Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: What Does if __name__ == "__main__" Mean in Python?
You’ve likely encountered Python’s if __name__ == "__main__"
idiom when reading other people’s code. No wonder—it’s widespread! You might have even used if __name__ == "__main__"
in your own scripts. But did you use it correctly?
Maybe you’ve programmed in a C-family language like Java before, and you wonder whether this construct is a clumsy accessory to using a main()
function as an entry point.
Syntactically, Python’s if __name__ == "__main__"
idiom is just a normal conditional block:
1if __name__ == "__main__":
2 ...
The indented block starting in line 2 contains all the code that Python will execute when the conditional statement in line 1 evaluates to True
. In the code example above, the specific code logic that you’d put in the conditional block is represented with a placeholder ellipsis (...
).
So—if there’s nothing special about the if __name__ == "__main__"
idiom, then why does it look confusing, and why does it continue to spark discussion in the Python community?
If the idiom still seems a little cryptic, and you’re not completely sure what it does, why you might want it, and when to use it, then you’ve come to the right place! In this tutorial, you’ll learn all about Python’s if __name__ == "__main__"
idiom—starting with what it really does in Python, and ending with a suggestion for a quicker way to refer to it.
Take the Quiz: Test your knowledge with our interactive “Python Name-Main Idiom” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Python Name-Main IdiomTest your knowledge of Python's if __name__ == "__main__" idiom by answering a series of questions! You've probably encountered the name-main idiom and might have even used it in your own scripts. But did you use it correctly?
Source Code: Click here to download the free source code that you’ll use to understand the name-main idiom.
In Short: It Allows You to Execute Code When the File Runs as a Script, but Not When It’s Imported as a Module
For most practical purposes, you can think of the conditional block that you open with if __name__ == "__main__"
as a way to store code that should only run when your file is executed as a script.
You’ll see what that means in a moment. For now, say you have the following file:
1# echo.py
2
3def echo(text: str, repetitions: int = 3) -> str:
4 """Imitate a real-world echo."""
5 echoed_text = ""
6 for i in range(repetitions, 0, -1):
7 echoed_text += f"{text[-i:]}\n"
8 return f"{echoed_text.lower()}."
9
10if __name__ == "__main__":
11 text = input("Yell something at a mountain: ")
12 print(echo(text))
In this example, you define a function, echo()
, that mimics a real-world echo by gradually printing fewer and fewer of the final letters of the input text.
Below that, in lines 10 to 12, you use the if __name__ == "__main__"
idiom. This code starts with the conditional statement if __name__ == "__main__"
in line 10. In the indented lines, 11 and 12, you then collect user input and call echo()
with that input. These two lines will execute when you run echo.py
as a script from your command line:
$ python echo.py
Yell something at a mountain: HELLOOOO ECHOOOOOOOOOO
ooo
oo
o
.
When you run the file as a script by passing the file object to your Python interpreter, the expression __name__ == "__main__"
returns True
. The code block under if
then runs, so Python collects user input and calls echo()
.
Try it out yourself! You can download all the code files that you’ll use in this tutorial from the link below:
Source Code: Click here to download the free source code that you’ll use to understand the name-main idiom.
At the same time, if you import echo()
in another module or a console session, then the nested code won’t run:
>>> from echo import echo
>>> print(echo("Please help me I'm stuck on a mountain"))
ain
in
n
.
In this case, you want to use echo()
in the context of another script or interpreter session, so you won’t need to collect user input. Running input()
would mess with your code by producing a side effect when importing echo
.
When you nest the code that’s specific to the script usage of your file under the if __name__ == "__main__"
idiom, then you avoid running code that’s irrelevant for imported modules.
Nesting code under if __name__ == "__main__"
allows you to cater to different use cases:
- Script: When run as a script, your code prompts the user for input, calls
echo()
, and prints the result. - Module: When you import
echo
as a module, thenecho()
gets defined, but no code executes. You provideecho()
to the main code session without any side effects.
By implementing the if __name__ == "__main__"
idiom in your code, you set up an additional entry point that allows you to use echo()
right from the command line.
There you go! You’ve now covered the most important information about this topic. Still, there’s more to find out, and there are some subtleties that can help you build a deeper understanding of this code specifically and Python more generally.
Read on to learn more about the name-main idiom, as this tutorial will refer to it for short.
How Does the Name-Main Idiom Work?
