Many people learn basic language and word skills by playing the game of hangman. First mentioned in print in the late nineteenth century and popularized in international media as the game show Wheel of Fortune, hangman appeals to many people. For beginning programmers who want a challenge or for more experienced coders looking for a bit of fun, writing hangman in Python is a rewarding endeavor.
Throughout this tutorial, you’ll build the hangman game in Python in a series of steps. The game will work as a command-line application. Throughout the process, you’ll also learn the basics of building computer games.
In this tutorial, you’ll:
- Learn the common elements of a computer game
- Track the state of a computer game
- Get and validate the user’s input
- Create a text-based user interface (TUI) for your game
- Figure out when the game is over and who the winner is
To get the most from this tutorial, you should be comfortable working with Python sets and lists. You don’t need to have any previous knowledge about game writing.
All the code that you’ll write in this tutorial is available for download at the link below:
Get Your Game: Click here to download the free code that you’ll write to create a hangman game with Python.
Demo: Command-Line Hangman Game in Python
In this tutorial, you’ll write a hangman game for your command line using Python. Once you’ve run all the steps to build the game, then you’ll end up with a text-based user interface (TUI) that will work something like the following:
Throughout this tutorial, you’ll run through several challenges designed to help you solve specific game-related problems. At the end, you’ll have a hangman game that works like the demo above.
Project Overview
The first step to building a good computer game is to come up with a good design. So, the design for your hangman game should begin with a good description of the game itself. You also need to have a general understanding of the common elements that make up a computer game. These two points are crucial for you to come up with a good design.
Description of the Hangman Game
While many people know the game well, it’s helpful to have a formal description of the game. You can use this description to resolve programming issues later during development. As with many things in life, the exact description of a hangman game could vary from person to person. Here’s a possible description of the game:
- Game setup: The game of hangman is for two or more players, comprising a selecting player and one or more guessing players.
- Word selection: The selecting player selects a word that the guessing players will try to guess.
- The selected word is traditionally represented as a series of underscores for each letter in the word.
- The selecting player also draws a scaffold to hold the hangman illustration.
- Guessing: The guessing players attempt to guess the word by selecting letters one at a time.
- Feedback: The selecting player indicates whether each guessed letter appears in the word.
- If the letter appears, then the selecting player replaces each underscore with the letter as it appears in the word.
- If the letter doesn’t appear, then the selecting player writes the letter in a list of guessed letters. Then, they draw the next piece of the hanged man. To draw the hanged man, they begin with the head, then the torso, the arms, and the legs for a total of six parts.
