Tuple Assignment, Packing, and Unpacking
In this lesson, you’ll learn about tuple assignment, packing, and unpacking. A literal tuple containing several items can be assigned to a single object. Assigning the packed object to a new tuple unpacks the individual items into the objects in the new tuple:
>>> t = ('spam', 'egg', 'bacon', 'tomato')
>>> t
('spam', 'egg', 'bacon', 'tomato')
>>> t[0]
'spam'
>>> t[1]
'egg'
>>> (s1, s2, s3, s4) = t
>>> s1
'spam'
>>> s2
'egg'
>>> s3
'bacon'
>>> s4
'tomato'
>>> (s1, s2, s3) = t
Traceback (most recent call last):
File "<input>", line 1, in <module>
(s1, s2, s3) = t
ValueError: too many values to unpack (expected 3)
>>> t
('spam', 'egg', 'bacon', 'tomato')
>>> (s1, s2, s3, s4, s5) = t
Traceback (most recent call last):
File "<input>", line 1, in <module>
(s1, s2, s3, s4, s5) = t
ValueError: not enough values to unpack (expected 5, got 4)
>>> (s1, s2, s3, s4) = ('spam', 'egg', 'bacon', 'tomato')
>>> s1
'spam'
>>> s2
'egg'
>>> s3
'bacon'
>>> s4
'tomato'
>>> (s1, s2, s3) = ('spam', 'egg', 'bacon', 'tomato')
Traceback (most recent call last):
File "<input>", line 1, in <module>
(s1, s2, s3) = t
ValueError: too many values to unpack (expected 3)
>>> t = 1, 2, 3
>>> t
(1, 2, 3)
>>> type(t)
<class 'tuple'>
>>> x1, x2, x3 = t
>>> x1, x2, x3
(1, 2, 3)
>>> x1, x2, x3 = 4, 5, 6
>>> x1, x2, x3
(4, 5, 6)
>>> t = 2,
>>> t
(2,)
>>> type(t)
<class 'tuple'>
>>> a = 'spam'
>>> b = 'egg'
>>> a, b
('spam', 'egg')
>>> # You need to define a temp variable to accomplish the swap.
>>> temp = a
>>> a = b
>>> b = temp
>>> a, b
('egg', 'spam')
>>> a = 'spam'
>>> b = 'egg'
>>> a, b
('spam', 'egg')
>>> # Ready for Magic Time!
>>> a, b = b, a
>>> a, b
('egg', 'spam')
>>> # Fibonacci series
>>> # the sum of two elements defines the next
>>> a, b = 0, 1
>>> while a < 30:
... print(a)
... a, b = b, a+b
...
0
1
1
2
3
5
8
13
21
00:00
In this video, I’m going to show you tuple assignment through packing and unpacking. A literal tuple containing several items can be assigned to a single object, such as the example object here, t
.
00:16 Assigning that packed object to a new tuple, unpacks the individual items into the objects in that new tuple. When unpacking, the number of variables on the left have to match the number of values that are inside of the tuple.
00:33 Let me have you explore that with some code. As you saw in the previous video, you can create a tuple just by typing the objects into the set of parentheses, and that will pack them all in there, into that single object. Again, you can access them via index.
00:56
Here’s an interesting idea. You can create another tuple of objects—in this case, (s1, s2, s3, s4)
, and you could assign it to the tuple that you created a moment ago, t
.
01:13
Now, s1
, s2
, s3
, and s4
will have unpacked that tuple during that assignment and placed them into the appropriate objects. It’s pretty neat!
01:27
Now, it’s important that they have the same number on both sides of that assignment. If you tried to assign it to (s1, s2, s3) = t
, you are going to raise an exception here, a ValueError
. There’s too many values to unpack.
01:41
It was expecting three on this side and t
, as you know, has four. So again, here’s t
. And what if you went with too many? Well, in this case, it was expecting to get five, but t
only provided four. Packing and unpacking could be done into one statement if you wanted to.
02:12 And here they all are. Again, the two sides have to be equal.
02:23 Here, again, too many values. When doing assignments like this, there’s a handful of situations where Python’s going to allow you to skip the parentheses.
02:41 And the same with the unpacking.
02:50 You can even do something like this, where both sides don’t have parentheses.
03:02 Even creating that singleton. It works the same whether the parentheses are included or not, so if you have any doubt as to whether they’re needed, go ahead and include them.
03:14 This tuple assignment allows for a bit of idiomatic Python. Frequently when programming, you have two variables whose values you need to swap. In most programming languages, it’s necessary to store one of the values into a temporary variable while the swap occurs. It would look something like this.
03:48
So you create a variable temp
, assign a
into it, assign b
into a
, and then say b = temp
. And there—you’ve swapped the two.
03:58
So again, you’re making a temporary variable that holds a
, taking b
, assigning it into a
, and then b
pulling that temp
back into it by reassigning it again.
04:08 That’s the swap. But in Python, the swap can be done with just a single tuple assignment.
04:24
Here you’re going to say a, b = b, a
. And you can see that the swap has occurred. It brings me to this kind of cool example that I saw from python.org. In teaching a little bit about programming they showed this example that I liked a lot about the Fibonacci series.
04:47
Here, we’re assigning the first two, 0
and 1
, and then creating a while
loop. And then inside the while
loop, you’re doing something very similar, just modifying it a little bit by assigning a
on the left to b
.
05:05
But you’re taking b
and you’re saying that now equals a+b
. Pretty neat! Next step is the conclusion and the course review.
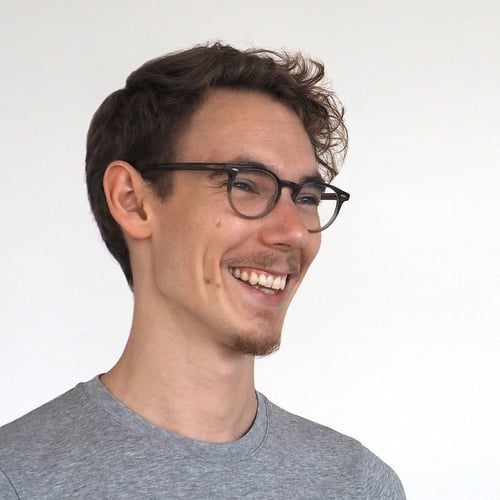
Dan Bader RP Team on May 8, 2020
The “magic” is that with Python you can do the swap without using an extra temporary variable. Many other programming languages would require the use of a temporary variable. Hope that clarifies it :)
reb24 on May 8, 2020
Ah Ok !!! Thanks Dan
kiran on July 25, 2020
Multiple Assignment (n, m = 300, 400) also called Tuple packing & unpacking?
Source: Multiple Assignment
Become a Member to join the conversation.
reb24 on May 8, 2020
If I run this:
I can’t see any magic happening or reason to use temp variable to accomplish the swap