At its core, the idiom is a conditional statement that checks whether the value of the variable __name__
is equal to the string "__main__"
:
- If the
__name__ == "__main__"
expression isTrue
, then the indented code following the conditional statement executes. - If the
__name__ == "__main__"
expression isFalse
, then Python skips the indented code.
But when is __name__
equal to the string "__main__"
? In the previous section, you learned that this is the case when you run your Python file as a script from the command line. While that covers most real-life use cases, maybe you want to go deeper.
Python sets the global __name__
of a module equal to "__main__"
if the Python interpreter runs your code in the top-level code environment:
“Top-level code” is the first user-specified Python module that starts running. It’s “top-level” because it imports all other modules that the program needs. (Source)
To better understand what that means, you’ll set up a small practical example. Create a Python file, call it namemain.py
, and add one line of code:
# namemain.py
print(__name__, type(__name__))
Your new file contains only a single line of code that prints the value and type of the global __name__
to the console.
Spin up your terminal and run the Python file as a script:
$ python namemain.py
__main__ <class 'str'>
The output shows you that the value of __name__
is the Python string "__main__"
if you run your file as a script.
Note: In the top-level code environment, the value of __name__
is always "__main__"
. The top-level code environment is often a module that you pass to the Python interpreter as a file argument, as you saw above. However, there are other options that can constitute the top-level code environment:
- The scope of an interactive prompt
- The Python module or package passed to the Python interpreter with the
-m
option, which stands for module - Python code read by the Python interpreter from standard input
- Python code passed to the Python interpreter with the
-c
option, which stands for command
If you’re curious to learn more about these options, then check out the Python documentation on what the top-level code environment is. The documentation illustrates each of these bullet points with a concise code snippet.
Now you know the value of __name__
when your code is executed in the top-level code environment.
But a conditional statement can only produce different results when the condition has a chance to evaluate in different ways. So, when is your code not run in the top-level code environment, and what happens to the value of __name__
in that case?
The code in your file isn’t run in the top-level code environment if you import your module. In that case, Python sets __name__
to the module’s name.
To try this out, start a Python console and import the code from namemain.py
as a module:
>>> import namemain
namemain <class 'str'>
Python executes the code stored in the global namespace of namemain.py
during the import, which means it’ll call print(__name__, type(__name__))
and write the output to the console.
In this case, however, the value of the module’s __name__
is different. It points to "namemain"
, a string that’s equal to the module’s name.
Note: You can import any file that contains Python code as a module, and Python will run the code in your file during import. The name of the module will usually be the filename without the file extension for Python files (.py
).
You just learned that for your top-level code environment, __name__
is always "__main__"
, so go ahead and confirm that within your interpreter session. Also check where the string "namemain"
comes from:
>>> __name__
'__main__'
>>> namemain.__name__
'namemain'
The global __name__
has the value "__main__"
, and .__name__
for the imported namemain
module has the value "namemain"
, which is the module’s name as a string.
Note: Most of the time, the top-level code environment will be the Python script that you execute and where you’re importing other modules. However, in this example, you can see that the top-level code environment isn’t strictly tied to a script run and can also be, for example, an interpreter session.
Now you know that the value of __name__
will have one of two values depending on where it lives:
- In the top-level code environment, the value of
__name__
is"__main__"
. - In an imported module, the value of
__name__
is the module’s name as a string.
Because Python follows these rules, you can find out whether or not a module is running in the top-level code environment. You do this by checking the value of __name__
with a conditional statement, which brings you full circle to the name-main idiom:
# namemain.py
print(__name__, type(__name__))
if __name__ == "__main__":
print("Nested code only runs in the top-level code environment")
With this conditional check in place, you can declare code that only executes when the module is run in the top-level code environment.
Add the idiom to namemain.py
as shown in the code block above, then run the file as a script once more:
$ python namemain.py
__main__ <class 'str'>
Nested code only runs in the top-level code environment
When running your code as a script, both calls to print()
execute.
Next, start a new interpreter session and import namemain
as a module once more:
>>> import namemain
namemain <class 'str'>
When you import your file as a module, the code that you nested under if __name__ == "__main__"
doesn’t execute.
Now that you know how the name-main idiom works in Python, you may wonder when and how exactly you should use it in your code—and when to avoid it!
When Should You Use the Name-Main Idiom in Python?
You use this idiom when you want to create an additional entry point for your script, so that your file is accessible as a stand-alone script as well as an importable module. You might want that when your script needs to collect user input.