- Winning conditions: The selecting player wins if the hanged man drawing is complete after six incorrect guesses, in which case the game is over. The guessing players win if they guess the word.
- If the guess is right, the game is over, and the guessing players win.
- If the guess is wrong, the game continues.
A game in progress is shown below. In this game, the word to be guessed is hangman:
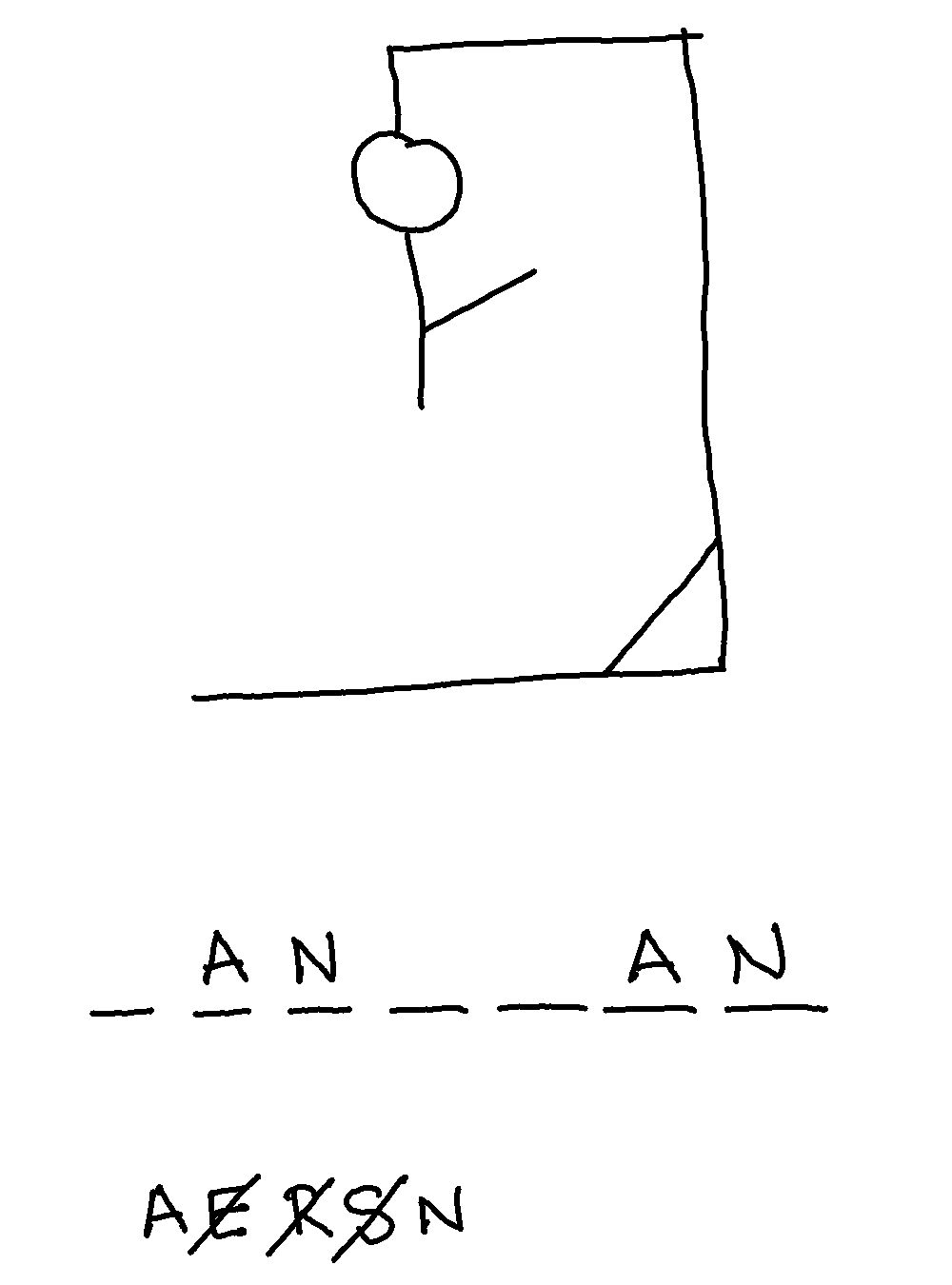
In this tutorial, to write the hangman game in Python, you’ll make a few additional design decisions:
- The game will be between the computer and one human player.
- The computer will act as the selecting player and will select the word to guess, process human input, and handle all output.
- The human player is the guessing player, hereafter simply referred to as the player. When the player knows the word, they continue to guess correct letters until the word is complete.
With this basic understanding of the game and some design decisions for the computer version, you can begin creating the game. First, however, you need to know the common elements of computer games and understand how they interact to produce the desired result.
Common Elements of a Computer Game
Every game, whether it’s a computer game or not, has a set of elements that make it a game. Without these elements, there’s no game:
- Initial setup: You get the game ready to play. This might mean setting the pieces on a chess board, dealing out cards, or rolling dice to see who goes first.
- Gameplay: When people think of the game, this is what they normally think of. The flow of gameplay is controlled by a game loop, which keeps the game moving and doesn’t end until the game is over. The game loop ensures the following happens:
- The user input is gathered and processed.
- The game state is updated based on reactions to the user input. This may include checking for end-of-game conditions.
- Any required output changes are made to reflect the new game state.
- The game loop repeats.
- Game ending: How the game ends depends on the game itself. Whether capturing the enemy king in checkmate, achieving a certain score in a card game, or having your piece cross the line in a board game, certain conditions determine the end of the game and the winner.
All games have a game loop. For example, in a chess game, you first set the pieces on the board and declare the current player. Then the game loop begins:
- The current player moves a piece, updating the game state.
- The checkmate condition is checked to determine whether the game is over.
- The current player is updated, and the loop starts again.
Real-world games blur the lines between the individual elements of a game. For example, moves in chess update both the game state and the game output. Despite this, the key elements of the game are always present. With them in mind, you can begin writing your first version of hangman in Python.
Prerequisites
The project that you’ll build in this tutorial will require familiarity with general Python programming. You should have basic knowledge of the following topics:
- Working with files and the
with
statement - Getting and processing the user’s input using the built-in
input()
function - Defining your own functions
- Writing
while
andfor
loops - Working with conditional statements
- Working with Python strings, lists, and sets
However, if you don’t have all this knowledge yet, then that’s okay! You might learn more by going ahead and giving the project a shot. You can always stop and review the resources linked here if you get stuck.
