Installing Packages With Pip
00:00 Next, you’ll learn how to install packages with pip. We’re going to cover the different ways you can install packages from PyPI, how you can install packages from other sources, like Git and other version control mechanisms, how you can update packages and how you can uninstall them again.
00:19
This is how you install packages with the pip
command line tool. Basically, you open a terminal, and you run the pip install
command, passing it the name of the package that you want to install.
00:31 And by default, this is going to install packages from the PyPI package repository. Pip also caches packages locally, to speed up repeated installs. Let’s take a look at a quick demo here.
00:44
I am in a new terminal session here, and now I am going to walk you through how to install a Python package using the pip install
command line.
00:52
So, the pip command line interface has a couple of useful commands, for example, there is the pip list
command, that I can use to inspect which packages are installed at the moment, so let’s run this.
01:03 So this lists all of the packages that are installed into my global Python environment right now, this is a really base line setup and it doesn’t really include anything besides pip and its dependencies, what I am going to do now is install a third-party package, and for that we’re going to use the Requests module.
01:21
So when you look at this list, you can see that it definitely does not include Requests right now, so let’s change that. So this is the pip install
command to install the Requests library, let’s run it now.
01:33
Alright, so the installation completed successfully, and now when I run the pip list
command again, Request should show up in that list. Yeah, here it is, we successfully installed Requests.
01:48 Let’s try it out, so I am going to jump into a Python interpreter session now, and I am going to try and import the Requests module that we just installed, alright, this succeeded, yeah, that looks pretty good, let’s try and actually fire off a real HTTP request.
02:18
Perfect, that worked just fine. Another handy command is pip show
, you can use it to display metadata and information about locally installed packages, so I am going to go ahead and run that on Requests now.
02:35 So you can see here, for example, the name and the version of the library that was installed, a quick summary, the homepage for the library, the license and also where it was installed locally on my machine.
02:47
So you just saw how to install a package from PyPI using the pip install
command, now what this will do is it will always install the latest version of the package that is currently available, so what do you do if you want to install an older, or a specific version of a package instead?
03:03
Pip has you covered there. You can actually pass so called version specifiers to package names when you install them through pip install
, with this pip install requests==2.1.3
command, I am installing a specific version of Requests and these version specifiers can get pretty flexible, for example, I can do this and actually pass a version range for this Requests module, so in this case, I would tell pip to install a version of Requests that is between version 2.0 and 3.0, so essentially, I would tell pip to install the latest version in the version 2 series of Requests.
03:44
One more feature when it comes to version specifiers, is the ~=
specifier. This tells pip to install a version of Requests that is compatible with version 2.1.3.
03:56 In practical terms, that means it’s going to try and install a version of the 2.1 branch of Requests, that is going to be at least version 2.1.3, so 2.1.4 and 2.1.5 and so on, would all be candidates for this.
04:16 Now this is handy for example if you want to get minor version updates automatically, but you still want to retain some control over larger upgrades, if you’re using version specifiers, I would generally recommend that you are very specific with them because this can help avoid surprises.
04:32 Later on in this course, we’re going to come back to these version specifiers, and you’re going to learn how to use them to set up fully reproducible Python environments for your applications.
04:42
Before you move on, here is a quick warning about installing packages globally using pip. When you use the pip install
command, by default, it will install Python packages into the global Python environment.
04:55 This means that any package you install this way is going to be shared across the whole system or across your whole user account, now this might be completely okay, if it’s done intentionally, for example, in order to install Python-based command line tools, like HTTPie in this case, you probably want to make sure that you can access the tool from anywhere in the system.
05:19 Most of the time you should prefer so called virtual environments, they are a way to keep your Python packages nice and separate by project, and that way you can make sure you’re not cluttering up the global environment, later on in this course, you are going to learn how to set up and use virtual environments, this will help you avoid version conflicts and we’ll make sure that each project you’re working on has its own Python environment, to install packages into.
05:45
Here is another useful feature for installing packages with pip. You just learned how you can install packages from a package repository like PyPI, but pip also supports other sources, for example, you can install packages directly from Git and other version control systems, here is what this would look like for installing a package that is hosted on GitHub, you would pass a URL pointing to a Git repository to the pip install
command.
06:14 And you can even control which branch or a specific commit in that repository you want to install. Here are a few examples that install the requests library directly from GitHub.
06:26
In the first example, I am putting the @master
specifier, to install the latest version of Requests directly from its master
branch on Git.
06:37 In the second example, I am using a commit hash specifier to install a very specific version of the Requests library. In the third example, I am pointing pip at a specific Git tag to install Requests from.
06:52 You can see here how this gives you a lot of flexibility, you might use this functionality to install a very specific versions of a library, for example, if you’re waiting for a bug fix to land in the official release, and you really can’t afford to wait so sometimes maybe you want to install the latest version of a library, directly from its source repository.
07:13 Or, you could use this feature to install private packages and libraries that are not available on PyPI. So you would just host your own library in a Git repository somewhere, and then point pip towards that.
07:29 Generally though, if you can install a package from a proper package repository like PyPI, then I would definitely do it. Installing a Python package directly from a version control system is a bit of a special case.
07:42 Typically, you wouldn’t use that functionality to install any old publicly available package, but you would reserve it for those special moments when you really need to be on the cutting edge and maybe need to install a specific commit of a package to get your program to work.