In the first section of this tutorial, you used the name-main idiom together with input()
to collect user input when running echo.py
as a script. That’s a great reason to use the name-main idiom!
There are also other ways to collect user input directly from the command line. For example, you could create a command-line entry point for a small Python script with sys.argv
and the name-main idiom:
1# echo.py
2
3import sys
4
5def echo(text: str, repetitions: int = 3) -> str:
6 """Imitate a real-world echo."""
7 echoed_text = ""
8 for i in range(repetitions, 0, -1):
9 echoed_text += f"{text[-i:]}\n"
10 return f"{echoed_text.lower()}."
11
12if __name__ == "__main__":
13 text = " ".join(sys.argv[1:])
14 print(echo(text))
Instead of collecting user input with input()
, you changed the code in echo.py
so that your users can provide the text as arguments directly from the command line:
$ python echo.py HELLOOOOO ECHOOOO
ooo
oo
o
.
Python collects an arbitrary number of words into sys.argv
, which is a list of strings that represents all inputs. Each word is considered a new argument when a whitespace character separates it from the others.
By taking the code execution that handles user input and nesting it in the name-main idiom, you provide an additional entry point to your script.
If you want to create an entry point for a package, then you should create a dedicated __main__.py
file for that purpose. This file represents an entry point that Python invokes when you run your package using the -m
option:
$ python -m venv venv
When you create a virtual environment using the venv
module, as shown above, then you run code defined in a __main__.py
file. The -m
option followed by the module name venv
invokes __main__.py
from the venv
module.
Because venv
is a package rather than a small command-line interface (CLI) script, it has a dedicated __main__.py
file as its entry point.
Note: There are additional advantages of nesting code, such as user input collection, under the name-main idiom. Because that nested code doesn’t execute during module imports, you can run unit tests from a separate testing module on your functions without producing side effects.
Side effects could otherwise occur because a testing module needs to import your module to run tests against your code.
In the wild, you may encounter many more reasons for using the name-main idiom in Python code. However, collecting user input, either through standard input or the command-line, is the primary suggested reason for using it.
When Should You Avoid the Name-Main Idiom?
Now that you’ve learned when to use the name-main idiom, it’s time to find out when it’s not the best idea to use it. You may be surprised to learn that in many cases, there are better options than nesting your code under if __name__ == "__main__"
in Python.
Sometimes, developers use the name-main idiom to add test runs to a script that combines code functionality and tests in the same file:
# adder.py
import unittest
def add(a: int, b: int) -> int:
return a + b
class TestAdder(unittest.TestCase):
def test_add_adds_two_numbers(self):
self.assertEqual(add(1, 2), 3)
if __name__ == "__main__":
unittest.main()
With this setup, you can run tests against your code when you execute it as a script:
$ python adder.py
.
----------------------------------------------------------------------
Ran 1 test in 0.000s
OK
Because you ran the file as a script, __name__
was equal to "__main__"
, the conditional expression returned True
, and Python called unittest.main()
. The tiny test suite ran, and your test succeeded.
At the same time, you didn’t create any unexpected code execution when importing your code as a module:
>>> import adder
>>> adder.add(1, 2)
3
It’s still possible to import the module and use the function that you’ve defined there. The unit test won’t run unless you execute the module in the top-level code environment.
While this works for small files, it’s generally not considered good practice. It’s not advised to mix tests and code in the same file. Instead, write your tests in a separate file. Following this advice generally makes for a more organized code base. This approach also removes any overhead, such as the need to import unittest
in your main script file.
Another reason that some programmers use the name-main idiom is to include a demonstration of what their code can do:
# echo_demo.py
def echo(text: str, repetitions: int = 3) -> str:
"""Imitate a real-world echo."""
echoed_text = ""
for i in range(repetitions, 0, -1):
echoed_text += f"{text[-i:]}\n"
return f"{echoed_text.lower()}."
if __name__ == "__main__":
print('Example call: echo("HELLO", repetitions=2)', end=f"\n{'-' * 42}\n")
print(echo("HELLO", repetitions=2))
Again, your users can still import the module without any side effects. Additionally, when they run echo_demo.py
as a script, they get a peek at its functionality:
$ python echo_demo.py
Example call: echo("HELLO", repetitions=2)
------------------------------------------
lo
o
.
You may find such demo code executions in the name-main idiom, but there are arguably much better ways to demonstrate how to use your program. You can write detailed docstrings with example runs that can double as doctests, and you can compose proper documentation for your project.