With this short overview of your hangman project and the prerequisites, you’re all set up to start Pythoning and having fun while coding.
Step 1: Set Up the Hangman Project
Your hangman game will select a word, handle user input, and display all output using a text-based user interface. You need code to handle each of these tasks. However, you’ll do everything using built-in and standard-library tools. You don’t need to install anything else.
For writing the game, you’ll use a single file called hangman.py
. This file will contain all the required code to handle the game’s operations. So, go ahead and create the file in your favorite code editor or IDE.
Next, go ahead and create a words.txt
file. This file will contain a list of words from which the game will select the target word. Click the collapsible section below to get the content of this file:
prettiest
close
dog
massive
hollow
cultured
seashore
explode
dizzy
minister
competent
thoughtful
harbor
tidy
dance
children
zesty
clean
ball
nostalgic
plan
week
strap
board
slope
bat
steep
mourn
cat
girl
ancient
street
mice
dare
wasteful
tub
limping
whimsical
eager
eggs
detail
experience
beds
train
place
cows
admit
rare
respect
loose
group
enjoy
internal
macabre
imported
superb
crooked
confused
hug
feigned
unkempt
coal
meddle
hapless
country
zealous
sick
pray
lake
tiny
key
empty
labored
delirious
ants
need
omniscient
onerous
damp
subtract
sack
connection
toad
gather
record
new
trashy
flow
river
sparkling
kneel
daughter
glue
allow
raspy
eminent
weak
wrong
pretend
receipt
celery
plain
fire
heal
damaging
honorable
foot
ignorant
substance
box
crime
giant
learned
itchy
smoke
likable
station
jaded
innocent
dead
straw
tray
chin
pack
geese
guess
wealthy
slippery
book
curly
swing
cure
flowers
rate
ignore
insidious
necessary
snakes
entertaining
rich
comb
lamentable
fuel
camera
multiply
army
exist
sulky
brief
worried
third
magical
wary
laborer
end
somber
authority
rainstorm
anxious
purpose
agreeable
spiky
toe
mixed
waiting
hungry
lopsided
flagrant
windy
ground
slap
please
white
hurry
governor
abandoned
reject
spiritual
abrasive
hunt
weather
endurable
hobbies
occur
bake
print
tire
juicy
blush
listen
trousers
daffy
scarecrow
rude
stem
bustling
nail
sneeze
bellicose
love
You can also download the complete source of your hangman game, including the words.txt
file, by clicking the link below:
Get Your Game: Click here to download the free code that you’ll write to create a hangman game with Python.
With this initial setup, you’re ready to start writing the code. Your first step will be to code a function for selecting the word to guess in your hangman game.
Step 2: Select a Word to Guess
The first step in playing hangman is to select the target word. When a human player selects a word for hangman, they pick one word from their own vocabulary. For the computer to select a word, it needs to have a vocabulary from which to select. Of course, its vocabulary doesn’t need to be as large as a human’s.
So, how would you select one word from your word list? Here’s a possible solution:
# hangman.py
from random import choice
def select_word():
with open("words.txt", mode="r") as words:
word_list = words.readlines()
return choice(word_list).strip()
In this code snippet, you create the select_word()
function to select the word to guess from the words.txt
file. You use a with
statement to open the file and the .readlines()
method to create a list of words.
Then, you use the random.choice()
function to choose a random word from the list. Notice that all the words in the file are written in lowercase letters. This detail will be important later when you need to compare the letters in the target words with the player’s input.
Here’s how your select_word()
works:
>>> from hangman import select_word
>>> select_word()
'toad'
>>> select_word()
'daffy'
>>> select_word()
'insidious'
Every time you call the function, you get a random word from your list of words. That’s the first step in playing the hangman game.
Creating a separate function to handle the vocabulary and word selection makes it easier to modify or expand the vocabulary later. It also makes your code more readable for others, or for yourself in a few months.
Step 3: Get and Validate the Player’s Input
Now, you need a way to get the player’s guesses at the command line. After all, a game isn’t much of a game if there isn’t some way for the player to influence the outcome.
In your hangman game, you have to get the player’s input and make sure that it’s valid. Remember when you created your list of words? All the words were in lowercase, so you should turn the player’s guesses into lowercase letters as well.
