerry'], 'j']
>>> x
['a', ['bb', ['ccc', 'ddd'], 'zz', 'ff'], 'g', ['hh', 'Jerry'], 'j']
00:00 In this video, you get a chance to work with a few list methods that do have return values.
00:08
The method .pop()
removes an element from the list. In this case, you specify the index of the item that you want to remove. By default, it’s going to remove the last item, with an index
of -1
, but the unique thing about it is that it actually returns the item that’s removed.
00:23
Let me have you take a look at it. Bring back that list of strings a
. So, .pop()
is going to remove and then return the item at index
. The default is the last, index=-1
.
00:35
Just calling .pop()
will not only remove 'lobster'
from the list, but it actually does return it. Here’s a
. So, it is possible to capture that, if you had b = a.pop()
, that actually would throw 'ham'
into b
. Let’s check. Yep! There it is. And a
is now short one more also. If you wanted to go from the other side,
01:02
you can put just the numeric index in, so let’s say 1
, which should remove 'egg'
. Yup, there it goes. And you can see now just three items remain. Negative indexing works also.
01:11
So if you were to say -2
, that should take out 'bacon'
, and now we’re down to just two. So again, the default is a -1
, removing the last item. And again, what’s kind of unique is the fact that it is not only popping something out of the list, but it’s returning it, so you could put it into another object.
01:35
The method .index()
searches for an object within the list and returns the index. It includes optional start and end indexes for where you want to search within the list.
01:54
So, what about .index()
? .index()
is going to return the first index of a particular value you’re searching for. If the object isn’t present, it’s going to raise an exception. We could say here we’re looking for 'tomato'
. And it says, “Okay, that’s index 3
.” a[3]
, yup, 'tomato'
. Okay, great! And along with that, you can specify a start
and stop
. So you could say, “Oh, start at the beginning, but only go up to 4
.” And it says, “Okay, well 'egg'
is at 1
,” but if your index was from 2
to 5
, that would raise an exception, a ValueError
, that 'egg'
isn’t within the list.
02:45
The next method is .count()
. It’ll return an integer based upon how many times an object appears within the list. So, .count()
just returns the number of occurrences of a particular value, so in this case, since a
is rather simple, we look for 'ham'
within a
—oh, there’s only 1
. Let’s make a new list.
03:13
b
is filled with a bunch of integers, but if you were to do b.count()
and look for how many times 6
occurs inside of here, you’d get 3
.
03:21
If you were to search for something that doesn’t exist inside there, say 0
, it would say, “Well, there’s 0
of those.” Or another integer, like 77
.
03:33
And the final method to cover is .copy()
. .copy()
returns a copy of the list. But one note that it is a shallow copy. I’ll do a quick explanation, but for further information, check out the link inside the description text below this video. Here’s a
.
03:53
In the case of a
, if you choose .copy()
, it’s going to return a copy of the list. Note that it says that it’s a shallow copy of the list. In this case—boom, there it is. It just returned that whole list in there.
04:08
So if you wanted to copy something, have b = a.copy()
, now does a == b
? Yes. Is a
b
? No, that’s not True
. It’s an independent object.
04:23
Okay. And just a note, so if you were to take a
and take the first item in a
and reassign it to something else, let’s say 'trees'
. So a
looks like that, b
looks like this. Okay, good to know. What they mean by shallow copy—the copied elements will be the first layer, whereas the objects in the second layer will be references.
04:46
So if you have an object like that more complex object you dealt with before of x
—you might remember? x
was nested, with three layers.
04:59
You can copy and paste it from the text below, if you’d like. So again, the structure of x
is pretty advanced. If you were to index the object at index 1
, again, it’s got a list in it and a sublist in it.
05:13
And x[1][1]
is that sublist here. Okay. So what happens if you make a new object y
and you .copy()
—again, a shallow copy—into y
? So, y
looks the same as x
, and y
is equal to x
, right?
05:29
But y is not x
? That’s True
, it’s not. That’s False
. Okay, what if you took y
, our new object, and you took the object at y[1][1]
and you modify it.
05:41
Let’s say—actually just do y[1][2]
. That’s 'ee'
. It’s within the sublist here at index 1
, and its index 2
is 'ee'
.
