Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: The Square Root Function in Python
The Python square root function, sqrt()
, is part of the math
module and is used to calculate the square root of a given number. To use it, you import the math
module and call math.sqrt()
with a non-negative number as an argument. For example, math.sqrt(9)
returns 3.0
.
This function works with both integers and floats and is essential for mathematical operations like solving equations and calculating geometric properties. In this tutorial, you’ll learn how to effectively use the square root function in Python.
By the end of this tutorial, you’ll understand how:
- Python’s
sqrt()
function calculates square roots using Python’smath.sqrt()
for quick and accurate results in your programs. math.sqrt()
calculates the square root of positive numbers and zero but raises an error for negative inputs.- Python’s square root function can be used to solve real-world problems like calculating distances using the Pythagorean theorem.
Time to dive in!
Python Pit Stop: This tutorial is a quick and practical way to find the info you need, so you’ll be back to your project in no time!
Free Bonus: Click here to get our free Python Cheat Sheet that shows you the basics of Python 3, like working with data types, dictionaries, lists, and Python functions.
Square Roots in Mathematics
In algebra, a square, x, is the result of a number, n, multiplied by itself: x = n²
You can calculate squares using Python:
>>> n = 5
>>> x = n**2
>>> x
25
The Python **
operator is used for calculating the power of a number. In this case, 5 squared, or 5 to the power of 2, is 25.
The square root, then, is the number n, which when multiplied by itself yields the square, x.
In this example, n, the square root of 25, is 5.
25 is an example of a perfect square. Perfect squares are the squares of integer values:
>>> 1**2
1
>>> 2**2
4
>>> 3**2
9
You might have memorized some of these perfect squares when you learned your multiplication tables in an elementary algebra class.
If you’re given a small perfect square, it may be straightforward enough to calculate or memorize its square root. But for most other squares, this calculation can get a bit more tedious. Often, an estimation is good enough when you don’t have a calculator.
Fortunately, as a Python developer, you do have a calculator, namely the Python interpreter!
The Python Square Root Function
Python’s math
module in the standard library can help you work on math-related problems in code. It contains many useful functions, such as remainder()
and factorial()
. It also includes the Python square root function, sqrt()
.
You’ll begin by importing math
:
>>> import math
That’s all it takes! You can now use math.sqrt()
to calculate square roots.
sqrt()
has a straightforward interface. It takes one parameter, x
, which as you saw before, stands for the square you want to calculate the square root for. In the example from earlier, this would be 25
.
The return value of sqrt()
is the square root of x
, as a floating-point number. In the example, this would be 5.0
.
Next, you’ll take a look at some examples of how to use sqrt()
and how not to use sqrt()
.
The Square Root of a Positive Number
One type of argument you can pass to sqrt()
is a positive number. This includes both int
and float
types.
For example, you can solve for the square root of 49
using sqrt()
:
>>> math.sqrt(49)
7.0
The return value is 7.0
, the square root of 49
, as a floating-point number.
Along with integers, you can also pass float
values:
>>> math.sqrt(70.5)
8.396427811873332
You can verify the accuracy of this square root by calculating its inverse:
>>> 8.396427811873332**2
70.5
The Square Root of Zero
Even 0
is a valid square to pass to the Python square root function:
>>> math.sqrt(0)
0.0
While you probably won’t need to calculate the square root of zero often, you may be passing a variable to sqrt()
whose value you don’t actually know. So, it’s good to know that it can handle zero in those cases.
The Square Root of Negative Numbers
The square of any real number can’t be negative. This is because a negative product is only possible if one factor is positive and the other is negative. A square, by definition, is the product of a number and itself, so it’s impossible to have a negative real square:
>>> math.sqrt(-25)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: math domain error
If you attempt to pass a negative number to sqrt()
, then you’ll get a ValueError
because negative numbers aren’t in the domain of possible real squares. Instead, the square root of a negative number would need to be complex, which is outside the scope of the Python square root function.
Square Roots in the Real World
To see a real-world application of the Python square root function, you’ll turn to the sport of tennis.