Additionally, the player shouldn’t be able to guess the same letter twice. It’d also be good to avoid numbers, special characters, and complete words as well.
So you need a two-part solution. The first part will gather the player’s input, and the second part will validate it. You can get the player’s input for the command line using the built-in input()
function:
# hangman.py
# ...
def get_player_input(guessed_letters):
while True:
player_input = input("Guess a letter: ").lower()
if _validate_input(player_input, guessed_letters):
return player_input
This is an example of an input validation loop, which you can use to restrict the user’s input. The loop iterates until the player provides valid data. In this case, that means a single letter that the player hasn’t already guessed.
You first set player_input
to the player’s input in lowercase. Then, you pass the guess to _validate_input()
along with the guessed_letters
set. Here’s the code for the _validate_input()
helper function:
# hangman.py
import string
# ...
def _validate_input(player_input, guessed_letters):
return (
len(player_input) == 1
and player_input in string.ascii_lowercase
and player_input not in guessed_letters
)
The _validate_input()
function performs three independent checks. It makes sure that:
- Exactly one character is entered.
- The input is a lowercase letter between
a
andz
, inclusive. - The input isn’t a letter that the player has already guessed.
All of these conditions must be true for the function to return True
. If any of those checks is false, then the function returns False
, and the input loop restarts. Here’s how this new code works:
>>> from hangman import get_player_input
>>> get_player_input({"a", "b"})
Guess a letter: 3
Guess a letter: e
'e'
>>> get_player_input({"a", "b"})
Guess a letter: a
Guess a letter: f
'f'
When you call get_player_input()
with a set containing previously guessed letters, you get a prompt that asks you to enter a letter. If you enter a non-letter character or a previous guess, then the function dismisses it because it’s not valid. Then, you get the prompt again. When you enter a valid letter, you get the letter back.
Now, you have a way to get and validate the player’s input. It’s time to decide when to end the game!
Step 4: Display the Guessed Letters and Word
Once you’ve selected the target word in a real-life game of hangman, you need to write an underscore, or blank, for each letter in the word. These blanks tell the players how many letters are in the target word. As players make guesses, you fill in the blanks with the correct letters. You should also keep track of incorrect letters, which you write to the side.
So, you now have two tasks:
- Display the letters that the player has guessed.
- Display the correct letters in their respective places in the word.
To track the letters that the player has guessed, you’ll use a Python set. Using a set allows you to efficiently check if the player has already guessed a given letter by using membership tests with the in
operator.
Showing the guessed letters takes advantage of the .join()
method of strings:
# hangman.py
# ...
def join_guessed_letters(guessed_letters):
return " ".join(sorted(guessed_letters))
The .join()
method builds a single string consisting of the members of the guessed_letters
set, separated by a whitespace character. The built-in sorted()
function allows you to sort the guessed letters alphabetically.
You use another function to build the word to show to the player:
# hangman.py
# ...
def build_guessed_word(target_word, guessed_letters):
current_letters = []
for letter in target_word:
if letter in guessed_letters:
current_letters.append(letter)
else:
current_letters.append("_")
return " ".join(current_letters)
This function uses a for
loop to build the current_letters
list. The loop looks at each letter in target_word
and determines whether the letter exists in guessed_letters
. If so, then your program adds letter
to current_letters
. If not, then you add an underscore (_
) to current_letters
. Then, you use the .join()
method to build a displayable word, which will include correct letters in their corresponding spots.
Go ahead and give these two functions a try. Remember that you should use a set of guessed letters and a target word.
Step 5: Draw the Hanged Man
Of course, there’s no hangman game without the actual hanged man, is there? You could simply print out the number of guesses the player has taken. But if you want to make the game look like hangman, then showing the hanged man is a good idea.
In this tutorial, you’ll build the hanged man using ASCII characters. Your solution consists of a list containing seven different strings. The first string will represent the scaffold, while the six remaining parts represent the body parts of the hanged man:
- Head
- Torso
- Right arm
- Left arm
- Right leg
- Left leg
You’ll use raw strings to build the different hangman states. A raw string requires an r
before the opening quote. These strings won’t interpret backslashes (\
) as special symbols, so you can use them in your illustration. Note that you’ll use the number of wrong guesses as an index to select which string to print.
