If you’re curious about web development, then you’ve likely encountered the abbreviation MVC, which stands for Model-View-Controller. You may know that it’s a common design pattern that’s fundamental to many Python web frameworks and even desktop applications.
But what exactly does it mean? If you’ve had a hard time wrapping your head around the concept, then keep on reading.
In this tutorial, you’ll:
- Approach understanding the MVC pattern through a Lego-based analogy
- Learn what models, views, and controllers are conceptually
- Tie your conceptual understanding back to concrete web development examples
- Investigate Flask code snippets to drive the point home
Maybe you built things with Lego as a kid, or maybe you’re still a Lego-aficionado today. But even if you’ve never pieced two Lego blocks together, keep on reading because the analogy might still be a good building block for your understanding.
Get Your Code: Click here to download an example Flask app that will help you understand MVC in Python web apps.
Take the Quiz: Test your knowledge with our interactive “Model-View-Controller (MVC) in Python Web Apps: Explained With Lego” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Model-View-Controller (MVC) in Python Web Apps: Explained With LegoIn this quiz, you'll test your understanding of the Model-View-Controller (MVC) design pattern, a fundamental concept in many Python web frameworks. By working through this quiz, you'll revisit the concepts of Models, Views, and Controllers, and how they relate to concrete web development examples.
Explaining the Model-View-Controller Pattern With Lego
Imagine that you’re ten years old and sitting on your family room floor. In front of you is a big bucket of Lego, or similar modular building blocks. There are blocks of all different shapes and sizes:
- 🟦🟦🟦 Some are blue, tall, and long.
- 🟥 Some are red and cube-shaped.
- 🟨🟨 Some are yellow, big, and wide.
With all of these different Lego pieces, there’s no telling what you could build!
Just as your mind is filling with the endless possibilities, you hear something coming from the direction of the couch. It’s your older brother, voicing a specific request. He’s saying, “Hey! Build me a spaceship!”
“Alright,” you think, “that could actually be pretty cool.” A spaceship it is!
So you get to work. You start pulling out the Lego blocks that you think you’re going to need. Some big, some small. Different colors for the outside of the spaceship, different colors for the engines.
Now that you have all of your building blocks in place, it’s time to assemble the spaceship. And after a few hours of hard work, you now have in front of you—a spaceship:
🟦
🟦🟥🟦
🟦🟥🟥🟥🟦
🟦🟥🟥🟥🟥🟥🟦
🟦🟥🟥🟥🟥🟥🟦
🟦🟥🟩🟩🟩🟥🟦
🟦🟥🟩🟦🟩🟥🟦
🟦🟥🟩🟩🟩🟥🟦
🟦🟥🟥🟥🟥🟥🟦
🟦🟥🟥🟥🟥🟥🟦
🟦🟥🟥🟥🟥🟥🟦
🟦🟥🟥🟥🟥🟥🟥🟥🟥🟥🟦
🟦🟥🟥🟥🟥🟥🟥🟥🟥🟥🟦
🟦🟥🟨🟨🟥🟥🟥🟨🟨🟥🟦
🟨🟨 🟨🟨
You run to find your brother and show him the finished product. “Wow, nice work!”, he says. Then he quietly adds:
Huh, I just asked for that a few hours ago, I didn’t have to do a thing, and here it is. I wish everything was that easy.
— Your Brother
What if I told you that building a web application using the MVC pattern is exactly like building something with Lego blocks?
User Sends a Request
In the case of the Lego spaceship, it was your brother who made a request and asked you to build something. In the case of a web app, it’s a user entering a URL and requesting to view a certain page.
Controller Interprets the Request
When you built the Lego spaceship for your brother, you were the controller. In a web app, the controller is the code that you write.
The controller is responsible for understanding the request and taking actions based on it. It’s the controller that gathers all of the necessary building blocks and organizes them appropriately.
Models Make the Products
The different types of Lego blocks are the models. You have different sizes and shapes, and you grab the ones that you need to build the spaceship. In a web app, models help the controller retrieve all of the information needed from the database to make the products.
Once the controller uses the models to retrieve the necessary items, everything needed is in place to assemble the final product.
View Represents the Final Product
In the Lego example, the spaceship is the view. It’s the final product that your brother—the person who made the request—gets to see.
In a web application, the view is the page that the user sees in their browser.
Summarizing Your Lego Adventure
You’ve built a spaceship, impressed your brother, and connected the process to building web applications using the MVC pattern. Here’s a quick recap that you can keep handy to help you remember how this story relates back to web development.
When Building With Lego:
- Your brother makes the request that you build a spaceship.
- You receive the request.