05:52
Okay, what if you take that and you reassign something to it? You say, “Okay, that’s equal to 'zz'
instead.” Okay, it took that. So there’s y
and you can see 'zz'
has been changed inside the list there. Well, a strange thing happens. Inside x
it changed it also, so this is a reference.
06:10
If you were to change something else about y
—like here, let’s say you change out this object at index 2
, and you say that’s equal to 'Bob'
. y
changes and x
doesn’t.
06:23 So a shallow copy means that it’s copying all the objects across, but only references to these sublists, and it’s something you need to be aware of that can kind of be a pitfall.
06:34
Again, I’ll include more information where you can learn a little bit more about it. And it’s the same behavior if you were to do like z = x[:]
using your slicing technique with just the colon.
06:47
Copy it like that. So z
would behave the same way if you took z[3][1]
, which is 'ii'
,
06:59
and you reassign it to 'Jerry'
. So there it is, sitting out here inside the substring. Okay. What happened to x
? That modified x
also.
07:09 So you need to be cautious when you’re using sublists within a list and making copies. Next up, you’re going to start practicing with tuples.
kiran on July 24, 2020
Hi can you update this airtical List and Tuples with following methods…?
mylist.index(<obj>[, <start>[, <end>]])
mylist.count(<obj>)
mylist.copy() - returns a shallow copy
if possible shallow vs deep copy too. Thank you.
jpkaiser51 on Jan. 1, 2021
In this video, when the code snippets are typed in, python info automatically pops up in the IDE. How do you get the code comments to show up when you write code in the repl…(I think it is the repl). For example, when the code a. is entered, the list of dot methods show up. Also, when append is selected, the append options automatically pop up. How to? Thank you, J
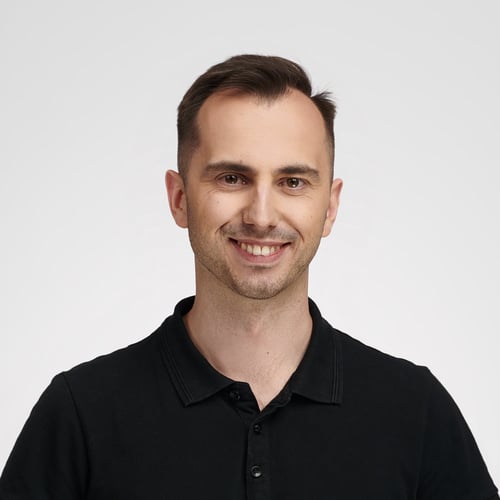
Bartosz Zaczyński RP Team on Jan. 4, 2021
@jpkaiser51 Chris uses an alternative Python REPL called bpython, which comes with all these amenities out of the box 😉
utkarshsteve on Dec. 5, 2021
Is slicing operation, shallow copy or deep copy?
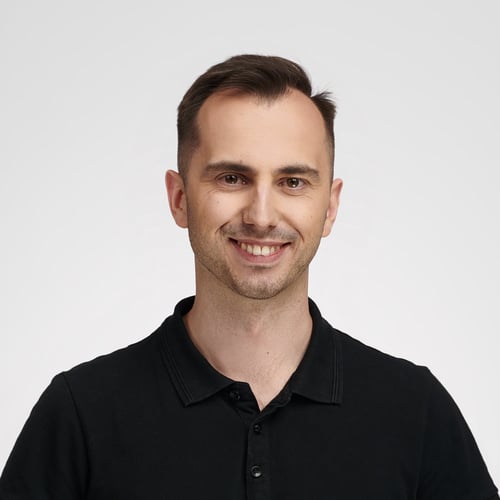
Bartosz Zaczyński RP Team on Dec. 5, 2021
@utkarshsteve Slicing results in making shallow copies:
>>> items = [object(), object(), object()]
>>> copies = items[:]
>>> [a is b for a, b in zip(items, copies)]
[True, True, True]
Use the copy
module if you need a deep copy instead:
>>> import copy
>>> deep_copies = copy.deepcopy(items)
>>> [a is b for a, b in zip(items, deep_copies)]
[False, False, False]
Become a Member to join the conversation.
kiran on July 24, 2020
Hi can you update this airtical List and Tuples with following methods…?
mylist.index(<obj>[, <start>[, <end>]]) mylist.count(<obj>) mylist.copy() - returns a shallow copy
if possible shallow vs deep copy too. Thank you.