The previous two examples cover two common suboptimal use cases of the name-main idiom. There are also other scenarios when it’s best to avoid the name-main idiom in Python:
-
A pure script: If you write a script that’s meant to be run as a script, then you can put your code execution into the global namespace without nesting it in the name-main idiom. You can use Python as a scripting language because it doesn’t enforce strong object-oriented patterns. You don’t have to stick to design patterns from other languages when you program in Python.
-
A complex command-line program: If you write a larger command-line application, then it’s best to create a separate file as your entry point. You then import the code from your module there instead of handling user input with the name-main idiom. For more complex command-line programs, you’ll also benefit from using the built-in
argparse
module instead ofsys.argv
.
Maybe you’ve used the name-main idiom for one of these suboptimal purposes before. If you want to learn more about how to write more idiomatic Python for each of these scenarios, then follow the provided links:
❌ Suboptimal Use Case | ✅ Better Alternative |
---|---|
Test code execution | Create a dedicated testing module |
Demonstrate code | Build project documentation and include examples in your docstrings |
Create a pure script | Run as a script |
Provide a complex CLI program entry point | Create a dedicated CLI module |
Even though you now know when to avoid the name-main idiom, you might still wonder about how to best use it in a valid scenario.
In What Way Should You Include the Name-Main Idiom?
The name-main idiom in Python is just a conditional statement, so you could use it anywhere in your file—even more than once! For most use cases, however, you’ll put one name-main idiom at the bottom of your script:
# All your code
if __name__ == "__main__":
...
You put the name-main idiom at the end of your script because the entry point for a Python script is always the top of the file. If you put the name-main idiom at the bottom of your file, then all your functions and classes get defined before Python evaluates the conditional expression.
Note: In Python, the code in a function or class body doesn’t run during definition. That code only executes when you call a function or instantiate a class.
However, although it’s uncommon to use more than one name-main idiom in a script, there may be a reason to do so in some cases. Python’s style guide document, PEP 8, is clear about where to put all your import statements:
Imports are always put at the top of the file, just after any module comments and docstrings, and before module globals and constants. (Source)
This is why you imported sys
at the top of the file in echo.py
:
# echo.py
import sys
def echo(text: str, repetitions: int = 3) -> str:
"""Imitate a real-world echo."""
echoed_text = ""
for i in range(repetitions, 0, -1):
echoed_text += f"{text[-i:]}\n"
return f"{echoed_text.lower()}."
if __name__ == "__main__":
text = " ".join(sys.argv[1:])
print(echo(text))
However, you don’t even need to import sys
at all when you simply want to import echo
as a module.
To address this and still stick with the style suggestions defined in PEP 8, you could use a second name-main idiom. By nesting the import of sys
in a name-main idiom, you can keep all imports at the top of the file but avoid importing sys
when you won’t need to use it:
# echo.py
if __name__ == "__main__":
import sys
def echo(text: str, repetitions: int = 3) -> str:
"""Imitate a real-world echo."""
echoed_text = ""
for i in range(repetitions, 0, -1):
echoed_text += f"{text[-i:]}\n"
return f"{echoed_text.lower()}."
if __name__ == "__main__":
text = " ".join(sys.argv[1:])
print(echo(text))
You nested the import of sys
under another name-main idiom. That way, you keep the import statement at the top of your file, but you avoid importing sys
when you use echo
as a module.
Note: You likely won’t encounter this setup frequently, but it serves as an example of when it may be helpful to use multiple name-main idioms in one file.
Still, readability counts, so keeping the import statement at the top without the second name-main idiom will often be the better choice. However, a second name-main idiom might come in handy if you’re working in an environment with restricted resources.
As you learned earlier in the tutorial, there are fewer occasions to use the name-main idiom than you might expect. For most of those use cases, putting one of these conditional checks at the bottom of your script will be your best choice.
Finally, you may wonder what code should go into the conditional code block. The Python documentation offers clear guidance about the idiomatic usage of the name-main idiom in that regard:
Putting as few statements as possible in the block below
if __name___ == '__main__'
can improve code clarity and correctness. (Source)
Keep the code under your name-main idiom to a minimum! When you start putting multiple lines of code nested under the name-main idiom, then you should instead define a main()
function and call that function:
# echo.py
import sys
def echo(text: str, repetitions: int = 3) -> str:
"""Imitate a real-world echo."""
echoed_text = ""
for i in range(repetitions, 0, -1):
echoed_text += f"{text[-i:]}\n"
return f"{echoed_text.lower()}."
def main() -> None:
text = " ".join(sys.argv[1:])
print(echo(text))
if __name__ == "__main__":
main()
This design pattern gives you the advantage that the code under the name-main idiom is clear and concise. Additionally, it makes it possible to call main()
even if you’ve imported your code as a module, for example to unit test its functions.