- You retrieve and organize all of the Lego blocks that you need to construct the spaceship.
- You use the blocks to build the spaceship and present the finished spaceship to your brother.
These are generic steps. You can repeat them to construct Lego builds of many different shapes and colors.
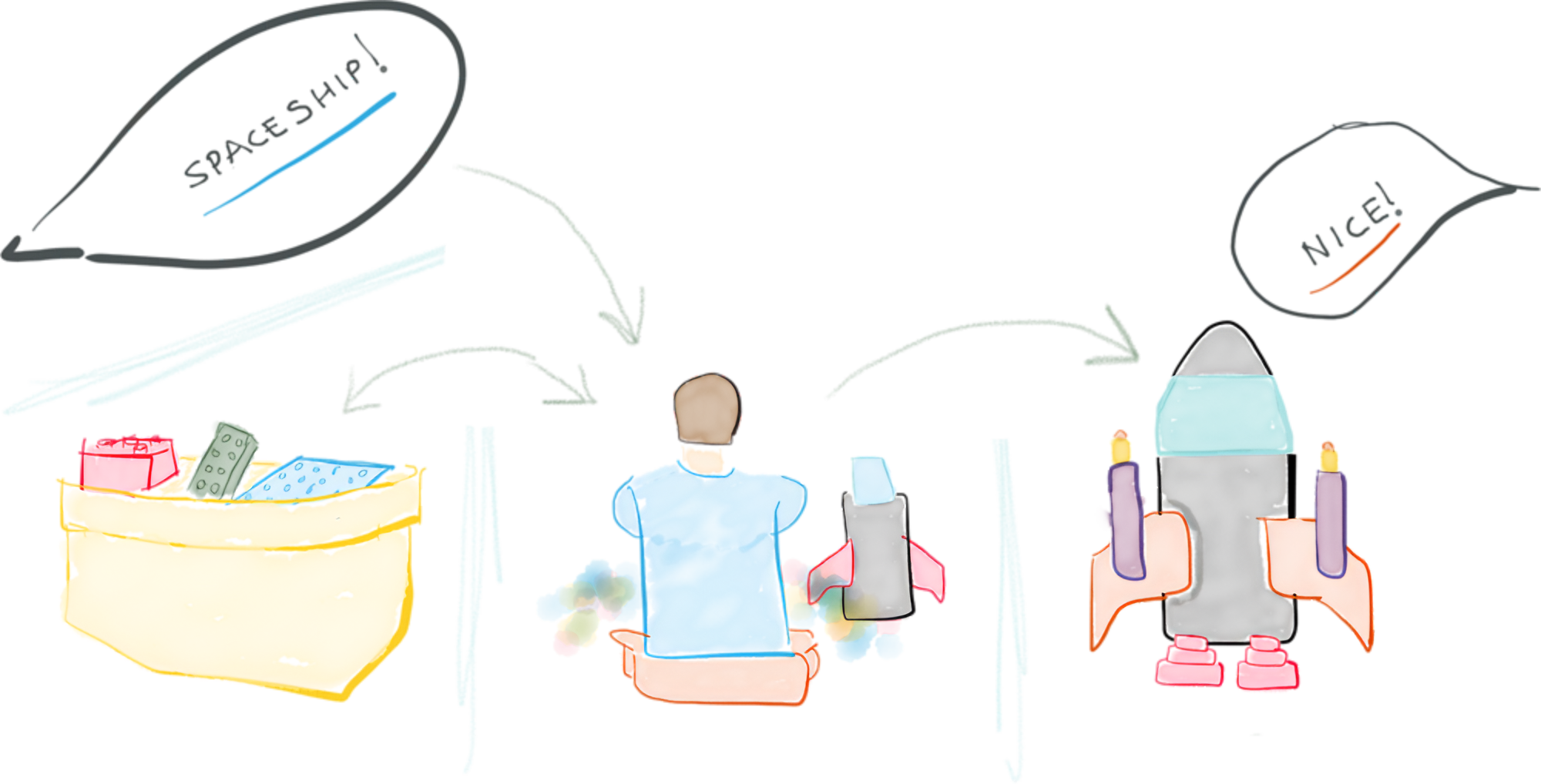
With this gently watercolored image etched into your brain, you can now revisit the web app version of the process to compare them.
When Building a Web App:
- A user requests to view a page by entering a URL.
- The controller receives that request.
- The controller uses the models to retrieve all of the necessary data, organizes it, and sends it off to the view.
- The view uses the data it receives to render the final webpage and presents it to the the user in their browser.