Imagine that Alex de Minaur, one of the fastest players in the world, has just hit a forehand from the back corner, where the baseline meets the sideline of the tennis court:
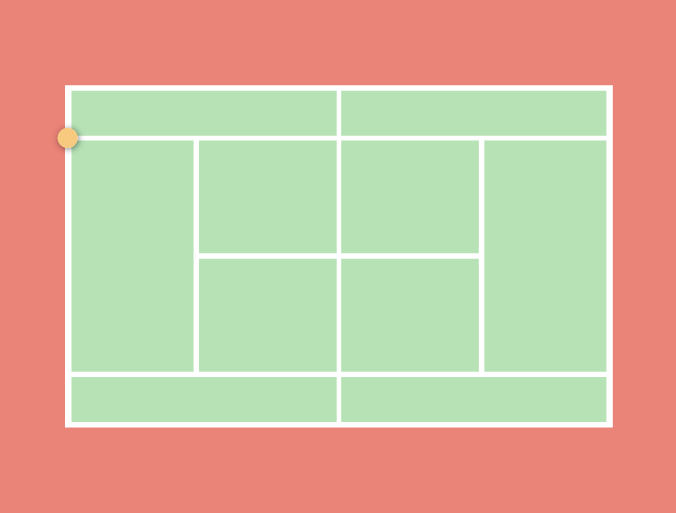
Now, assume his opponent has countered with a drop shot—one that would place the ball short with little forward momentum—to the opposite corner, where the other sideline meets the net:
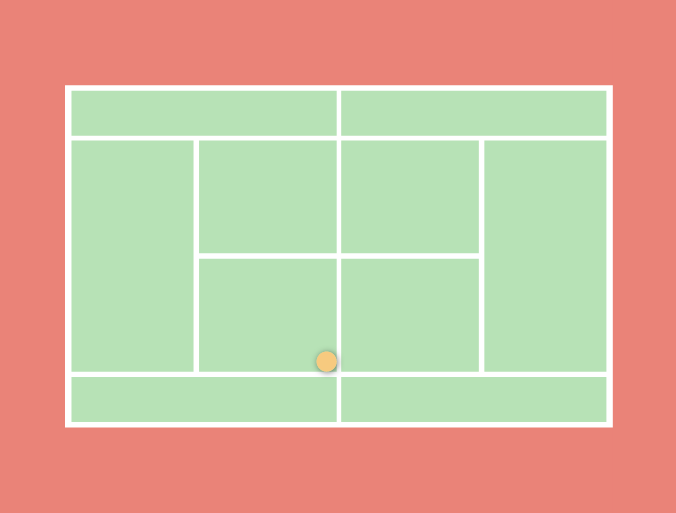
How far must Nadal run to reach the ball?
You can determine from regulation tennis court dimensions that the baseline is 27 feet long, and the sideline on one side of the net is 39 feet long. So, essentially, this boils down to solving for the hypotenuse of a right triangle:
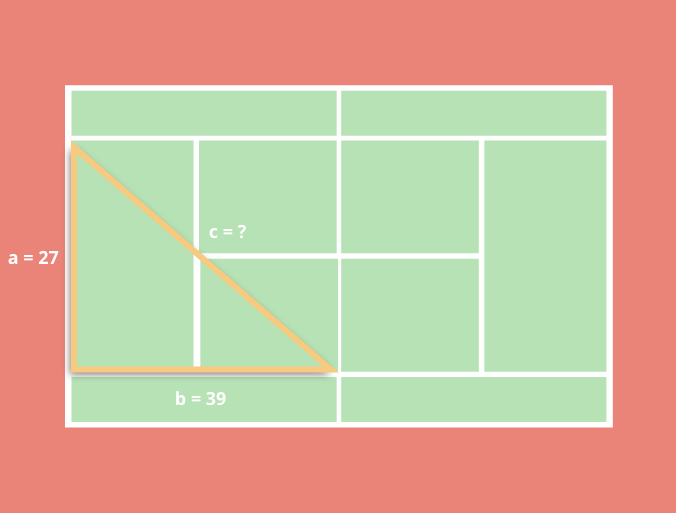
Using a valuable equation from geometry, the Pythagorean theorem, you know that a² + b² = c², where a and b are the legs of the right triangle and c is the hypotenuse.
Therefore, you can calculate the distance Nadal must run by rearranging the equation to solve for c:
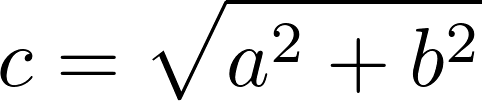
You can solve this equation using the Python square root function:
>>> a = 27
>>> b = 39
>>> math.sqrt(a**2 + b**2)
47.43416490252569
So, Nadal must run about 47.4 feet (14.5 meters) in order to reach the ball and save the point.
Conclusion
Congratulations! You now know all about the Python square root function.
In this tutorial, you’ve covered:
- A brief introduction to square roots
- The ins and outs of the Python square root function,
sqrt()
- A practical application of
sqrt()
using a real-world example
Knowing how to use sqrt()
is only part of the equation. Understanding when to use it is equally important. Now that you know both, go ahead and apply your newfound mastery of the Python square root function!
FAQs
You now have some experience with square roots in Python. Below, you’ll find a few questions and answers that sum up the most important concepts that you’ve covered in this tutorial.
You can use these questions to check your understanding or to recap and solidify what you’ve just learned. After each question, you’ll find a brief explanation hidden in a collapsible section. Click the Show/Hide toggle to reveal the answer.
To find the square root in Python, you use the math.sqrt()
function. First, import the math
module, then call math.sqrt()
with the number you want to find the square root of. For example, math.sqrt(16)
returns 4.0
.
Yes, you can calculate the square root of negative numbers in Python, but not with the math.sqrt()
function. Instead, you should use cmath.sqrt()
. Attempting to calculate the square root of a negative number with math.sqrt()
will result in a ValueError
because this function can’t handle negative numbers.
You can use both math.sqrt()
and pow()
to find a square root in Python. While both will return the same result for non-negative numbers, math.sqrt()
is more readable and specifically designed for this purpose.
To use the square root function in Python, you need to import it from the math
module. Use the statement import math
and then call math.sqrt()
to find the square root of a number.
Yes, you can calculate the square root without importing the math
module by using the exponent operator **
or the built-in pow()
function. For example, 4**0.5
will return 2.0
, giving you the same result as math.sqrt()
.
Watch Now This tutorial has a related video course created by the Real Python team. Watch it together with the written tutorial to deepen your understanding: The Square Root Function in Python