Note: Defining main()
in Python means something different from in other languages, such as Java and C. In Python, naming this function main is just a convention. You could name the function anything—and as you’ve seen before, you don’t even need to use it at all.
Other object-oriented languages define a main()
function as the entry point for a program. In that case, the interpreter implicitly calls a function named main()
, and your program won’t work without it.
In this section, you’ve learned that you should probably write the name-main idiom at the bottom of your script.
If you have multiple lines of code that you’re planning to nest under if __name__ == "__main__"
, then it’s better to refactor that code into a main()
function that you call from the conditional block under the name-main idiom.
Now that you know how you can use the name-main idiom, you may wonder why it looks more cryptic than other Python code that you’re used to.
Is the Idiom Boilerplate Code That Should Be Simplified?
If you’re coming from a different object-oriented programming language, you might think that Python’s name-main idiom is an entry point akin to the main()
functions in Java or C, but clumsier:
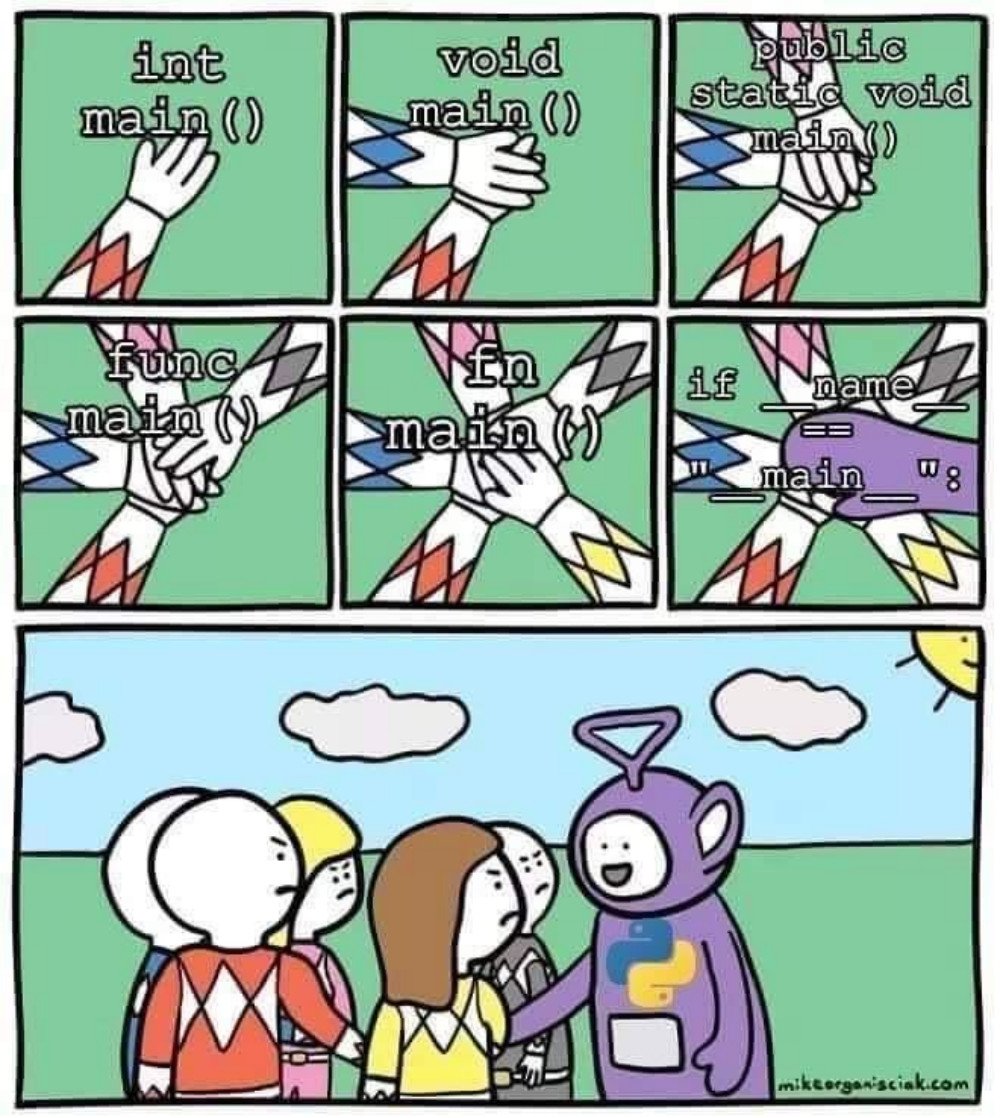
While definitely funny and relatable, this meme is misleading because it implies that the name-main idiom is comparable to main()
entry point functions in other languages.