Again, these are generic steps that your web app does many times over to serve all sorts of information and pages to your users:
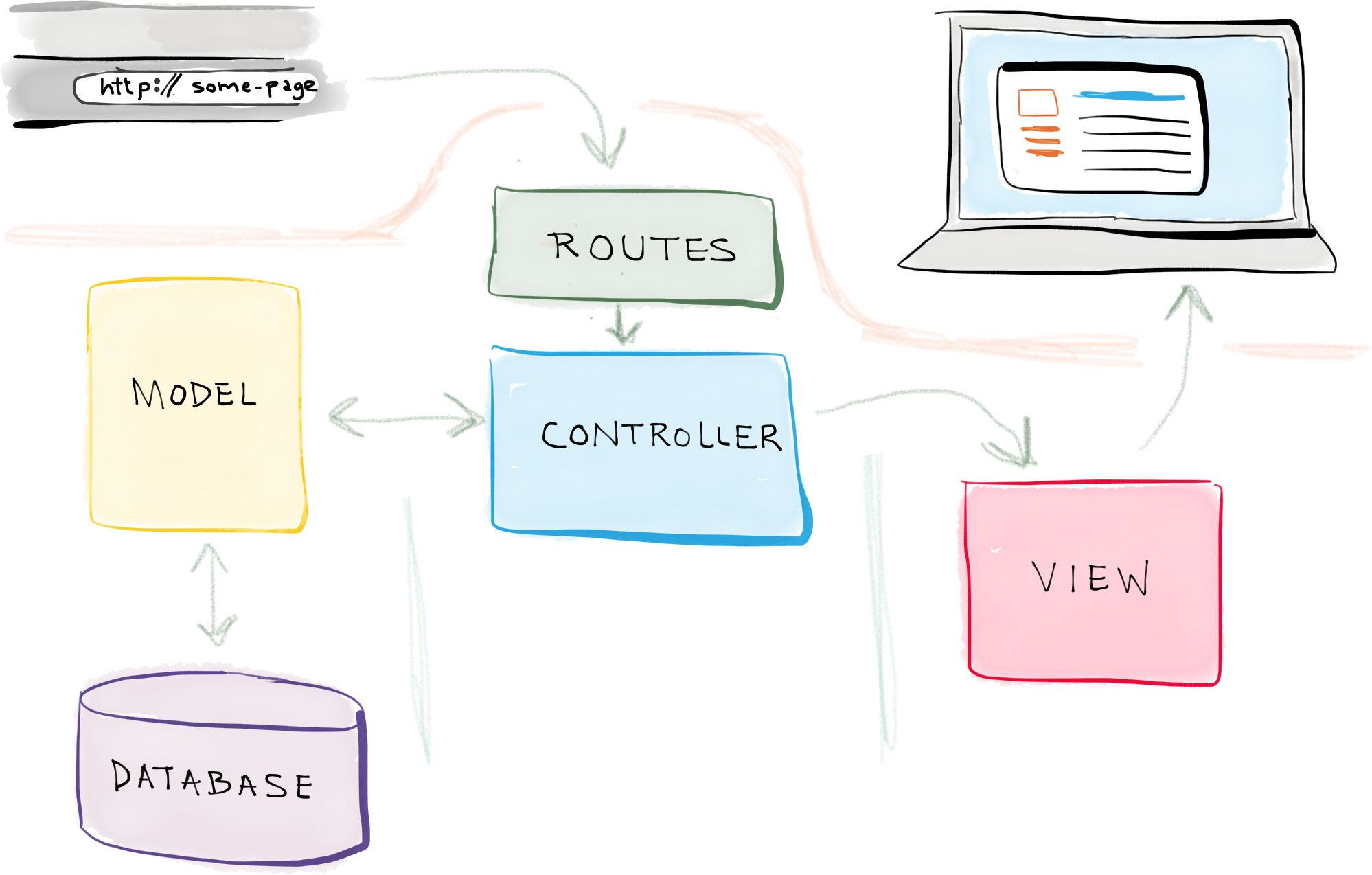
If you mentally store this second image next to the first one, then you’ll have a complete analogy that you can refer back to whenever you want to remember what the MVC pattern is all about.
Here’s another overview, summarized in a table:
Lego | Website | |
---|---|---|
Wish | Brother’s request | URL request |
Execution | You | Controller |
Building blocks | Lego | Models |
Presentable product | Spaceship | View |
Nice work! Now that you’ve painted this analogy into your memory, you’ve built a solid foundation for understanding the Model-View-Controller pattern. Next, you can go a little deeper and consider what it all means from a more technical perspective, focusing on Python web development.
Exploring the Model-View-Controller Pattern in Python Web Development
When you type a URL in your browser to access a web application, you’re making a request to view a certain page within the application. But how does the application know which page to render and display?