Here’s the code that generates the hanged man:
# hangman.py
# ...
def draw_hanged_man(wrong_guesses):
hanged_man = [
r"""
-----
| |
|
|
|
|
|
|
|
|
-------
""",
r"""
-----
| |
O |
|
|
|
|
|
|
|
-------
""",
r"""
-----
| |
O |
--- |
| |
| |
|
|
|
|
-------
""",
r"""
-----
| |
O |
--- |
/ | |
| |
|
|
|
|
-------
""",
r"""
-----
| |
O |
--- |
/ | \ |
| |
|
|
|
|
-------
""",
r"""
-----
| |
O |
--- |
/ | \ |
| |
--- |
/ |
| |
|
-------
""",
r"""
-----
| |
O |
--- |
/ | \ |
| |
--- |
/ \ |
| | |
|
-------
""",
]
print(hanged_man[wrong_guesses])
During the game, you track the number of incorrect guesses that the player has made, and then you pass that into the draw_hanged_man()
function to print the appropriate hangman state using ASCII characters.
Here’s how this function works in practice:
>>> from hangman import draw_hanged_man
>>> draw_hanged_man(0)
-----
| |
|
|
|
|
|
|
|
|
-------
>>> draw_hanged_man(6)
-----
| |
O |
--- |
/ | \ |
| |
--- |
/ \ |
| | |
|
-------
When you call draw_hanged_man()
with an integer index as an argument, you get an ASCII drawing that represents the current stage in your hangman game. That looks nice, doesn’t it?
Step 6: Figure Out When the Game Is Over
Games normally end due to a condition set up by the player’s input. Perhaps the player has finally reached the goal, or they failed some task and lost the game.
Your hangman game ends when one of two events happens:
- The player makes six incorrect guesses.
- The player guesses the word correctly.
Both of these outcomes stem from the player’s input. So, it makes sense to check for them in the game loop, which is where you gather, validate, and process all player input. Encapsulating these checks into a function is a good idea as well.
Since there are two conditions to check, you need to pass the game_over()
function all the necessary information to check for both. It needs the number of taken guesses, the current word, and the guessed letters:
# hangman.py
# ...
MAX_INCORRECT_GUESSES = 6
# ...
def game_over(wrong_guesses, target_word, guessed_letters):
if wrong_guesses == MAX_INCORRECT_GUESSES:
return True
if set(target_word) <= guessed_letters:
return True
return False
Checking for the number of wrong guesses is straightforward, but checking if the player has correctly guessed the word might need a little explanation.
The built-in set()
function turns any iterable into a set
object. Because every item in a set is unique, this creates a set consisting of the letters that make up target_word
. Then, you compare this set to letters_guessed
using the <=
operator, which checks if every item in the left-hand set is a member of the right-hand set. If so, then the player has guessed all the letters.
Note: You used multiple if
statements in game_over()
for readability. You could also write this function as follows:
# hangman.py
# ...
def game_over(guesses_taken, target_word, letters_guessed):
return (
guesses_taken == MAX_INCORRECT_GUESSES
or set(target_word) <= letters_guessed
)
Both implementations work equally well. Deciding which one to use is up to you and your personal taste.
Now that you have all the ingredients in place, it’s time to bake the cake!
Step 7: Run the Game Loop
Up to this point, you’ve assembled the functions and code that cover most of the important parts of your hangman game. Those parts include the following:
- Selecting a random word to guess
- Gathering and processing the player’s input
- Showing the word with unguessed letters hidden
- Showing the hanged man drawing
- Tracking the letters guessed and guesses taken
- Checking if the game is over
The last task is to put them together into a whole game. You’ll need a game loop to control the game’s evolution. However, not everything goes into the loop. Before the loop starts, you need to set up the game to play. After the loop is over, you need to end the game cleanly.
The initial setup includes providing an initial game state. To determine the initial state, you need to pick the word to guess, the input letters, the mystery word in its current form, and the number of wrong guesses. Once you’ve set these variables, then you can invite the player to play:
# hangman.py
# ...
if __name__ == "__main__":
# Initial setup
target_word = select_word()
guessed_letters = set()
guessed_word = build_guessed_word(target_word, guessed_letters)
wrong_guesses = 0
print("Welcome to Hangman!")
With the game set up, you can begin the game loop. Remember, the game loop doesn’t end until the player either guesses the word or runs out of guesses.