Python’s name-main idiom isn’t special. It’s just a conditional check. It may look cryptic at first, especially when you’re starting to work with Python and you’re used to Python’s slim and elegant syntax. After all, the name-main idiom includes a dunder variable from the global namespace, and a string that’s a dunder value as well.
So it’s not the type of entry point that main represents in other languages. But why does it look the way it does? You may have copied and pasted the idiom many times, or even typed it out, and wondered why Python doesn’t have a more concise syntax for it.
If you browse the archives of the Python-ideas mailing list, rejected PEPs, and the Python discussion forum, then you’ll find a number of attempts to change the idiom.
If you read some of these discussions, then you’ll notice that many seasoned Pythonistas argue that the idiom isn’t cryptic and shouldn’t be changed. They give multiple reasons:
- It’s short: Most suggested changes save only two lines of code.
- It has a limited use case: You should only use it if you need to run a file both as a module as well as a script. You shouldn’t need to use it very often.
- It exposes complexity: Dunder variables and methods are a big part of Python once you peek a bit deeper. That can make the current idiom an entry point for learners that sparks curiosity and gives them a first look under the hood of Python’s syntax.
- It maintains backward compatibility: The name-main idiom has been a de facto standard in the language for a long time, which means that changing it would break backward compatibility.
Okay, so you’re stuck with if __name__ == "__main__"
for the time being. It seems like it’d be helpful to find a good way to refer to it consistently and concisely!
Expand the section below for a bit of context and a few suggestions on how you could talk about the name-main idiom without twisting your tongue or knotting your fingers:
You’ll probably discuss using the name-main idiom at some point in your Python career. It’s a long expression to write and even more cumbersome to say out loud, so you might as well find a good way to talk about it.
There are different ways to refer to it in the Python community. Most mentions online include the whole if __name__ == "__main__"
expression followed by a word:
- The
if __name__ == "__main__"
convention (Source) - The
if __name__ == "__main__"
expression (Source) - The
if __name__ ...
idiom (Source) - The
if __name__ == "__main__": ...
idiom (Source) - The
if __name__ == "__main__"
idiom (Source) - The executable stanza (Source)
As you may notice, there’s no strict convention around how to talk about if __name__ == "__main__"
, but you probably won’t do wrong if you follow the general consensus of calling it the if __name__ == "__main__"
idiom.
If you want to promote standardizing a shorter name for the idiom, then tell your friends to call it the name-main idiom. That’s what we’ll be calling it at Real Python! If you find the term useful, then maybe it’ll catch on.
If you’re curious, take a plunge into some of the linked discussions in the various Python community channels to learn more about why developers argue for keeping the name-main idiom as it is.
Conclusion
You’ve learned what the if __name__ == "__main__"
idiom does in Python. It allows you to write code that executes when you run the file as a script, but not when you import it as a module. It’s best to use it when you want to collect user input during a script run and avoid side effects when importing your module—for example, to unit test its functions.
You also got to know some common but suboptimal use cases and learned about better and more idiomatic approaches that you can take in those scenarios. Maybe you’ve accepted Python’s name-main idiom after learning more about it, but if you still dislike it, then it’s good to know that you can probably replace its use in most cases.
When do you use the name-main idiom in your Python code? While reading this tutorial, did you discover a way to replace it, or is there a good use case that we missed? Share your thoughts in the comments below.
Take the Quiz: Test your knowledge with our interactive “Python Name-Main Idiom” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Python Name-Main IdiomTest your knowledge of Python's if __name__ == "__main__" idiom by answering a series of questions! You've probably encountered the name-main idiom and might have even used it in your own scripts. But did you use it correctly?
Source Code: Click here to download the free source code that you’ll use to understand the name-main idiom.
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: What Does if __name__ == "__main__" Mean in Python?