07:59 This is definitely useful but I would also handle it with care, and stick to PyPI when possible.
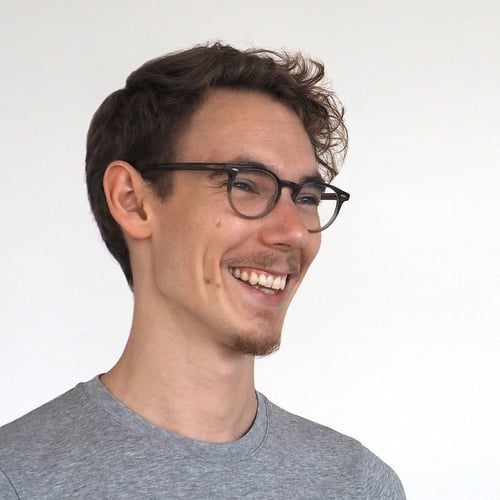
Dan Bader RP Team on Sept. 18, 2019
The built-in dir
function returns a list of valid attributes for an object. So these are not directory names or anything related to the filesystem, but methods and variables on the requests
module.
If you see a 'get'
in there for example it means there’s a requests.get
attribute (in this case it’s the .get()
method). Hope this helps you out :)
brunofl on Jan. 2, 2020
Nice! I had no idea about this feature of using pip to install directly from git repo.
theartofdonaldlsmith on Nov. 27, 2020
Dan, Sometimes you illustrate with just pip, other times with pip3. How do we know when to use which? or does it matter?
Thanks! Don
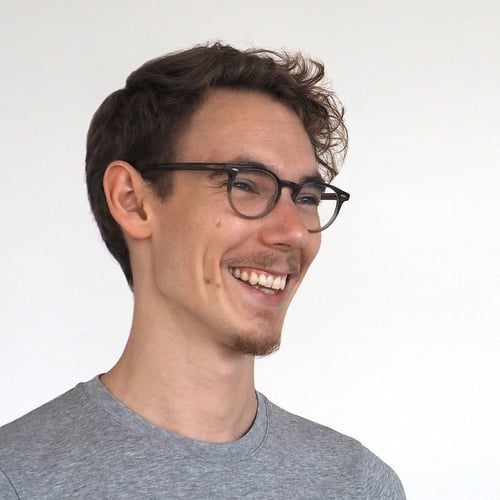
Dan Bader RP Team on Nov. 28, 2020
Once you’ve activated the virtual environment pip
will refer to the correct version of the pip package manager automatically, so you no longer need to use the pip3
command at that point.
theartofdonaldlsmith on Nov. 28, 2020
Dan,
I guess this will make more since to me after I learn more about virtual environments. I know what virtual environments are and I’ve used them for running Windows 7 in on a windows 10 computer. I use PyCharm and it setups up a VE automatically for each project. After finishing all of lesson 2, I now understand the value of using VEs.
At first I was skeptical of buying this course. I figured I could watch the first lesson and if I wasn’t learning anything useful I could get a refund. While I’m retired and learning Python for fun, I’m glad I bought your course. I’ve taken a lot of python classes online, but none of them have even touched PyPI and the package library. I’ve seen it, but just thought it was another package like NumPy, and I didn’t fully understand it’s purpose.
Thanks! Don
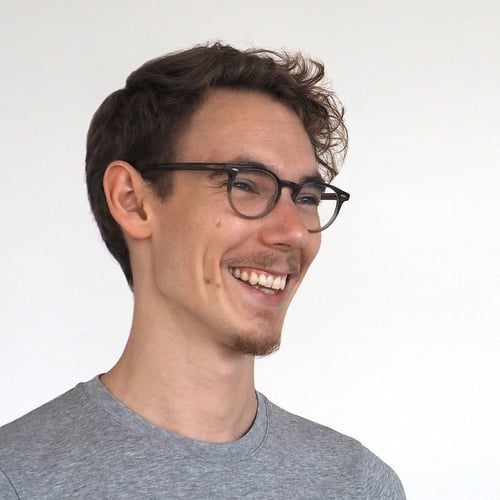
Dan Bader RP Team on Nov. 30, 2020
Thanks for your comment there Don, I really appreciate the feedback. Happy Pythoning :)
Ozzy Campos on Feb. 17, 2021
Great course - One thing I’d note is that it’s incredibly common in many circles to have to collaborate with Anaconda, it would be great to get more info on how pip and anaconda (and brew) interact and relate. When I started doing some installs with pip and some with anaconda all my scripts (mainly geospatial stuff) that used to work just in Anaconda are now all messed up. I think I’m going to finish this course and then start fresh with all my environments.
Anonymous on March 7, 2022
i’m very sleep deprived and i did something stupid. following this tutorial i somehow screwed up and uninstalled pip and pip3 (at least i thonk thats what i did). bash didn’t even recognize any pip commands after that. to try and fix the problem i went into a the envs folder in anaconda3 and copied the pip and pip3 files from an environment i had previously created and then pasted them in user/opt/anaconda3/bin. that seemed to work (i was able to use the pip list command) but I dont know if it will cause problems down fhe line.
Become a Member to join the conversation.
AugustoVal on Sept. 18, 2019
Hello Dan,
I hope you are doing well,
Quick question. Are these requests directories?
Thanking you in advances