Every time the loop iterates, there are several tasks to do:
- Checking whether the game is over
- Showing the hanged man drawing
- Showing the guessed word or target word
- Showing the guessed letters
- Getting a letter guess from the player
- Checking whether the input letter is in the target word
- Updating the displayed word and guessed letters
Here’s one way to write the game loop:
# hangman.py
# ...
if __name__ == "__main__":
# ...
# Game loop
while not game_over(wrong_guesses, target_word, guessed_letters):
draw_hanged_man(wrong_guesses)
print(f"Your word is: {guessed_word}")
print(
"Current guessed letters: "
f"{join_guessed_letters(guessed_letters)}\n"
)
player_guess = get_player_input(guessed_letters)
if player_guess in target_word:
print("Great guess!")
else:
print("Sorry, it's not there.")
wrong_guesses += 1
guessed_letters.add(player_guess)
guessed_word = build_guessed_word(target_word, guessed_letters)
The loop doesn’t end until the game is over, so you can use the game_over()
function to control the loop. Inside the loop, you start by showing the initial hangman drawing. Next, you display the target word and, finally, the guessed letters.
Then, you get and validate the player’s input. In the conditional statement, you then check whether the player’s guess is in the target word. If that’s the case, then you print a success message. Otherwise, you print a failure message and increment the wrong_guesses
counter by 1
.
Finally, you add the current guess to guessed_letters
and build an updated word using the build_guessed_word()
function.
When the game is over and the game loop finishes, you have some final tasks to handle:
# hangman.py
# ...
if __name__ == "__main__":
# ...
# Game over
draw_hanged_man(wrong_guesses)
if wrong_guesses == MAX_INCORRECT_GUESSES:
print("Sorry, you lost!")
else:
print("Congrats! You did it!")
print(f"Your word was: {target_word}")
First, you call draw_hanged_man()
to show the final hanged man drawing. This is necessary because you only show the hanged man at the top of the game loop. Therefore, the final iteration doesn’t get to update the drawing.
At this point, the player either ran out of guesses and lost, or guessed the word correctly and won. You can tell by inspecting the final number of wrong guesses and printing out the appropriate message.
Now, your hangman game is ready for the first match. Go ahead and run the game from your command line:
$ python hangman.py
Welcome to Hangman!
-----
| |
|
|
|
|
|
|
|
|
-------
Your word is: _ _ _ _ _
Current guessed letters:
Guess a letter: e
Sorry, it's not there.
-----
| |
O |
|
|
|
|
|
|
|
-------
Your word is: _ _ _ _ _
Current guessed letters: e
Guess a letter:
Wow! Your hangman game works as expected! You’re all set up to start using the game for fun and practice. You can download the complete source for this hangman implementation by clicking the link below:
Get Your Game: Click here to download the free code that you’ll write to create a hangman game with Python.
Conclusion
Building the hangman game in Python is a great way to grow your programming skills, learn new techniques, and introduce yourself to the world of computer games. By writing the game for your command line in Python, you’ve participated in several coding and design challenges, including taking the user’s input, processing it, and displaying user-friendly outputs.
In this tutorial, you’ve learned how to:
- Structure a computer game with different elements
- Track the state of your game
- Get and process the user’s input
- Create a text-based user interface (TUI) for your game
- Determine whether the game is over and who won
You now have the tools to add to your hangman game or go off and conquer other similar games.
Next Steps
Now that you’ve finished building your hangman game, you can go a step further and continue improving the game. Alternatively, you can change gears and jump into other cool projects. Here are some great next steps for you to continue learning Python and building projects:
- Build a Wordle Clone With Python and Rich: In this step-by-step project, you’ll build your own Wordle clone with Python. Your game will run in the terminal, and you’ll use Rich to ensure your word-guessing app looks good. Learn how to build a command-line application from scratch and then challenge your friends to a wordly competition!
- Build a Quiz Application With Python: In this step-by-step project, you’ll build a Python quiz application for the terminal. Your app will ask you multiple-choice questions that you can use to strengthen your own knowledge or challenge your friends to test theirs.
- Build a Dice-Rolling Application With Python: In this step-by-step project, you’ll build a dice-rolling simulator app with a minimal text-based user interface using Python. The app will simulate the rolling of up to six dice. Each individual die will have six sides.
- Raining Outside? Build a Weather CLI App With Python: In this tutorial, you’ll write a nicely formatted Python CLI app that displays information about the current weather in any city that you provide the name for.
- Build a Python Directory Tree Generator for the Command Line: In this step-by-step project, you’ll create a Python directory tree generator application for your command line. You’ll code the command-line interface with
argparse
and traverse the file system usingpathlib
.