When building a web app, you define what are known as routes. Essentially, routes are URL patterns associated with different pages. So when someone enters a URL, the application tries to match that URL to one of the predefined routes behind the scenes.
So, there are really four major components at play here: controllers, models, views, and routes.
Routing Requests
Each route is associated with a controller. More specifically, it’s associated with a certain function within a controller, known as a controller action. So when you enter a URL, the application attempts to find a matching route. If it’s successful, then it calls that route’s associated controller action.
You can start learning how the Model-View-Controller pattern works in Python web development by considering a basic Flask route as an example:
app.py
# ...
@app.route("/")
def home():
pass
Here, you establish that the base route ("/"
) is associated with the home()
view function, which conceptually is a controller action. When someone requests that base route, then your web app will call home()
.
Coding Models and Controllers
Within the controller action, you typically do two main things:
- You retrieve all of the necessary data from a database using the models.
- You pass that data to a view, which renders the requested page.
You generally add the data that you retrieve via the models to a data structure, like a list or dictionary. Then, you send that data structure to the view.
Go ahead and expand your Flask example app with these functionalities:
app.py
1# ...
2
3@app.route("/")
4def home():
5 """Searches the database for entries, then displays them."""
6 db = get_db()
7 cur = db.execute("SELECT * FROM entries ORDER BY id DESC;")
8 entries = cur.fetchall()
9 return render_template("index.html", entries=entries)
In your updated view function, you fetch data from the database in lines 6 to 8. The get_db()
function that you see in this code snippet is a placeholder for a database connector function. You’ll need to write the code for this function so that it works with the database that you use—for example, a Python SQL library.
Note: The code in these examples conceptually explains the process of a Flask app, but it doesn’t quite work like this in isolation. If you download the materials repo, then you’ll get a fully functioning Flask example app that uses the same code, but also adds the necessary Flask boilerplate code and a script to construct a SQLite database that you can interact with.
In line 8, you end up with a list, which you assign to the variable entries
. Then in line 9, you send that list to the index.html
template and make the data accessible through a variable that you also call entries
.
Building the Views
Finally, the index.html
file represents your view. Because you used the controller action to send the data structure to the template file, you can now access the data in there. You can then work with the information contained within the data structure to render the HTML content of the page that the user ultimately sees in their browser.
In your Flask app example, you can loop through entries
and display each one using the Jinja syntax:
templates/index.html
1...
2
3<ul>
4 {% for entry in entries %}
5 <li>
6 <strong>{{ entry[1] }}:</strong>
7 {{ entry[2] }}
8 </li>
9 {% else %}
10 <li>No entries yet. Add some!</li>
11 {% endfor %}
12</ul>
13
14...
If you have entries in your database, then your user will get to see all the entries listed one after another. You also added an optional else
clause in line 9, which renders a descriptive text message if there aren’t any entries yet.
Summarizing the Model-View-Controller Pattern
Now you’ve done a second lap around the concepts of the Model-View-Controller pattern, this time using Python’s Flask web framework as a guide rail. Here’s a more detailed, technical summary of the MVC request process:
- A user requests to view a page by entering a URL.
- The application matches the URL to a predefined route.
- The application calls the controller action associated with the route.
- The controller action uses the models to retrieve all of the necessary data from a database, places the data in a data structure, and loads a view, passing along the data structure.
- The view accesses the data structure and uses it to render the requested page, which the application then presents to the user in their browser.
It’s not quite the same as building a Lego spaceship for your brother, but maybe you can see the conceptual similarities in the two processes.
If you want to play with the example Flask app and explore the additional code that’s necessary to run it, then you can download the code here:
Get Your Code: Click here to download an example Flask app that will help you understand MVC in Python web apps.
Conclusion
It’s time to come back from time-traveling to your Lego-wielding past. You took a trip to gather memories and analogies, and applied them to improve your understanding of the Model-View-Controller (MVC) pattern for creating Python web apps.
In this tutorial, you learned how to:
- Build a spaceship for your brother
- Conceptualize models, views, and controllers in web applications
- Relate the MVC concepts to concrete code examples in Flask
Do you have another analogy that you like to use to remember what the MVC pattern is all about? Share your thoughts in the comments below!
Take the Quiz: Test your knowledge with our interactive “Model-View-Controller (MVC) in Python Web Apps: Explained With Lego” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Model-View-Controller (MVC) in Python Web Apps: Explained With LegoIn this quiz, you'll test your understanding of the Model-View-Controller (MVC) design pattern, a fundamental concept in many Python web frameworks. By working through this quiz, you'll revisit the concepts of Models, Views, and Controllers, and how they relate to concrete web development examples.